Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Shared / MS / Internal / DecoderFallbackWithFailureFlag.cs / 1 / DecoderFallbackWithFailureFlag.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: DecoderFallbackWithFailureFlag is used when the developer wants Encoding.GetChars() method to fail // without throwing an exception when decoding cannot be performed. // Usage pattern is: // DecoderFallbackWithFailureFlag fallback = new DecoderFallbackWithFailureFlag(); // Encoding e = Encoding.GetEncoding(codePage, EncoderFallback.ExceptionFallback, fallback); // e.GetChars(bytesToDecode); // if (fallback.HasFailed) // { // // Perform fallback and reset the failure flag. // fallback.HasFailed = false; // } // // History: // 05/03/2005 : mleonov - Created // //--------------------------------------------------------------------------- using System.Text; namespace MS.Internal { ////// This class is similar to the standard DecoderExceptionFallback class, /// except that for performance reasons it sets a Boolean failure flag /// instead of throwing exception. /// internal class DecoderFallbackWithFailureFlag : DecoderFallback { public DecoderFallbackWithFailureFlag() { } public override DecoderFallbackBuffer CreateFallbackBuffer() { return new FallbackBuffer(this); } ////// The maximum number of characters this instance can return. /// public override int MaxCharCount { get { return 0; } } ////// Returns whether decoding failed. /// public bool HasFailed { get { return _hasFailed; } set { _hasFailed = value; } } private bool _hasFailed; // false by default ////// A special implementation of DecoderFallbackBuffer that sets the failure flag /// in the parent DecoderFallbackWithFailureFlag class. /// private class FallbackBuffer : DecoderFallbackBuffer { public FallbackBuffer(DecoderFallbackWithFailureFlag parent) { _parent = parent; } ////// Indicates whether a substitute string can be emitted if an input byte sequence cannot be decoded. /// Parameters specify an input byte sequence, and the index position of a byte in the input. /// /// An input array of bytes. /// The index position of a byte in bytesUnknown. ///true if a string exists that can be inserted in the output /// instead of decoding the byte specified in bytesUnknown; /// false if the input byte should be ignored. public override bool Fallback(byte[] bytesUnknown, int index) { _parent.HasFailed = true; return false; } ////// Retrieves the next character in the fallback buffer. /// ///The next Unicode character in the fallback buffer. public override char GetNextChar() { return (char)0; } ////// Prepares the GetNextChar method to retrieve the preceding character in the fallback buffer. /// ///true if the MovePrevious operation was successful; otherwise, false. public override bool MovePrevious() { return false; } ////// Gets the number of characters in this instance of DecoderFallbackBuffer that remain to be processed. /// public override int Remaining { get { return 0; } } private DecoderFallbackWithFailureFlag _parent; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: DecoderFallbackWithFailureFlag is used when the developer wants Encoding.GetChars() method to fail // without throwing an exception when decoding cannot be performed. // Usage pattern is: // DecoderFallbackWithFailureFlag fallback = new DecoderFallbackWithFailureFlag(); // Encoding e = Encoding.GetEncoding(codePage, EncoderFallback.ExceptionFallback, fallback); // e.GetChars(bytesToDecode); // if (fallback.HasFailed) // { // // Perform fallback and reset the failure flag. // fallback.HasFailed = false; // } // // History: // 05/03/2005 : mleonov - Created // //--------------------------------------------------------------------------- using System.Text; namespace MS.Internal { ////// This class is similar to the standard DecoderExceptionFallback class, /// except that for performance reasons it sets a Boolean failure flag /// instead of throwing exception. /// internal class DecoderFallbackWithFailureFlag : DecoderFallback { public DecoderFallbackWithFailureFlag() { } public override DecoderFallbackBuffer CreateFallbackBuffer() { return new FallbackBuffer(this); } ////// The