Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Imaging / RenderTargetBitmap.cs / 1 / RenderTargetBitmap.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation. All Rights Reserved. // // File: BitmapImage.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { #region RenderTargetBitmap ////// RenderTargetBitmap provides caching functionality for a BitmapSource. /// public sealed class RenderTargetBitmap : System.Windows.Media.Imaging.BitmapSource { ////// RenderTargetBitmap - used in conjuction with BitmapVisualManager /// /// The width of the Bitmap /// The height of the Bitmap /// Horizontal DPI of the Bitmap /// Vertical DPI of the Bitmap /// Format of the Bitmap. ////// Critical - access critical resources /// PublicOK - All inputs verified /// [SecurityCritical] public RenderTargetBitmap( int pixelWidth, int pixelHeight, double dpiX, double dpiY, PixelFormat pixelFormat ) : base(true) { if (pixelFormat.Format == PixelFormatEnum.Default) { pixelFormat = PixelFormats.Pbgra32; } else if (pixelFormat.Format != PixelFormatEnum.Pbgra32) { throw new System.ArgumentException( SR.Get(SRID.Effect_PixelFormat, pixelFormat), "pixelFormat" ); } if (pixelWidth <= 0) { throw new ArgumentOutOfRangeException("pixelWidth", SR.Get(SRID.ParameterMustBeGreaterThanZero)); } if (pixelHeight <= 0) { throw new ArgumentOutOfRangeException("pixelHeight", SR.Get(SRID.ParameterMustBeGreaterThanZero)); } if (dpiX < DoubleUtil.DBL_EPSILON) { dpiX = 96.0; } if (dpiY < DoubleUtil.DBL_EPSILON) { dpiY = 96.0; } _bitmapInit.BeginInit(); _pixelWidth = pixelWidth; _pixelHeight = pixelHeight; _dpiX = dpiX; _dpiY = dpiY; _format = pixelFormat; _bitmapInit.EndInit(); FinalizeCreation(); } ////// Internal ctor /// internal RenderTargetBitmap() : base(true) { } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new RenderTargetBitmap(); } ////// Common Copy method used to implement CloneCore(), CloneCurrentValueCore(), /// GetAsFrozenCore(), and GetCurrentValueAsFrozenCore() /// ////// Critical - access unmanaged resource /// TreatAsSafe - making a clone which is safe /// [SecurityCritical, SecurityTreatAsSafe] private void CopyCommon(RenderTargetBitmap sourceBitmap) { _bitmapInit.BeginInit(); _pixelWidth = sourceBitmap._pixelWidth; _pixelHeight = sourceBitmap._pixelHeight; _dpiX = sourceBitmap._dpiX; _dpiY = sourceBitmap._dpiY; _format = sourceBitmap._format; // // In order to make a deep clone we need to // create a new bitmap with the contents of the // existing bitmap and then create a render target // from the new bitmap. // using (FactoryMaker myFactory = new FactoryMaker()) { BitmapSourceSafeMILHandle newBitmapHandle = null; IntPtr pIMILBitmap = IntPtr.Zero; SafeMILHandle milBitmapHandle = null; // Create an IWICBitmap HRESULT.Check(UnsafeNativeMethods.WICImagingFactory.CreateBitmapFromSource( myFactory.ImagingFactoryPtr, WicSourceHandle, WICBitmapCreateCacheOptions.WICBitmapCacheOnLoad, out newBitmapHandle)); lock (_syncObject) { WicSourceHandle = newBitmapHandle; } Guid guidMILBs = MILGuidData.IID_IMILBitmapSource; // QI for IMILBitmapSource HRESULT.Check(UnsafeNativeMethods.MILUnknown.QueryInterface( newBitmapHandle, ref guidMILBs, out pIMILBitmap)); // Creating this will automatically do the garbage collection (and release) milBitmapHandle = new SafeMILHandle(pIMILBitmap, 0); // Now create a Render target from that Bitmap HRESULT.Check(UnsafeNativeMethods.MILFactory2.CreateBitmapRenderTargetForBitmap( myFactory.FactoryPtr, pIMILBitmap, out _renderTargetBitmap)); } _bitmapInit.EndInit(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { RenderTargetBitmap sourceBitmap = (RenderTargetBitmap)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { RenderTargetBitmap sourceBitmap = (RenderTargetBitmap)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { RenderTargetBitmap sourceBitmap = (RenderTargetBitmap)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { RenderTargetBitmap sourceBitmap = (RenderTargetBitmap)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.GetCurrentValueAsFrozenCore . ////// Renders the specified Visual tree to the BitmapRenderTarget. /// public void Render(Visual visual) { BitmapVisualManager bmv = new BitmapVisualManager(this); bmv.Render(visual); // Render indirectly calls RenderTargetContentsChanged(); } ////// Renders the specified Visual tree to the BitmapRenderTarget /// This renders the visual without transform, clip, offset and /// guidelines applied to it. /// internal void RenderForBitmapEffect(Visual visual, Matrix worldTransform, Rect windowClip) { // first clear the render target Clear(); BitmapVisualManager bmv = new BitmapVisualManager(this); bmv.Render(visual, worldTransform, windowClip, true); // Render indirectly calls RenderTargetContentsChanged(); } ////// Clears the render target and sets every pixel to black transparent /// ////// Critical - access unmanaged resource /// PublicOk - Clear is safe (no inputs) /// [SecurityCritical] public void Clear() { HRESULT.Check(MILRenderTargetBitmap.Clear(_renderTargetBitmap)); RenderTargetContentsChanged(); } /// /// Get the MIL RenderTarget /// ////// Critical - returns unmanaged resource /// internal SafeMILHandle MILRenderTarget { [SecurityCritical] get { return _renderTargetBitmap; } } /// /// Notify that the contents of the render target have changed /// ////// Critical - access critical resource _convertedDUCEMILPtr /// TreatAsSafe - Critical data is used internally and not exposed /// [SecurityCritical, SecurityTreatAsSafe] internal void RenderTargetContentsChanged() { // If the render target has changed, we need to update the UCE resource. We ensure // this happens by throwing away our reference to the previous DUCE bitmap source // and forcing the creation of a new one. _isSourceCached = false; if (_convertedDUCEMILPtr != null) { _convertedDUCEMILPtr.Close(); _convertedDUCEMILPtr = null; } // Register for update in the next render pass RegisterForAsyncUpdateResource(); FireChanged(); } /// /// Create the unmanaged resources /// ////// Critical - creates critical resource /// [SecurityCritical] internal override void FinalizeCreation() { try { using (FactoryMaker myFactory = new FactoryMaker()) { SafeMILHandle renderTargetBitmap = null; HRESULT.Check(UnsafeNativeMethods.MILFactory2.CreateBitmapRenderTarget( myFactory.FactoryPtr, (uint)_pixelWidth, (uint)_pixelHeight, _format.Format, (float)_dpiX, (float)_dpiY, MILRTInitializationFlags.MIL_RT_INITIALIZE_DEFAULT, out renderTargetBitmap)); Debug.Assert(renderTargetBitmap != null && !renderTargetBitmap.IsInvalid); BitmapSourceSafeMILHandle bitmapSource = null; HRESULT.Check(MILRenderTargetBitmap.GetBitmap( renderTargetBitmap, out bitmapSource)); Debug.Assert(bitmapSource != null && !bitmapSource.IsInvalid); lock (_syncObject) { _renderTargetBitmap = renderTargetBitmap; WicSourceHandle = bitmapSource; // For the purpose of rendering a RenderTargetBitmap, we always