Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Markup / XamlTreeBuilderBamlRecordWriter.cs / 1 / XamlTreeBuilderBamlRecordWriter.cs
/****************************************************************************\ * * File: XamlTreeBuilderBamlRecordWriter.cs * * Purpose: Class that writes baml records when building a tree directly * from xaml * * History: * 6/06/01: rogerg Created * 5/29/03: peterost Ported to wcp * 11/13/03: peterost split from XamlTreeBuilder.cs * * Copyright (C) 2003 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Windows; using System.Text; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using MS.Utility; namespace System.Windows.Markup { ////// Override class for BamlRecordWriter when building tree Directly from XAML. /// We do this so in the [....] case we can build the tree directly instead /// of writing to a BAMLStream. Note that building a tree directly from xaml /// DOES NOT support defer loaded content /// internal class XamlTreeBuilderBamlRecordWriter : BamlRecordWriter { #region Constructors internal XamlTreeBuilderBamlRecordWriter( XamlTreeBuilder treeBuilder, Stream stream, ParserContext parserContext, bool isSerializer ) : base(stream,parserContext,false) { _treeBuilder = treeBuilder; _writerStream = stream as WriterStream; // If we're in a nested parser, then we need to track it in our // _customSerializerNestingLevel counter. Otherwise we'll flush // Baml records to the reader thread too soon in WriteBamlRecord. if( isSerializer ) { _customSerializerNestingLevel = 1; } Debug.Assert(null != _writerStream,"Stream for Writer is not a WriterStream"); } #endregion Constructors #region Overrides internal override void WriteBamlRecord( BamlRecord bamlRecord, int lineNumber, int linePosition) { bool bamlRecordHandled = false; // Try to load the Baml record. This will work if we're in [....] mode // (default case), or if we're in async mode and processing the root. bamlRecordHandled = TreeBuilder.BamlRecordWriterSyncUpdate(bamlRecord, lineNumber, linePosition); // If it didn't get handled, write the baml record to the baml stream. if (!bamlRecordHandled) { // Write the record. base.WriteBamlRecord(bamlRecord, lineNumber, linePosition); // Nowe we see if we can let the reader see this record. We can't if we're within // a custom serializer. (This was added so that templates & styles wouldn't get sealed // before being fully loaded, an alternative would be to use ISupportInitialize.) // Is this a start record? BamlElementStartRecord bamlElementStartRecord = bamlRecord as BamlElementStartRecord; if (bamlElementStartRecord != null) { // Yes, it's a start record. If we're not already under a custom serializer, // but this element has one, then start nesting. if (_customSerializerNestingLevel == 0 && MapTable.HasSerializerForTypeId(bamlElementStartRecord.TypeId)) { _customSerializerNestingLevel = 1; } // Or, if we're already under a custom serializer, increment the nesting level. else if (_customSerializerNestingLevel > 0) { ++_customSerializerNestingLevel; } } // This isn't a start record. But if we're under a custom serializer, // we might need to update the nesting level. else if (_customSerializerNestingLevel != 0) { // If this is an end element record, decrement the nesting level. BamlElementEndRecord bamlElementEndRecord = bamlRecord as BamlElementEndRecord; if (bamlElementEndRecord != null) { --_customSerializerNestingLevel; } } // If we're not (now) under a custom serializer context, tell the reader // about all of the records we've written. if (_customSerializerNestingLevel == 0) { WriterStream.UpdateReaderLength(WriterStream.Length ); } } } ////// sets the number of Records that can be read at a time /// in async mode. main use is for debugging. /// /// number of Records we are allowed to read internal override void SetMaxAsyncRecords(int maxAsyncRecords) { TreeBuilder.MaxAsyncRecords = maxAsyncRecords; } ////// Called to determine if it is okay for ParentNodes to be updated /// since building the tree directly we can't seek back to update /// the parent nodes so false is always returned. /// internal override bool UpdateParentNodes { get { return false; } } // If building a tree directly, just store the strings directly in the BAML record. // Don't have to precreate the DP object, stream it out and then re-create it as this // is extra