Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / UIAutomation / UIAutomationProvider / MS / Internal / Automation / UiaCoreProviderApi.cs / 1 / UiaCoreProviderApi.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Imports from unmanaged UiaCore DLL // // History: // 06/02/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Security; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Runtime.InteropServices; using Microsoft.Internal; namespace MS.Internal.Automation { internal static class UiaCoreProviderApi { //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods // // Provider-side methods... // #region Provider methods ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: This method is used to return an IRawElementProviderSimple associated with an HWND to UIAutomation in response to a WM_GETOBJECT /// The returned value is simply an LRESULT, so is harmless, and the input values are verfied on the unmanaged side, so it is not abusable. /// [SecurityCritical,SecurityTreatAsSafe] internal static IntPtr UiaReturnRawElementProvider(IntPtr hwnd, IntPtr wParam, IntPtr lParam, IRawElementProviderSimple el) { return RawUiaReturnRawElementProvider( hwnd, wParam, lParam, el ); } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: This converts an hwnd to a MiniHwndProxy, which while technically implementing IRawElementProviderSimple, has none of the functionality /// and is therefore simply a harmless hwnd container. /// [SecurityCritical,SecurityTreatAsSafe] internal static IRawElementProviderSimple UiaHostProviderFromHwnd(IntPtr hwnd) { IRawElementProviderSimple provider; CheckError(RawUiaHostProviderFromHwnd(hwnd, out provider)); return provider; } #endregion Provider methods // // Event methods (client and provider) // #region Event methods ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: Causes an AutomationEvent to fire, requires a functional IRawElementProvider, so cannot even be used to spoof events from other AutomationElements. /// [SecurityCritical,SecurityTreatAsSafe] internal static void UiaRaiseAutomationPropertyChangedEvent(IRawElementProviderSimple provider, int propertyId, object oldValue, object newValue) { CheckError(RawUiaRaiseAutomationPropertyChangedEvent(provider, propertyId, oldValue, newValue)); } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: Causes an AutomationEvent to fire, requires a functional IRawElementProvider, so cannot even be used to spoof events from other AutomationElements. /// [SecurityCritical,SecurityTreatAsSafe] internal static void UiaRaiseAutomationEvent(IRawElementProviderSimple provider, int eventId) { CheckError(RawUiaRaiseAutomationEvent(provider, eventId)); } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: Causes an AutomationEvent to fire, requires a functional IRawElementProvider, so cannot even be used to spoof events from other AutomationElements. /// [SecurityCritical,SecurityTreatAsSafe] internal static void UiaRaiseStructureChangedEvent(IRawElementProviderSimple provider, StructureChangeType structureChangeType, int[] runtimeId) { CheckError(RawUiaRaiseStructureChangedEvent(provider, structureChangeType, runtimeId, runtimeId == null ? 0 : runtimeId.Length)); } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: Causes an AutomationEvent to fire, requires a functional IRawElementProvider, so cannot even be used to spoof events from other AutomationElements. /// [SecurityCritical,SecurityTreatAsSafe] internal static void UiaRaiseAsyncContentLoadedEvent(IRawElementProviderSimple provider, AsyncContentLoadedState asyncContentLoadedState, double PercentComplete) { CheckError(RawUiaRaiseAsyncContentLoadedEvent(provider, asyncContentLoadedState, PercentComplete)); } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: Simply checks whether clients are listening in order to know whether to fire AutomationEvents. This is information we WANT available to /// Partial Trust users, so is not an information disclosure risk. /// [SecurityCritical,SecurityTreatAsSafe] internal static bool UiaClientsAreListening() { return RawUiaClientsAreListening(); } #endregion Event methods #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods // Check hresult for error... private static void CheckError(int hr) { if (hr >= 0) { return; } Marshal.ThrowExceptionForHR(hr); } #endregion Private Methods #region Raw API methods // // Provider-side methods... // [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaReturnRawElementProvider", CharSet = CharSet.Unicode)] private static extern IntPtr RawUiaReturnRawElementProvider(IntPtr hwnd, IntPtr wParam, IntPtr lParam, IRawElementProviderSimple el); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaHostProviderFromHwnd", CharSet = CharSet.Unicode)] private static extern int RawUiaHostProviderFromHwnd(IntPtr hwnd, [MarshalAs(UnmanagedType.Interface)] out