treat it as if it's // not cached. This is to ensure we never render and write to the same bitmap source // by the UCE thread and managed thread. _isSourceCached = false; } } CreationCompleted = true; UpdateCachedSettings(); } catch { _bitmapInit.Reset(); throw; } } ////// Critical - unmanaged resource - not safe for handing out /// [SecurityCritical] private SafeMILHandle /* IMILRenderTargetBitmap */ _renderTargetBitmap; } #endregion // RenderTargetBitmap } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation. All Rights Reserved. // // File: BitmapImage.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { #region RenderTargetBitmap ////// RenderTargetBitmap provides caching functionality for a BitmapSource. /// public sealed class RenderTargetBitmap : System.Windows.Media.Imaging.BitmapSource { ////// RenderTargetBitmap - used in conjuction with BitmapVisualManager /// /// The width of the Bitmap /// The height of the Bitmap /// Horizontal DPI of the Bitmap /// Vertical DPI of the Bitmap /// Format of the Bitmap. ////// Critical - access critical resources /// PublicOK - All inputs verified /// [SecurityCritical] public RenderTargetBitmap( int pixelWidth, int pixelHeight, double dpiX, double dpiY, PixelFormat pixelFormat ) : base(true) { if (pixelFormat.Format == PixelFormatEnum.Default) { pixelFormat = PixelFormats.Pbgra32; } else if (pixelFormat.Format != PixelFormatEnum.Pbgra32) { throw new System.ArgumentException( SR.Get(SRID.Effect_PixelFormat, pixelFormat), "pixelFormat" ); } if (pixelWidth <= 0) { throw new ArgumentOutOfRangeException("pixelWidth", SR.Get(SRID.ParameterMustBeGreaterThanZero)); } if (pixelHeight <= 0) { throw new ArgumentOutOfRangeException("pixelHeight", SR.Get(SRID.ParameterMustBeGreaterThanZero)); } if (dpiX < DoubleUtil.DBL_EPSILON) { dpiX = 96.0; } if (dpiY < DoubleUtil.DBL_EPSILON) { dpiY = 96.0; } _bitmapInit.BeginInit(); _pixelWidth = pixelWidth; _pixelHeight = pixelHeight; _dpiX = dpiX; _dpiY = dpiY; _format = pixelFormat; _bitmapInit.EndInit(); FinalizeCreation(); } ////// Internal ctor /// internal RenderTargetBitmap() : base(true) { } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new RenderTargetBitmap(); } ////// Common Copy method used to implement CloneCore(), CloneCurrentValueCore(), /// GetAsFrozenCore(), and GetCurrentValueAsFrozenCore() /// ////// Critical - access unmanaged resource /// TreatAsSafe - making a clone which is safe /// [SecurityCritical, SecurityTreatAsSafe] private void CopyCommon(RenderTargetBitmap sourceBitmap) { _bitmapInit.BeginInit(); _pixelWidth = sourceBitmap._pixelWidth; _pixelHeight = sourceBitmap._pixelHeight; _dpiX = sourceBitmap._dpiX; _dpiY = sourceBitmap._dpiY; _format = sourceBitmap._format; // // In order to make a deep clone we need to // create a new bitmap with the contents of the // existing bitmap and then create a render target // from the new bitmap. // using (FactoryMaker myFactory = new FactoryMaker()) { BitmapSourceSafeMILHandle newBitmapHandle = null; IntPtr pIMILBitmap = IntPtr.Zero; SafeMILHandle milBitmapHandle = null; // Create an IWICBitmap HRESULT.Check(UnsafeNativeMethods.WICImagingFactory.CreateBitmapFromSource( myFactory.ImagingFactoryPtr, WicSourceHandle, WICBitmapCreateCacheOptions.WICBitmapCacheOnLoad, out newBitmapHandle)); lock (_syncObject) { WicSourceHandle = newBitmapHandle; } Guid guidMILBs = MILGuidData.IID_IMILBitmapSource; // QI for IMILBitmapSource