Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Statements / Confirm.cs / 1305376 / Confirm.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Statements { using System; using System.Collections.Generic; using System.ComponentModel; using System.Runtime; using System.Activities.Validation; using System.Collections.ObjectModel; using System.Linq; using System.Activities.Expressions; public sealed class Confirm : NativeActivity { static Constraint confirmWithNoTarget = Confirm.ConfirmWithNoTarget(); InternalConfirm internalConfirm; DefaultConfirmation defaultConfirmation; VariablecurrentCompensationToken; public Confirm() : base() { this.currentCompensationToken = new Variable (); } public InArgument Target { get; set; } DefaultConfirmation DefaultConfirmation { get { if (this.defaultConfirmation == null) { this.defaultConfirmation = new DefaultConfirmation() { Target = new InArgument (this.currentCompensationToken), }; } return this.defaultConfirmation; } } InternalConfirm InternalConfirm { get { if (this.internalConfirm == null) { this.internalConfirm = new InternalConfirm() { Target = new InArgument (new ArgumentValue { ArgumentName = "Target" }), }; } return this.internalConfirm; } } protected override void CacheMetadata(NativeActivityMetadata metadata) { RuntimeArgument targetArgument = new RuntimeArgument("Target", typeof(CompensationToken), ArgumentDirection.In); metadata.Bind(this.Target, targetArgument); metadata.SetArgumentsCollection( new Collection { targetArgument }); metadata.SetImplementationVariablesCollection( new Collection { this.currentCompensationToken }); Fx.Assert(DefaultConfirmation != null, "DefaultConfirmation must be valid"); Fx.Assert(InternalConfirm != null, "InternalConfirm must be valid"); metadata.SetImplementationChildrenCollection( new Collection { DefaultConfirmation, InternalConfirm }); } internal override IList InternalGetConstraints() { return new List (1) { confirmWithNoTarget }; } static Constraint ConfirmWithNoTarget() { DelegateInArgument element = new DelegateInArgument { Name = "element" }; DelegateInArgument validationContext = new DelegateInArgument { Name = "validationContext" }; Variable assertFlag = new Variable { Name = "assertFlag" }; Variable > elements = new Variable >() { Name = "elements" }; Variable index = new Variable () { Name = "index" }; return new Constraint { Body = new ActivityAction { Argument1 = element, Argument2 = validationContext, Handler = new Sequence { Variables = { assertFlag, elements, index }, Activities = { new If { Condition = new InArgument ((env)=>element.Get(env).Target != null), Then = new Assign { To = assertFlag, Value = true }, Else = new Sequence { Activities = { new Assign > { To = elements, Value = new GetParentChain { ValidationContext = validationContext, }, }, new While(env => (assertFlag.Get(env)!=true) && index.Get(env) < elements.Get(env).Count()) { Body = new Sequence { Activities = { new If(env => (elements.Get(env).ElementAt(index.Get(env))).GetType() == typeof(CompensationParticipant)) { Then = new Assign { To = assertFlag, Value = true }, }, new Assign { To=index, Value=new InArgument (env => index.Get(env) + 1) }, } } } } } }, new AssertValidation { Assertion = new InArgument (assertFlag), Message = new InArgument (SR.ConfirmWithNoTargetConstraint) } } } } }; } protected override void Execute(NativeActivityContext context) { CompensationExtension compensationExtension = context.GetExtension (); if (compensationExtension == null) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.ConfirmWithoutCompensableActivity(this.DisplayName))); } if (Target.IsEmpty) { CompensationToken ambientCompensationToken = (CompensationToken)context.Properties.Find(CompensationToken.PropertyName); CompensationTokenData ambientTokenData = ambientCompensationToken == null ? null : compensationExtension.Get(ambientCompensationToken.CompensationId); if (ambientTokenData != null && ambientTokenData.IsTokenValidInSecondaryRoot) { this.currentCompensationToken.Set(context, ambientCompensationToken); if (ambientTokenData.ExecutionTracker.Count > 0) { context.ScheduleActivity(DefaultConfirmation); } } else { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.InvalidConfirmActivityUsage(this.DisplayName))); } } else { CompensationToken compensationToken = Target.Get(context); CompensationTokenData tokenData = compensationToken == null ? null : compensationExtension.Get(compensationToken.CompensationId); if (compensationToken == null) { throw FxTrace.Exception.Argument("Target", SR.InvalidCompensationToken(this.DisplayName)); } if (compensationToken.ConfirmCalled) { // No-Op return; } if (tokenData == null || tokenData.CompensationState != CompensationState.Completed) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.CompensableActivityAlreadyConfirmedOrCompensated)); } // A valid in-arg was passed... tokenData.CompensationState = CompensationState.Confirming; compensationToken.ConfirmCalled = true; context.ScheduleActivity(InternalConfirm); } } protected override void Cancel(NativeActivityContext context) { // Suppress Cancel } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
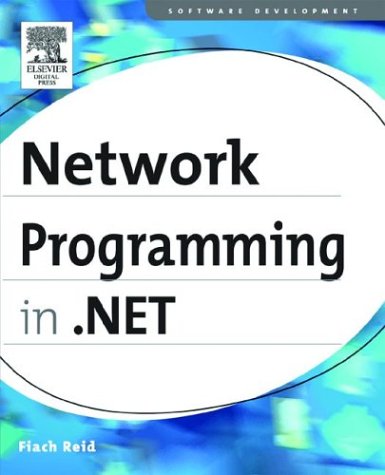
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaExporter.cs
- Internal.cs
- ObjectCloneHelper.cs
- DispatcherObject.cs
- SqlServices.cs
- AuthorizationSection.cs
- QilXmlWriter.cs
- CellLabel.cs
- ClassHandlersStore.cs
- ThousandthOfEmRealDoubles.cs
- PrincipalPermission.cs
- FormatException.cs
- VirtualDirectoryMappingCollection.cs
- StickyNote.cs
- ConfigXmlText.cs
- SecurityTokenProvider.cs
- KnownIds.cs
- SecuritySessionClientSettings.cs
- EventItfInfo.cs
- LoginCancelEventArgs.cs
- TypeToTreeConverter.cs
- DiagnosticsConfiguration.cs
- Margins.cs
- ProviderSettingsCollection.cs
- TextFormatterContext.cs
- XamlClipboardData.cs
- GiveFeedbackEvent.cs
- AppSecurityManager.cs
- StreamUpdate.cs
- SpotLight.cs
- GeneratedView.cs
- OpenTypeCommon.cs
- PaginationProgressEventArgs.cs
- NoClickablePointException.cs
- EventLogPermissionEntry.cs
- EditorPartChrome.cs
- PointCollection.cs
- DeploymentSection.cs
- Visual.cs
- NonSerializedAttribute.cs
- HttpListenerRequestTraceRecord.cs
- InvalidEnumArgumentException.cs
- CqlQuery.cs
- XmlElementAttribute.cs
- ExpressionBuilderContext.cs
- SqlDataSourceCustomCommandEditor.cs
- Size.cs
- SqlFactory.cs
- XmlUtil.cs
- CachedFontFace.cs
- BinaryObjectReader.cs
- HttpStreams.cs
- ProgressBarBrushConverter.cs
- StringValidatorAttribute.cs
- BufferedReceiveElement.cs
- CommandBinding.cs
- NumericPagerField.cs
- ProgressBar.cs
- ToolStripItemImageRenderEventArgs.cs
- ReadOnlyDictionary.cs
- ResourceDisplayNameAttribute.cs
- StylusEventArgs.cs
- XamlTreeBuilderBamlRecordWriter.cs
- RectKeyFrameCollection.cs
- TimeSpanConverter.cs
- TracePayload.cs
- CodeAttributeDeclaration.cs
- ServiceProviders.cs
- TreeNodeMouseHoverEvent.cs
- SwitchCase.cs
- FormsAuthenticationTicket.cs
- EntityClassGenerator.cs
- StoryFragments.cs
- DocumentPageHost.cs
- PropertyDescriptorGridEntry.cs
- FormsAuthenticationUser.cs
- StrokeNode.cs
- EventLevel.cs
- ZipIOExtraFieldZip64Element.cs
- ErrorProvider.cs
- XhtmlBasicObjectListAdapter.cs
- WebBrowsableAttribute.cs
- FontStretchConverter.cs
- RangeValuePattern.cs
- DataGridViewColumnCollectionDialog.cs
- NotSupportedException.cs
- BaseUriHelper.cs
- SqlColumnizer.cs
- TimelineClockCollection.cs
- ImageAttributes.cs
- IndexedGlyphRun.cs
- TransactionScope.cs
- WebResponse.cs
- HttpProfileBase.cs
- SchemaReference.cs
- BindUriHelper.cs
- MILUtilities.cs
- HtmlTableRowCollection.cs
- StyleXamlTreeBuilder.cs
- SettingsSavedEventArgs.cs