Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Markup / StyleBamlTreeBuilder.cs / 1 / StyleBamlTreeBuilder.cs
/****************************************************************************\ * * File: StyleTreeBuilderBamlTranslator.cs * * Purpose: Class that builds a style object from BAML * * History: * 11/13/03: peterost Created * * Copyright (C) 2003 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Windows; using System.Text; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Threading; using MS.Utility; namespace System.Windows.Markup { ////// StyleTreeBuilderBamlTranslator is the TreeBuilder implementation that loads a Style object /// from BAML. /// internal class StyleTreeBuilderBamlTranslator : TreeBuilderBamlTranslator { #region Constructors ////// Constructor. Set up associated baml reader to /// read the style baml records. /// public StyleTreeBuilderBamlTranslator( ParserContext parserContext, // context Stream bamlStream, // baml stream, when reading from a file BamlRecord bamlStartRecord, // baml records start, when loading from a dictionary BamlRecord bamlIndexRecord, // Index Record in bamlRecords to start parsing at ParserStack bamlReaderStack, // reader stack ArrayList rootList, // List of root objects for overall parse. XamlParseMode parseMode, // [....] or async parse mode int maxAsyncRecords) // number to read in async mode before yielding { BamlStream = bamlStream; RecordReader = new StyleBamlRecordReader(bamlStream, bamlStartRecord, bamlIndexRecord, parserContext, bamlReaderStack, rootList); XamlParseMode = parseMode; MaxAsyncRecords = maxAsyncRecords; // Set associated record reader async info RecordReader.XamlParseMode = XamlParseMode; RecordReader.MaxAsyncRecords = MaxAsyncRecords; } #endregion Constructors #region Overrides ////// Internal Avalon method. Used to parse style section of a BAML file. /// ///An array containing the root objects in the style portion of a BAML stream public override object ParseFragment() { // if in synchronous mode then just read the baml stream and then // return. if going into async mode then build up the first tag // synchronously and then post a queue item. if (XamlParseMode == XamlParseMode.Synchronous) { RecordReader.Read(); } else { // read in the first record since binder at present // needs this. bool moreData = true; // sit in synchronous read until get first root. Need this // until we get async binder support. while (GetRoot() == null && moreData) { moreData = RecordReader.Read(true /* single record mode*/); } if (moreData && GetRoot() != null) { // before going async want to switch to async stream interfaces // so we don't block on I/O. // setup stream Manager on Reader and kick of Async Writes StreamManager = new ReadWriteStreamManager(); // RecordReader no points to the ReaderWriter stream which // is different from our BAMLStream member. RecordReader.BamlStream = StreamManager.ReaderStream; // now spin a thread to read the BAML. This can change // once we get Read support that fails if contents isn't // available instead of blocks. ThreadStart threadStart = new ThreadStart(ReadBamlAsync); Thread thread = new Thread(threadStart); thread.Start(); // post a work item to do the rest. Post(); } } return GetRoot(); } #endregion Overrides } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: StyleTreeBuilderBamlTranslator.cs * * Purpose: Class that builds a style object from BAML * * History: * 11/13/03: peterost Created * * Copyright (C) 2003 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Windows; using System.Text; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Threading; using MS.Utility; namespace System.Windows.Markup { ////// StyleTreeBuilderBamlTranslator is the TreeBuilder implementation that loads a Style object /// from BAML. /// internal class StyleTreeBuilderBamlTranslator : TreeBuilderBamlTranslator { #region Constructors ////// Constructor. Set up associated baml reader to /// read the style baml records. /// public StyleTreeBuilderBamlTranslator( ParserContext parserContext, // context Stream bamlStream, // baml stream, when reading from a file BamlRecord bamlStartRecord, // baml records start, when loading from a dictionary BamlRecord bamlIndexRecord, // Index Record in bamlRecords to start parsing at ParserStack bamlReaderStack, // reader stack ArrayList rootList, // List of root objects for overall parse. XamlParseMode parseMode, // [....] or async parse