HRESULT.Check(UnsafeNativeMethods.MILUnknown.QueryInterface( newBitmapHandle, ref guidMILBs, out pIMILBitmap)); // Creating this will automatically do the garbage collection (and release) milBitmapHandle = new SafeMILHandle(pIMILBitmap, 0); // Now create a Render target from that Bitmap HRESULT.Check(UnsafeNativeMethods.MILFactory2.CreateBitmapRenderTargetForBitmap( myFactory.FactoryPtr, pIMILBitmap, out _renderTargetBitmap)); } _bitmapInit.EndInit(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { RenderTargetBitmap sourceBitmap = (RenderTargetBitmap)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { RenderTargetBitmap sourceBitmap = (RenderTargetBitmap)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { RenderTargetBitmap sourceBitmap = (RenderTargetBitmap)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { RenderTargetBitmap sourceBitmap = (RenderTargetBitmap)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.GetCurrentValueAsFrozenCore . ////// Renders the specified Visual tree to the BitmapRenderTarget. /// public void Render(Visual visual) { BitmapVisualManager bmv = new BitmapVisualManager(this); bmv.Render(visual); // Render indirectly calls RenderTargetContentsChanged(); } ////// Renders the specified Visual tree to the BitmapRenderTarget /// This renders the visual without transform, clip, offset and /// guidelines applied to it. /// internal void RenderForBitmapEffect(Visual visual, Matrix worldTransform, Rect windowClip) { // first clear the render target Clear(); BitmapVisualManager bmv = new BitmapVisualManager(this); bmv.Render(visual, worldTransform, windowClip, true); // Render indirectly calls RenderTargetContentsChanged(); } ////// Clears the render target and sets every pixel to black transparent /// ////// Critical - access unmanaged resource /// PublicOk - Clear is safe (no inputs) /// [SecurityCritical] public void Clear() { HRESULT.Check(MILRenderTargetBitmap.Clear(_renderTargetBitmap)); RenderTargetContentsChanged(); } /// /// Get the MIL RenderTarget /// ////// Critical - returns unmanaged resource /// internal SafeMILHandle MILRenderTarget { [SecurityCritical] get { return _renderTargetBitmap; } } /// /// Notify that the contents of the render target have changed /// ////// Critical - access critical resource _convertedDUCEMILPtr /// TreatAsSafe - Critical data is used internally and not exposed /// [SecurityCritical, SecurityTreatAsSafe] internal void RenderTargetContentsChanged() { // If the render target has changed, we need to update the UCE resource. We ensure // this happens by throwing away our reference to the previous DUCE bitmap source // and forcing the creation of a new one. _isSourceCached = false; if (_convertedDUCEMILPtr != null) { _convertedDUCEMILPtr.Close(); _convertedDUCEMILPtr = null; } // Register for update in the next render pass RegisterForAsyncUpdateResource(); FireChanged(); } /// /// Create the unmanaged resources /// ////// Critical - creates critical resource /// [SecurityCritical] internal override void FinalizeCreation() { try { using (FactoryMaker myFactory = new FactoryMaker()) { SafeMILHandle renderTargetBitmap = null; HRESULT.Check(UnsafeNativeMethods.MILFactory2.CreateBitmapRenderTarget( myFactory.FactoryPtr, (uint)_pixelWidth, (uint)_pixelHeight, _format.Format, (float)_dpiX, (float)_dpiY, MILRTInitializationFlags.MIL_RT_INITIALIZE_DEFAULT, out renderTargetBitmap)); Debug.Assert(renderTargetBitmap != null && !renderTargetBitmap.IsInvalid); BitmapSourceSafeMILHandle