Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / XmlEncApr2001.cs / 1 / XmlEncApr2001.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Security { using HexBinary = System.Runtime.Remoting.Metadata.W3cXsd2001.SoapHexBinary; using System; using System.ServiceModel; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.IO; using System.Text; using System.Threading; using System.Xml; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.IdentityModel.Tokens; using System.IdentityModel.Selectors; using System.Security.Cryptography.X509Certificates; using System.ServiceModel.Security.Tokens; using System.ServiceModel.Channels; using System.ServiceModel.Security; using System.Runtime.Serialization; using KeyIdentifierEntry = WSSecurityTokenSerializer.KeyIdentifierEntry; using KeyIdentifierClauseEntry = WSSecurityTokenSerializer.KeyIdentifierClauseEntry; using TokenEntry = WSSecurityTokenSerializer.TokenEntry; using SignedXml = System.IdentityModel.SignedXml; class XmlEncApr2001 : WSSecurityTokenSerializer.SerializerEntries { WSSecurityTokenSerializer tokenSerializer; public XmlEncApr2001(WSSecurityTokenSerializer tokenSerializer) { this.tokenSerializer = tokenSerializer; } public override void PopulateKeyIdentifierClauseEntries(IListkeyIdentifierClauseEntries) { keyIdentifierClauseEntries.Add(new EncryptedKeyClauseEntry(this.tokenSerializer)); } internal class EncryptedKeyClauseEntry : KeyIdentifierClauseEntry { WSSecurityTokenSerializer tokenSerializer; public EncryptedKeyClauseEntry(WSSecurityTokenSerializer tokenSerializer) { this.tokenSerializer = tokenSerializer; } protected override XmlDictionaryString LocalName { get { return XD.XmlEncryptionDictionary.EncryptedKey; } } protected override XmlDictionaryString NamespaceUri { get { return XD.XmlEncryptionDictionary.Namespace; } } public override SecurityKeyIdentifierClause ReadKeyIdentifierClauseCore(XmlDictionaryReader reader) { string encryptionMethod = null; string carriedKeyName = null; SecurityKeyIdentifier encryptingKeyIdentifier = null; byte[] encryptedKey = null; reader.ReadStartElement(XD.XmlEncryptionDictionary.EncryptedKey, NamespaceUri); if (reader.IsStartElement(XD.XmlEncryptionDictionary.EncryptionMethod, NamespaceUri)) { encryptionMethod = reader.GetAttribute(XD.XmlEncryptionDictionary.AlgorithmAttribute, null); bool isEmptyElement = reader.IsEmptyElement; reader.ReadStartElement(); if (!isEmptyElement) { while (reader.IsStartElement()) { reader.Skip(); } reader.ReadEndElement(); } } if (this.tokenSerializer.CanReadKeyIdentifier(reader)) { encryptingKeyIdentifier = this.tokenSerializer.ReadKeyIdentifier(reader); } reader.ReadStartElement(XD.XmlEncryptionDictionary.CipherData, NamespaceUri); reader.ReadStartElement(XD.XmlEncryptionDictionary.CipherValue, NamespaceUri); encryptedKey = reader.ReadContentAsBase64(); reader.ReadEndElement(); reader.ReadEndElement(); if (reader.IsStartElement(XD.XmlEncryptionDictionary.CarriedKeyName, NamespaceUri)) { reader.ReadStartElement(); carriedKeyName = reader.ReadString(); reader.ReadEndElement(); } reader.ReadEndElement(); return new EncryptedKeyIdentifierClause(encryptedKey, encryptionMethod, encryptingKeyIdentifier, carriedKeyName); } public override bool SupportsCore(SecurityKeyIdentifierClause keyIdentifierClause) { return keyIdentifierClause is EncryptedKeyIdentifierClause; } public override void WriteKeyIdentifierClauseCore(XmlDictionaryWriter writer, SecurityKeyIdentifierClause keyIdentifierClause) { EncryptedKeyIdentifierClause encryptedKeyClause = keyIdentifierClause as EncryptedKeyIdentifierClause; writer.WriteStartElement(XD.XmlEncryptionDictionary.Prefix.Value, XD.XmlEncryptionDictionary.EncryptedKey, NamespaceUri); if (encryptedKeyClause.EncryptionMethod != null) { writer.WriteStartElement(XD.XmlEncryptionDictionary.Prefix.Value, XD.XmlEncryptionDictionary.EncryptionMethod, NamespaceUri); writer.WriteAttributeString(XD.XmlEncryptionDictionary.AlgorithmAttribute, null, encryptedKeyClause.EncryptionMethod); if (encryptedKeyClause.EncryptionMethod == XD.SecurityAlgorithmDictionary.RsaOaepKeyWrap.Value) { writer.WriteStartElement(XmlSignatureStrings.Prefix, XD.XmlSignatureDictionary.DigestMethod, XD.XmlSignatureDictionary.Namespace); writer.WriteAttributeString(XD.XmlSignatureDictionary.Algorithm, null, SecurityAlgorithms.Sha1Digest); writer.WriteEndElement(); } writer.WriteEndElement(); } if (encryptedKeyClause.EncryptingKeyIdentifier != null) { this.tokenSerializer.WriteKeyIdentifier(writer, encryptedKeyClause.EncryptingKeyIdentifier); } writer.WriteStartElement(XD.XmlEncryptionDictionary.Prefix.Value, XD.XmlEncryptionDictionary.CipherData, NamespaceUri); writer.WriteStartElement(XD.XmlEncryptionDictionary.Prefix.Value, XD.XmlEncryptionDictionary.CipherValue, NamespaceUri); byte[] encryptedKey = encryptedKeyClause.GetEncryptedKey(); writer.WriteBase64(encryptedKey, 0, encryptedKey.Length); writer.WriteEndElement(); writer.WriteEndElement(); if (encryptedKeyClause.CarriedKeyName != null) { writer.WriteElementString(XD.XmlEncryptionDictionary.Prefix.Value, XD.XmlEncryptionDictionary.CarriedKeyName, NamespaceUri, encryptedKeyClause.CarriedKeyName); } writer.WriteEndElement(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
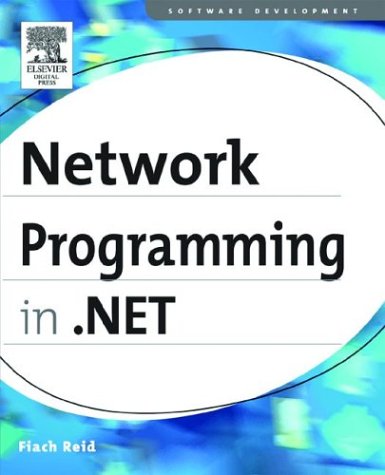
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewItem.cs
- BCryptNative.cs
- UInt64Storage.cs
- TokenCreationException.cs
- AsyncPostBackErrorEventArgs.cs
- EncodingTable.cs
- ByteKeyFrameCollection.cs
- BuiltInExpr.cs
- WMIInterop.cs
- CodeDirectionExpression.cs
- Connection.cs
- CapabilitiesRule.cs
- WindowsScrollBar.cs
- DataGridTable.cs
- FlowDecision.cs
- DictionaryBase.cs
- MenuItemStyle.cs
- UniqueCodeIdentifierScope.cs
- TypefaceMetricsCache.cs
- ComNativeDescriptor.cs
- SByte.cs
- MapPathBasedVirtualPathProvider.cs
- ClientFormsIdentity.cs
- CommandSet.cs
- XXXOnTypeBuilderInstantiation.cs
- CardSpaceSelector.cs
- UntrustedRecipientException.cs
- XamlStream.cs
- FactoryGenerator.cs
- BuildProviderCollection.cs
- EncodingTable.cs
- VisualStyleRenderer.cs
- ClientApiGenerator.cs
- AnnotationAuthorChangedEventArgs.cs
- IConvertible.cs
- BlockUIContainer.cs
- TextEditor.cs
- CfgArc.cs
- DateTimeOffsetConverter.cs
- StorageConditionPropertyMapping.cs
- PowerModeChangedEventArgs.cs
- EmptyCollection.cs
- QueryReaderSettings.cs
- LocatorPart.cs
- JoinQueryOperator.cs
- ClientFormsIdentity.cs
- SpeechSynthesizer.cs
- InheritanceService.cs
- RegisteredHiddenField.cs
- GlyphRun.cs
- DataGridItemAttachedStorage.cs
- ChannelBinding.cs
- TerminatorSinks.cs
- FixedStringLookup.cs
- DataBindingHandlerAttribute.cs
- BaseParser.cs
- LifetimeServices.cs
- CompleteWizardStep.cs
- ControlAdapter.cs
- PerfService.cs
- DrawingAttributeSerializer.cs
- IdentityNotMappedException.cs
- PathFigure.cs
- BaseTemplateBuildProvider.cs
- NewExpression.cs
- InvokeCompletedEventArgs.cs
- StylusPointDescription.cs
- PrintEvent.cs
- PtsCache.cs
- DataControlFieldCollection.cs
- XmlObjectSerializerReadContext.cs
- LocalizabilityAttribute.cs
- WebPartsPersonalization.cs
- BindingsSection.cs
- Compiler.cs
- AllMembershipCondition.cs
- XmlSchemaComplexContentRestriction.cs
- VariableQuery.cs
- updateconfighost.cs
- AddressUtility.cs
- FrameDimension.cs
- EntityParameterCollection.cs
- TextTrailingWordEllipsis.cs
- RayHitTestParameters.cs
- OleDbEnumerator.cs
- DiscardableAttribute.cs
- OperatingSystem.cs
- WSSecureConversationFeb2005.cs
- RecordManager.cs
- SplitterDesigner.cs
- COSERVERINFO.cs
- OleAutBinder.cs
- AttributeEmitter.cs
- WpfGeneratedKnownProperties.cs
- WebSysDisplayNameAttribute.cs
- DataGridViewCellEventArgs.cs
- NetworkInterface.cs
- HTMLTextWriter.cs
- XamlTreeBuilderBamlRecordWriter.cs
- WebPartManagerInternals.cs