Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / ValidationHelper.cs / 1305600 / ValidationHelper.cs
//---------------------------------------------------------------------------- // // File: ValidationHelper.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Helpers for TOM parameter validation. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; // Invariant.Assert using System.ComponentModel; using System.Windows; using System.Windows.Media; internal static class ValidationHelper { //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods // Verifies a TextPointer is non-null and // is associated with a given TextContainer. // // Throws an appropriate exception if a test fails. internal static void VerifyPosition(ITextContainer tree, ITextPointer position) { VerifyPosition(tree, position, "position"); } // Verifies a TextPointer is non-null and is associated with a given TextContainer. // // Throws an appropriate exception if a test fails. internal static void VerifyPosition(ITextContainer container, ITextPointer position, string paramName) { if (position == null) { throw new ArgumentNullException(paramName); } if (position.TextContainer != container) { throw new ArgumentException(SR.Get(SRID.NotInAssociatedTree, paramName)); } } // Verifies two positions are safe to use as a logical text span. // // Throws ArgumentNullException if startPosition == null || endPosition == null // ArgumentException if startPosition.TextContainer != endPosition.TextContainer or // startPosition > endPosition internal static void VerifyPositionPair(ITextPointer startPosition, ITextPointer endPosition) { if (startPosition == null) { throw new ArgumentNullException("startPosition"); } if (endPosition == null) { throw new ArgumentNullException("endPosition"); } if (startPosition.TextContainer != endPosition.TextContainer) { throw new ArgumentException(SR.Get(SRID.InDifferentTextContainers, "startPosition", "endPosition")); } if (startPosition.CompareTo(endPosition) > 0) { throw new ArgumentException(SR.Get(SRID.BadTextPositionOrder, "startPosition", "endPosition")); } } // Throws an ArgumentException if direction is not a valid enum. internal static void VerifyDirection(LogicalDirection direction, string argumentName) { if (direction != LogicalDirection.Forward && direction != LogicalDirection.Backward) { throw new InvalidEnumArgumentException(argumentName, (int)direction, typeof(LogicalDirection)); } } // Throws an ArgumentException if edge is not a valid enum. internal static void VerifyElementEdge(ElementEdge edge, string param) { if (edge != ElementEdge.BeforeStart && edge != ElementEdge.AfterStart && edge != ElementEdge.BeforeEnd && edge != ElementEdge.AfterEnd) { throw new InvalidEnumArgumentException(param, (int)edge, typeof(ElementEdge)); } } // ............................................................... // // TextSchema Validation // // ............................................................... // Checks whether it is valid to insert the child object at passed position. internal static void ValidateChild(TextPointer position, object child, string paramName) { Invariant.Assert(position != null); if (child == null) { throw new ArgumentNullException(paramName); } if (!TextSchema.IsValidChild(/*position:*/position, /*childType:*/child.GetType())) { throw new ArgumentException(SR.Get(SRID.TextSchema_ChildTypeIsInvalid, position.Parent.GetType().Name, child.GetType().Name)); } // The new child should not be currently in other text tree if (child is TextElement) { if (((TextElement)child).Parent != null) { throw new ArgumentException(SR.Get(SRID.TextSchema_TheChildElementBelongsToAnotherTreeAlready, child.GetType().Name)); } } else { Invariant.Assert(child is UIElement); // Cannot call UIElement.Parent across assembly boundary. So skip this part of validation. This condition will be checked elsewhere anyway. //if (((UIElement)child).Parent != null) //{ // throw new ArgumentException(SR.Get(SRID.TextSchema_TheChildElementBelongsToAnotherTreeAlready, child.GetType().Name)); //} } } #endregion Internal methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
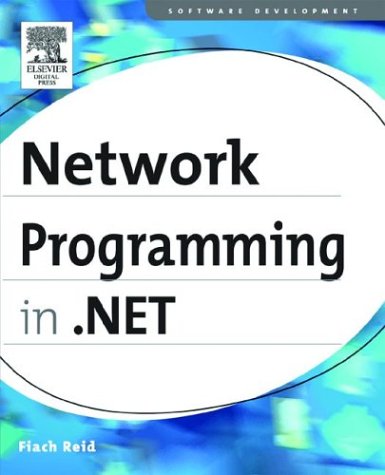
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IconHelper.cs
- ResourceReader.cs
- Ops.cs
- ISAPIWorkerRequest.cs
- FilterableAttribute.cs
- _MultipleConnectAsync.cs
- CheckBox.cs
- LineGeometry.cs
- ConditionCollection.cs
- TraceUtility.cs
- PageStatePersister.cs
- MarkupCompilePass2.cs
- BaseParagraph.cs
- HandoffBehavior.cs
- SmiConnection.cs
- ToolStripTextBox.cs
- SplitterPanel.cs
- TransformerInfoCollection.cs
- QilChoice.cs
- processwaithandle.cs
- Calendar.cs
- DependencyPropertyKind.cs
- Message.cs
- UiaCoreProviderApi.cs
- BidOverLoads.cs
- PenCursorManager.cs
- SecurityContext.cs
- Confirm.cs
- EmbeddedMailObject.cs
- SqlDependency.cs
- StringHelper.cs
- ExpressionVisitor.cs
- CheckableControlBaseAdapter.cs
- CompensationHandlingFilter.cs
- InputScope.cs
- WrappedIUnknown.cs
- JsonReaderWriterFactory.cs
- EncryptRequest.cs
- EventLogTraceListener.cs
- BindingOperations.cs
- SchemaElementLookUpTable.cs
- ViewSimplifier.cs
- PathParser.cs
- DocumentsTrace.cs
- regiisutil.cs
- StrokeNodeEnumerator.cs
- Confirm.cs
- FontDriver.cs
- TypeConverter.cs
- DebugViewWriter.cs
- ExceptionHandlersDesigner.cs
- DataServiceBehavior.cs
- Subset.cs
- UniqueID.cs
- DataGridViewRowCollection.cs
- _ProxyRegBlob.cs
- XmlParserContext.cs
- NameValueConfigurationElement.cs
- AnnotationHelper.cs
- SizeLimitedCache.cs
- MasterPageBuildProvider.cs
- RadioButtonList.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- TracedNativeMethods.cs
- BasePropertyDescriptor.cs
- HTMLTextWriter.cs
- MediaTimeline.cs
- ExitEventArgs.cs
- ValidationError.cs
- Int64Animation.cs
- WindowsToolbarItemAsMenuItem.cs
- COM2ExtendedTypeConverter.cs
- CompModSwitches.cs
- EqualityComparer.cs
- SrgsRule.cs
- InternalBufferOverflowException.cs
- AttributedMetaModel.cs
- ServiceReference.cs
- TypedTableBaseExtensions.cs
- glyphs.cs
- TreeViewImageKeyConverter.cs
- ValueUtilsSmi.cs
- TreeNodeConverter.cs
- cookiecontainer.cs
- RenderDataDrawingContext.cs
- ViewEvent.cs
- QueryOutputWriterV1.cs
- SystemResourceHost.cs
- SafeEventLogReadHandle.cs
- DataTableReader.cs
- EntityWrapperFactory.cs
- Expression.cs
- PropertyDescriptorComparer.cs
- RequestResizeEvent.cs
- DragSelectionMessageFilter.cs
- SrgsOneOf.cs
- CustomValidator.cs
- ColumnTypeConverter.cs
- HttpCookieCollection.cs
- AutomationAttributeInfo.cs