Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Data / XmlNamespaceMappingCollection.cs / 1 / XmlNamespaceMappingCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Implementation of XmlNamespaceMappingCollection object. // // Specs: http://avalon/connecteddata/M5%20Specs/XmlDataSource.mht // http://avalon/connecteddata/M5%20Specs/WCP%20DataSources.mht // //--------------------------------------------------------------------------- using System; using System.Collections; // IEnumerator using System.Collections.Generic; // ICollectionusing System.Xml; using System.Windows.Markup; using MS.Utility; namespace System.Windows.Data { /// /// XmlNamespaceMappingCollection Class /// Used to declare namespaces to be used in Xml data binding XPath queries /// [Localizability(LocalizationCategory.NeverLocalize)] public class XmlNamespaceMappingCollection : XmlNamespaceManager, ICollection, IAddChildInternal { /// /// Constructor /// public XmlNamespaceMappingCollection() : base(new NameTable()) {} #region IAddChild ////// IAddChild implementation /// /// void IAddChild.AddChild(object value) { AddChild(value); } ////// /// IAddChild implementation /// /// protected virtual void AddChild(object value) { XmlNamespaceMapping mapping = value as XmlNamespaceMapping; if (mapping == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMapping, value.GetType().FullName), "value"); Add(mapping); } ////// IAddChild implementation /// /// void IAddChild.AddText(string text) { AddText(text); } ////// IAddChild implementation /// /// protected virtual void AddText(string text) { if (text == null) throw new ArgumentNullException("text"); XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } #endregion #region ICollection/// /// Add XmlNamespaceMapping /// ///mapping is null public void Add(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); // this.AddNamespace(mapping.Prefix, mapping.Uri.OriginalString); } ////// Remove all XmlNamespaceMappings /// ////// This is potentially an expensive operation. /// It may be cheaper to simply create a new XmlNamespaceMappingCollection. /// public void Clear() { int count = Count; XmlNamespaceMapping[] array = new XmlNamespaceMapping[count]; CopyTo(array, 0); for (int i = 0; i < count; ++i) { Remove(array[i]); } } ////// Add XmlNamespaceMapping /// ///mapping is null public bool Contains(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); return (this.LookupNamespace(mapping.Prefix) == mapping.Uri.OriginalString); } ////// Copy XmlNamespaceMappings to array /// public void CopyTo(XmlNamespaceMapping[] array, int arrayIndex) { if (array == null) throw new ArgumentNullException("array"); int i = arrayIndex; int maxLength = array.Length; foreach (XmlNamespaceMapping mapping in this) { if (i >= maxLength) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array")); array[i] = mapping; ++ i; } } ////// Remove XmlNamespaceMapping /// ////// true if the mapping was removed /// ///mapping is null public bool Remove(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); if (Contains(mapping)) { this.RemoveNamespace(mapping.Prefix, mapping.Uri.OriginalString); return true; } return false; } ////// Count of the number of XmlNamespaceMappings /// public int Count { get { int count = 0; foreach (XmlNamespaceMapping mapping in this) { ++ count; } return count; } } ////// Value to indicate if this collection is read only /// public bool IsReadOnly { get { return false; } } ////// IEnumerable implementation. /// ////// This enables the serializer to serialize the contents of the XmlNamespaceMappingCollection. /// The default namespaces (xnm, xml, and string.Empty) are not included in this enumeration. /// public override IEnumerator GetEnumerator() { return ProtectedGetEnumerator(); } ////// IEnumerable (generic) implementation. /// IEnumeratorIEnumerable .GetEnumerator() { return ProtectedGetEnumerator(); } /// /// Protected member for use by IEnumerable implementations. /// protected IEnumeratorProtectedGetEnumerator() { IEnumerator enumerator = BaseEnumerator; while (enumerator.MoveNext()) { string