Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataWeb / Server / System / Data / Services / SegmentInfo.cs / 1 / SegmentInfo.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Structure containing information about a segment (Uri is made // up of bunch of segments, each segment is seperated by '/' character) // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { using System; using System.Collections; using System.Data.Services.Providers; using System.Diagnostics; using System.Linq; ///Contains the information regarding a segment that makes up the uri [DebuggerDisplay("SegmentInfo={Identifier} -> {TargetKind} '{TargetElementType}'")] internal class SegmentInfo { ///Returns the identifier for this segment i.e. string part without the keys. private string identifier; ///Returns the values that constitute the key as specified in the request. private KeyInstance key; ///Returns the query that's being composed for this segment private IEnumerable requestEnumerable; ///Whether the segment targets a single result or not. private bool singleResult; ///Resource container if applicable. private ResourceContainer targetContainer; ///The type of element targeted by this segment. private Type targetElementType; ///The kind of resource targeted by this segment. private RequestTargetKind targetKind; ///Returns the source for this segment private RequestTargetSource targetSource; ///Service operation being invoked. private ServiceOperation operation; ///Operation parameters. private object[] operationParameters; ///Returns the property that is being projected in this segment, if there's any. private ResourceProperty projectedProperty; ///Empty constructor. internal SegmentInfo() { } ///Copy constructor. /// Anotherto get a shallow copy of. internal SegmentInfo(SegmentInfo other) { Debug.Assert(other != null, "other != null"); this.Identifier = other.Identifier; this.Key = other.Key; this.Operation = other.Operation; this.OperationParameters = other.OperationParameters; this.ProjectedProperty = other.ProjectedProperty; this.RequestEnumerable = other.RequestEnumerable; this.SingleResult = other.SingleResult; this.TargetContainer = other.TargetContainer; this.TargetElementType = other.TargetElementType; this.TargetKind = other.TargetKind; this.TargetSource = other.TargetSource; } /// Returns the identifier for this segment i.e. string part without the keys. internal string Identifier { get { return this.identifier; } set { this.identifier = value; } } ///Returns the values that constitute the key as specified in the request. internal KeyInstance Key { get { return this.key; } set { this.key = value; } } ///Returns the query that's being composed for this segment internal IEnumerable RequestEnumerable { get { return this.requestEnumerable; } set { this.requestEnumerable = value; } } ///Whether the segment targets a single result or not. internal bool SingleResult { get { return this.singleResult; } set { this.singleResult = value; } } ///Resource container if applicable. internal ResourceContainer TargetContainer { get { return this.targetContainer; } set { this.targetContainer = value; } } ///The type of element targeted by this segment. internal Type TargetElementType { get { return this.targetElementType; } set { this.targetElementType = value; } } ///The kind of resource targeted by this segment. internal RequestTargetKind TargetKind { get { return this.targetKind; } set { this.targetKind = value; } } ///Returns the source for this segment internal RequestTargetSource TargetSource { get { return this.targetSource; } set { this.targetSource = value; } } ///Service operation being invoked. internal ServiceOperation Operation { get { return this.operation; } set { this.operation = value; } } ///Operation parameters. internal object[] OperationParameters { get { return this.operationParameters; } set { this.operationParameters = value; } } ///Returns the property that is being projected in this segment, if there's any. internal ResourceProperty ProjectedProperty { get { return this.projectedProperty; } set { this.projectedProperty = value; } } ///Returns true if this segment has a key filter with values; false otherwise. internal bool HasKeyValues { get { return this.Key != null && !this.Key.IsEmpty; } } ////// Determines whether the target kind is a direct reference to an element /// i.e. either you have a $value or you are accessing a resource via key property /// (/Customers(1) or /Customers(1)/BestFriend/Orders('Foo'). Either case the value /// cannot be null. /// /// Kind of request to evaluate. ////// A characteristic of a direct reference is that if its value /// is null, a 404 error should be returned. /// internal bool IsDirectReference { get { return this.TargetKind == RequestTargetKind.PrimitiveValue || #if ASTORIA_OPEN_OBJECT this.TargetKind == RequestTargetKind.OpenPropertyValue || #endif this.HasKeyValues; } } ///Returns the query for this segment, possibly null. internal IQueryable RequestQueryable { get { return this.RequestEnumerable as IQueryable; } set { this.RequestEnumerable = value; } } #if DEBUG ///In DEBUG builds, ensures that invariants for the class hold. internal void AssertValid() { WebUtil.DebugEnumIsDefined(this.TargetKind); WebUtil.DebugEnumIsDefined(this.TargetSource); Debug.Assert(this.TargetKind != RequestTargetKind.Nothing, "targetKind != RequestTargetKind.Nothing"); Debug.Assert( this.TargetContainer == null || this.TargetSource != RequestTargetSource.None, "'None' targets should not have a resource container."); Debug.Assert( this.TargetKind != RequestTargetKind.Resource || this.TargetContainer != null || #if ASTORIA_OPEN_OBJECT this.TargetKind == RequestTargetKind.OpenProperty || #endif this.TargetSource == RequestTargetSource.ServiceOperation, "All resource targets (except for some service operations and open properties) should have a container."); Debug.Assert( this.TargetContainer == null || this.TargetContainer.ElementType.IsAssignableFrom(this.TargetElementType), "If targetContainer is assigned, it should be equal to (or assignable to) the segment's element type."); Debug.Assert( !String.IsNullOrEmpty(this.Identifier) || RequestTargetSource.None == this.TargetSource || RequestTargetKind.VoidServiceOperation == this.TargetKind, "identifier must not be empty or null except for none or void service operation"); Debug.Assert( this.ProjectedProperty == null || this.TargetKind == RequestTargetKind.PrimitiveValue || this.ProjectedProperty.Name == this.Identifier, "If the property is specified, the name should match with the identifier"); } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Structure containing information about a segment (Uri is made // up of bunch of segments, each segment is seperated by '/' character) // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { using System; using System.Collections; using System.Data.Services.Providers; using System.Diagnostics; using System.Linq; ///Contains the information regarding a segment that makes up the uri [DebuggerDisplay("SegmentInfo={Identifier} -> {TargetKind} '{TargetElementType}'")] internal class SegmentInfo { ///Returns the identifier for this segment i.e. string part without the keys. private string identifier; ///Returns the values that constitute the key as specified in the request. private KeyInstance key; ///Returns the query that's being composed for this segment private IEnumerable requestEnumerable; ///Whether the segment targets a single result or not. private bool singleResult; ///Resource container if applicable. private ResourceContainer targetContainer; ///The type of element targeted by this segment. private Type targetElementType; ///The kind of resource targeted by this segment. private RequestTargetKind targetKind; ///Returns