Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / xsp / System / Web / Extensions / ui / ServiceReference.cs / 2 / ServiceReference.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Security.Permissions; using System.Web; using System.Web.Compilation; using System.Web.UI; using System.Web.Resources; using System.Web.Script.Services; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal), DefaultProperty("Path") ] public class ServiceReference { private string _path; private bool _inlineScript; public ServiceReference() { } public ServiceReference(string path) { Path = path; } [ ResourceDescription("ServiceReference_InlineScript"), DefaultValue(false), Category("Behavior") ] public bool InlineScript { get { return _inlineScript; } set { _inlineScript = value; } } [ ResourceDescription("ServiceReference_Path"), DefaultValue(""), Category("Behavior"), UrlProperty() ] public string Path { get { if (_path == null) { return String.Empty; } return _path; } set { _path = value; } } private string GetInlineScript(Control containingControl, HttpContext context, bool debug) { // Do not attempt to resolve inline service references on PageMethod requests. if (RestHandlerFactory.IsRestMethodCall(context.Request)) { return String.Empty; } string servicePath = GetServicePath(containingControl, false); try { servicePath = VirtualPathUtility.Combine(context.Request.FilePath, servicePath); } catch { throw new ArgumentException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.WebService_InvalidInlineVirtualPath, servicePath)); } return WebServiceClientProxyGenerator.GetInlineClientProxyScript(servicePath, context, debug); } private string GetServicePath(Control containingControl, bool encodeSpaces) { string path = Path; if (String.IsNullOrEmpty(path)) { throw new InvalidOperationException(AtlasWeb.ServiceReference_PathCannotBeEmpty); } if (encodeSpaces) { path = containingControl.ResolveClientUrl(path); } else { path = containingControl.ResolveUrl(path); } return path; } private string GetProxyPath(Control containingControl, bool debug) { if (debug) return GetServicePath(containingControl, true) + RestHandlerFactory.ClientDebugProxyRequestPathInfo; else return GetServicePath(containingControl, true) + RestHandlerFactory.ClientProxyRequestPathInfo; } internal void Register(Control containingControl, HttpContext context, ScriptManager scriptManager, bool debug) { if (InlineScript) { RenderClientScriptBlock(GetInlineScript(containingControl, context, debug), scriptManager); } else { RegisterClientScriptInclude(GetProxyPath(containingControl, debug), scriptManager); } } private static void RegisterClientScriptInclude(string path, ScriptManager scriptManager) { scriptManager.RegisterClientScriptIncludeInternal(scriptManager, typeof(ScriptManager), path, path); } private static void RenderClientScriptBlock(string script, ScriptManager scriptManager) { scriptManager.RegisterClientScriptBlockInternal(scriptManager, typeof(ScriptManager), script, script, true); } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public override string ToString() { if (!String.IsNullOrEmpty(Path)) { return Path; } else { return GetType().Name; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Security.Permissions; using System.Web; using System.Web.Compilation; using System.Web.UI; using System.Web.Resources; using System.Web.Script.Services; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal), DefaultProperty("Path") ] public class ServiceReference { private string _path; private bool _inlineScript; public ServiceReference() { } public ServiceReference(string path) { Path = path; } [ ResourceDescription("ServiceReference_InlineScript"), DefaultValue(false), Category("Behavior") ] public bool InlineScript { get { return _inlineScript; } set { _inlineScript = value; } } [ ResourceDescription("ServiceReference_Path"), DefaultValue(""), Category("Behavior"), UrlProperty() ] public string Path { get { if (_path == null) { return String.Empty; } return _path; } set { _path = value; } } private string GetInlineScript(Control containingControl, HttpContext context, bool debug) { // Do not attempt to resolve inline service references on PageMethod requests. if (RestHandlerFactory.IsRestMethodCall(context.Request)) { return String.Empty; } string servicePath = GetServicePath(containingControl, false); try { servicePath = VirtualPathUtility.Combine(context.Request.FilePath, servicePath); } catch { throw new ArgumentException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.WebService_InvalidInlineVirtualPath, servicePath)); } return WebServiceClientProxyGenerator.GetInlineClientProxyScript(servicePath, context, debug); } private string GetServicePath(Control containingControl, bool encodeSpaces) { string path = Path; if (String.IsNullOrEmpty(path)) { throw new InvalidOperationException(AtlasWeb.ServiceReference_PathCannotBeEmpty); } if (encodeSpaces) { path = containingControl.ResolveClientUrl(path); } else { path = containingControl.ResolveUrl(path); } return path; } private string GetProxyPath(Control containingControl, bool debug) { if (debug) return GetServicePath(containingControl, true) + RestHandlerFactory.ClientDebugProxyRequestPathInfo; else return GetServicePath(containingControl, true) + RestHandlerFactory.ClientProxyRequestPathInfo; } internal void Register(Control containingControl, HttpContext context, ScriptManager scriptManager, bool debug) { if (InlineScript) { RenderClientScriptBlock(GetInlineScript(containingControl, context, debug), scriptManager); } else { RegisterClientScriptInclude(GetProxyPath(containingControl, debug), scriptManager); } } private static void RegisterClientScriptInclude(string path, ScriptManager scriptManager) { scriptManager.RegisterClientScriptIncludeInternal(scriptManager, typeof(ScriptManager), path, path); } private static void RenderClientScriptBlock(string script, ScriptManager scriptManager) { scriptManager.RegisterClientScriptBlockInternal(scriptManager, typeof(ScriptManager), script, script, true); } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public override string ToString() { if (!String.IsNullOrEmpty(Path)) { return Path; } else { return GetType().Name; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
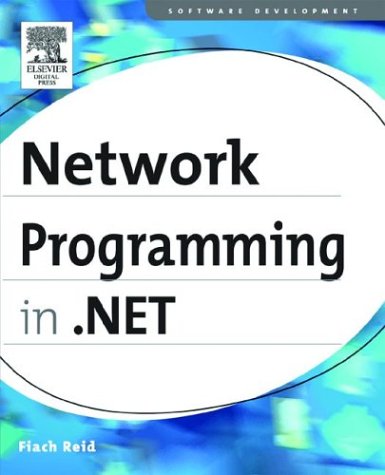
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlButton.cs
- TraceContextEventArgs.cs
- OperationInfoBase.cs
- TraceSwitch.cs
- DispatcherObject.cs
- XmlArrayAttribute.cs
- HostExecutionContextManager.cs
- XmlValueConverter.cs
- XmlComplianceUtil.cs
- QueryOperationResponseOfT.cs
- EngineSite.cs
- IndexerNameAttribute.cs
- NamespaceInfo.cs
- ContravarianceAdapter.cs
- WebPartZone.cs
- HtmlLink.cs
- LockedHandleGlyph.cs
- SettingsPropertyIsReadOnlyException.cs
- BCryptNative.cs
- DATA_BLOB.cs
- TrackingCondition.cs
- MutableAssemblyCacheEntry.cs
- ListViewItemEventArgs.cs
- CapabilitiesAssignment.cs
- util.cs
- ComponentCache.cs
- Deflater.cs
- ColorAnimation.cs
- DocumentGrid.cs
- OdbcException.cs
- RtType.cs
- HelpProvider.cs
- UTF32Encoding.cs
- MasterPageParser.cs
- NetworkInterface.cs
- InvalidOleVariantTypeException.cs
- UnsafeNativeMethods.cs
- WebPartCloseVerb.cs
- ProcessHostMapPath.cs
- StrongName.cs
- AndAlso.cs
- SqlFormatter.cs
- StaticDataManager.cs
- CodeExpressionStatement.cs
- DNS.cs
- SHA256.cs
- SafeCloseHandleCritical.cs
- ChannelSinkStacks.cs
- VirtualPathProvider.cs
- SafeEventHandle.cs
- ProgressBarHighlightConverter.cs
- ConnectionPointCookie.cs
- PerspectiveCamera.cs
- StickyNote.cs
- ServiceRoute.cs
- AsyncOperation.cs
- HttpCookieCollection.cs
- ServerIdentity.cs
- RepeaterItemEventArgs.cs
- PageVisual.cs
- KeyValueInternalCollection.cs
- _TransmitFileOverlappedAsyncResult.cs
- Hash.cs
- CrossSiteScriptingValidation.cs
- SafeReadContext.cs
- DiscreteKeyFrames.cs
- login.cs
- StreamGeometryContext.cs
- embossbitmapeffect.cs
- QueryOutputWriter.cs
- RtfNavigator.cs
- PropertyChangedEventManager.cs
- NumericExpr.cs
- NetTcpSecurityElement.cs
- HotSpotCollection.cs
- SourceFileInfo.cs
- SpecularMaterial.cs
- WebPartConnectionsCancelVerb.cs
- HtmlEmptyTagControlBuilder.cs
- TreeNodeBindingCollection.cs
- DynamicPropertyReader.cs
- DialogResultConverter.cs
- DocumentApplicationJournalEntry.cs
- TaskFormBase.cs
- NativeMethods.cs
- PrinterSettings.cs
- ArrayWithOffset.cs
- CompilerWrapper.cs
- ReservationCollection.cs
- CompositeControl.cs
- QueryServiceConfigHandle.cs
- SiteMapDataSourceView.cs
- UriTemplateTableMatchCandidate.cs
- ToolStripContentPanelRenderEventArgs.cs
- Visual3D.cs
- TableAdapterManagerNameHandler.cs
- PowerModeChangedEventArgs.cs
- SQLBoolean.cs
- TableLayoutColumnStyleCollection.cs
- SortKey.cs