prefix = (string) enumerator.Current; // ignore the default namespaces added automatically in the XmlNamespaceManager if (prefix == "xmlns" || prefix == "xml") continue; string ns = this.LookupNamespace(prefix); // ignore the empty prefix if the namespace has not been reassigned if ((prefix == string.Empty) && (ns == string.Empty)) continue; Uri uri = new Uri(ns, UriKind.RelativeOrAbsolute); XmlNamespaceMapping xnm = new XmlNamespaceMapping(prefix, uri); yield return xnm; } } // The iterator above cannot access base.GetEnumerator directly - this // causes build warning 1911, and makes MoveNext throw a security // exception under partial trust (bug 1785518). Accessing it indirectly // through this property fixes the problem. private IEnumerator BaseEnumerator { get { return base.GetEnumerator(); } } #endregion ICollection } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Implementation of XmlNamespaceMappingCollection object. // // Specs: http://avalon/connecteddata/M5%20Specs/XmlDataSource.mht // http://avalon/connecteddata/M5%20Specs/WCP%20DataSources.mht // //--------------------------------------------------------------------------- using System; using System.Collections; // IEnumerator using System.Collections.Generic; // ICollectionusing System.Xml; using System.Windows.Markup; using MS.Utility; namespace System.Windows.Data { /// /// XmlNamespaceMappingCollection Class /// Used to declare namespaces to be used in Xml data binding XPath queries /// [Localizability(LocalizationCategory.NeverLocalize)] public class XmlNamespaceMappingCollection : XmlNamespaceManager, ICollection, IAddChildInternal { /// /// Constructor /// public XmlNamespaceMappingCollection() : base(new NameTable()) {} #region IAddChild ////// IAddChild implementation /// /// void IAddChild.AddChild(object value) { AddChild(value); } ////// /// IAddChild implementation /// /// protected virtual void AddChild(object value) { XmlNamespaceMapping mapping = value as XmlNamespaceMapping; if (mapping == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMapping, value.GetType().FullName), "value"); Add(mapping); } ////// IAddChild implementation /// /// void IAddChild.AddText(string text) { AddText(text); } ////// IAddChild implementation /// /// protected virtual void AddText(string text) { if (text == null) throw new ArgumentNullException("text"); XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } #endregion #region ICollection/// /// Add XmlNamespaceMapping /// ///mapping is null public void Add(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); // this.AddNamespace(mapping.Prefix, mapping.Uri.OriginalString); } ////// Remove all XmlNamespaceMappings /// ////// This is potentially an expensive operation. /// It may be cheaper to simply create a new XmlNamespaceMappingCollection. /// public void Clear() { int count = Count; XmlNamespaceMapping[] array = new XmlNamespaceMapping[count]; CopyTo(array, 0); for (int i = 0; i < count; ++i) { Remove(array[i]); } } ////// Add XmlNamespaceMapping /// ///mapping is null public bool Contains(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); return (this.LookupNamespace(mapping.Prefix) == mapping.Uri.OriginalString); } ////// Copy XmlNamespaceMappings to array /// public void CopyTo(XmlNamespaceMapping[] array, int arrayIndex) { if (array == null) throw new ArgumentNullException("array"); int i = arrayIndex; int maxLength = array.Length; foreach (XmlNamespaceMapping mapping in this) { if (i >= maxLength) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array")); array[i] = mapping; ++ i; } } ////// Remove XmlNamespaceMapping /// ////// true if the mapping was removed /// ///mapping is null public bool Remove(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); if (Contains(mapping)) { this.RemoveNamespace(mapping.Prefix, mapping.Uri.OriginalString); return true; } return false; } ////// Count of the number of XmlNamespaceMappings /// public int Count { get { int count = 0; foreach (XmlNamespaceMapping mapping in this) { ++ count; } return count; } } ////// Value