the source for this segment private RequestTargetSource targetSource; ///Service operation being invoked. private ServiceOperation operation; ///Operation parameters. private object[] operationParameters; ///Returns the property that is being projected in this segment, if there's any. private ResourceProperty projectedProperty; ///Empty constructor. internal SegmentInfo() { } ///Copy constructor. /// Anotherto get a shallow copy of. internal SegmentInfo(SegmentInfo other) { Debug.Assert(other != null, "other != null"); this.Identifier = other.Identifier; this.Key = other.Key; this.Operation = other.Operation; this.OperationParameters = other.OperationParameters; this.ProjectedProperty = other.ProjectedProperty; this.RequestEnumerable = other.RequestEnumerable; this.SingleResult = other.SingleResult; this.TargetContainer = other.TargetContainer; this.TargetElementType = other.TargetElementType; this.TargetKind = other.TargetKind; this.TargetSource = other.TargetSource; } /// Returns the identifier for this segment i.e. string part without the keys. internal string Identifier { get { return this.identifier; } set { this.identifier = value; } } ///Returns the values that constitute the key as specified in the request. internal KeyInstance Key { get { return this.key; } set { this.key = value; } } ///Returns the query that's being composed for this segment internal IEnumerable RequestEnumerable { get { return this.requestEnumerable; } set { this.requestEnumerable = value; } } ///Whether the segment targets a single result or not. internal bool SingleResult { get { return this.singleResult; } set { this.singleResult = value; } } ///Resource container if applicable. internal ResourceContainer TargetContainer { get { return this.targetContainer; } set { this.targetContainer = value; } } ///The type of element targeted by this segment. internal Type TargetElementType { get { return this.targetElementType; } set { this.targetElementType = value; } } ///The kind of resource targeted by this segment. internal RequestTargetKind TargetKind { get { return this.targetKind; } set { this.targetKind = value; } } ///Returns the source for this segment internal RequestTargetSource TargetSource { get { return this.targetSource; } set { this.targetSource = value; } } ///Service operation being invoked. internal ServiceOperation Operation { get { return this.operation; } set { this.operation = value; } } ///Operation parameters. internal object[] OperationParameters { get { return this.operationParameters; } set { this.operationParameters = value; } } ///Returns the property that is being projected in this segment, if there's any. internal ResourceProperty ProjectedProperty { get { return this.projectedProperty; } set { this.projectedProperty = value; } } ///Returns true if this segment has a key filter with values; false otherwise. internal bool HasKeyValues { get { return this.Key != null && !this.Key.IsEmpty; } } ////// Determines whether the target kind is a direct reference to an element /// i.e. either you have a $value or you are accessing a resource via key property /// (/Customers(1) or /Customers(1)/BestFriend/Orders('Foo'). Either case the value /// cannot be null. /// /// Kind of request to evaluate. ////// A characteristic of a direct reference is that if its value /// is null, a 404 error should be returned. /// internal bool IsDirectReference { get { return this.TargetKind == RequestTargetKind.PrimitiveValue || #if ASTORIA_OPEN_OBJECT this.TargetKind == RequestTargetKind.OpenPropertyValue || #endif this.HasKeyValues; } } ///Returns the query for this segment, possibly null. internal IQueryable RequestQueryable { get { return this.RequestEnumerable as IQueryable; } set { this.RequestEnumerable = value; } } #if DEBUG ///In DEBUG builds, ensures that invariants for the class hold. internal void