Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Diagnostics / Eventing / Reader / EventLevel.cs / 1305376 / EventLevel.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: EventLevel ** ** Purpose: ** This public class describes the metadata for a specific Level ** defined by a Provider. An instance of this class is obtained from ** a ProviderMetadata object. ** ============================================================*/ using System.Collections.Generic; namespace System.Diagnostics.Eventing.Reader { ////// Describes the metadata for a specific Level defined by a Provider. /// An instance of this class is obtained from a ProviderMetadata object. /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class EventLevel { private int value; private string name; private string displayName; private bool dataReady; ProviderMetadata pmReference; object syncObject; //called from EventMetadata internal EventLevel(int value, ProviderMetadata pmReference) { this.value = value; this.pmReference = pmReference; this.syncObject = new object(); } //called from ProviderMetadata internal EventLevel(string name, int value, string displayName) { this.value = value; this.name = name; this.displayName = displayName; this.dataReady = true; this.syncObject = new object(); } internal void PrepareData() { if (dataReady == true) return; lock (syncObject) { if (dataReady == true) return; IEnumerableresult = pmReference.Levels; this.name = null; this.displayName = null; this.dataReady = true; foreach (EventLevel lev in result) { if (lev.Value == this.value) { this.name = lev.Name; this.displayName = lev.DisplayName; break; } } } } public string Name { get { PrepareData(); return this.name; } } public int Value { get { return this.value; } } public string DisplayName { get { PrepareData(); return this.displayName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
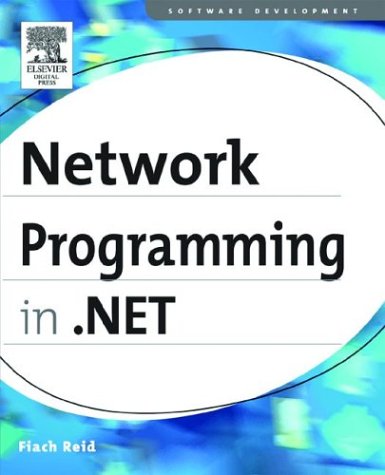
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Parameter.cs
- PartialCachingAttribute.cs
- FlowDocumentPage.cs
- CryptoKeySecurity.cs
- InputBuffer.cs
- ListViewItemMouseHoverEvent.cs
- Cursors.cs
- SubclassTypeValidatorAttribute.cs
- XmlJsonWriter.cs
- RegexCaptureCollection.cs
- DataGridViewSelectedRowCollection.cs
- ReadOnlyCollectionBase.cs
- LinqExpressionNormalizer.cs
- SqlCacheDependencyDatabase.cs
- CodeRegionDirective.cs
- BamlBinaryWriter.cs
- ObjectDisposedException.cs
- ObjectFullSpanRewriter.cs
- CustomError.cs
- DocumentViewerConstants.cs
- CodeGenHelper.cs
- ProgressBarRenderer.cs
- SafeNativeMethods.cs
- Helpers.cs
- CorrelationValidator.cs
- AssemblyName.cs
- QilSortKey.cs
- Composition.cs
- DataExpression.cs
- DataBinding.cs
- SafePEFileHandle.cs
- IIS7UserPrincipal.cs
- Path.cs
- SmiContext.cs
- TreeViewItemAutomationPeer.cs
- DataSvcMapFileSerializer.cs
- _SslStream.cs
- SqlProviderUtilities.cs
- TreeWalker.cs
- JsonServiceDocumentSerializer.cs
- SByte.cs
- AddInToken.cs
- XmlNodeChangedEventManager.cs
- OutputCacheSection.cs
- QilStrConcatenator.cs
- Stroke.cs
- SupportsEventValidationAttribute.cs
- CollectionViewSource.cs
- DotAtomReader.cs
- TypeSource.cs
- TypeDependencyAttribute.cs
- XmlWriterSettings.cs
- DefaultObjectMappingItemCollection.cs
- RtfToken.cs
- ScaleTransform.cs
- Hex.cs
- Label.cs
- webclient.cs
- RightsManagementPermission.cs
- MSHTMLHostUtil.cs
- DeploymentSectionCache.cs
- URLAttribute.cs
- XamlPathDataSerializer.cs
- QilName.cs
- RuntimeConfigLKG.cs
- WhitespaceRuleLookup.cs
- SoapAttributeAttribute.cs
- OfTypeExpression.cs
- RegexCode.cs
- SubtreeProcessor.cs
- InvokeDelegate.cs
- SqlError.cs
- LinqDataSourceStatusEventArgs.cs
- CellNormalizer.cs
- COM2ExtendedUITypeEditor.cs
- ComponentDispatcher.cs
- ScriptReference.cs
- RenamedEventArgs.cs
- OdbcRowUpdatingEvent.cs
- CompositeScriptReference.cs
- EncryptedReference.cs
- ICollection.cs
- PrePostDescendentsWalker.cs
- TimeoutConverter.cs
- XmlCountingReader.cs
- WebScriptServiceHost.cs
- TreeNodeSelectionProcessor.cs
- SqlServices.cs
- WinInetCache.cs
- TextServicesPropertyRanges.cs
- WebBrowserDocumentCompletedEventHandler.cs
- SerializerProvider.cs
- WebResourceUtil.cs
- PartialCachingControl.cs
- Parameter.cs
- ExpressionDumper.cs
- PromptStyle.cs
- XmlExpressionDumper.cs
- GroupBox.cs
- WebHttpBindingElement.cs