maximum number of characters this instance can return. /// public override int MaxCharCount { get { return 0; } } ////// Returns whether decoding failed. /// public bool HasFailed { get { return _hasFailed; } set { _hasFailed = value; } } private bool _hasFailed; // false by default ////// A special implementation of DecoderFallbackBuffer that sets the failure flag /// in the parent DecoderFallbackWithFailureFlag class. /// private class FallbackBuffer : DecoderFallbackBuffer { public FallbackBuffer(DecoderFallbackWithFailureFlag parent) { _parent = parent; } ////// Indicates whether a substitute string can be emitted if an input byte sequence cannot be decoded. /// Parameters specify an input byte sequence, and the index position of a byte in the input. /// /// An input array of bytes. /// The index position of a byte in bytesUnknown. ///true if a string exists that can be inserted in the output /// instead of decoding the byte specified in bytesUnknown; /// false if the input byte should be ignored. public override bool Fallback(byte[] bytesUnknown, int index) { _parent.HasFailed = true; return false; } ////// Retrieves the next character in the fallback buffer. /// ///The next Unicode character in the fallback buffer. public override char GetNextChar() { return (char)0; } ////// Prepares the GetNextChar method to retrieve the preceding character in the fallback buffer. /// ///true if the MovePrevious operation was successful; otherwise, false. public override bool MovePrevious() { return false; } ////// Gets the number of characters in this instance of DecoderFallbackBuffer that remain to be processed. /// public override int Remaining { get { return 0; } } private DecoderFallbackWithFailureFlag _parent; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
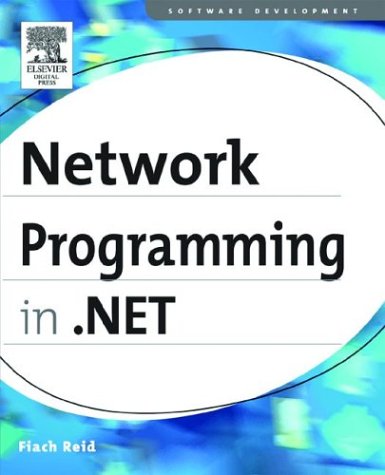
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringFormat.cs
- FontUnit.cs
- MenuTracker.cs
- Roles.cs
- SafeProcessHandle.cs
- WorkflowFileItem.cs
- TailPinnedEventArgs.cs
- DBSchemaRow.cs
- DataGridViewDataConnection.cs
- ProgressBar.cs
- MessageQueueCriteria.cs
- HttpCapabilitiesSectionHandler.cs
- TextPattern.cs
- XhtmlBasicCalendarAdapter.cs
- PageParserFilter.cs
- XPathMessageFilterElementCollection.cs
- XmlSchemaComplexContentExtension.cs
- LingerOption.cs
- DesignerDataColumn.cs
- BulletedList.cs
- IInstanceTable.cs
- ClientConfigurationSystem.cs
- ResolvedKeyFrameEntry.cs
- FormViewInsertEventArgs.cs
- SqlDependencyUtils.cs
- IItemContainerGenerator.cs
- GlyphRun.cs
- FactoryId.cs
- Fault.cs
- WebSysDescriptionAttribute.cs
- DoubleAnimation.cs
- NamedObject.cs
- FormViewPagerRow.cs
- ExpressionPrefixAttribute.cs
- FileSystemInfo.cs
- DataGridViewDataErrorEventArgs.cs
- FixedSOMTableCell.cs
- TargetPerspective.cs
- CngProvider.cs
- AccessedThroughPropertyAttribute.cs
- RequestCacheManager.cs
- LiteralTextParser.cs
- TextTreeFixupNode.cs
- _Rfc2616CacheValidators.cs
- InvalidProgramException.cs
- sqlpipe.cs
- returneventsaver.cs
- XamlSerializationHelper.cs
- BaseCAMarshaler.cs
- CodeEntryPointMethod.cs
- TextRangeBase.cs
- HtmlFormParameterWriter.cs
- RegexRunner.cs
- GrammarBuilderBase.cs
- List.cs
- StatusBarAutomationPeer.cs
- DomainUpDown.cs
- Int32.cs
- WebPartVerb.cs
- DataSetMappper.cs
- HttpModuleActionCollection.cs
- RegistryKey.cs
- GradientBrush.cs
- ExpressionWriter.cs
- XmlNamespaceMappingCollection.cs
- OdbcConnectionString.cs
- MobileUITypeEditor.cs
- TransportManager.cs
- SecondaryViewProvider.cs
- XmlSchemaAppInfo.cs
- JoinSymbol.cs
- StringAnimationUsingKeyFrames.cs
- RefType.cs
- AnimationStorage.cs
- FontNamesConverter.cs
- SerTrace.cs
- SynchronizedRandom.cs
- precedingsibling.cs
- Membership.cs
- URLIdentityPermission.cs
- MetadataPropertyAttribute.cs
- ConstrainedDataObject.cs
- SourceFilter.cs
- RectValueSerializer.cs
- GeometryConverter.cs
- ArraySegment.cs
- SelectionRange.cs
- DbBuffer.cs
- PropertyChangeTracker.cs
- ObjectCloneHelper.cs
- ArglessEventHandlerProxy.cs
- serverconfig.cs
- TrackingServices.cs
- FormsAuthenticationUserCollection.cs
- JumpPath.cs
- TabletDevice.cs
- ContextBase.cs
- XmlQualifiedName.cs
- InvokeMethodDesigner.xaml.cs
- RegionData.cs