Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / System / data / design / SimpleNameService.cs / 1 / SimpleNameService.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Data.Design { using System; using System.Diagnostics; using System.Collections; using System.Globalization; using System.Text.RegularExpressions; using System.Design; internal class SimpleNamedObject:INamedObject{ object _obj; public SimpleNamedObject(object obj){ _obj = obj; } public string Name { get{ if (_obj is INamedObject){ return (_obj as INamedObject).Name; } else if (_obj is string){ return _obj as string; } else { return _obj.ToString(); } } set { if (_obj is INamedObject){ (_obj as INamedObject).Name = value; } else if (_obj is string){ _obj = value; } else { // do nothing } } } } internal class SimpleNamedObjectCollection:ArrayList, INamedObjectCollection{ private static SimpleNameService myNameService; protected virtual INameService NameService { get { if( SimpleNamedObjectCollection.myNameService == null ) { SimpleNamedObjectCollection.myNameService = new SimpleNameService(); } return SimpleNamedObjectCollection.myNameService; } } public INameService GetNameService() { return NameService; } } ////// SimpleNameService is intended to have some basic naming rule as following /// 1. Use the identifier regular expression /// 2. Name is case insensitive /// 3. Limit length to 1024 /// 4. Undone: Use CodeGen's NameHandler Bug 45100 /// internal class SimpleNameService:INameService { internal const int DEFAULT_MAX_TRIALS = 100000; private const int MAX_LENGTH = 1024; private int maxNumberOfTrials = DEFAULT_MAX_TRIALS; private static readonly string regexAlphaCharacter = @"[\p{L}\p{Nl}]"; private static readonly string regexUnderscoreCharacter = @"\p{Pc}"; private static readonly string regexIdentifierCharacter = @"[\p{L}\p{Nl}\p{Nd}\p{Mn}\p{Mc}\p{Cf}]"; private static readonly string regexIdentifierStart = "(" + regexAlphaCharacter + "|(" + regexUnderscoreCharacter + regexIdentifierCharacter + "))"; private static readonly string regexIdentifier = regexIdentifierStart + regexIdentifierCharacter + "*"; private static SimpleNameService defaultInstance; private bool caseSensitive = true; internal SimpleNameService() { } internal static SimpleNameService DefaultInstance { get { if (defaultInstance == null) { defaultInstance = new SimpleNameService(); } return defaultInstance; } } ////// return proposed if it is not exist /// /// /// ///public string CreateUniqueName(INamedObjectCollection container, string proposed){ if( !NameExist(container, proposed) ) { this.ValidateName(proposed); return proposed; } return this.CreateUniqueName(container, proposed, 1); } /// /// Return typeName1 or typeName2, etc. /// /// /// ///public string CreateUniqueName(INamedObjectCollection container, Type type) { return this.CreateUniqueName(container, type.Name, 1); } /// /// try proposedNameRoot + startSuffix first then ... /// /// /// ///public string CreateUniqueName(INamedObjectCollection container, string proposedNameRoot, int startSuffix){ return CreateUniqueNameOnCollection(container, proposedNameRoot, startSuffix); } public string CreateUniqueNameOnCollection(ICollection container, string proposedNameRoot, int startSuffix) { int suffix = startSuffix; if (suffix < 0){ Debug.Fail("startSuffix should >= 0"); suffix = 0; } // It will throw if proposedNameRoot is bad! ValidateName(proposedNameRoot); string current = proposedNameRoot + suffix.ToString(System.Globalization.CultureInfo.CurrentCulture); while (NameExist(container, current)){ suffix++; if (suffix >= maxNumberOfTrials){ throw new InternalException(VSDExceptions.COMMON.SIMPLENAMESERVICE_NAMEOVERFLOW_MSG, VSDExceptions.COMMON.SIMPLENAMESERVICE_NAMEOVERFLOW_CODE,true); } current = proposedNameRoot + suffix.ToString(System.Globalization.CultureInfo.CurrentCulture); } ValidateName(current); return current; } /// /// Check to see if the name is exist in the container /// /// /// ///private bool NameExist(ICollection container, string nameTobeChecked){ return NameExist(container, null, nameTobeChecked); } /// /// Check to see if the name is exist in the container /// /// /// ///private bool NameExist(ICollection container, INamedObject objTobeChecked, string nameTobeChecked) { if (StringUtil.Empty(nameTobeChecked) && objTobeChecked != null) { nameTobeChecked = objTobeChecked.Name; } foreach (INamedObject obj in container) { if (obj != objTobeChecked && StringUtil.EqualValue(obj.Name, nameTobeChecked, !caseSensitive)){ return true; } } return false; } /// /// Should