Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / security / system / security / authentication / ExtendedProtection / ExtendedProtectionPolicyTypeConverter.cs / 1305376 / ExtendedProtectionPolicyTypeConverter.cs
//------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; namespace System.Security.Authentication.ExtendedProtection { public class ExtendedProtectionPolicyTypeConverter : TypeConverter { public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { ExtendedProtectionPolicy policy = value as ExtendedProtectionPolicy; if (policy != null) { Type[] parameterTypes; object[] parameterValues; if (policy.PolicyEnforcement == PolicyEnforcement.Never) { parameterTypes = new Type[] { typeof(PolicyEnforcement) }; parameterValues = new object[] { PolicyEnforcement.Never }; } else { parameterTypes = new Type[] { typeof(PolicyEnforcement), typeof(ProtectionScenario), typeof(ICollection) }; object[] customServiceNames = null; if (policy.CustomServiceNames != null && policy.CustomServiceNames.Count > 0) { customServiceNames = new object[policy.CustomServiceNames.Count]; ((ICollection)policy.CustomServiceNames).CopyTo(customServiceNames, 0); } parameterValues = new object[] { policy.PolicyEnforcement, policy.ProtectionScenario, customServiceNames }; } ConstructorInfo constructor = typeof(ExtendedProtectionPolicy).GetConstructor(parameterTypes); return new InstanceDescriptor(constructor, parameterValues); } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
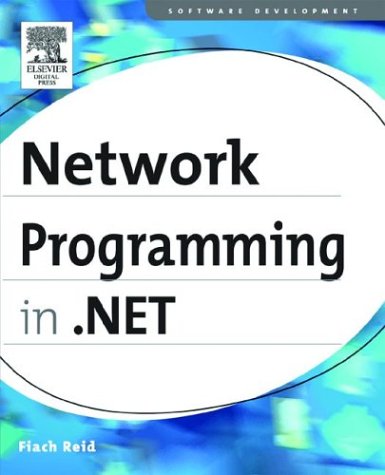
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentApplication.cs
- XmlProcessingInstruction.cs
- InputScopeConverter.cs
- Predicate.cs
- SuspendDesigner.cs
- DataTemplateSelector.cs
- SessionSymmetricMessageSecurityProtocolFactory.cs
- XmlnsDefinitionAttribute.cs
- compensatingcollection.cs
- AbandonedMutexException.cs
- BrowserCapabilitiesCodeGenerator.cs
- StackSpiller.Bindings.cs
- CfgParser.cs
- TextOptions.cs
- UriTemplateDispatchFormatter.cs
- BamlRecordHelper.cs
- ColumnMapVisitor.cs
- HashMembershipCondition.cs
- ResourceReader.cs
- OleDbMetaDataFactory.cs
- ViewKeyConstraint.cs
- AuthStoreRoleProvider.cs
- ProtocolReflector.cs
- ConnectionStringsExpressionBuilder.cs
- RowUpdatedEventArgs.cs
- OperationFormatStyle.cs
- PathStreamGeometryContext.cs
- DrawingImage.cs
- XsdBuildProvider.cs
- TypedTableBaseExtensions.cs
- ProtectedConfigurationSection.cs
- VariableQuery.cs
- MsmqOutputMessage.cs
- ScaleTransform.cs
- RequestQueryProcessor.cs
- wmiutil.cs
- CornerRadius.cs
- PlatformCulture.cs
- URLMembershipCondition.cs
- CompModSwitches.cs
- ViewManager.cs
- PerformanceCounterCategory.cs
- TCPClient.cs
- EpmContentSerializerBase.cs
- TextBoxAutomationPeer.cs
- GrammarBuilderPhrase.cs
- SQLBytesStorage.cs
- QilChoice.cs
- DataGridViewSelectedRowCollection.cs
- OleDbSchemaGuid.cs
- DefaultAuthorizationContext.cs
- SymmetricAlgorithm.cs
- TemplatePartAttribute.cs
- ReturnEventArgs.cs
- BamlRecords.cs
- TypeConverters.cs
- MasterPageBuildProvider.cs
- DesignerVerb.cs
- ArrayExtension.cs
- OSEnvironmentHelper.cs
- SqlCommandBuilder.cs
- TypeExtensionConverter.cs
- ManualResetEvent.cs
- DataGridItem.cs
- ReadOnlyTernaryTree.cs
- XmlSyndicationContent.cs
- TaskFactory.cs
- DataSourceView.cs
- ExpandCollapsePattern.cs
- LinqDataSourceStatusEventArgs.cs
- MatrixCamera.cs
- HttpContext.cs
- lengthconverter.cs
- VScrollProperties.cs
- MatrixAnimationUsingKeyFrames.cs
- EmptyEnumerator.cs
- SBCSCodePageEncoding.cs
- PropertyMapper.cs
- WebPartConnectionsDisconnectVerb.cs
- ClientSideQueueItem.cs
- FileCodeGroup.cs
- Listbox.cs
- ExpressionBuilder.cs
- HttpCachePolicy.cs
- GroupByExpressionRewriter.cs
- PeerUnsafeNativeMethods.cs
- RenderDataDrawingContext.cs
- ProxyHwnd.cs
- ProofTokenCryptoHandle.cs
- iisPickupDirectory.cs
- CodeIterationStatement.cs
- TextDpi.cs
- Misc.cs
- BackgroundFormatInfo.cs
- ElementsClipboardData.cs
- MonthCalendarDesigner.cs
- FilterInvalidBodyAccessException.cs
- ListCollectionView.cs
- FeatureSupport.cs
- CalculatedColumn.cs