Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Recognition / SrgsGrammar / SrgsRule.cs / 1 / SrgsRule.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 [....] Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Xml; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Speech.Internal; using System.Speech.Internal.SrgsParser; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Recognition.SrgsGrammar { /// TODOC <_include file='doc\Rule.uex' path='docs/doc[@for="Rule"]/*' /> [Serializable] [DebuggerDisplay ("Rule={_id.ToString()} Scope={_scope.ToString()}")] [DebuggerTypeProxy (typeof (SrgsRuleDebugDisplay))] public class SrgsRule : IRule { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors /// TODOC <_include file='doc\Rule.uex' path='docs/doc[@for="Rule.Rule1"]/*' /> private SrgsRule () { _elements = new SrgsElementList (); } /// TODOC <_include file='doc\Rule.uex' path='docs/doc[@for="Rule.Rule2"]/*' /> public SrgsRule (string id) : this () { XmlParser.ValidateRuleId (id); Id = id; } /// TODOC <_include file='doc\Rule.uex' path='docs/doc[@for="Rule.Rule2"]/*' /> public SrgsRule (string id, params SrgsElement [] elements) : this () { Helpers.ThrowIfNull (elements, "elements"); XmlParser.ValidateRuleId (id); Id = id; for (int iElement = 0; iElement < elements.Length; iElement++) { if (elements [iElement] == null) { throw new ArgumentNullException ("elements", SR.Get (SRID.ParamsEntryNullIllegal)); } ((Collection) _elements).Add (elements [iElement]); } } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region public Method /// /// TODOC /// /// public void Add (SrgsElement element) { Helpers.ThrowIfNull (element, "element"); Elements.Add (element); } #endregion //******************************************************************** // // Public Properties // //******************************************************************** #region public Properties /// TODOC <_include file='doc\Rule.uex' path='docs/doc[@for="Rule.Elements"]/*' /> public CollectionElements { get { return _elements; } } /// TODOC <_include file='doc\Rule.uex' path='docs/doc[@for="Rule.Id"]/*' /> public string Id { get { return _id; } set { XmlParser.ValidateRuleId (value); _id = value; } } /// TODOC <_include file='doc\Rule.uex' path='docs/doc[@for="Rule.Scope"]/*' /> public SrgsRuleScope Scope { get { return _scope; } set { _scope = value; _isScopeSet = true; } } #if !NO_STG /// |summary| /// classname /// |/summary| public string BaseClass { set { // base value can be null #pragma warning disable 56526 _baseclass = value; #pragma warning restore 56526 } get { return _baseclass; } } /// |summary| /// OnInit /// |/summary| public string Script { set { Helpers.ThrowIfEmptyOrNull (value, "value"); _script = value; } get { return _script; } } /// |summary| /// OnInit /// |/summary| public string OnInit { set { ValidateIdentifier (value); _onInit = value; } get { return _onInit; } } /// |summary| /// OnParse /// |/summary| public string OnParse { set { ValidateIdentifier (value); _onParse = value; } get { return _onParse; } } /// |summary| /// OnError /// |/summary| public string OnError { set { ValidateIdentifier (value); _onError = value; } get { return _onError; } } /// |summary| /// OnRecognition /// |/summary| public string OnRecognition { set { ValidateIdentifier (value); _onRecognition = value; } get { return _onRecognition; } } #endif #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods internal void WriteSrgs (XmlWriter writer) { // Empty rule are not allowed if (Elements.Count == 0) { XmlParser.ThrowSrgsException (SRID.InvalidEmptyRule, "rule", _id); } // Write writer.WriteStartElement ("rule"); writer.WriteAttributeString ("id", _id); if (_isScopeSet) { switch (_scope) { case SrgsRuleScope.Private: writer.WriteAttributeString ("scope", "private"); break; case