Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / DrawListViewSubItemEventArgs.cs / 1305376 / DrawListViewSubItemEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.ComponentModel; using System.Drawing; using System.Windows.Forms.Internal; using Microsoft.Win32; using System.Windows.Forms.VisualStyles; ////// /// This class contains the information a user needs to paint ListView sub-items (Details view only). /// public class DrawListViewSubItemEventArgs : EventArgs { private readonly Graphics graphics; private readonly Rectangle bounds; private readonly ListViewItem item; private readonly ListViewItem.ListViewSubItem subItem; private readonly int itemIndex; private readonly int columnIndex; private readonly ColumnHeader header; private readonly ListViewItemStates itemState; private bool drawDefault; ////// /// Creates a new DrawListViewSubItemEventArgs with the given parameters. /// public DrawListViewSubItemEventArgs(Graphics graphics, Rectangle bounds, ListViewItem item, ListViewItem.ListViewSubItem subItem, int itemIndex, int columnIndex, ColumnHeader header, ListViewItemStates itemState) { this.graphics = graphics; this.bounds = bounds; this.item = item; this.subItem = subItem; this.itemIndex = itemIndex; this.columnIndex = columnIndex; this.header = header; this.itemState = itemState; } ////// /// Causes the item do be drawn by the system instead of owner drawn. /// public bool DrawDefault { get { return drawDefault; } set { drawDefault = value; } } ////// /// Graphics object with which painting should be done. /// public Graphics Graphics { get { return graphics; } } ////// /// The rectangle outlining the area in which the painting should be done. /// public Rectangle Bounds { get { return bounds; } } ////// /// The parent item. /// public ListViewItem Item { get { return item; } } ////// /// The parent item. /// public ListViewItem.ListViewSubItem SubItem { get { return subItem; } } ////// /// The index in the ListView of the parent item. /// public int ItemIndex { get { return itemIndex; } } ////// /// The column index of this sub-item. /// public int ColumnIndex { get { return columnIndex; } } ////// /// The header of this sub-item's column /// public ColumnHeader Header { get { return header; } } ////// /// Miscellaneous state information pertaining to the parent item. /// public ListViewItemStates ItemState { get { return itemState; } } ////// /// Draws the sub-item's background. /// public void DrawBackground() { Color backColor = (itemIndex == -1) ? item.BackColor : subItem.BackColor; using (Brush backBrush = new SolidBrush(backColor)) { Graphics.FillRectangle(backBrush, bounds); } } ////// /// Draws a focus rectangle in the given bounds, if the item has focus. /// public void DrawFocusRectangle(Rectangle bounds) { if((itemState & ListViewItemStates.Focused) == ListViewItemStates.Focused) { ControlPaint.DrawFocusRectangle(graphics, Rectangle.Inflate(bounds, -1, -1), item.ForeColor, item.BackColor); } } ////// /// Draws the sub-item's text (overloaded) /// public void DrawText() { // Map the ColumnHeader::TextAlign to the TextFormatFlags. HorizontalAlignment hAlign = header.TextAlign; TextFormatFlags flags = (hAlign == HorizontalAlignment.Left) ? TextFormatFlags.Left : ((hAlign == HorizontalAlignment.Center) ? TextFormatFlags.HorizontalCenter : TextFormatFlags.Right); flags |= TextFormatFlags.WordEllipsis; DrawText(flags); } ////// /// Draws the sub-item's text (overloaded) - takes a TextFormatFlags argument. /// [ SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters") // We want to measure the size of blank space. // So we don't have to localize it. ] public void DrawText(TextFormatFlags flags) { string text = (itemIndex == -1) ? item.Text : subItem.Text; Font font = (itemIndex == -1) ? item.Font : subItem.Font; Color color = (itemIndex == -1) ? item.ForeColor : subItem.ForeColor; int padding = TextRenderer.MeasureText(" ", font).Width; Rectangle newBounds = Rectangle.Inflate(bounds, -padding, 