AssertValid() { WebUtil.DebugEnumIsDefined(this.TargetKind); WebUtil.DebugEnumIsDefined(this.TargetSource); Debug.Assert(this.TargetKind != RequestTargetKind.Nothing, "targetKind != RequestTargetKind.Nothing"); Debug.Assert( this.TargetContainer == null || this.TargetSource != RequestTargetSource.None, "'None' targets should not have a resource container."); Debug.Assert( this.TargetKind != RequestTargetKind.Resource || this.TargetContainer != null || #if ASTORIA_OPEN_OBJECT this.TargetKind == RequestTargetKind.OpenProperty || #endif this.TargetSource == RequestTargetSource.ServiceOperation, "All resource targets (except for some service operations and open properties) should have a container."); Debug.Assert( this.TargetContainer == null || this.TargetContainer.ElementType.IsAssignableFrom(this.TargetElementType), "If targetContainer is assigned, it should be equal to (or assignable to) the segment's element type."); Debug.Assert( !String.IsNullOrEmpty(this.Identifier) || RequestTargetSource.None == this.TargetSource || RequestTargetKind.VoidServiceOperation == this.TargetKind, "identifier must not be empty or null except for none or void service operation"); Debug.Assert( this.ProjectedProperty == null || this.TargetKind == RequestTargetKind.PrimitiveValue || this.ProjectedProperty.Name == this.Identifier, "If the property is specified, the name should match with the identifier"); } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
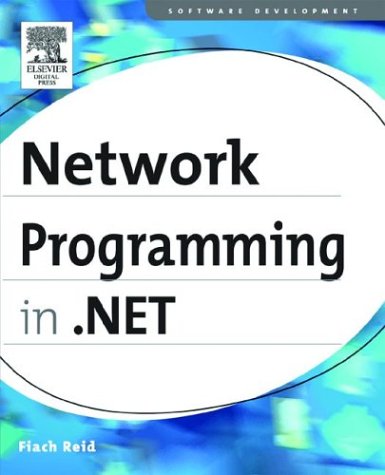
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DateTimeSerializationSection.cs
- CharAnimationUsingKeyFrames.cs
- NameHandler.cs
- WebPermission.cs
- WindowsTreeView.cs
- DataSourceView.cs
- ScaleTransform.cs
- Symbol.cs
- ToolboxDataAttribute.cs
- ErrorRuntimeConfig.cs
- WebPartConnectionCollection.cs
- _AuthenticationState.cs
- XmlSchemaSimpleType.cs
- XmlNamespaceMappingCollection.cs
- ContextStaticAttribute.cs
- DataKeyArray.cs
- EntityDataSourceEntityTypeFilterItem.cs
- SqlDependency.cs
- DrawingImage.cs
- DrawingCollection.cs
- BackgroundFormatInfo.cs
- XmlEncApr2001.cs
- BooleanSwitch.cs
- DetailsViewAutoFormat.cs
- AvTraceDetails.cs
- DockingAttribute.cs
- TiffBitmapDecoder.cs
- ShapeTypeface.cs
- XPathDocumentIterator.cs
- FormViewRow.cs
- DataObjectFieldAttribute.cs
- HttpWebResponse.cs
- SafeProcessHandle.cs
- InputProcessorProfiles.cs
- ReadOnlyDictionary.cs
- RepeaterItemCollection.cs
- CloudCollection.cs
- TreeNodeBinding.cs
- PerformanceCounterTraceRecord.cs
- SystemInformation.cs
- MessageBox.cs
- RequiredFieldValidator.cs
- ColorBlend.cs
- EnumConverter.cs
- LinkArea.cs
- XmlNavigatorStack.cs
- ADConnectionHelper.cs
- PeerApplicationLaunchInfo.cs
- CodeMemberProperty.cs
- DocumentGrid.cs
- InvalidEnumArgumentException.cs
- IdnMapping.cs
- WindowExtensionMethods.cs
- RequiredArgumentAttribute.cs
- ActivityExecutionFilter.cs
- SQLBytes.cs
- Registry.cs
- CqlParser.cs
- DataGridViewBindingCompleteEventArgs.cs
- DataSourceNameHandler.cs
- ConstraintConverter.cs
- Image.cs
- ValidationHelper.cs
- DiffuseMaterial.cs
- Token.cs
- MatrixValueSerializer.cs
- StrokeCollection2.cs
- ConfigXmlElement.cs
- Constant.cs
- EdmConstants.cs
- CodeFieldReferenceExpression.cs
- TcpTransportBindingElement.cs
- ThreadInterruptedException.cs
- AppDomainManager.cs
- ClientData.cs
- SessionStateSection.cs
- CqlBlock.cs
- SettingsPropertyIsReadOnlyException.cs
- KeyMatchBuilder.cs
- _SslSessionsCache.cs
- StylesEditorDialog.cs
- ListViewItemCollectionEditor.cs
- CodeMemberProperty.cs
- LocatorPartList.cs
- WindowsTreeView.cs
- SqlComparer.cs
- StreamWithDictionary.cs
- CodeExpressionCollection.cs
- MessageQueuePermission.cs
- DetailsViewDeleteEventArgs.cs
- FixedSOMElement.cs
- RtfControlWordInfo.cs
- BooleanFunctions.cs
- MessageAction.cs
- SQLStringStorage.cs
- CalendarAutoFormatDialog.cs
- TaskExceptionHolder.cs
- QilChoice.cs
- Bidi.cs
- SqlPersonalizationProvider.cs