Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / System / Windows / Generated / VectorConverter.cs / 1 / VectorConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Converters; using System.Windows; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows { ////// VectorConverter - Converter class for converting instances of other types to and from Vector instances /// public sealed class VectorConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a Vector from the given object. /// ////// The Vector which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Vector. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of Vector. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return Vector.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of Vector to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of Vector, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the Vector instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Vector) { Vector instance = (Vector)value; if (destinationType == typeof(string)) { // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Converters; using System.Windows; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows { ////// VectorConverter - Converter class for converting instances of other types to and from Vector instances /// public sealed class VectorConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a Vector from the given object. /// ////// The Vector which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Vector. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of Vector. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return Vector.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of Vector to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of Vector, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the Vector instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Vector) { Vector instance = (Vector)value; if (destinationType == typeof(string)) { // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
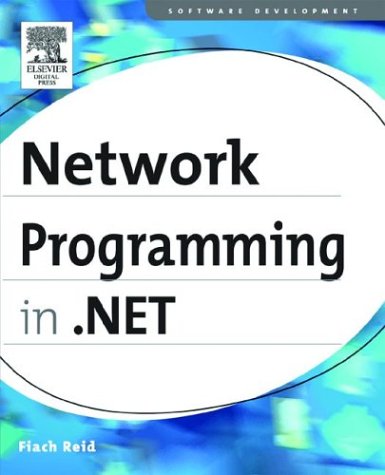
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- shaperfactoryquerycacheentry.cs
- SymLanguageVendor.cs
- AnnotationComponentManager.cs
- VirtualDirectoryMapping.cs
- codemethodreferenceexpression.cs
- ClrProviderManifest.cs
- GcHandle.cs
- WebPartAuthorizationEventArgs.cs
- InfoCardBaseException.cs
- ResourceSet.cs
- TypeValidationEventArgs.cs
- OverflowException.cs
- GrammarBuilder.cs
- Msmq.cs
- ErrorStyle.cs
- RootBrowserWindowAutomationPeer.cs
- BrushMappingModeValidation.cs
- MdImport.cs
- LocalServiceSecuritySettingsElement.cs
- ShaperBuffers.cs
- DockProviderWrapper.cs
- SoapIgnoreAttribute.cs
- FloatUtil.cs
- ColumnHeaderConverter.cs
- NavigationService.cs
- PermissionSet.cs
- UriScheme.cs
- PersonalizationProvider.cs
- FormattedText.cs
- MediaPlayer.cs
- ShaderRenderModeValidation.cs
- ObjectCache.cs
- HTTPNotFoundHandler.cs
- ADRole.cs
- AutomationIdentifierGuids.cs
- TableRow.cs
- TextParagraph.cs
- InstanceLockedException.cs
- XamlFigureLengthSerializer.cs
- ChtmlPhoneCallAdapter.cs
- TemplateBaseAction.cs
- EntityDataSourceSelectingEventArgs.cs
- StringExpressionSet.cs
- SQLBytes.cs
- OperatingSystem.cs
- Mappings.cs
- DbBuffer.cs
- MiniAssembly.cs
- ProfessionalColors.cs
- SR.cs
- PrincipalPermissionMode.cs
- entitydatasourceentitysetnameconverter.cs
- AttributeParameterInfo.cs
- SourceElementsCollection.cs
- sqlstateclientmanager.cs
- LineServices.cs
- TextSelectionProcessor.cs
- ConsoleCancelEventArgs.cs
- webbrowsersite.cs
- RSAProtectedConfigurationProvider.cs
- _LocalDataStore.cs
- DeriveBytes.cs
- RSAOAEPKeyExchangeFormatter.cs
- EdmSchemaError.cs
- AttachedPropertyBrowsableAttribute.cs
- TextSpan.cs
- CroppedBitmap.cs
- MultiByteCodec.cs
- PersonalizationProvider.cs
- PersonalizableTypeEntry.cs
- StateWorkerRequest.cs
- SystemWebExtensionsSectionGroup.cs
- ViewStateModeByIdAttribute.cs
- XsdDateTime.cs
- DefaultValueConverter.cs
- PropertyChangingEventArgs.cs
- WeakKeyDictionary.cs
- StatusBarAutomationPeer.cs
- ViewBase.cs
- Frame.cs
- ElasticEase.cs
- StateDesigner.TransitionInfo.cs
- HttpTransportSecurity.cs
- CommandEventArgs.cs
- SqlFactory.cs
- PageClientProxyGenerator.cs
- WebServiceHostFactory.cs
- StaticResourceExtension.cs
- AsyncDataRequest.cs
- BitmapEffectDrawingContent.cs
- ClrProviderManifest.cs
- NavigatorOutput.cs
- XmlSchemaSimpleType.cs
- FlowPanelDesigner.cs
- PriorityChain.cs
- HttpResponse.cs
- SerialErrors.cs
- BitmapEffectRenderDataResource.cs
- GenericsNotImplementedException.cs
- RegexWorker.cs