SrgsRuleScope.Public: writer.WriteAttributeString ("scope", "public"); break; } } #if !NO_STG // Write the 'baseclass' attribute if (_baseclass != null) { writer.WriteAttributeString ("sapi", "baseclass", XmlParser.sapiNamespace, _baseclass); } #endif // Write if (_dynamic != RuleDynamic.NotSet) { writer.WriteAttributeString ("sapi", "dynamic", XmlParser.sapiNamespace, _dynamic == RuleDynamic.True ? "true" : "false"); } #if !NO_STG // Write the 'onInit' code snippet if (OnInit != null) { writer.WriteAttributeString ("sapi", "onInit", XmlParser.sapiNamespace, OnInit); } // Write if (OnParse != null) { writer.WriteAttributeString ("sapi", "onParse", XmlParser.sapiNamespace, OnParse); } // Write if (OnError != null) { writer.WriteAttributeString ("sapi", "onError", XmlParser.sapiNamespace, OnError); } // Write if (OnRecognition != null) { writer.WriteAttributeString ("sapi", "onRecognition", XmlParser.sapiNamespace, OnRecognition); } #endif // Write body and footer. Type previousElementType = null; foreach (SrgsElement element in _elements) { // Insert space between consecutive SrgsText elements. Type elementType = element.GetType (); if ((elementType == typeof (SrgsText)) && (elementType == previousElementType)) { writer.WriteString (" "); } previousElementType = elementType; element.WriteSrgs (writer); } writer.WriteEndElement (); #if !NO_STG // Write the
Link Menu
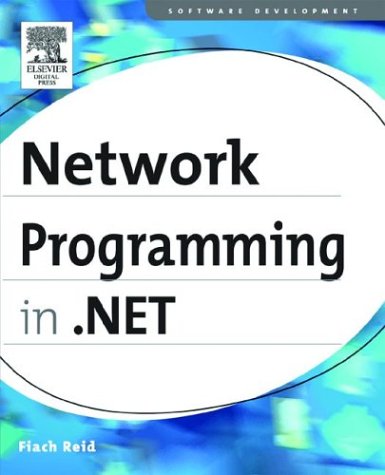
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GrammarBuilder.cs
- PeerNameRegistration.cs
- XamlToRtfParser.cs
- AppliedDeviceFiltersEditor.cs
- WebBrowserHelper.cs
- WebServiceReceiveDesigner.cs
- MimeWriter.cs
- DesignerForm.cs
- SpanIndex.cs
- CharacterHit.cs
- SqlGenerator.cs
- PreviewPrintController.cs
- cookieexception.cs
- ByteStream.cs
- Frame.cs
- AtomicFile.cs
- TraceUtils.cs
- XmlDomTextWriter.cs
- OleDbParameterCollection.cs
- ClosableStream.cs
- ListComponentEditorPage.cs
- ResourceDictionary.cs
- WebBaseEventKeyComparer.cs
- WebPartsSection.cs
- ProfileSettingsCollection.cs
- PropertyEmitter.cs
- WCFServiceClientProxyGenerator.cs
- SourceElementsCollection.cs
- XpsPartBase.cs
- PropertyRef.cs
- ThumbButtonInfo.cs
- StyleCollectionEditor.cs
- ComboBox.cs
- ExpandCollapseProviderWrapper.cs
- BrowserDefinition.cs
- ParameterToken.cs
- EntityDataSourceChangingEventArgs.cs
- HttpHeaderCollection.cs
- XmlNavigatorFilter.cs
- MimeParameter.cs
- PersonalizationAdministration.cs
- ThreadPool.cs
- ColumnTypeConverter.cs
- TdsEnums.cs
- WorkerRequest.cs
- ListDataHelper.cs
- ProviderMetadata.cs
- DataProtection.cs
- RepeaterItemCollection.cs
- Compress.cs
- DesignerSerializationVisibilityAttribute.cs
- DataRowView.cs
- SqlColumnizer.cs
- RuleSettingsCollection.cs
- CacheSection.cs
- NonBatchDirectoryCompiler.cs
- IssuedSecurityTokenParameters.cs
- OleDbSchemaGuid.cs
- DesignerDataSchemaClass.cs
- DataGridViewHeaderCell.cs
- ReadOnlyHierarchicalDataSourceView.cs
- UdpDiscoveryEndpoint.cs
- IssuedTokenClientBehaviorsElement.cs
- ExceptionUtil.cs
- Deserializer.cs
- DataGridViewCell.cs
- AutomationEvent.cs
- TaskFactory.cs
- ValueSerializer.cs
- ClientType.cs
- AbstractSvcMapFileLoader.cs
- XamlTreeBuilderBamlRecordWriter.cs
- DecoderReplacementFallback.cs
- _RequestCacheProtocol.cs
- NavigationProperty.cs
- SerializationSectionGroup.cs
- InternalBufferManager.cs
- DurableDispatcherAddressingFault.cs
- ReferencedType.cs
- EventLogger.cs
- PageSettings.cs
- TextContainerChangedEventArgs.cs
- ListDictionaryInternal.cs
- HelpEvent.cs
- AttributeUsageAttribute.cs
- SettingsProperty.cs
- InputReportEventArgs.cs
- SelectionChangedEventArgs.cs
- MaterialGroup.cs
- XmlSortKey.cs
- TextDecoration.cs
- AdapterDictionary.cs
- DataGridHyperlinkColumn.cs
- mda.cs
- ScriptDescriptor.cs
- SqlStatistics.cs
- ADRole.cs
- GreenMethods.cs
- ListControl.cs
- Accessors.cs