Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Shared / MS / Internal / IO / SynchronizingStream.cs / 1305600 / SynchronizingStream.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: SynchronizingStream.cs // // Description: Stream that locks on given syncRoot before entering any public API's. // // History: 01/20/06 - brucemac - created //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.IO; using System.Windows; namespace MS.Internal.IO.Packaging { ////// Wrap returned stream to protect non-thread-safe API's from race conditions /// internal class SynchronizingStream : Stream { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Serializes access by locking on the given object /// /// stream to read from (baseStream) /// object to lock on internal SynchronizingStream(Stream stream, Object syncRoot) { if (stream == null) throw new ArgumentNullException("stream"); if (syncRoot == null) throw new ArgumentNullException("syncRoot"); _baseStream = stream; _syncRoot = syncRoot; } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// Return the bytes requested /// /// destination buffer /// offset to write into that buffer /// how many bytes requested ///how many bytes were written into buffer public override int Read(byte[] buffer, int offset, int count) { lock (_syncRoot) { CheckDisposed(); return (_baseStream.Read(buffer, offset, count)); } } ////// Read a single byte /// ///The unsigned byte cast to an Int32, or -1 if at the end of the stream. public override int ReadByte() { lock (_syncRoot) { CheckDisposed(); return (_baseStream.ReadByte()); } } ////// Write a single byte /// /// byte to write public override void WriteByte(byte b) { lock (_syncRoot) { CheckDisposed(); _baseStream.WriteByte(b); } } ////// Seek /// /// only zero is supported /// only SeekOrigin.Begin is supported ///zero public override long Seek(long offset, SeekOrigin origin) { lock (_syncRoot) { CheckDisposed(); return _baseStream.Seek(offset, origin); } } ////// SetLength /// ///not supported public override void SetLength(long newLength) { lock (_syncRoot) { CheckDisposed(); _baseStream.SetLength(newLength); } } ////// Write /// ///not supported public override void Write(byte[] buf, int offset, int count) { lock (_syncRoot) { CheckDisposed(); _baseStream.Write(buf, offset, count); } } ////// Flush /// ///not supported public override void Flush() { lock (_syncRoot) { CheckDisposed(); _baseStream.Flush(); } } //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ ////// Is stream readable? /// public override bool CanRead { get { lock (_syncRoot) { return ((_baseStream != null) && _baseStream.CanRead); } } } ////// Is stream seekable? /// ///We MUST support seek as this is used to implement ILockBytes.ReadAt() public override bool CanSeek { get { lock (_syncRoot) { return ((_baseStream != null) && _baseStream.CanSeek); } } } ////// Is stream writeable? /// public override bool CanWrite { get { lock (_syncRoot) { return ((_baseStream != null) && _baseStream.CanWrite); } } } ////// Logical byte position in this stream /// public override long Position { get { lock (_syncRoot) { CheckDisposed(); return _baseStream.Position; } } set { lock (_syncRoot) { CheckDisposed(); _baseStream.Position = value; } } } ////// Length /// public override long Length { get { lock (_syncRoot) { CheckDisposed(); return _baseStream.Length; } } } //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ protected override void Dispose(bool disposing) { lock (_syncRoot) { try { if (disposing && (_baseStream != null)) { // close the underlying Stream _baseStream.Close(); // NOTE: We cannot set _syncRoot to null because it is used // on entry to every public method and property. } } finally { base.Dispose(disposing); _baseStream = null; } } } //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- ////// CheckDisposed /// ///Pre-condition that lock has been acquired. private void CheckDisposed() { if (_baseStream == null) throw new ObjectDisposedException("Stream"); } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private Stream _baseStream; // stream we are wrapping private Object _syncRoot; // object to lock on } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: SynchronizingStream.cs // // Description: Stream that locks on given syncRoot before entering any public API's. // // History: 01/20/06 - brucemac - created //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.IO; using System.Windows; namespace MS.Internal.IO.Packaging { ////// Wrap returned stream to protect non-thread-safe API's from race conditions /// internal class SynchronizingStream : Stream { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Serializes access by locking on the given object /// /// stream to read from (baseStream) /// object to lock on internal SynchronizingStream(Stream stream, Object syncRoot) { if (stream == null) throw new ArgumentNullException("stream"); if (syncRoot == null) throw new ArgumentNullException("syncRoot"); _baseStream = stream; _syncRoot = syncRoot; } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// Return the bytes requested /// /// destination buffer /// offset to write into that buffer /// how many bytes requested ///how many bytes were written into buffer public override int