bitmapSource = null; HRESULT.Check(MILRenderTargetBitmap.GetBitmap( renderTargetBitmap, out bitmapSource)); Debug.Assert(bitmapSource != null && !bitmapSource.IsInvalid); lock (_syncObject) { _renderTargetBitmap = renderTargetBitmap; WicSourceHandle = bitmapSource; // For the purpose of rendering a RenderTargetBitmap, we always treat it as if it's // not cached. This is to ensure we never render and write to the same bitmap source // by the UCE thread and managed thread. _isSourceCached = false; } } CreationCompleted = true; UpdateCachedSettings(); } catch { _bitmapInit.Reset(); throw; } } ////// Critical - unmanaged resource - not safe for handing out /// [SecurityCritical] private SafeMILHandle /* IMILRenderTargetBitmap */ _renderTargetBitmap; } #endregion // RenderTargetBitmap } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
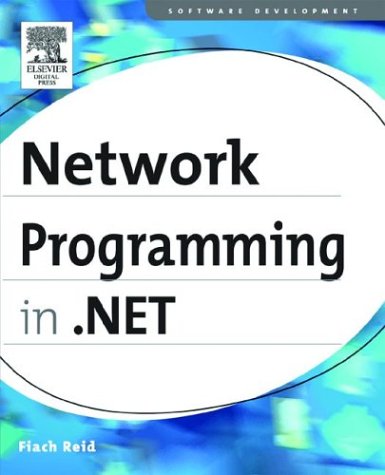
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReflectionServiceProvider.cs
- SessionStateSection.cs
- BinaryUtilClasses.cs
- ColorPalette.cs
- Buffer.cs
- SqlInternalConnection.cs
- ZoneMembershipCondition.cs
- MDIControlStrip.cs
- StructuralComparisons.cs
- SymbolMethod.cs
- SqlServer2KCompatibilityCheck.cs
- CopyCodeAction.cs
- BuildManagerHost.cs
- UnitySerializationHolder.cs
- EntityClientCacheKey.cs
- MediaEntryAttribute.cs
- TextClipboardData.cs
- ReadOnlyObservableCollection.cs
- VirtualDirectoryMappingCollection.cs
- RecordManager.cs
- StringResourceManager.cs
- Utils.cs
- InstanceKeyCompleteException.cs
- CoTaskMemUnicodeSafeHandle.cs
- EmptyElement.cs
- SmtpReplyReader.cs
- SoapReflectionImporter.cs
- UIElementPropertyUndoUnit.cs
- IsolatedStorageFilePermission.cs
- ContentPlaceHolder.cs
- SqlServices.cs
- CornerRadiusConverter.cs
- NotifyParentPropertyAttribute.cs
- ITextView.cs
- CodeDefaultValueExpression.cs
- DataGrid.cs
- UnsafeNativeMethods.cs
- CqlWriter.cs
- StorageModelBuildProvider.cs
- DataServiceProcessingPipelineEventArgs.cs
- DataBindingCollectionConverter.cs
- StrongTypingException.cs
- WebPartCatalogAddVerb.cs
- AvTraceFormat.cs
- WorkflowServiceInstance.cs
- ContextMenu.cs
- HMAC.cs
- TreeNode.cs
- ScopeCompiler.cs
- HttpModuleActionCollection.cs
- TextDecoration.cs
- RuntimeIdentifierPropertyAttribute.cs
- RuleElement.cs
- httpapplicationstate.cs
- RectKeyFrameCollection.cs
- EntityParameterCollection.cs
- SecurityDescriptor.cs
- DataGridViewSortCompareEventArgs.cs
- ObjectQuery_EntitySqlExtensions.cs
- SafeHandle.cs
- DataGridViewCheckBoxColumn.cs
- InternalCache.cs
- XmlElementCollection.cs
- CommandBindingCollection.cs
- SizeF.cs
- SettingsAttributeDictionary.cs
- DeferredSelectedIndexReference.cs
- ScrollViewerAutomationPeer.cs
- OneOfElement.cs
- HttpRawResponse.cs
- UrlMapping.cs
- SqlLiftWhereClauses.cs
- ConsoleTraceListener.cs
- RegionIterator.cs
- Pair.cs
- FeatureManager.cs
- BaseCodePageEncoding.cs
- CodeAttributeArgumentCollection.cs
- DependencyProperty.cs
- XmlDocumentSerializer.cs
- Int32AnimationUsingKeyFrames.cs
- BindingExpressionBase.cs
- SiteMapDataSourceView.cs
- CursorInteropHelper.cs
- DefaultHttpHandler.cs
- GuidTagList.cs
- PixelShader.cs
- CalendarDay.cs
- XamlStyleSerializer.cs
- TextTreeInsertElementUndoUnit.cs
- XmlUtf8RawTextWriter.cs
- XPathParser.cs
- URLMembershipCondition.cs
- WebPartDescription.cs
- NameTable.cs
- CriticalHandle.cs
- PrimarySelectionAdorner.cs
- ListItemConverter.cs
- UniqueConstraint.cs
- IApplicationTrustManager.cs