Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Discovery / System / ServiceModel / Channels / UdpRetransmissionSettings.cs / 1305376 / UdpRetransmissionSettings.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Channels { using System; using System.Runtime; using System.ServiceModel.Discovery; sealed class UdpRetransmissionSettings { int maxUnicastRetransmitCount; int maxMulticastRetransmitCount; TimeSpan delayLowerBound; TimeSpan delayUpperBound; TimeSpan maxDelayPerRetransmission; int delayLowerBoundMilliseconds; int delayUpperBoundMilliseconds; int maxDelayMilliseconds; public UdpRetransmissionSettings(int maxUnicastRetransmitCount, int maxMulticastRetransmitCount) : this(maxUnicastRetransmitCount, maxMulticastRetransmitCount, TimeSpan.FromMilliseconds(50), TimeSpan.FromMilliseconds(250), TimeSpan.FromMilliseconds(500)) { } public UdpRetransmissionSettings(int maxUnicastRetransmitCount, int maxMulticastRetransmitCount, TimeSpan delayLowerBound, TimeSpan delayUpperBound, TimeSpan maxDelayPerRetransmission) { if (maxUnicastRetransmitCount < 0) { throw FxTrace.Exception.ArgumentOutOfRange("maxUnicastRetransmitCount", maxUnicastRetransmitCount, SR.ArgumentOutOfMinRange(0)); } if (maxMulticastRetransmitCount < 0) { throw FxTrace.Exception.ArgumentOutOfRange("maxMulticastRetransmitCount", maxMulticastRetransmitCount, SR.ArgumentOutOfMinRange(0)); } if (delayLowerBound < TimeSpan.Zero) { throw FxTrace.Exception.ArgumentOutOfRange("delayLowerBound", delayLowerBound, SR.ArgumentOutOfMinRange(TimeSpan.Zero)); } if (delayUpperBound < TimeSpan.Zero) { throw FxTrace.Exception.ArgumentOutOfRange("delayUpperBound", delayUpperBound, SR.ArgumentOutOfMinRange(TimeSpan.Zero)); } if (maxDelayPerRetransmission < TimeSpan.Zero) { throw FxTrace.Exception.ArgumentOutOfRange("maxDelayPerRetransmission", maxDelayPerRetransmission, SR.ArgumentOutOfMinRange(TimeSpan.Zero)); } this.maxUnicastRetransmitCount = maxUnicastRetransmitCount; this.maxMulticastRetransmitCount = maxMulticastRetransmitCount; this.delayLowerBound = delayLowerBound; this.delayUpperBound = delayUpperBound; this.maxDelayPerRetransmission = maxDelayPerRetransmission; this.delayLowerBoundMilliseconds = -1; this.delayUpperBoundMilliseconds = -1; this.maxDelayMilliseconds = -1; ValidateSettings(); } //this constructor disables retransmission internal UdpRetransmissionSettings() : this(0, 0) { } UdpRetransmissionSettings(UdpRetransmissionSettings other) : this(other.maxUnicastRetransmitCount, other.maxMulticastRetransmitCount, other.delayLowerBound, other.delayUpperBound, other.maxDelayPerRetransmission) { } public int MaxUnicastRetransmitCount { get { return this.maxUnicastRetransmitCount; } set { const int min = 0; if (value < min) { throw FxTrace.Exception.ArgumentOutOfRange("value", value, SR.ArgumentOutOfMinRange(min)); } this.maxUnicastRetransmitCount = value; } } public int MaxMulticastRetransmitCount { get { return this.maxMulticastRetransmitCount; } set { const int min = 0; if (value < min) { throw FxTrace.Exception.ArgumentOutOfRange("value", value, SR.ArgumentOutOfMinRange(min)); } this.maxMulticastRetransmitCount = value; } } public TimeSpan DelayLowerBound { get { return this.delayLowerBound; } set { if (value < TimeSpan.Zero) { throw FxTrace.Exception.ArgumentOutOfRange("value", value, SR.ArgumentOutOfMinRange(TimeSpan.Zero)); } this.delayLowerBound = value; } } public TimeSpan DelayUpperBound { get { return this.delayUpperBound; } set { if (value < TimeSpan.Zero) { throw FxTrace.Exception.ArgumentOutOfRange("value", value, SR.ArgumentOutOfMinRange(TimeSpan.Zero)); } this.delayUpperBound = value; } } public TimeSpan MaxDelayPerRetransmission { get { return this.maxDelayPerRetransmission; } set { if (value < TimeSpan.Zero) { throw FxTrace.Exception.ArgumentOutOfRange("value", value, SR.ArgumentOutOfMinRange(TimeSpan.Zero)); } this.maxDelayPerRetransmission = value; } } //called at send time to avoid repeated rounding and casting internal int GetDelayLowerBound() { if (this.delayLowerBoundMilliseconds < 0) { this.delayLowerBoundMilliseconds = TimeoutHelper.ToMilliseconds(this.delayLowerBound); } return this.delayLowerBoundMilliseconds; } //called at send time to avoid repeated rounding and casting internal int GetDelayUpperBound() { if (this.delayUpperBoundMilliseconds < 0) { this.delayUpperBoundMilliseconds = TimeoutHelper.ToMilliseconds(this.delayUpperBound); } return this.delayUpperBoundMilliseconds; } //called at send time to avoid repeated rounding and casting internal int GetMaxDelayPerRetransmission() { if (this.maxDelayMilliseconds < 0) { this.maxDelayMilliseconds = TimeoutHelper.ToMilliseconds(this.maxDelayPerRetransmission); } return this.maxDelayMilliseconds; } internal bool Enabled { get { return this.maxUnicastRetransmitCount > 0 || this.maxMulticastRetransmitCount > 0; } } internal void ValidateSettings() { if (this.delayLowerBound > this.delayUpperBound) { throw FxTrace.Exception.ArgumentOutOfRange("DelayLowerBound", this.delayLowerBound, SR.Property1LessThanOrEqualToProperty2("DelayLowerBound", this.delayLowerBound, "DelayUpperBound", this.delayUpperBound)); } if (this.delayUpperBound > this.maxDelayPerRetransmission) { throw FxTrace.Exception.ArgumentOutOfRange("DelayUpperBound", this.delayUpperBound, SR.Property1LessThanOrEqualToProperty2("DelayUpperBound", this.delayUpperBound, "MaxDelayPerRetransmission", this.maxDelayPerRetransmission)); } } internal UdpRetransmissionSettings Clone() { return new UdpRetransmissionSettings(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
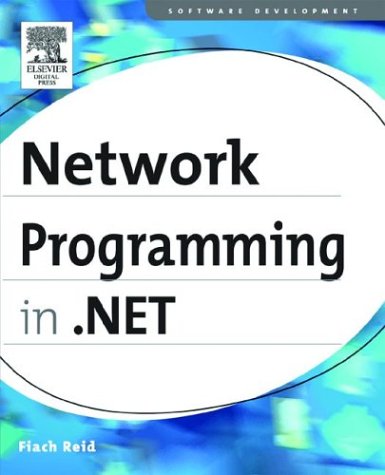
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UInt64Converter.cs
- ObjectItemCollection.cs
- PreservationFileReader.cs
- TextTreeRootNode.cs
- JournalEntryListConverter.cs
- DataControlFieldsEditor.cs
- EasingKeyFrames.cs
- followingquery.cs
- ToolStripItemDataObject.cs
- WebScriptClientGenerator.cs
- ZoneButton.cs
- ExpressionPrefixAttribute.cs
- StrokeNode.cs
- BufferedConnection.cs
- AnonymousIdentificationModule.cs
- ModelFunctionTypeElement.cs
- NonVisualControlAttribute.cs
- StateBag.cs
- PenThreadWorker.cs
- DataGridViewCellCancelEventArgs.cs
- ComponentManagerBroker.cs
- GetReadStreamResult.cs
- SymLanguageType.cs
- shaperfactoryquerycachekey.cs
- DesignOnlyAttribute.cs
- ObjectStateManager.cs
- smtpconnection.cs
- DesignerProperties.cs
- InvalidDataContractException.cs
- EnumType.cs
- OdbcEnvironmentHandle.cs
- ValueTypeFixupInfo.cs
- ScrollViewerAutomationPeer.cs
- HtmlUtf8RawTextWriter.cs
- ParallelDesigner.cs
- SimpleFileLog.cs
- ConnectionsZoneAutoFormat.cs
- BufferedReceiveManager.cs
- Switch.cs
- BackStopAuthenticationModule.cs
- SpecialFolderEnumConverter.cs
- COM2ExtendedTypeConverter.cs
- SafeHandle.cs
- WsatTransactionFormatter.cs
- GeometryModel3D.cs
- XamlContextStack.cs
- UriExt.cs
- MethodBody.cs
- InheritedPropertyChangedEventArgs.cs
- AssemblyAttributesGoHere.cs
- ResourceManager.cs
- DbConnectionClosed.cs
- ExpressionBuilderContext.cs
- SerializationSectionGroup.cs
- Rotation3DKeyFrameCollection.cs
- PathStreamGeometryContext.cs
- ExpandableObjectConverter.cs
- ThreadStaticAttribute.cs
- ProjectionPath.cs
- DataControlFieldCell.cs
- ContextMenuStrip.cs
- DPCustomTypeDescriptor.cs
- GeneralTransform3DGroup.cs
- DataGridViewColumnTypePicker.cs
- ConfigurationManagerInternalFactory.cs
- OptimalTextSource.cs
- RenderTargetBitmap.cs
- AnchoredBlock.cs
- DataGridComponentEditor.cs
- ToolStripCustomTypeDescriptor.cs
- DataObject.cs
- PublisherIdentityPermission.cs
- TcpProcessProtocolHandler.cs
- PointLight.cs
- TraceFilter.cs
- IdnElement.cs
- WebPartDeleteVerb.cs
- Int32KeyFrameCollection.cs
- IODescriptionAttribute.cs
- TemplateXamlParser.cs
- SQlBooleanStorage.cs
- ProxyWebPartManager.cs
- XPathSelectionIterator.cs
- CacheMemory.cs
- PrinterSettings.cs
- DataControlExtensions.cs
- DataGridViewDataErrorEventArgs.cs
- EntityClientCacheEntry.cs
- Substitution.cs
- TransformerTypeCollection.cs
- Control.cs
- BuiltInExpr.cs
- UriSection.cs
- RepeatBehaviorConverter.cs
- BCLDebug.cs
- SQLByteStorage.cs
- RemotingConfiguration.cs
- PreviewKeyDownEventArgs.cs
- XPathNodeHelper.cs
- MaterialGroup.cs