mode int maxAsyncRecords) // number to read in async mode before yielding { BamlStream = bamlStream; RecordReader = new StyleBamlRecordReader(bamlStream, bamlStartRecord, bamlIndexRecord, parserContext, bamlReaderStack, rootList); XamlParseMode = parseMode; MaxAsyncRecords = maxAsyncRecords; // Set associated record reader async info RecordReader.XamlParseMode = XamlParseMode; RecordReader.MaxAsyncRecords = MaxAsyncRecords; } #endregion Constructors #region Overrides ////// Internal Avalon method. Used to parse style section of a BAML file. /// ///An array containing the root objects in the style portion of a BAML stream public override object ParseFragment() { // if in synchronous mode then just read the baml stream and then // return. if going into async mode then build up the first tag // synchronously and then post a queue item. if (XamlParseMode == XamlParseMode.Synchronous) { RecordReader.Read(); } else { // read in the first record since binder at present // needs this. bool moreData = true; // sit in synchronous read until get first root. Need this // until we get async binder support. while (GetRoot() == null && moreData) { moreData = RecordReader.Read(true /* single record mode*/); } if (moreData && GetRoot() != null) { // before going async want to switch to async stream interfaces // so we don't block on I/O. // setup stream Manager on Reader and kick of Async Writes StreamManager = new ReadWriteStreamManager(); // RecordReader no points to the ReaderWriter stream which // is different from our BAMLStream member. RecordReader.BamlStream = StreamManager.ReaderStream; // now spin a thread to read the BAML. This can change // once we get Read support that fails if contents isn't // available instead of blocks. ThreadStart threadStart = new ThreadStart(ReadBamlAsync); Thread thread = new Thread(threadStart); thread.Start(); // post a work item to do the rest. Post(); } } return GetRoot(); } #endregion Overrides } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
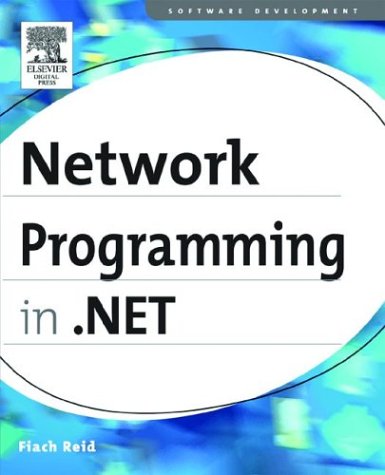
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TriggerAction.cs
- ping.cs
- LinqToSqlWrapper.cs
- DoubleAnimationClockResource.cs
- NeutralResourcesLanguageAttribute.cs
- FormDesigner.cs
- DataGridrowEditEndingEventArgs.cs
- Visitors.cs
- SqlDataSourceConfigureSelectPanel.cs
- MenuItemAutomationPeer.cs
- exports.cs
- RadialGradientBrush.cs
- CodeMemberProperty.cs
- Internal.cs
- ReflectionTypeLoadException.cs
- PolicyException.cs
- DbConnectionFactory.cs
- NativeMethods.cs
- AssociationType.cs
- MimeObjectFactory.cs
- FixedSOMTable.cs
- CodeLinePragma.cs
- ViewPort3D.cs
- EncoderExceptionFallback.cs
- DatePickerAutomationPeer.cs
- XDRSchema.cs
- CodeArgumentReferenceExpression.cs
- DataSourceExpressionCollection.cs
- Win32SafeHandles.cs
- SqlError.cs
- CipherData.cs
- XmlDataSourceNodeDescriptor.cs
- TableItemPattern.cs
- EqualityArray.cs
- ServicePoint.cs
- InstanceData.cs
- BinHexDecoder.cs
- HttpCookiesSection.cs
- SchemaContext.cs
- SafeWaitHandle.cs
- HMACSHA384.cs
- InternalDispatchObject.cs
- ChangePasswordDesigner.cs
- CompilerGeneratedAttribute.cs
- ColorConverter.cs
- DataPointer.cs
- Timer.cs
- HtmlTableRow.cs
- CircleHotSpot.cs
- State.cs
- EmptyEnumerator.cs
- ToolStripItemRenderEventArgs.cs
- BezierSegment.cs
- ECDiffieHellmanCng.cs
- SmtpReplyReaderFactory.cs
- XamlPointCollectionSerializer.cs
- ZoneIdentityPermission.cs
- PrinterSettings.cs
- EntitySetDataBindingList.cs
- DataBoundLiteralControl.cs
- ProfileService.cs
- ActivityTrace.cs
- GenericsInstances.cs
- TextTreeNode.cs
- StrongName.cs
- MenuItemBinding.cs
- MenuBase.cs
- Pair.cs
- WebPartVerbsEventArgs.cs
- ScriptingProfileServiceSection.cs
- SqlUdtInfo.cs
- GetWinFXPath.cs
- SelectionEditor.cs
- BuildResultCache.cs
- ConfigurationManagerInternalFactory.cs
- TableRowGroup.cs
- EntityDataSourceDataSelectionPanel.cs
- CompilerTypeWithParams.cs
- WebService.cs
- relpropertyhelper.cs
- MailAddressCollection.cs
- PasswordPropertyTextAttribute.cs
- IisTraceListener.cs
- XmlDocumentSerializer.cs
- ImageIndexConverter.cs
- ISFTagAndGuidCache.cs
- sqlstateclientmanager.cs
- SystemIPv6InterfaceProperties.cs
- SimpleHandlerBuildProvider.cs
- EntityDataSourceQueryBuilder.cs
- PersonalizablePropertyEntry.cs
- ClassData.cs
- RefType.cs
- StylusOverProperty.cs
- Size.cs
- RevocationPoint.cs
- SchemeSettingElement.cs
- SignedXml.cs
- OleDbRowUpdatedEvent.cs
- MobileComponentEditorPage.cs