Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Ast / TryExpression.cs / 1305376 / TryExpression.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Dynamic.Utils; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { ////// Represents a try/catch/finally/fault block. /// /// The body is protected by the try block. /// The handlers consist of a set of #if !SILVERLIGHT [DebuggerTypeProxy(typeof(Expression.TryExpressionProxy))] #endif public sealed class TryExpression : Expression { private readonly Type _type; private readonly Expression _body; private readonly ReadOnlyCollections that can either be catch or filters. /// The fault runs if an exception is thrown. /// The finally runs regardless of how control exits the body. /// Only one of fault or finally can be supplied. /// The return type of the try block must match the return type of any associated catch statements. /// _handlers; private readonly Expression _finally; private readonly Expression _fault; internal TryExpression(Type type, Expression body, Expression @finally, Expression fault, ReadOnlyCollection handlers) { _type = type; _body = body; _handlers = handlers; _finally = @finally; _fault = fault; } /// /// Gets the static type of the expression that this ///represents. (Inherited from .) /// The public sealed override Type Type { get { return _type; } } ///that represents the static type of the expression. /// Returns the node type of this ///. (Inherited from .) /// The public sealed override ExpressionType NodeType { get { return ExpressionType.Try; } } ///that represents this expression. /// Gets the public Expression Body { get { return _body; } } ///representing the body of the try block. /// /// Gets the collection of public ReadOnlyCollections associated with the try block. /// Handlers { get { return _handlers; } } /// /// Gets the public Expression Finally { get { return _finally; } } ///representing the finally block. /// /// Gets the public Expression Fault { get { return _fault; } } ///representing the fault block. /// /// Dispatches to the specific visit method for this node type. /// protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitTry(this); } ////// Creates a new expression that is like this one, but using the /// supplied children. If all of the children are the same, it will /// return this expression. /// /// Theproperty of the result. /// The property of the result. /// The property of the result. /// The property of the result. /// This expression if no children changed, or an expression with the updated children. public TryExpression Update(Expression body, IEnumerablehandlers, Expression @finally, Expression fault) { if (body == Body && handlers == Handlers && @finally == Finally && fault == Fault) { return this; } return Expression.MakeTry(Type, body, @finally, fault, handlers); } } public partial class Expression { /// /// Creates a /// The body of the try block. /// The body of the fault block. ///representing a try block with a fault block and no catch statements. /// The created public static TryExpression TryFault(Expression body, Expression fault) { return MakeTry(null, body, null, fault, null); } ///. /// Creates a /// The body of the try block. /// The body of the finally block. ///representing a try block with a finally block and no catch statements. /// The created public static TryExpression TryFinally(Expression body, Expression @finally) { return MakeTry(null, body, @finally, null, null); } ///. /// Creates a /// The body of the try block. /// The array of zero or morerepresenting a try block with any number of catch statements and neither a fault nor finally block. /// s representing the catch statements to be associated with the try block. /// The created public static TryExpression TryCatch(Expression body, params CatchBlock[] handlers) { return MakeTry(null, body, null, null, handlers); } ///. /// Creates a /// The body of the try block. /// The body of the finally block. /// The array of zero or morerepresenting a try block with any number of catch statements and a finally block. /// s representing the catch statements to be associated with the try block. /// The created public static TryExpression TryCatchFinally(Expression body, Expression @finally, params CatchBlock[] handlers) { return MakeTry(null, body, @finally, null, handlers); } ///. /// Creates a /// The result type of the try expression. If null, bodh and all handlers must have identical type. /// The body of the try block. /// The body of the finally block. Pass null if the try block has no