IRawElementProviderSimple provider); // Event APIs... [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaRaiseAutomationPropertyChangedEvent", CharSet = CharSet.Unicode)] private static extern int RawUiaRaiseAutomationPropertyChangedEvent(IRawElementProviderSimple provider, int id, object oldValue, object newValue); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaRaiseAutomationEvent", CharSet = CharSet.Unicode)] private static extern int RawUiaRaiseAutomationEvent(IRawElementProviderSimple provider, int id); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaRaiseStructureChangedEvent", CharSet = CharSet.Unicode)] private static extern int RawUiaRaiseStructureChangedEvent(IRawElementProviderSimple provider, StructureChangeType structureChangeType, int[] runtimeId, int runtimeIdLen); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaRaiseAsyncContentLoadedEvent", CharSet = CharSet.Unicode)] private static extern int RawUiaRaiseAsyncContentLoadedEvent(IRawElementProviderSimple provider, AsyncContentLoadedState asyncContentLoadedState, double PercentComplete); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaClientsAreListening", CharSet = CharSet.Unicode)] private static extern bool RawUiaClientsAreListening(); #endregion Raw API methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Imports from unmanaged UiaCore DLL // // History: // 06/02/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Security; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Runtime.InteropServices; using Microsoft.Internal; namespace MS.Internal.Automation { internal static class UiaCoreProviderApi { //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods // // Provider-side methods... // #region Provider methods ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: This method is used to return an IRawElementProviderSimple associated with an HWND to UIAutomation in response to a WM_GETOBJECT /// The returned value is simply an LRESULT, so is harmless, and the input values are verfied on the unmanaged side, so it is not abusable. /// [SecurityCritical,SecurityTreatAsSafe] internal static IntPtr UiaReturnRawElementProvider(IntPtr hwnd, IntPtr wParam, IntPtr lParam, IRawElementProviderSimple el) { return RawUiaReturnRawElementProvider( hwnd, wParam, lParam, el ); } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: This converts an hwnd to a MiniHwndProxy, which while technically implementing IRawElementProviderSimple, has none of the functionality /// and is therefore simply a harmless hwnd container. /// [SecurityCritical,SecurityTreatAsSafe] internal static IRawElementProviderSimple UiaHostProviderFromHwnd(IntPtr hwnd) { IRawElementProviderSimple provider; CheckError(RawUiaHostProviderFromHwnd(hwnd, out provider)); return provider; } #endregion Provider methods // // Event methods (client and provider) // #region Event methods ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: Causes an AutomationEvent to fire, requires a functional IRawElementProvider, so cannot even be used to spoof events from other AutomationElements. /// [SecurityCritical,SecurityTreatAsSafe] internal static void UiaRaiseAutomationPropertyChangedEvent(IRawElementProviderSimple provider, int propertyId, object oldValue, object newValue) { CheckError(RawUiaRaiseAutomationPropertyChangedEvent(provider, propertyId, oldValue, newValue)); } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: Causes an AutomationEvent to fire, requires a functional IRawElementProvider, so cannot even be used to spoof events from other AutomationElements. /// [SecurityCritical,SecurityTreatAsSafe] internal static void UiaRaiseAutomationEvent(IRawElementProviderSimple provider, int eventId) { CheckError(RawUiaRaiseAutomationEvent(provider, eventId)); } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: Causes an AutomationEvent to fire, requires a functional IRawElementProvider, so cannot even be used to spoof events from other AutomationElements. /// [SecurityCritical,SecurityTreatAsSafe] internal static void UiaRaiseStructureChangedEvent(IRawElementProviderSimple provider, StructureChangeType structureChangeType, int[] runtimeId) { CheckError(RawUiaRaiseStructureChangedEvent(provider, structureChangeType, runtimeId, runtimeId == null ? 