to indicate if this collection is read only /// public bool IsReadOnly { get { return false; } } ////// IEnumerable implementation. /// ////// This enables the serializer to serialize the contents of the XmlNamespaceMappingCollection. /// The default namespaces (xnm, xml, and string.Empty) are not included in this enumeration. /// public override IEnumerator GetEnumerator() { return ProtectedGetEnumerator(); } ////// IEnumerable (generic) implementation. /// IEnumeratorIEnumerable .GetEnumerator() { return ProtectedGetEnumerator(); } /// /// Protected member for use by IEnumerable implementations. /// protected IEnumeratorProtectedGetEnumerator() { IEnumerator enumerator = BaseEnumerator; while (enumerator.MoveNext()) { string prefix = (string) enumerator.Current; // ignore the default namespaces added automatically in the XmlNamespaceManager if (prefix == "xmlns" || prefix == "xml") continue; string ns = this.LookupNamespace(prefix); // ignore the empty prefix if the namespace has not been reassigned if ((prefix == string.Empty) && (ns == string.Empty)) continue; Uri uri = new Uri(ns, UriKind.RelativeOrAbsolute); XmlNamespaceMapping xnm = new XmlNamespaceMapping(prefix, uri); yield return xnm; } } // The iterator above cannot access base.GetEnumerator directly - this // causes build warning 1911, and makes MoveNext throw a security // exception under partial trust (bug 1785518). Accessing it indirectly // through this property fixes the problem. private IEnumerator BaseEnumerator { get { return base.GetEnumerator(); } } #endregion ICollection } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
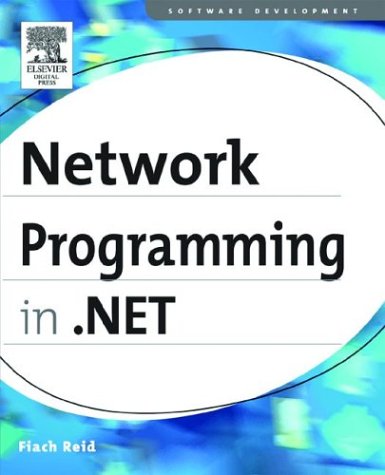
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BamlResourceContent.cs
- Debug.cs
- ChameleonKey.cs
- RectAnimationClockResource.cs
- IconEditor.cs
- EdgeProfileValidation.cs
- XmlDocumentSerializer.cs
- CodeAssignStatement.cs
- PropertyToken.cs
- WebPartAddingEventArgs.cs
- _AutoWebProxyScriptEngine.cs
- XmlNodeComparer.cs
- FrameDimension.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- NativeMethods.cs
- MediaEntryAttribute.cs
- ClosableStream.cs
- ExceptionUtil.cs
- ThreadTrace.cs
- RepeaterItemEventArgs.cs
- ProxyDataContractResolver.cs
- UIPropertyMetadata.cs
- Rect.cs
- HandleDictionary.cs
- DataGridViewAdvancedBorderStyle.cs
- MemberAssignmentAnalysis.cs
- Filter.cs
- ConfigurationStrings.cs
- ProcessHostServerConfig.cs
- BoundPropertyEntry.cs
- XmlSchemaAppInfo.cs
- HttpStaticObjectsCollectionWrapper.cs
- MergeLocalizationDirectives.cs
- OuterGlowBitmapEffect.cs
- DateTimeFormatInfoScanner.cs
- CharEntityEncoderFallback.cs
- DynamicField.cs
- WebPartActionVerb.cs
- SrgsElementFactory.cs
- ExitEventArgs.cs
- oledbmetadatacolumnnames.cs
- Font.cs
- WindowsGraphicsCacheManager.cs
- Itemizer.cs
- Type.cs
- StatusBarAutomationPeer.cs
- PathGeometry.cs
- CompressEmulationStream.cs
- EncodingTable.cs
- StorageFunctionMapping.cs
- FileDialogCustomPlace.cs
- LocatorBase.cs
- ServiceDescriptions.cs
- OciEnlistContext.cs
- Knowncolors.cs
- ContractBase.cs
- HttpHandlersSection.cs
- InvalidateEvent.cs
- EventPrivateKey.cs
- DoubleUtil.cs
- ConditionalWeakTable.cs
- WhereaboutsReader.cs
- MenuItemStyle.cs
- EntityDataSourceSelectedEventArgs.cs
- TextEditor.cs
- DispatchWrapper.cs
- ServiceNameCollection.cs
- DataViewListener.cs
- ComponentResourceManager.cs
- ToolStripPanel.cs
- ServicePointManagerElement.cs
- _CommandStream.cs
- TemplateContent.cs
- TextEmbeddedObject.cs
- TreeChangeInfo.cs
- SmiRequestExecutor.cs
- StrongNameIdentityPermission.cs
- COMException.cs
- ModuleBuilderData.cs
- Soap12ProtocolImporter.cs
- AdRotator.cs
- MultiBindingExpression.cs
- DescendentsWalkerBase.cs
- Tracking.cs
- PageStatePersister.cs
- TraceLevelStore.cs
- CryptoHelper.cs
- CaseCqlBlock.cs
- MessageQueuePermissionAttribute.cs
- XmlNodeReader.cs
- WebPartCatalogAddVerb.cs
- TypeUtil.cs
- VirtualPathProvider.cs
- VisualStyleInformation.cs
- CodeGenerator.cs
- InvalidOperationException.cs
- LicenseException.cs
- XPathCompileException.cs
- DataTableReaderListener.cs
- infer.cs