Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / ManagedLibraries / Security / System / Security / Cryptography / Xml / CipherData.cs / 1305376 / CipherData.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // CipherData.cs // // This object implements the CipherData element. // // 04/01/2001 // namespace System.Security.Cryptography.Xml { using System; using System.Collections; using System.Xml; [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CipherData { private XmlElement m_cachedXml = null; private CipherReference m_cipherReference = null; private byte[] m_cipherValue = null; public CipherData () {} public CipherData (byte[] cipherValue) { this.CipherValue = cipherValue; } public CipherData (CipherReference cipherReference) { this.CipherReference = cipherReference; } private bool CacheValid { get { return (m_cachedXml != null); } } public CipherReference CipherReference { get { return m_cipherReference; } set { if (value == null) throw new ArgumentNullException("value"); if (this.CipherValue != null) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_CipherValueElementRequired")); m_cipherReference = value; m_cachedXml = null; } } public byte[] CipherValue { get { return m_cipherValue; } set { if (value == null) throw new ArgumentNullException("value"); if (this.CipherReference != null) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_CipherValueElementRequired")); m_cipherValue = (byte[]) value.Clone(); m_cachedXml = null; } } public XmlElement GetXml () { if (CacheValid) return m_cachedXml; XmlDocument document = new XmlDocument(); document.PreserveWhitespace = true; return GetXml(document); } internal XmlElement GetXml (XmlDocument document) { // Create the CipherData element XmlElement cipherDataElement = (XmlElement)document.CreateElement("CipherData", EncryptedXml.XmlEncNamespaceUrl); if (CipherValue != null) { XmlElement cipherValueElement = document.CreateElement("CipherValue", EncryptedXml.XmlEncNamespaceUrl); cipherValueElement.AppendChild(document.CreateTextNode(Convert.ToBase64String(CipherValue))); cipherDataElement.AppendChild(cipherValueElement); } else { // No CipherValue specified, see if there is a CipherReference if (CipherReference == null) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_CipherValueElementRequired")); cipherDataElement.AppendChild(CipherReference.GetXml(document)); } return cipherDataElement; } public void LoadXml (XmlElement value) { if (value == null) throw new ArgumentNullException("value"); XmlNamespaceManager nsm = new XmlNamespaceManager(value.OwnerDocument.NameTable); nsm.AddNamespace("enc", EncryptedXml.XmlEncNamespaceUrl); XmlNode cipherValueNode = value.SelectSingleNode("enc:CipherValue", nsm); XmlNode cipherReferenceNode = value.SelectSingleNode("enc:CipherReference", nsm); if (cipherValueNode != null) { if (cipherReferenceNode != null) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_CipherValueElementRequired")); m_cipherValue = Convert.FromBase64String(Utils.DiscardWhiteSpaces(cipherValueNode.InnerText)); } else if (cipherReferenceNode != null) { m_cipherReference = new CipherReference(); m_cipherReference.LoadXml((XmlElement) cipherReferenceNode); } else { throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_CipherValueElementRequired")); } // Save away the cached value m_cachedXml = value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // CipherData.cs // // This object implements the CipherData element. // // 04/01/2001 // namespace System.Security.Cryptography.Xml { using System; using System.Collections; using System.Xml; [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CipherData { private XmlElement m_cachedXml = null; private CipherReference m_cipherReference = null; private byte[] m_cipherValue = null; public CipherData () {} public CipherData (byte[] cipherValue) { this.CipherValue = cipherValue; } public CipherData (CipherReference cipherReference) { this.CipherReference = cipherReference; } private bool CacheValid { get { return (m_cachedXml != null); } } public CipherReference CipherReference { get { return m_cipherReference; } set { if (value == null) throw new ArgumentNullException("value"); if (this.CipherValue != null) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_CipherValueElementRequired")); m_cipherReference = value; m_cachedXml = null; } } public byte[] CipherValue { get { return m_cipherValue; } set { if (value == null) throw new ArgumentNullException("value"); if (this.CipherReference != null) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_CipherValueElementRequired")); m_cipherValue = (byte[]) value.Clone(); m_cachedXml = null; } } public XmlElement GetXml () { if (CacheValid) return m_cachedXml; XmlDocument document = new XmlDocument(); document.PreserveWhitespace = true; return GetXml(document); } internal XmlElement GetXml (XmlDocument document) { // Create the CipherData element XmlElement cipherDataElement = (XmlElement)document.CreateElement("CipherData", EncryptedXml.XmlEncNamespaceUrl); if (CipherValue != null) { XmlElement cipherValueElement = document.CreateElement("CipherValue", EncryptedXml.XmlEncNamespaceUrl); cipherValueElement.AppendChild(document.CreateTextNode(Convert.ToBase64String(CipherValue))); cipherDataElement.AppendChild(cipherValueElement); } else { // No CipherValue specified, see if there is a CipherReference if (CipherReference == null) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_CipherValueElementRequired")); cipherDataElement.AppendChild(CipherReference.GetXml(document)); } return cipherDataElement; } public void LoadXml (XmlElement value) { if (value == null) throw new ArgumentNullException("value"); XmlNamespaceManager nsm = new XmlNamespaceManager(value.OwnerDocument.NameTable); nsm.AddNamespace("enc", EncryptedXml.XmlEncNamespaceUrl); XmlNode cipherValueNode = value.SelectSingleNode("enc:CipherValue", nsm); XmlNode cipherReferenceNode = value.SelectSingleNode("enc:CipherReference", nsm); if (cipherValueNode != null) { if (cipherReferenceNode != null) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_CipherValueElementRequired")); m_cipherValue = Convert.FromBase64String(Utils.DiscardWhiteSpaces(cipherValueNode.InnerText)); } else if (cipherReferenceNode != null) { m_cipherReference = new CipherReference(); m_cipherReference.LoadXml((XmlElement) cipherReferenceNode); } else { throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_CipherValueElementRequired")); } // Save away the cached value m_cachedXml = value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
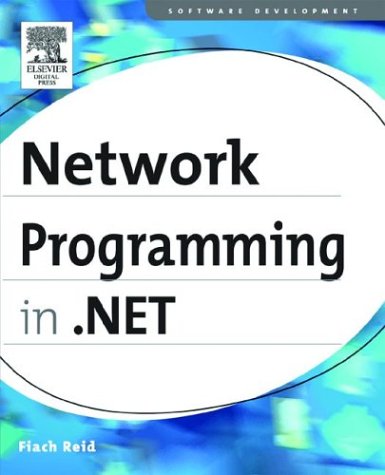
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SingleResultAttribute.cs
- XmlSchemaNotation.cs
- QuaternionAnimationUsingKeyFrames.cs
- XmlTextWriter.cs
- SystemNetworkInterface.cs
- HasCopySemanticsAttribute.cs
- DynamicILGenerator.cs
- DbProviderFactoriesConfigurationHandler.cs
- ObfuscateAssemblyAttribute.cs
- GetWinFXPath.cs
- MethodCallTranslator.cs
- SchemaCollectionCompiler.cs
- SerializationHelper.cs
- ASCIIEncoding.cs
- ClaimTypes.cs
- XMLSchema.cs
- ImageField.cs
- GCHandleCookieTable.cs
- ListItemParagraph.cs
- Compilation.cs
- DataGridViewSelectedRowCollection.cs
- XamlWriter.cs
- Transform.cs
- ClientBuildManagerCallback.cs
- assemblycache.cs
- ChangesetResponse.cs
- CompiledXpathExpr.cs
- ToolStripContentPanel.cs
- TabControlCancelEvent.cs
- CustomError.cs
- XmlSubtreeReader.cs
- SafeSecurityHelper.cs
- ComPlusThreadInitializer.cs
- UrlMappingCollection.cs
- ScrollProperties.cs
- _HelperAsyncResults.cs
- ProcessRequestArgs.cs
- Calendar.cs
- ViewKeyConstraint.cs
- HtmlLink.cs
- HighContrastHelper.cs
- Baml2006KeyRecord.cs
- Stopwatch.cs
- DrawListViewSubItemEventArgs.cs
- HttpHandlerAction.cs
- TreeChangeInfo.cs
- PeerNameRegistration.cs
- InputScope.cs
- DataTableMapping.cs
- CalendarButton.cs
- XmlComment.cs
- OrderingQueryOperator.cs
- XhtmlBasicPhoneCallAdapter.cs
- SqlNotificationRequest.cs
- UmAlQuraCalendar.cs
- NumericUpDownAccelerationCollection.cs
- DbDeleteCommandTree.cs
- AlternationConverter.cs
- ListControlBoundActionList.cs
- InvokeBinder.cs
- VirtualizedItemPattern.cs
- DoubleSumAggregationOperator.cs
- DependencyObjectPropertyDescriptor.cs
- ConfigurationManager.cs
- SamlAssertionDirectKeyIdentifierClause.cs
- FileVersion.cs
- RequestCacheEntry.cs
- BehaviorEditorPart.cs
- SharedPersonalizationStateInfo.cs
- HandlerBase.cs
- CodeTypeParameterCollection.cs
- SqlInternalConnectionTds.cs
- storepermissionattribute.cs
- Crc32Helper.cs
- SimpleFieldTemplateUserControl.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- MenuAutoFormat.cs
- NotifyInputEventArgs.cs
- QueryStringParameter.cs
- ObjectDataSourceStatusEventArgs.cs
- PersonalizationEntry.cs
- SymLanguageVendor.cs
- DrawingGroupDrawingContext.cs
- DBCSCodePageEncoding.cs
- SmiGettersStream.cs
- BitmapCache.cs
- MenuItemStyleCollection.cs
- DrawListViewColumnHeaderEventArgs.cs
- RawStylusActions.cs
- ColumnBinding.cs
- DependencyPropertyHelper.cs
- UserControlCodeDomTreeGenerator.cs
- DataBindingExpressionBuilder.cs
- AppDomainShutdownMonitor.cs
- OneOfConst.cs
- SQLByteStorage.cs
- FixedNode.cs
- PeerResolverBindingElement.cs
- Command.cs
- EntityClientCacheEntry.cs