0 : runtimeId.Length)); } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: Causes an AutomationEvent to fire, requires a functional IRawElementProvider, so cannot even be used to spoof events from other AutomationElements. /// [SecurityCritical,SecurityTreatAsSafe] internal static void UiaRaiseAsyncContentLoadedEvent(IRawElementProviderSimple provider, AsyncContentLoadedState asyncContentLoadedState, double PercentComplete) { CheckError(RawUiaRaiseAsyncContentLoadedEvent(provider, asyncContentLoadedState, PercentComplete)); } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: Simply checks whether clients are listening in order to know whether to fire AutomationEvents. This is information we WANT available to /// Partial Trust users, so is not an information disclosure risk. /// [SecurityCritical,SecurityTreatAsSafe] internal static bool UiaClientsAreListening() { return RawUiaClientsAreListening(); } #endregion Event methods #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods // Check hresult for error... private static void CheckError(int hr) { if (hr >= 0) { return; } Marshal.ThrowExceptionForHR(hr); } #endregion Private Methods #region Raw API methods // // Provider-side methods... // [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaReturnRawElementProvider", CharSet = CharSet.Unicode)] private static extern IntPtr RawUiaReturnRawElementProvider(IntPtr hwnd, IntPtr wParam, IntPtr lParam, IRawElementProviderSimple el); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaHostProviderFromHwnd", CharSet = CharSet.Unicode)] private static extern int RawUiaHostProviderFromHwnd(IntPtr hwnd, [MarshalAs(UnmanagedType.Interface)] out IRawElementProviderSimple provider); // Event APIs... [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaRaiseAutomationPropertyChangedEvent", CharSet = CharSet.Unicode)] private static extern int RawUiaRaiseAutomationPropertyChangedEvent(IRawElementProviderSimple provider, int id, object oldValue, object newValue); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaRaiseAutomationEvent", CharSet = CharSet.Unicode)] private static extern int RawUiaRaiseAutomationEvent(IRawElementProviderSimple provider, int id); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaRaiseStructureChangedEvent", CharSet = CharSet.Unicode)] private static extern int RawUiaRaiseStructureChangedEvent(IRawElementProviderSimple provider, StructureChangeType structureChangeType, int[] runtimeId, int runtimeIdLen); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaRaiseAsyncContentLoadedEvent", CharSet = CharSet.Unicode)] private static extern int RawUiaRaiseAsyncContentLoadedEvent(IRawElementProviderSimple provider, AsyncContentLoadedState asyncContentLoadedState, double PercentComplete); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaClientsAreListening", CharSet = CharSet.Unicode)] private static extern bool RawUiaClientsAreListening(); #endregion Raw API methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
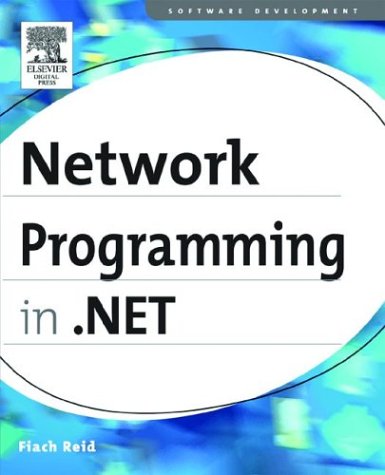
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GenericNameHandler.cs
- UpdatePanelTriggerCollection.cs
- IPipelineRuntime.cs
- ProcessHostMapPath.cs
- DataViewSettingCollection.cs
- EmbeddedMailObject.cs
- NotCondition.cs
- ColumnHeader.cs
- SQLString.cs
- DelegateTypeInfo.cs
- TextBox.cs
- x509store.cs
- XmlSecureResolver.cs
- SystemException.cs
- SqlServer2KCompatibilityAnnotation.cs
- ZipIOLocalFileDataDescriptor.cs
- PageStatePersister.cs
- UserMapPath.cs
- BamlLocalizableResource.cs
- SharedPersonalizationStateInfo.cs
- ContextDataSource.cs
- unsafeIndexingFilterStream.cs
- Keywords.cs
- InternalConfigSettingsFactory.cs
- InputBindingCollection.cs
- AssemblyCollection.cs
- HotSpot.cs
- MatrixAnimationUsingKeyFrames.cs
- ObjRef.cs
- PathStreamGeometryContext.cs
- DataGridColumn.cs
- TranslateTransform3D.cs
- WebUtil.cs
- EdmItemCollection.OcAssemblyCache.cs
- BulletedList.cs
- UnsafeNativeMethodsTablet.cs
- EncryptedKey.cs
- SafePEFileHandle.cs
- LoadedEvent.cs
- UriSection.cs
- PropertySegmentSerializer.cs
- SystemInfo.cs
- SupportingTokenSpecification.cs
- RegistryPermission.cs
- LogicalCallContext.cs
- PeerUnsafeNativeMethods.cs
- XmlAttributeAttribute.cs
- Registry.cs
- itemelement.cs
- AnimationException.cs
- SimpleType.cs
- DiagnosticsConfigurationHandler.cs
- ButtonPopupAdapter.cs
- datacache.cs
- WindowsStatic.cs
- Executor.cs
- WaveHeader.cs
- FormatConvertedBitmap.cs
- Int32RectConverter.cs
- MatrixConverter.cs
- LookupNode.cs
- MarginsConverter.cs
- OracleCommandBuilder.cs
- DbConnectionClosed.cs
- HtmlString.cs
- CultureInfoConverter.cs
- DataSourceControl.cs
- WinInetCache.cs
- WCFBuildProvider.cs
- SemaphoreFullException.cs
- UndoEngine.cs
- SequentialActivityDesigner.cs
- WindowsListViewGroup.cs
- Operators.cs
- SaveFileDialogDesigner.cs
- UnsafePeerToPeerMethods.cs
- MenuRenderer.cs
- Membership.cs
- BitmapEffectCollection.cs
- DataControlCommands.cs
- RadialGradientBrush.cs
- NavigationPropertyEmitter.cs
- SQLDecimal.cs
- SystemIPGlobalStatistics.cs
- XmlMessageFormatter.cs
- _SslState.cs
- StructuralComparisons.cs
- ComMethodElement.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- ChtmlTextBoxAdapter.cs
- TypedTableGenerator.cs
- ChangeInterceptorAttribute.cs
- Run.cs
- PkcsMisc.cs
- MarshalByValueComponent.cs
- OpenTypeCommon.cs
- RouteItem.cs
- CreateUserWizardStep.cs
- TimeSpan.cs
- EdmItemError.cs