throw NameValidationException when invalid name passed. /// valid name syntx only /// /// public virtual void ValidateName(string name) { if (StringUtil.EmptyOrSpace(name)){ throw new NameValidationException(SR.GetString(SR.CM_NameNotEmptyExcption)); } if (name.Length > MAX_LENGTH){ throw new NameValidationException(SR.GetString(SR.CM_NameTooLongExcption)); } Match m = Regex.Match(name, regexIdentifier); if(!m.Success) { throw new NameValidationException(SR.GetString(SR.CM_NameInvalid, name)); } } ////// Should throw NameValidationException when invalid name passed. /// Valid syntax + dup /// This function is used when adding a new object to the collection /// /// public void ValidateUniqueName(INamedObjectCollection container, string proposedName){ ValidateUniqueName(container, null, proposedName); } // Check if the name is unique and valid // This function is useful when renaming an existing object. public void ValidateUniqueName(INamedObjectCollection container, INamedObject namedObject, string proposedName) { ValidateName(proposedName); if (NameExist(container, namedObject, proposedName)) { throw new NameValidationException(SR.GetString(SR.CM_NameExist, proposedName)); } } } ////// Used to validate the DataSet objects: tables, columns, etc. /// internal class DataSetNameService : SimpleNameService { private static DataSetNameService defaultInstance; internal static new DataSetNameService DefaultInstance { get { if (defaultInstance == null) { defaultInstance = new DataSetNameService(); } return defaultInstance; } } ////// Do nothing. Leave the name validation to DataSet /// /// public override void ValidateName(string name) { } } ////// Used to validate the sources /// internal class SourceNameService : SimpleNameService { private static SourceNameService defaultInstance; internal static new SourceNameService DefaultInstance { get { if (defaultInstance == null) { defaultInstance = new SourceNameService(); } return defaultInstance; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
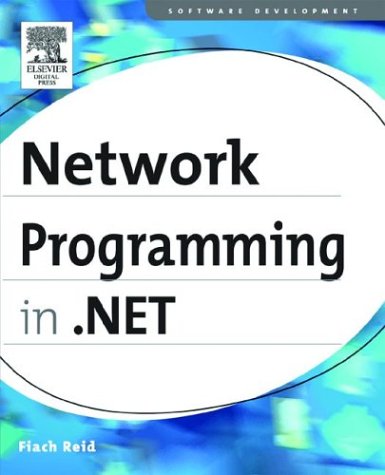
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EditorBrowsableAttribute.cs
- EntityContainerAssociationSetEnd.cs
- ClientSettingsStore.cs
- MenuScrollingVisibilityConverter.cs
- CqlIdentifiers.cs
- _HelperAsyncResults.cs
- DoubleAnimationClockResource.cs
- ObjectViewListener.cs
- HeaderCollection.cs
- VectorAnimationBase.cs
- BinaryOperationBinder.cs
- TextBoxAutoCompleteSourceConverter.cs
- TimeManager.cs
- WebPartManagerInternals.cs
- ContentDesigner.cs
- SQLConvert.cs
- DrawingContextWalker.cs
- SqlUserDefinedAggregateAttribute.cs
- SafeReadContext.cs
- FixedSOMTableCell.cs
- SortDescriptionCollection.cs
- WinInetCache.cs
- DesignerVerb.cs
- SqlWebEventProvider.cs
- GridViewPageEventArgs.cs
- MessageEventSubscriptionService.cs
- SynchronizedDispatch.cs
- ServiceParser.cs
- SoapIncludeAttribute.cs
- TiffBitmapDecoder.cs
- TextParentUndoUnit.cs
- AQNBuilder.cs
- XamlReader.cs
- TraceFilter.cs
- MustUnderstandSoapException.cs
- DoubleAnimation.cs
- DebugView.cs
- ReversePositionQuery.cs
- InternalBase.cs
- MachineKeySection.cs
- TextRangeEdit.cs
- AuthenticationConfig.cs
- DesignerProperties.cs
- SecUtil.cs
- TableNameAttribute.cs
- ScriptResourceInfo.cs
- PageBuildProvider.cs
- FileDetails.cs
- ApplicationSecurityManager.cs
- SoapProcessingBehavior.cs
- FlowPosition.cs
- RuntimeConfigLKG.cs
- LinearKeyFrames.cs
- ICollection.cs
- CurrencyWrapper.cs
- CharacterString.cs
- ConfigurationException.cs
- ListDictionaryInternal.cs
- Typography.cs
- RemoteHelper.cs
- SafeEventHandle.cs
- Operator.cs
- Int64Converter.cs
- PointCollectionConverter.cs
- Panel.cs
- WorkflowLayouts.cs
- HtmlElementEventArgs.cs
- SqlSelectStatement.cs
- PropertiesTab.cs
- XmlTextWriter.cs
- XmlSchemaType.cs
- GridProviderWrapper.cs
- DataViewSettingCollection.cs
- ExpressionEditorAttribute.cs
- DataGridViewTopRowAccessibleObject.cs
- RenderTargetBitmap.cs
- CodeDomDecompiler.cs
- DisplayNameAttribute.cs
- SRDisplayNameAttribute.cs
- TreeViewAutomationPeer.cs
- XmlSchemaCompilationSettings.cs
- DataRelationPropertyDescriptor.cs
- InputLanguage.cs
- SettingsContext.cs
- XmlChoiceIdentifierAttribute.cs
- CustomAttributeBuilder.cs
- filewebresponse.cs
- SuppressIldasmAttribute.cs
- XmlQueryStaticData.cs
- SelectionProcessor.cs
- CubicEase.cs
- SmtpAuthenticationManager.cs
- DateTimeConstantAttribute.cs
- HttpClientCertificate.cs
- DiscoveryClientBindingElement.cs
- HashAlgorithm.cs
- ModelUIElement3D.cs
- ToolStripDropDownItem.cs
- KeyFrames.cs
- PatternMatchRules.cs