Read(byte[] buffer, int offset, int count) { lock (_syncRoot) { CheckDisposed(); return (_baseStream.Read(buffer, offset, count)); } } ////// Read a single byte /// ///The unsigned byte cast to an Int32, or -1 if at the end of the stream. public override int ReadByte() { lock (_syncRoot) { CheckDisposed(); return (_baseStream.ReadByte()); } } ////// Write a single byte /// /// byte to write public override void WriteByte(byte b) { lock (_syncRoot) { CheckDisposed(); _baseStream.WriteByte(b); } } ////// Seek /// /// only zero is supported /// only SeekOrigin.Begin is supported ///zero public override long Seek(long offset, SeekOrigin origin) { lock (_syncRoot) { CheckDisposed(); return _baseStream.Seek(offset, origin); } } ////// SetLength /// ///not supported public override void SetLength(long newLength) { lock (_syncRoot) { CheckDisposed(); _baseStream.SetLength(newLength); } } ////// Write /// ///not supported public override void Write(byte[] buf, int offset, int count) { lock (_syncRoot) { CheckDisposed(); _baseStream.Write(buf, offset, count); } } ////// Flush /// ///not supported public override void Flush() { lock (_syncRoot) { CheckDisposed(); _baseStream.Flush(); } } //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ ////// Is stream readable? /// public override bool CanRead { get { lock (_syncRoot) { return ((_baseStream != null) && _baseStream.CanRead); } } } ////// Is stream seekable? /// ///We MUST support seek as this is used to implement ILockBytes.ReadAt() public override bool CanSeek { get { lock (_syncRoot) { return ((_baseStream != null) && _baseStream.CanSeek); } } } ////// Is stream writeable? /// public override bool CanWrite { get { lock (_syncRoot) { return ((_baseStream != null) && _baseStream.CanWrite); } } } ////// Logical byte position in this stream /// public override long Position { get { lock (_syncRoot) { CheckDisposed(); return _baseStream.Position; } } set { lock (_syncRoot) { CheckDisposed(); _baseStream.Position = value; } } } ////// Length /// public override long Length { get { lock (_syncRoot) { CheckDisposed(); return _baseStream.Length; } } } //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ protected override void Dispose(bool disposing) { lock (_syncRoot) { try { if (disposing && (_baseStream != null)) { // close the underlying Stream _baseStream.Close(); // NOTE: We cannot set _syncRoot to null because it is used // on entry to every public method and property. } } finally { base.Dispose(disposing); _baseStream = null; } } } //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- ////// CheckDisposed /// ///Pre-condition that lock has been acquired. private void CheckDisposed() { if (_baseStream == null) throw new ObjectDisposedException("Stream"); } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private Stream _baseStream; // stream we are wrapping private Object _syncRoot; // object to lock on } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
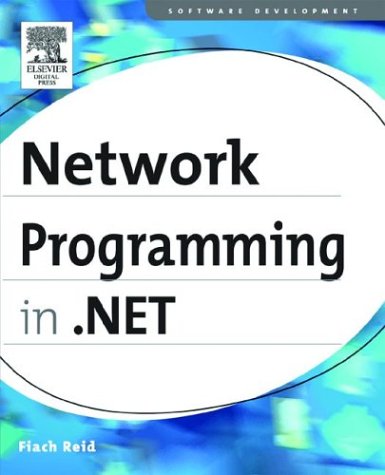
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QilSortKey.cs
- TraceUtility.cs
- Root.cs
- WebPermission.cs
- LastQueryOperator.cs
- Types.cs
- SchemaNotation.cs
- Run.cs
- CharacterHit.cs
- DeclaredTypeValidator.cs
- SqlCaseSimplifier.cs
- BooleanProjectedSlot.cs
- MexHttpBindingElement.cs
- ChannelBinding.cs
- HostSecurityManager.cs
- Hex.cs
- PermissionAttributes.cs
- UndirectedGraph.cs
- dbdatarecord.cs
- ItemType.cs
- IndentedWriter.cs
- JournalEntry.cs
- SchemaInfo.cs
- LineInfo.cs
- HttpApplicationStateWrapper.cs
- TransactionFormatter.cs
- Mappings.cs
- RootBuilder.cs
- SerializationException.cs
- DataGridRow.cs
- EntityDataSourceChangedEventArgs.cs
- LocalizationComments.cs
- SimpleWorkerRequest.cs
- QuarticEase.cs
- Dispatcher.cs
- ScriptResourceAttribute.cs
- XamlPoint3DCollectionSerializer.cs
- ProcessProtocolHandler.cs
- LeftCellWrapper.cs
- PeerCollaboration.cs
- ListItem.cs
- Dynamic.cs
- ImageFormat.cs
- SqlRemoveConstantOrderBy.cs
- MasterPageCodeDomTreeGenerator.cs
- unitconverter.cs
- BooleanSwitch.cs
- WebBrowserHelper.cs
- SimpleColumnProvider.cs
- FakeModelItemImpl.cs
- FormViewDeleteEventArgs.cs
- RegexStringValidator.cs
- InfoCardTraceRecord.cs
- DateTime.cs
- XmlBoundElement.cs
- TextParagraphProperties.cs
- EqualityComparer.cs
- SystemIPv4InterfaceProperties.cs
- PowerModeChangedEventArgs.cs
- BasePropertyDescriptor.cs
- ConnectionPoolManager.cs
- BreakSafeBase.cs
- ConfigurationStrings.cs
- FileIOPermission.cs
- BitmapEffect.cs
- ContentWrapperAttribute.cs
- XmlEntity.cs
- Model3DCollection.cs
- PlatformCulture.cs
- Hash.cs
- CompleteWizardStep.cs
- DictionarySurrogate.cs
- DiscoveryInnerClientManaged11.cs
- DragDrop.cs
- _NtlmClient.cs
- CounterNameConverter.cs
- FrugalMap.cs
- EventMappingSettings.cs
- NavigationWindowAutomationPeer.cs
- InternalBase.cs
- UInt16Storage.cs
- XmlnsDefinitionAttribute.cs
- PingOptions.cs
- Int16Animation.cs
- SqlUtil.cs
- ReceiveErrorHandling.cs
- ScriptReference.cs
- HandleCollector.cs
- SQLInt32Storage.cs
- SmtpFailedRecipientsException.cs
- XmlDocument.cs
- Animatable.cs
- ConfigurationSection.cs
- ResXBuildProvider.cs
- XmlSerializableServices.cs
- JavaScriptSerializer.cs
- GridViewEditEventArgs.cs
- EncodingTable.cs
- ResourceIDHelper.cs
- ControlPaint.cs