Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataSet / System / Data / TypedTableBaseExtensions.cs / 1 / TypedTableBaseExtensions.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Linq.Expressions; using System.Globalization; using System.Diagnostics; namespace System.Data { ////// This static class defines the extension methods that add LINQ operator functionality /// within IEnumerableDT and IOrderedEnumerableDT. /// public static class TypedTableBaseExtensions { ////// LINQ's Where operator for generic EnumerableRowCollection. /// public static EnumerableRowCollectionWhere ( this TypedTableBase source, Func predicate) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); EnumerableRowCollection erc = new EnumerableRowCollection ((DataTable)source); return erc.Where (predicate); } /// /// LINQ's OrderBy operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderBy ( this TypedTableBase source, Func keySelector) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); EnumerableRowCollection erc = new EnumerableRowCollection ((DataTable)source); return erc.OrderBy (keySelector); } /// /// LINQ's OrderBy operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderBy ( this TypedTableBase source, Func keySelector, IComparer comparer) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); EnumerableRowCollection erc = new EnumerableRowCollection ((DataTable)source); return erc.OrderBy (keySelector, comparer); } /// /// LINQ's OrderByDescending operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderByDescending ( this TypedTableBase source, Func keySelector) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); EnumerableRowCollection erc = new EnumerableRowCollection ((DataTable)source); return erc.OrderByDescending (keySelector); } /// /// LINQ's OrderByDescending operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderByDescending ( this TypedTableBase source, Func keySelector, IComparer comparer) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); EnumerableRowCollection erc = new EnumerableRowCollection ((DataTable)source); return erc.OrderByDescending (keySelector, comparer); } /// /// Executes a Select (Projection) on EnumerableDataTable. If the selector returns a different /// type than the type of rows, then AsLinqDataView is disabled, and the returning EnumerableDataTable /// represents an enumerable over the LINQ Query. /// public static EnumerableRowCollectionSelect( this TypedTableBase source, Func selector) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); EnumerableRowCollection erc = new EnumerableRowCollection ((DataTable)source); return erc.Select (selector); } /// /// This method returns a IEnumerable of TRow. /// /// /// The source DataTable to make enumerable. /// ////// IEnumerable of datarows. /// public static EnumerableRowCollectionAsEnumerable (this TypedTableBase source) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); return new EnumerableRowCollection (source as DataTable); } } //end class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Linq.Expressions; using System.Globalization; using System.Diagnostics; namespace System.Data { ////// This static class defines the extension methods that add LINQ operator functionality /// within IEnumerableDT and IOrderedEnumerableDT. /// public static class TypedTableBaseExtensions { ////// LINQ's Where operator for generic EnumerableRowCollection. /// public static EnumerableRowCollectionWhere ( this TypedTableBase source, Func predicate) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); EnumerableRowCollection erc = new EnumerableRowCollection ((DataTable)source); return erc.Where (predicate); } /// /// LINQ's OrderBy operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderBy ( this TypedTableBase source, Func keySelector) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); EnumerableRowCollection erc = new EnumerableRowCollection ((DataTable)source); return erc.OrderBy (keySelector); } /// /// LINQ's OrderBy operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderBy ( this TypedTableBase source, Func keySelector, IComparer comparer) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); EnumerableRowCollection erc = new EnumerableRowCollection ((DataTable)source); return