Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Stylus / RawStylusInputReport.cs / 1305600 / RawStylusInputReport.cs
using System; using System.ComponentModel; using System.Windows; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Input.StylusPlugIns; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationCore; // SecurityHelper using MS.Internal; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// The RawStylusInputReport class encapsulates the raw input provided /// from a stylus. /// ////// It is important to note that the InputReport class only contains /// blittable types. This is required so that the report can be /// marshalled across application domains. /// internal class RawStylusInputReport : InputReport { ///////////////////////////////////////////////////////////////////// ////// Constructs an instance of the RawStylusInputReport class. /// /// /// The mode in which the input is being provided. /// /// /// The time when the input occured. /// /// /// The PresentationSource over which the stylus moved. /// /// /// The PenContext. /// /// /// The set of actions being reported. /// /// /// Tablet device id. /// /// /// Stylus device id. /// /// /// Raw stylus data. /// ////// Critical: This handles critical data in the form of PresentationSource. /// TreatAsSafe:There are demands on the critical data(PresentationSource) /// [SecurityCritical,SecurityTreatAsSafe] internal RawStylusInputReport( InputMode mode, int timestamp, PresentationSource inputSource, PenContext penContext, RawStylusActions actions, int tabletDeviceId, int stylusDeviceId, int[] data) : base(inputSource, InputType.Stylus, mode, timestamp) { // Validate parameters if (!RawStylusActionsHelper.IsValid(actions)) { throw new InvalidEnumArgumentException(SR.Get(SRID.Enum_Invalid, "actions")); } if (data == null && actions != RawStylusActions.InRange) { throw new ArgumentNullException("data"); } _penContext = new SecurityCriticalDataClass(penContext); _actions = actions; _tabletDeviceId = tabletDeviceId; _stylusDeviceId = stylusDeviceId; _data = data; _isSynchronize = false; } ///////////////////////////////////////////////////////////////////// /// /// Constructs an instance of the RawStylusInputReport class. /// /// /// The mode in which the input is being provided. /// /// /// The time when the input occured. /// /// /// The PresentationSource over which the stylus moved. /// /// /// The set of actions being reported. /// /// /// Raw stylus data StylusPointDescription. /// /// /// Raw stylus data. /// ////// Critical: This handles critical data in the form of PresentationSource. /// TreatAsSafe:There are demands on the critical data(PresentationSource) /// [SecurityCritical,SecurityTreatAsSafe] internal RawStylusInputReport( InputMode mode, int timestamp, PresentationSource inputSource, RawStylusActions actions, StylusPointDescription mousePointDescription, int[] mouseData) : base(inputSource, InputType.Stylus, mode, timestamp) { // Validate parameters if (!RawStylusActionsHelper.IsValid(actions)) { throw new InvalidEnumArgumentException(SR.Get(SRID.Enum_Invalid, "actions")); } if (mouseData == null) { throw new ArgumentNullException("mouseData"); } _penContext = new SecurityCriticalDataClass(null); _actions = actions; _tabletDeviceId = 0; _stylusDeviceId = 0; _data = mouseData; _isSynchronize = false; _mousePointDescription = mousePointDescription; _isMouseInput = true; } ///////////////////////////////////////////////////////////////////// /// /// Read-only access to the set of actions that were reported. /// internal RawStylusActions Actions { get { return _actions; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to stylus context id that reported the data. /// internal int TabletDeviceId { get { return _tabletDeviceId; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to stylus context id that reported the data. /// ////// Critical: provides access to critical member _penContext /// internal PenContext PenContext { [SecurityCritical] get { return _penContext.Value; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to stylus context id that reported the data. /// ////// Critical: This handles critical data _penContext /// TreatAsSafe: We're returning safe data /// internal StylusPointDescription StylusPointDescription { [SecurityCritical, SecurityTreatAsSafe] get { return _isMouseInput ? _mousePointDescription : _penContext.Value.StylusPointDescription; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to stylus device id that reported the data. /// internal int StylusDeviceId { get { return _stylusDeviceId; } } ///////////////////////////////////////////////////////////////////// internal StylusDevice StylusDevice { get { return _stylusDevice; } set { _stylusDevice = value; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to the raw data that was reported. /// ////// Critical: Access the critical field - _data /// TreatAsSafe: No input is taken. It's safe to a clone. /// [SecurityCritical, SecurityTreatAsSafe] internal int[] GetRawPacketData() { if (_data == null) return null; return (int[])_data.Clone(); } ///////////////////////////////////////////////////////////////////// ////// Critical: Access the critical field - _data /// TreatAsSafe: No input is taken. It's safe to return the last tablet point. /// [SecurityCritical, SecurityTreatAsSafe] internal Point GetLastTabletPoint() { int packetLength = StylusPointDescription.GetInputArrayLengthPerPoint(); int lastXIndex = _data.Length - packetLength; return new Point(_data[lastXIndex], _data[lastXIndex + 1]); } ///////////////////////////////////////////////////////////////////// ////// Critical - Hands out ref to internal data that can be used to spoof input. /// internal int[] Data { [SecurityCritical] get { return _data; } } ///////////////////////////////////////////////////////////////////// ////// Critical - Setting property can be used to spoof input. /// internal RawStylusInput RawStylusInput { get { return _rawStylusInput.Value; } [SecurityCritical] set { _rawStylusInput.Value = value; } } ///////////////////////////////////////////////////////////////////// internal bool Synchronized { get { return _isSynchronize; } set { _isSynchronize = value; } } ///////////////////////////////////////////////////////////////////// RawStylusActions _actions; int _tabletDeviceId; ////// Critical: Marked critical to prevent inadvertant spread to transparent code /// private SecurityCriticalDataClass_penContext; int _stylusDeviceId; /// /// Critical to prevent accidental spread to transparent code /// [SecurityCritical] int[] _data; StylusDevice _stylusDevice; // cached value looked up from _stylusDeviceId SecurityCriticalDataForSet_rawStylusInput; bool _isSynchronize; // Set from StylusDevice.Synchronize. bool _isMouseInput; // Special case of mouse input being sent to plugins StylusPointDescription _mousePointDescription; // layout for mouse points } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
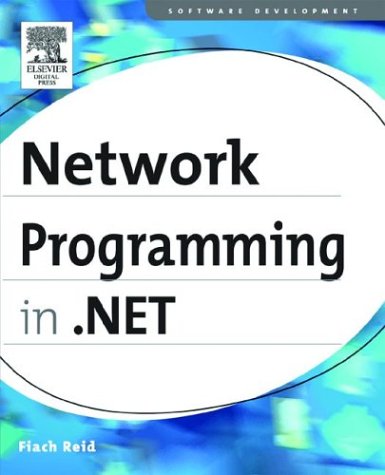
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BitmapSizeOptions.cs
- VectorConverter.cs
- EventLogEntryCollection.cs
- WebConfigurationHost.cs
- DataGridViewTopLeftHeaderCell.cs
- DataGridViewDataConnection.cs
- DataContext.cs
- VBCodeProvider.cs
- SchemaNames.cs
- ListControlDataBindingHandler.cs
- SiteMapNodeItemEventArgs.cs
- Ref.cs
- QueryServiceConfigHandle.cs
- PointAnimationBase.cs
- Substitution.cs
- UTF8Encoding.cs
- AnnotationResourceCollection.cs
- DES.cs
- XmlEntityReference.cs
- HtmlFormAdapter.cs
- XmlChoiceIdentifierAttribute.cs
- DataColumnCollection.cs
- ReadOnlyDictionary.cs
- WorkflowClientDeliverMessageWrapper.cs
- ReliableChannelBinder.cs
- DataTableMapping.cs
- GacUtil.cs
- StringBuilder.cs
- XmlMapping.cs
- RowTypeElement.cs
- SqlMethodAttribute.cs
- ObjectManager.cs
- UpdatePanelControlTrigger.cs
- XmlSiteMapProvider.cs
- ObjectMemberMapping.cs
- HostedHttpRequestAsyncResult.cs
- DiagnosticTrace.cs
- DataGridViewButtonColumn.cs
- PixelFormat.cs
- BooleanConverter.cs
- InheritedPropertyChangedEventArgs.cs
- PropertyDescriptor.cs
- ValidationEventArgs.cs
- KnowledgeBase.cs
- UrlParameterReader.cs
- ToolbarAUtomationPeer.cs
- WebFaultClientMessageInspector.cs
- DocumentCollection.cs
- BulletedListEventArgs.cs
- CommandEventArgs.cs
- StrokeNode.cs
- MessageDecoder.cs
- SourceExpressionException.cs
- DataControlPagerLinkButton.cs
- EntityDataSourceValidationException.cs
- DataExpression.cs
- SystemColors.cs
- SqlNodeTypeOperators.cs
- AsyncResult.cs
- TemplateColumn.cs
- ClientSettingsProvider.cs
- BaseCollection.cs
- MissingMethodException.cs
- ConnectionStringsExpressionBuilder.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- RetriableClipboard.cs
- GroupBox.cs
- PersonalizationState.cs
- QuotaThrottle.cs
- DirectoryGroupQuery.cs
- View.cs
- ServiceXNameTypeConverter.cs
- StringTraceRecord.cs
- DataGridParentRows.cs
- HttpHeaderCollection.cs
- HyperLinkField.cs
- Clock.cs
- Bold.cs
- DataTable.cs
- CompressEmulationStream.cs
- Debug.cs
- ThicknessKeyFrameCollection.cs
- SqlConnection.cs
- EventListenerClientSide.cs
- HyperLinkColumn.cs
- CodeSubDirectoriesCollection.cs
- IteratorFilter.cs
- UIElementIsland.cs
- NetworkInformationPermission.cs
- DragDropManager.cs
- BaseCodeDomTreeGenerator.cs
- DbConnectionPool.cs
- BindToObject.cs
- OleDbPermission.cs
- SuppressMergeCheckAttribute.cs
- BezierSegment.cs
- DataObjectCopyingEventArgs.cs
- Pair.cs
- HwndSource.cs
- MouseDevice.cs