finally block associated with it. /// The body of the t block. Pass null if the try block has no fault block associated with it. /// A collection ofrepresenting a try block with the specified elements. /// s representing the catch statements to be associated with the try block. /// The created public static TryExpression MakeTry(Type type, Expression body, Expression @finally, Expression fault, IEnumerable. handlers) { RequiresCanRead(body, "body"); var @catch = handlers.ToReadOnly(); ContractUtils.RequiresNotNullItems(@catch, "handlers"); ValidateTryAndCatchHaveSameType(type, body, @catch); if (fault != null) { if (@finally != null || @catch.Count > 0) { throw Error.FaultCannotHaveCatchOrFinally(); } RequiresCanRead(fault, "fault"); } else if (@finally != null) { RequiresCanRead(@finally, "finally"); } else if (@catch.Count == 0) { throw Error.TryMustHaveCatchFinallyOrFault(); } return new TryExpression(type ?? body.Type, body, @finally, fault, @catch); } //Validate that the body of the try expression must have the same type as the body of every try block. private static void ValidateTryAndCatchHaveSameType(Type type, Expression tryBody, ReadOnlyCollection handlers) { // Type unification ... all parts must be reference assignable to "type" if (type != null) { if (type != typeof(void)) { if (!TypeUtils.AreReferenceAssignable(type, tryBody.Type)) { throw Error.ArgumentTypesMustMatch(); } foreach (var cb in handlers) { if (!TypeUtils.AreReferenceAssignable(type, cb.Body.Type)) { throw Error.ArgumentTypesMustMatch(); } } } } else if (tryBody == null || tryBody.Type == typeof(void)) { //The body of every try block must be null or have void type. foreach (CatchBlock cb in handlers) { if (cb.Body != null && cb.Body.Type != typeof(void)) { throw Error.BodyOfCatchMustHaveSameTypeAsBodyOfTry(); } } } else { //Body of every catch must have the same type of body of try. type = tryBody.Type; foreach (CatchBlock cb in handlers) { if (cb.Body == null || !TypeUtils.AreEquivalent(cb.Body.Type, type)) { throw Error.BodyOfCatchMustHaveSameTypeAsBodyOfTry(); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
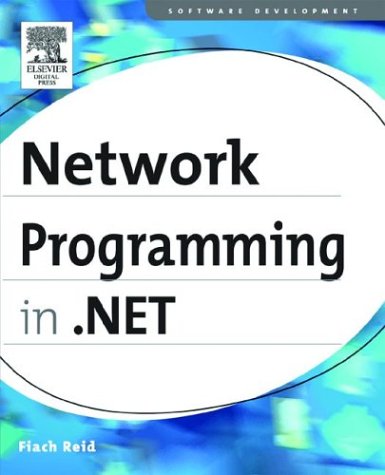
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EnumerableRowCollectionExtensions.cs
- LifetimeServices.cs
- AbstractDataSvcMapFileLoader.cs
- StorageComplexTypeMapping.cs
- SHA384.cs
- HotSpotCollection.cs
- XmlObjectSerializerWriteContext.cs
- SafeFileMappingHandle.cs
- XmlSchemaSimpleContentRestriction.cs
- XmlSchemaGroup.cs
- DesignerUtils.cs
- DependencyPropertyConverter.cs
- ClientUrlResolverWrapper.cs
- PeerServiceMessageContracts.cs
- JpegBitmapDecoder.cs
- dataprotectionpermission.cs
- NativeCppClassAttribute.cs
- httpapplicationstate.cs
- PageCanvasSize.cs
- DbConnectionFactory.cs
- Style.cs
- InputScopeAttribute.cs
- XamlRtfConverter.cs
- DecoderNLS.cs
- LayoutEngine.cs
- BindingWorker.cs
- NamespaceDisplayAutomationPeer.cs
- ModuleBuilderData.cs
- SapiRecognizer.cs
- UnsafeNativeMethods.cs
- TerminateSequenceResponse.cs
- TypeExtensionSerializer.cs
- WebPartPersonalization.cs
- SqlFlattener.cs
- BindingOperations.cs
- CommandID.cs
- ExtensionQuery.cs
- DocumentCollection.cs
- SchemaElementDecl.cs
- HotSpot.cs
- SocketConnection.cs
- ErrorRuntimeConfig.cs
- TypeDescriptionProvider.cs
- ClientEventManager.cs
- SqlInternalConnection.cs
- Mapping.cs
- PolicyManager.cs
- TimeEnumHelper.cs
- ViewPort3D.cs
- ProcessModelSection.cs
- PersistenceTypeAttribute.cs
- GroupQuery.cs
- DivideByZeroException.cs
- Visitor.cs
- WebBrowser.cs
- GradientStopCollection.cs
- ListSourceHelper.cs
- DefaultSection.cs
- SmtpAuthenticationManager.cs
- BinaryFormatter.cs
- LoginNameDesigner.cs
- ErrorFormatter.cs
- ItemCheckedEvent.cs
- Activator.cs
- cookieexception.cs
- EdgeModeValidation.cs
- SetterBase.cs
- CfgRule.cs
- PeerInvitationResponse.cs
- PropertySourceInfo.cs
- ListSortDescriptionCollection.cs
- PriorityItem.cs
- DocumentAutomationPeer.cs
- FixedSOMTextRun.cs
- SerializationEventsCache.cs
- DuplicateContext.cs
- DataGridViewComboBoxColumn.cs
- Utils.cs
- GeometryConverter.cs
- SqlRewriteScalarSubqueries.cs
- RegexCode.cs
- IntSecurity.cs
- UserValidatedEventArgs.cs
- DivideByZeroException.cs
- TabControlDesigner.cs
- DefaultCommandExtensionCallback.cs
- RepeaterCommandEventArgs.cs
- TimeoutException.cs
- ConstantProjectedSlot.cs
- FontSource.cs
- DataProtectionSecurityStateEncoder.cs
- RuntimeWrappedException.cs
- UniqueSet.cs
- BCLDebug.cs
- SmiEventSink.cs
- sitestring.cs
- Addressing.cs
- Int64.cs
- StorageAssociationTypeMapping.cs
- Parameter.cs