work, so custom serialization is not allowed. internal override void WriteProperty(XamlPropertyNode xamlPropertyNode) { BaseWriteProperty(xamlPropertyNode); } #endregion Overrides #region Properties ////// TreeBuilder associated with this class /// internal XamlTreeBuilder TreeBuilder { get { return _treeBuilder; } } ////// Writer stream records are written to. /// Hold onto this so we can updateThe Reader length to keep the /// BamlRecords class unaware of the ReaderWriter Stream /// private WriterStream WriterStream { get { return _writerStream; } } ////// When creating a tree directly from Xaml, use the record reader's /// BamlRecordManager, since its record releasing behavior is modified /// based on the markup it is reading in /// internal override BamlRecordManager BamlRecordManager { get { return _treeBuilder.RecordReader.BamlRecordManager; } } #endregion Properties #region Data XamlTreeBuilder _treeBuilder; WriterStream _writerStream; int _customSerializerNestingLevel = 0; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: XamlTreeBuilderBamlRecordWriter.cs * * Purpose: Class that writes baml records when building a tree directly * from xaml * * History: * 6/06/01: rogerg Created * 5/29/03: peterost Ported to wcp * 11/13/03: peterost split from XamlTreeBuilder.cs * * Copyright (C) 2003 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Windows; using System.Text; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using MS.Utility; namespace System.Windows.Markup { ////// Override class for BamlRecordWriter when building tree Directly from XAML. /// We do this so in the [....] case we can build the tree directly instead /// of writing to a BAMLStream. Note that building a tree directly from xaml /// DOES NOT support defer loaded content /// internal class XamlTreeBuilderBamlRecordWriter : BamlRecordWriter { #region Constructors internal XamlTreeBuilderBamlRecordWriter( XamlTreeBuilder treeBuilder, Stream stream, ParserContext parserContext, bool isSerializer ) : base(stream,parserContext,false) { _treeBuilder = treeBuilder; _writerStream = stream as WriterStream; // If we're in a nested parser, then we need to track it in our // _customSerializerNestingLevel counter. Otherwise we'll flush // Baml records to the reader thread too soon in WriteBamlRecord. if( isSerializer ) { _customSerializerNestingLevel = 1; } Debug.Assert(null != _writerStream,"Stream for Writer is not a WriterStream"); } #endregion Constructors #region Overrides internal override void WriteBamlRecord( BamlRecord bamlRecord, int lineNumber, int linePosition) { bool bamlRecordHandled = false; // Try to load the Baml record. This will work if we're in [....] mode // (default case), or if we're in async mode and processing the root. bamlRecordHandled = TreeBuilder.BamlRecordWriterSyncUpdate(bamlRecord, lineNumber, linePosition); // If it didn't get handled, write the baml record to the baml stream. if (!bamlRecordHandled) { // Write the record. base.WriteBamlRecord(bamlRecord, lineNumber, linePosition); // Nowe we see if we can let the reader see this record. We can't if we're within // a custom serializer. (This was added so that templates & styles wouldn't get sealed // before being fully loaded, an alternative would be to use ISupportInitialize.) // Is this a start record? BamlElementStartRecord bamlElementStartRecord = bamlRecord as BamlElementStartRecord; if (bamlElementStartRecord != null) { // Yes, it's a start record. If we're not already under a custom serializer, // but this element has one, then start nesting. if (_customSerializerNestingLevel == 0 && MapTable.HasSerializerForTypeId(bamlElementStartRecord.TypeId)) { _customSerializerNestingLevel = 1; } // Or, if we're already under a custom serializer, increment the nesting level. else if (_customSerializerNestingLevel > 0) { ++_customSerializerNestingLevel; } } // This isn't a start record. But if we're under a custom serializer, // we might need to update the nesting level. else if (_customSerializerNestingLevel != 0) { // If this is an end element record, decrement the nesting level. BamlElementEndRecord bamlElementEndRecord = bamlRecord as BamlElementEndRecord; if (bamlElementEndRecord != null) { --_customSerializerNestingLevel; } } // If we're not (now) under a custom serializer context, tell the reader // about all of the records