Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Data / BindingOperations.cs / 1305600 / BindingOperations.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Helper operations for data bindings. // // See spec at http://avalon/connecteddata/Specs/Data%20Binding.mht // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.ComponentModel; using System.Globalization; using System.Threading; using System.Windows; using System.Windows.Controls; using System.Windows.Markup; using System.Windows.Threading; using MS.Internal.Data; namespace System.Windows.Data { ////// Operations to manipulate data bindings. /// public static class BindingOperations { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- ////// Attach a BindingExpression to a property. /// ////// A new BindingExpression is created from the given description, and attached to /// the given property of the given object. This method is the way to /// attach a Binding to an arbitrary DependencyObject that may not expose /// its own SetBinding method. /// /// object on which to attach the Binding /// property to which to attach the Binding /// description of the Binding ///target and dp and binding cannot be null public static BindingExpressionBase SetBinding(DependencyObject target, DependencyProperty dp, BindingBase binding) { if (target == null) throw new ArgumentNullException("target"); if (dp == null) throw new ArgumentNullException("dp"); if (binding == null) throw new ArgumentNullException("binding"); // target.VerifyAccess(); BindingExpressionBase bindExpr = binding.CreateBindingExpression(target, dp); // target.SetValue(dp, bindExpr); return bindExpr; } ////// Retrieve a BindingBase. /// ////// This method returns null if no Binding has been set on the given /// property. /// /// object from which to retrieve the binding /// property from which to retrieve the binding ///target and dp cannot be null public static BindingBase GetBindingBase(DependencyObject target, DependencyProperty dp) { BindingExpressionBase b = GetBindingExpressionBase(target, dp); return (b != null) ? b.ParentBindingBase : null; } ////// Retrieve a Binding. /// ////// This method returns null if no Binding has been set on the given /// property. /// /// object from which to retrieve the binding /// property from which to retrieve the binding ///target and dp cannot be null public static Binding GetBinding(DependencyObject target, DependencyProperty dp) { return GetBindingBase(target, dp) as Binding; } ////// Retrieve a PriorityBinding. /// ////// This method returns null if no Binding has been set on the given /// property. /// /// object from which to retrieve the binding /// property from which to retrieve the binding ///target and dp cannot be null public static PriorityBinding GetPriorityBinding(DependencyObject target, DependencyProperty dp) { return GetBindingBase(target, dp) as PriorityBinding; } ////// Retrieve a MultiBinding. /// ////// This method returns null if no Binding has been set on the given /// property. /// /// object from which to retrieve the binding /// property from which to retrieve the binding ///target and dp cannot be null public static MultiBinding GetMultiBinding(DependencyObject target, DependencyProperty dp) { return GetBindingBase(target, dp) as MultiBinding; } ////// Retrieve a BindingExpressionBase. /// ////// This method returns null if no Binding has been set on the given /// property. /// /// object from which to retrieve the BindingExpression /// property from which to retrieve the BindingExpression ///target and dp cannot be null public static BindingExpressionBase GetBindingExpressionBase(DependencyObject target, DependencyProperty dp) { if (target == null) throw new ArgumentNullException("target"); if (dp == null) throw new ArgumentNullException("dp"); // target.VerifyAccess(); Expression expr = StyleHelper.GetExpression(target, dp); return expr as