Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / FileDialogCustomPlace.cs / 1305376 / FileDialogCustomPlace.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.IO; using System.Security; using System.Security.Permissions; using System.Text; namespace System.Windows.Forms { //Sample Guids // internal const string ComputerFolder = "0AC0837C-BBF8-452A-850D-79D08E667CA7"; // internal const string Favorites = "1777F761-68AD-4D8A-87BD-30B759FA33DD"; // internal const string Documents = "FDD39AD0-238F-46AF-ADB4-6C85480369C7"; // internal const string Profile = "5E6C858F-0E22-4760-9AFE-EA3317B67173"; public class FileDialogCustomPlace { private string _path = ""; private Guid _knownFolderGuid = Guid.Empty; public FileDialogCustomPlace(string path) { this.Path = path; } public FileDialogCustomPlace(Guid knownFolderGuid) { this.KnownFolderGuid = knownFolderGuid; } public string Path { get { if (string.IsNullOrEmpty(this._path)) { return String.Empty; } return this._path; } set { this._path = value ?? ""; this._knownFolderGuid = Guid.Empty; } } public Guid KnownFolderGuid { get { return this._knownFolderGuid; } set { this._path = String.Empty; this._knownFolderGuid = value; } } public override string ToString() { return string.Format(System.Globalization.CultureInfo.CurrentCulture, "{0} Path: {1} KnownFolderGuid: {2}", base.ToString(), this.Path, this.KnownFolderGuid); } internal FileDialogNative.IShellItem GetNativePath() { //This can throw in a multitude of ways if the path or Guid doesn't correspond //to an actual filesystem directory. Caller is responsible for handling these situations. string filePathString = ""; if (!string.IsNullOrEmpty(this._path)) { filePathString = this._path; } else { filePathString = GetFolderLocation(this._knownFolderGuid); } if (string.IsNullOrEmpty(filePathString)) { return null; } else { return FileDialog.GetShellItemForPath(filePathString); } } private static string GetFolderLocation(Guid folderGuid) { //returns a null string if the path can't be found //SECURITY: This exposes the filesystem path of the GUID. The returned value // must not be made available to user code. if (!UnsafeNativeMethods.IsVista) { return null; } StringBuilder path = new StringBuilder(NativeMethods.MAX_PATH); int result = UnsafeNativeMethods.Shell32.SHGetFolderPathEx(ref folderGuid, 0, IntPtr.Zero, path, (uint)path.Capacity); if (NativeMethods.S_OK == result) { string ret = path.ToString(); return ret; } else { // 0x80070002 is an explicit FileNotFound error. return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.IO; using System.Security; using System.Security.Permissions; using System.Text; namespace System.Windows.Forms { //Sample Guids // internal const string ComputerFolder = "0AC0837C-BBF8-452A-850D-79D08E667CA7"; // internal const string Favorites = "1777F761-68AD-4D8A-87BD-30B759FA33DD"; // internal const string Documents = "FDD39AD0-238F-46AF-ADB4-6C85480369C7"; // internal const string Profile = "5E6C858F-0E22-4760-9AFE-EA3317B67173"; public class FileDialogCustomPlace { private string _path = ""; private Guid _knownFolderGuid = Guid.Empty; public FileDialogCustomPlace(string path) { this.Path = path; } public FileDialogCustomPlace(Guid knownFolderGuid) { this.KnownFolderGuid = knownFolderGuid; } public string Path { get { if (string.IsNullOrEmpty(this._path)) { return String.Empty; } return this._path; } set { this._path = value ?? ""; this._knownFolderGuid = Guid.Empty; } } public Guid KnownFolderGuid { get { return this._knownFolderGuid; } set { this._path = String.Empty; this._knownFolderGuid = value; } } public override string ToString() { return string.Format(System.Globalization.CultureInfo.CurrentCulture, "{0} Path: {1} KnownFolderGuid: {2}", base.ToString(), this.Path, this.KnownFolderGuid); } internal FileDialogNative.IShellItem GetNativePath() { //This can throw in a multitude of ways if the path or Guid doesn't correspond //to an actual filesystem directory. Caller is responsible for handling these situations. string filePathString = ""; if (!string.IsNullOrEmpty(this._path)) { filePathString = this._path; } else { filePathString = GetFolderLocation(this._knownFolderGuid); } if (string.IsNullOrEmpty(filePathString)) { return null; } else { return FileDialog.GetShellItemForPath(filePathString); } } private static string GetFolderLocation(Guid folderGuid) { //returns a null string if the path can't be found //SECURITY: This exposes the filesystem path of the GUID. The returned value // must not be made available to user code. if (!UnsafeNativeMethods.IsVista) { return null; } StringBuilder path = new StringBuilder(NativeMethods.MAX_PATH); int result = UnsafeNativeMethods.Shell32.SHGetFolderPathEx(ref folderGuid, 0, IntPtr.Zero, path, (uint)path.Capacity); if (NativeMethods.S_OK == result) { string ret = path.ToString(); return ret; } else { // 0x80070002 is an explicit FileNotFound error. return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
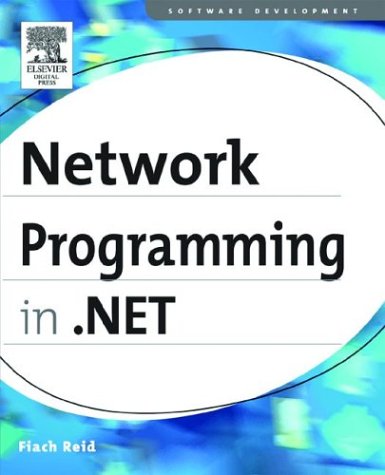
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Binding.cs
- XPathNodeList.cs
- RoleBoolean.cs
- TraceContext.cs
- SafeNativeMemoryHandle.cs
- ItemsPresenter.cs
- NumberFunctions.cs
- CommandValueSerializer.cs
- BitmapMetadataEnumerator.cs
- FtpRequestCacheValidator.cs
- KeyInfo.cs
- SmtpLoginAuthenticationModule.cs
- HttpDictionary.cs
- TransformerInfo.cs
- XmlSchemaDocumentation.cs
- ObjectFullSpanRewriter.cs
- XamlClipboardData.cs
- Int64Animation.cs
- SspiSafeHandles.cs
- ImageKeyConverter.cs
- ValueTypePropertyReference.cs
- CheckBoxFlatAdapter.cs
- DrawingDrawingContext.cs
- PrimitiveSchema.cs
- MatrixAnimationUsingPath.cs
- DeviceContext2.cs
- WebRequest.cs
- RuntimeEnvironment.cs
- MarkupProperty.cs
- SystemKeyConverter.cs
- SqlDataSourceCommandParser.cs
- InfoCardRSACryptoProvider.cs
- SystemUnicastIPAddressInformation.cs
- XmlEncoding.cs
- CommonDialog.cs
- SqlUDTStorage.cs
- ChangeBlockUndoRecord.cs
- ArcSegment.cs
- lengthconverter.cs
- ChildDocumentBlock.cs
- MenuItem.cs
- EnumValAlphaComparer.cs
- GlyphingCache.cs
- PeerNameResolver.cs
- ListParaClient.cs
- WebPartConnectionsEventArgs.cs
- ProcessStartInfo.cs
- Profiler.cs
- TextContainerChangedEventArgs.cs
- GAC.cs
- CustomLineCap.cs
- controlskin.cs
- CodeTypeOfExpression.cs
- DecoderFallbackWithFailureFlag.cs
- DbConnectionPoolIdentity.cs
- SchemaTableOptionalColumn.cs
- KnownIds.cs
- CodePropertyReferenceExpression.cs
- PartManifestEntry.cs
- DataBindingHandlerAttribute.cs
- TagMapInfo.cs
- DataControlFieldTypeEditor.cs
- NativeCompoundFileAPIs.cs
- RelationshipWrapper.cs
- LineProperties.cs
- BufferedStream.cs
- SpecularMaterial.cs
- LockCookie.cs
- ServiceCredentialsElement.cs
- NetworkInterface.cs
- DrawingBrush.cs
- ExtensionDataObject.cs
- StyleBamlTreeBuilder.cs
- TrackingServices.cs
- GetWinFXPath.cs
- CngKey.cs
- FrameworkPropertyMetadata.cs
- MatrixKeyFrameCollection.cs
- DynamicFilter.cs
- Exceptions.cs
- DetailsViewRow.cs
- X509CertificateCollection.cs
- SpellCheck.cs
- CodeExpressionCollection.cs
- OneOfElement.cs
- Typography.cs
- DataBoundLiteralControl.cs
- ErrorHandler.cs
- RuleDefinitions.cs
- HeaderUtility.cs
- SendingRequestEventArgs.cs
- RootBuilder.cs
- MsmqHostedTransportManager.cs
- RightsManagementSuppressedStream.cs
- XmlStreamNodeWriter.cs
- ComPersistableTypeElement.cs
- Vector3DValueSerializer.cs
- IpcClientManager.cs
- DesignerVerb.cs
- WebPartEditorCancelVerb.cs