we've written. if (_customSerializerNestingLevel == 0) { WriterStream.UpdateReaderLength(WriterStream.Length ); } } } ////// sets the number of Records that can be read at a time /// in async mode. main use is for debugging. /// /// number of Records we are allowed to read internal override void SetMaxAsyncRecords(int maxAsyncRecords) { TreeBuilder.MaxAsyncRecords = maxAsyncRecords; } ////// Called to determine if it is okay for ParentNodes to be updated /// since building the tree directly we can't seek back to update /// the parent nodes so false is always returned. /// internal override bool UpdateParentNodes { get { return false; } } // If building a tree directly, just store the strings directly in the BAML record. // Don't have to precreate the DP object, stream it out and then re-create it as this // is extra work, so custom serialization is not allowed. internal override void WriteProperty(XamlPropertyNode xamlPropertyNode) { BaseWriteProperty(xamlPropertyNode); } #endregion Overrides #region Properties ////// TreeBuilder associated with this class /// internal XamlTreeBuilder TreeBuilder { get { return _treeBuilder; } } ////// Writer stream records are written to. /// Hold onto this so we can updateThe Reader length to keep the /// BamlRecords class unaware of the ReaderWriter Stream /// private WriterStream WriterStream { get { return _writerStream; } } ////// When creating a tree directly from Xaml, use the record reader's /// BamlRecordManager, since its record releasing behavior is modified /// based on the markup it is reading in /// internal override BamlRecordManager BamlRecordManager { get { return _treeBuilder.RecordReader.BamlRecordManager; } } #endregion Properties #region Data XamlTreeBuilder _treeBuilder; WriterStream _writerStream; int _customSerializerNestingLevel = 0; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
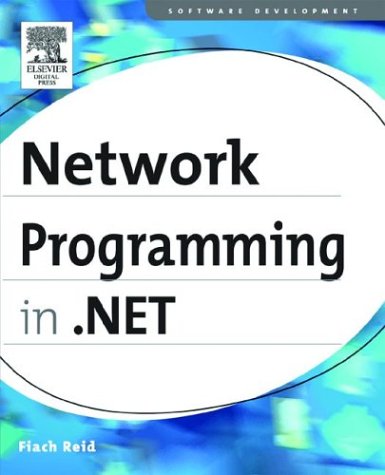
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DefaultProxySection.cs
- Int16Converter.cs
- TextTreeTextNode.cs
- BamlRecordHelper.cs
- SamlAssertion.cs
- MergeFilterQuery.cs
- VerticalConnector.xaml.cs
- AbstractDataSvcMapFileLoader.cs
- ImageAttributes.cs
- MarshalDirectiveException.cs
- HttpServerUtilityWrapper.cs
- DataBoundControl.cs
- IItemContainerGenerator.cs
- RootBrowserWindow.cs
- UnsafeNativeMethodsCLR.cs
- HttpCookieCollection.cs
- CodeCommentStatementCollection.cs
- SqlRewriteScalarSubqueries.cs
- PerformanceCounterPermission.cs
- EdmPropertyAttribute.cs
- DiscoveryDocumentLinksPattern.cs
- Selector.cs
- Transform3D.cs
- CodeDOMProvider.cs
- AdapterUtil.cs
- TextPointerBase.cs
- CodeNamespaceCollection.cs
- StorageInfo.cs
- HwndTarget.cs
- EffectiveValueEntry.cs
- TypeUsageBuilder.cs
- ConfigurationException.cs
- SupportsPreviewControlAttribute.cs
- XmlSchemaSimpleContentExtension.cs
- FontFamilyConverter.cs
- ZipIOLocalFileDataDescriptor.cs
- ListBindingConverter.cs
- OdbcFactory.cs
- DataGridPagingPage.cs
- ObjectIDGenerator.cs
- WorkflowTimerService.cs
- WebPartTracker.cs
- ListDictionaryInternal.cs
- ContentElement.cs
- ReadOnlyDataSourceView.cs
- MarshalDirectiveException.cs
- DateTime.cs
- ObjectCloneHelper.cs
- OdbcConnectionString.cs
- ListViewInsertionMark.cs
- NamedObject.cs
- StandardMenuStripVerb.cs
- DebugView.cs
- RadialGradientBrush.cs
- UpdateRecord.cs
- DrawingState.cs
- SafeTokenHandle.cs
- HtmlShimManager.cs
- TdsParserHelperClasses.cs
- ProcessModuleCollection.cs
- CommentEmitter.cs
- CfgArc.cs
- UseAttributeSetsAction.cs
- ColorContext.cs
- VariantWrapper.cs
- SystemNetworkInterface.cs
- WorkflowDesigner.cs
- XmlRootAttribute.cs
- TextPattern.cs
- EntitySetBase.cs
- StorageSetMapping.cs
- SessionStateModule.cs
- ResourceBinder.cs
- EnumCodeDomSerializer.cs
- ProfilePropertyNameValidator.cs
- SqlConnection.cs
- OutputCacheProfileCollection.cs
- RoleManagerModule.cs
- IConvertible.cs
- AsyncPostBackErrorEventArgs.cs
- PresentationAppDomainManager.cs
- ArrangedElementCollection.cs
- propertytag.cs
- ReadOnlyTernaryTree.cs
- NamespaceQuery.cs
- DataRowCollection.cs
- ContextMenu.cs
- Facet.cs
- BindingList.cs
- ListBox.cs
- ModuleBuilder.cs
- CellTreeNodeVisitors.cs
- Image.cs
- SoapClientProtocol.cs
- BitmapEffectGroup.cs
- DynamicDiscoveryDocument.cs
- XmlSchemaAnnotated.cs
- ArrayTypeMismatchException.cs
- FieldCollectionEditor.cs
- MonthChangedEventArgs.cs