BindingExpressionBase; } ////// Retrieve a BindingExpression. /// ////// This method returns null if no Binding has been set on the given /// property. /// /// object from which to retrieve the BindingExpression /// property from which to retrieve the BindingExpression ///target and dp cannot be null public static BindingExpression GetBindingExpression(DependencyObject target, DependencyProperty dp) { BindingExpressionBase expr = GetBindingExpressionBase(target, dp); PriorityBindingExpression pb = expr as PriorityBindingExpression; if (pb != null) expr = pb.ActiveBindingExpression; return expr as BindingExpression; } ////// Retrieve a MultiBindingExpression. /// ////// This method returns null if no MultiBinding has been set on the given /// property. /// /// object from which to retrieve the MultiBindingExpression /// property from which to retrieve the MultiBindingExpression ///target and dp cannot be null public static MultiBindingExpression GetMultiBindingExpression(DependencyObject target, DependencyProperty dp) { return GetBindingExpressionBase(target, dp) as MultiBindingExpression; } ////// Retrieve a PriorityBindingExpression. /// ////// This method returns null if no PriorityBinding has been set on the given /// property. /// /// object from which to retrieve the PriorityBindingExpression /// property from which to retrieve the PriorityBindingExpression ///target and dp cannot be null public static PriorityBindingExpression GetPriorityBindingExpression(DependencyObject target, DependencyProperty dp) { return GetBindingExpressionBase(target, dp) as PriorityBindingExpression; } ////// Remove data Binding (if any) from a property. /// ////// If the given property is data-bound, via a Binding, PriorityBinding or MultiBinding, /// the BindingExpression is removed, and the property's value changes to what it /// would be as if no local value had ever been set. /// If the given property is not data-bound, this method has no effect. /// /// object from which to remove Binding /// property from which to remove Binding ///target and dp cannot be null public static void ClearBinding(DependencyObject target, DependencyProperty dp) { if (target == null) throw new ArgumentNullException("target"); if (dp == null) throw new ArgumentNullException("dp"); // target.VerifyAccess(); if (IsDataBound(target, dp)) target.ClearValue(dp); } ////// Remove all data Binding (if any) from a DependencyObject. /// /// object from which to remove bindings ///DependencyObject target cannot be null public static void ClearAllBindings(DependencyObject target) { if (target == null) throw new ArgumentNullException("target"); // target.VerifyAccess(); LocalValueEnumerator lve = target.GetLocalValueEnumerator(); // Batch properties that have BindingExpressions since clearing // during a local value enumeration is illegal ArrayList batch = new ArrayList(8); while (lve.MoveNext()) { LocalValueEntry entry = lve.Current; if (IsDataBound(target, entry.Property)) { batch.Add(entry.Property); } } // Clear all properties that are storing BindingExpressions for (int i = 0; i < batch.Count; i++) { target.ClearValue((DependencyProperty)batch[i]); } } ///Return true if the property is currently data-bound ///DependencyObject target cannot be null public static bool IsDataBound(DependencyObject target, DependencyProperty dp) { if (target == null) throw new ArgumentNullException("target"); if (dp == null) throw new ArgumentNullException("dp"); // target.VerifyAccess(); object o = StyleHelper.GetExpression(target, dp); return (o is BindingExpressionBase); } //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- // return false if this is an invalid value for UpdateSourceTrigger internal static bool IsValidUpdateSourceTrigger(UpdateSourceTrigger value) { switch (value) { case UpdateSourceTrigger.Default: case UpdateSourceTrigger.PropertyChanged: case UpdateSourceTrigger.LostFocus: case UpdateSourceTrigger.Explicit: return true; default: return false; } } // The following properties and methods have no internal callers. They // can be called by suitably privileged external callers via reflection. // They are intended to be used by test programs and the DRT. // Enable or disable the cleanup pass. For use by tests that measure // perf, to avoid noise from the cleanup pass. internal static bool IsCleanupEnabled { get { return DataBindEngine.CurrentDataBindEngine.CleanupEnabled; } set { DataBindEngine.CurrentDataBindEngine.CleanupEnabled = value; } } // Force a cleanup pass (even if IsCleanupEnabled is true). For use // by leak-detection tests, to avoid false leak reports about objects // held by the DataBindEngine that can be cleaned up. Returns true // if something was actually cleaned up. internal static bool Cleanup() { return DataBindEngine.CurrentDataBindEngine.Cleanup(); } // Print various interesting statistics internal static void PrintStats() { DataBindEngine.CurrentDataBindEngine.AccessorTable.PrintStats(); } // Trace the size of the accessor table after each generation internal static bool TraceAccessorTableSize { get { return DataBindEngine.CurrentDataBindEngine.AccessorTable.TraceSize; } set { DataBindEngine.CurrentDataBindEngine.AccessorTable.TraceSize = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
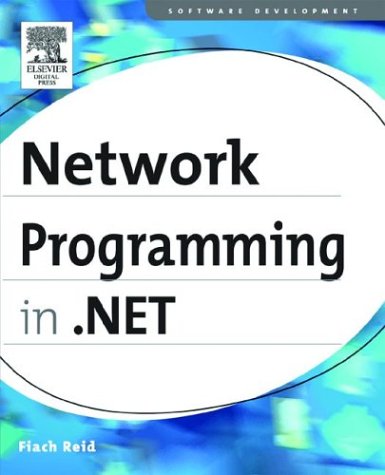
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPart.cs
- WebReferencesBuildProvider.cs
- safelinkcollection.cs
- MsmqDiagnostics.cs
- DocumentGridPage.cs
- EntityDataSourceView.cs
- SqlPersonalizationProvider.cs
- DateTimeValueSerializerContext.cs
- XmlSchemaProviderAttribute.cs
- QuadraticBezierSegment.cs
- BooleanConverter.cs
- UInt16.cs
- _AutoWebProxyScriptEngine.cs
- ViewSimplifier.cs
- MemberMaps.cs
- XamlFrame.cs
- COM2PictureConverter.cs
- NamespaceQuery.cs
- WindowsFormsSynchronizationContext.cs
- GroupBoxRenderer.cs
- WebBrowserNavigatingEventHandler.cs
- WrappedIUnknown.cs
- CaseInsensitiveOrdinalStringComparer.cs
- ConnectionStringsExpressionBuilder.cs
- WebPartUserCapability.cs
- Point3DKeyFrameCollection.cs
- WebBrowserUriTypeConverter.cs
- GroupQuery.cs
- BinaryFormatterWriter.cs
- EntityContainerAssociationSetEnd.cs
- SecurityUniqueId.cs
- GeometryCollection.cs
- RepeatBehavior.cs
- ConfigurationElementProperty.cs
- ServerReliableChannelBinder.cs
- XmlHierarchicalDataSourceView.cs
- ScalarOps.cs
- SqlDataSourceWizardForm.cs
- LabelLiteral.cs
- InheritablePropertyChangeInfo.cs
- CorrelationValidator.cs
- ReverseInheritProperty.cs
- MiniLockedBorderGlyph.cs
- Brush.cs
- InProcStateClientManager.cs
- TaskExtensions.cs
- HighContrastHelper.cs
- CharAnimationUsingKeyFrames.cs
- CellLabel.cs
- ScopedMessagePartSpecification.cs
- MaterialCollection.cs
- ObjectDataSource.cs
- ToolStripPanelRenderEventArgs.cs
- UInt16Storage.cs
- AttachmentCollection.cs
- AdCreatedEventArgs.cs
- MetadataWorkspace.cs
- DtdParser.cs
- GregorianCalendar.cs
- FreezableDefaultValueFactory.cs
- RichTextBox.cs
- RectangleGeometry.cs
- LicenseManager.cs
- HtmlInputSubmit.cs
- basecomparevalidator.cs
- datacache.cs
- LookupBindingPropertiesAttribute.cs
- ScriptManager.cs
- UserControlBuildProvider.cs
- LoadedOrUnloadedOperation.cs
- InternalCache.cs
- PageCache.cs
- SegmentInfo.cs
- InternalControlCollection.cs
- LinqDataSourceView.cs
- XmlnsPrefixAttribute.cs
- HttpListenerRequestUriBuilder.cs
- Line.cs
- HostingEnvironmentSection.cs
- Matrix3D.cs
- XhtmlCssHandler.cs
- RuntimeConfig.cs
- SimpleBitVector32.cs
- Light.cs
- Domain.cs
- MenuCommand.cs
- EventListenerClientSide.cs
- AdobeCFFWrapper.cs
- SqlUnionizer.cs
- SQLInt32.cs
- SynchronizedInputAdaptor.cs
- CompiledQueryCacheKey.cs
- EncoderExceptionFallback.cs
- XmlReader.cs
- LayoutDump.cs
- DataGridItemCollection.cs
- ReadOnlyDictionary.cs
- MergeFailedEvent.cs
- AnnotationComponentManager.cs
- Misc.cs