erc.OrderBy (keySelector, comparer); } /// /// LINQ's OrderByDescending operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderByDescending ( this TypedTableBase source, Func keySelector) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); EnumerableRowCollection erc = new EnumerableRowCollection ((DataTable)source); return erc.OrderByDescending (keySelector); } /// /// LINQ's OrderByDescending operator for generic EnumerableRowCollection. /// public static OrderedEnumerableRowCollectionOrderByDescending ( this TypedTableBase source, Func keySelector, IComparer comparer) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); EnumerableRowCollection erc = new EnumerableRowCollection ((DataTable)source); return erc.OrderByDescending (keySelector, comparer); } /// /// Executes a Select (Projection) on EnumerableDataTable. If the selector returns a different /// type than the type of rows, then AsLinqDataView is disabled, and the returning EnumerableDataTable /// represents an enumerable over the LINQ Query. /// public static EnumerableRowCollectionSelect( this TypedTableBase source, Func selector) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); EnumerableRowCollection erc = new EnumerableRowCollection ((DataTable)source); return erc.Select (selector); } /// /// This method returns a IEnumerable of TRow. /// /// /// The source DataTable to make enumerable. /// ////// IEnumerable of datarows. /// public static EnumerableRowCollectionAsEnumerable (this TypedTableBase source) where TRow : DataRow { DataSetUtil.CheckArgumentNull(source, "source"); return new EnumerableRowCollection (source as DataTable); } } //end class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
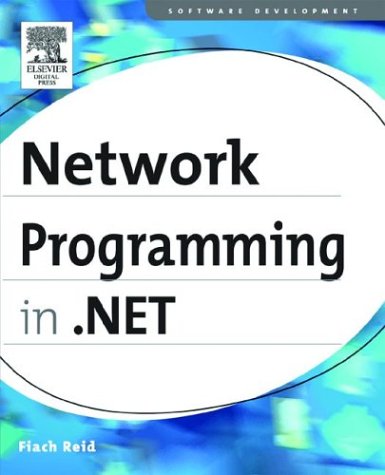
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SeekableReadStream.cs
- LinkLabelLinkClickedEvent.cs
- XamlInt32CollectionSerializer.cs
- XPathNodeInfoAtom.cs
- FileDialogCustomPlace.cs
- dataSvcMapFileLoader.cs
- CodeTypeMember.cs
- CollectionViewSource.cs
- _DisconnectOverlappedAsyncResult.cs
- StartUpEventArgs.cs
- SoapSchemaMember.cs
- Volatile.cs
- InlineUIContainer.cs
- PersonalizationStateQuery.cs
- Control.cs
- SHA512CryptoServiceProvider.cs
- SettingsSavedEventArgs.cs
- MemoryFailPoint.cs
- WebConfigurationHostFileChange.cs
- XmlSchemaValidationException.cs
- nulltextnavigator.cs
- DPTypeDescriptorContext.cs
- CriticalFinalizerObject.cs
- InheritedPropertyChangedEventArgs.cs
- RequestQueryParser.cs
- XPathItem.cs
- ExpressionBindingCollection.cs
- InternalResources.cs
- UniqueConstraint.cs
- DesignerDataParameter.cs
- CodeArrayIndexerExpression.cs
- IconHelper.cs
- GenerateDerivedKeyRequest.cs
- CollectionChange.cs
- ObjectTag.cs
- CodePageUtils.cs
- InkCanvasAutomationPeer.cs
- ResourceDisplayNameAttribute.cs
- CodeCompileUnit.cs
- Comparer.cs
- WCFBuildProvider.cs
- Mappings.cs
- UndoManager.cs
- XPathDocumentIterator.cs
- Rect3D.cs
- WS2007FederationHttpBinding.cs
- StylusOverProperty.cs
- XmlSchemas.cs
- CodeAttributeDeclaration.cs
- HttpNamespaceReservationInstallComponent.cs
- BooleanSwitch.cs
- ReferenceSchema.cs
- DynamicQueryableWrapper.cs
- ParagraphResult.cs
- AutoResetEvent.cs
- SortQueryOperator.cs
- SecurityKeyIdentifierClause.cs
- CurrentChangingEventManager.cs
- SortDescription.cs
- TcpStreams.cs
- M3DUtil.cs
- NumericUpDownAccelerationCollection.cs
- IPHostEntry.cs
- RelationshipDetailsRow.cs
- XpsLiterals.cs
- ChunkedMemoryStream.cs
- ServiceX509SecurityTokenProvider.cs
- WebBrowserContainer.cs
- StorageEntityContainerMapping.cs
- DataServiceClientException.cs
- SharedUtils.cs
- DateTimeOffset.cs
- IndexedString.cs
- StorageAssociationTypeMapping.cs
- FtpWebResponse.cs
- HitTestResult.cs
- LinkedList.cs
- DirectionalLight.cs
- NavigationWindow.cs
- InkCanvasSelection.cs
- ToolBarPanel.cs
- ListItemCollection.cs
- SafeLibraryHandle.cs
- Journal.cs
- ErrorFormatterPage.cs
- PropertyValueUIItem.cs
- DocumentCollection.cs
- ReadOnlyDataSource.cs
- ClientSession.cs
- MimeObjectFactory.cs
- HScrollBar.cs
- DurableTimerExtension.cs
- SystemIPInterfaceStatistics.cs
- EdmItemCollection.cs
- CustomTypeDescriptor.cs
- InlineObject.cs
- ByteAnimation.cs
- ComplusTypeValidator.cs
- ValidationVisibilityAttribute.cs
- _NTAuthentication.cs