0); TextRenderer.DrawText(graphics, text, font, newBounds, color, flags); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.ComponentModel; using System.Drawing; using System.Windows.Forms.Internal; using Microsoft.Win32; using System.Windows.Forms.VisualStyles; ////// /// This class contains the information a user needs to paint ListView sub-items (Details view only). /// public class DrawListViewSubItemEventArgs : EventArgs { private readonly Graphics graphics; private readonly Rectangle bounds; private readonly ListViewItem item; private readonly ListViewItem.ListViewSubItem subItem; private readonly int itemIndex; private readonly int columnIndex; private readonly ColumnHeader header; private readonly ListViewItemStates itemState; private bool drawDefault; ////// /// Creates a new DrawListViewSubItemEventArgs with the given parameters. /// public DrawListViewSubItemEventArgs(Graphics graphics, Rectangle bounds, ListViewItem item, ListViewItem.ListViewSubItem subItem, int itemIndex, int columnIndex, ColumnHeader header, ListViewItemStates itemState) { this.graphics = graphics; this.bounds = bounds; this.item = item; this.subItem = subItem; this.itemIndex = itemIndex; this.columnIndex = columnIndex; this.header = header; this.itemState = itemState; } ////// /// Causes the item do be drawn by the system instead of owner drawn. /// public bool DrawDefault { get { return drawDefault; } set { drawDefault = value; } } ////// /// Graphics object with which painting should be done. /// public Graphics Graphics { get { return graphics; } } ////// /// The rectangle outlining the area in which the painting should be done. /// public Rectangle Bounds { get { return bounds; } } ////// /// The parent item. /// public ListViewItem Item { get { return item; } } ////// /// The parent item. /// public ListViewItem.ListViewSubItem SubItem { get { return subItem; } } ////// /// The index in the ListView of the parent item. /// public int ItemIndex { get { return itemIndex; } } ////// /// The column index of this sub-item. /// public int ColumnIndex { get { return columnIndex; } } ////// /// The header of this sub-item's column /// public ColumnHeader Header { get { return header; } } ////// /// Miscellaneous state information pertaining to the parent item. /// public ListViewItemStates ItemState { get { return itemState; } } ////// /// Draws the sub-item's background. /// public void DrawBackground() { Color backColor = (itemIndex == -1) ? item.BackColor : subItem.BackColor; using (Brush backBrush = new SolidBrush(backColor)) { Graphics.FillRectangle(backBrush, bounds); } } ////// /// Draws a focus rectangle in the given bounds, if the item has focus. /// public void DrawFocusRectangle(Rectangle bounds) { if((itemState & ListViewItemStates.Focused) == ListViewItemStates.Focused) { ControlPaint.DrawFocusRectangle(graphics, Rectangle.Inflate(bounds, -1, -1), item.ForeColor, item.BackColor); } } ////// /// Draws the sub-item's text (overloaded) /// public void DrawText() { // Map the ColumnHeader::TextAlign to the TextFormatFlags. HorizontalAlignment hAlign = header.TextAlign; TextFormatFlags flags = (hAlign == HorizontalAlignment.Left) ? TextFormatFlags.Left : ((hAlign == HorizontalAlignment.Center) ? TextFormatFlags.HorizontalCenter : TextFormatFlags.Right); flags |= TextFormatFlags.WordEllipsis; DrawText(flags); } ////// /// Draws the sub-item's text (overloaded) - takes a TextFormatFlags argument. /// [ SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters") // We want to measure the size of blank space. // So we don't have to localize it. ] public void DrawText(TextFormatFlags flags) { string text = (itemIndex == -1) ? item.Text : subItem.Text; Font font = (itemIndex == -1) ? item.Font : subItem.Font; Color color = (itemIndex == -1) ? item.ForeColor : subItem.ForeColor; int padding = TextRenderer.MeasureText(" ", font).Width; Rectangle newBounds = Rectangle.Inflate(bounds, -padding, 0); TextRenderer.DrawText(graphics, text, font, newBounds, color, flags); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
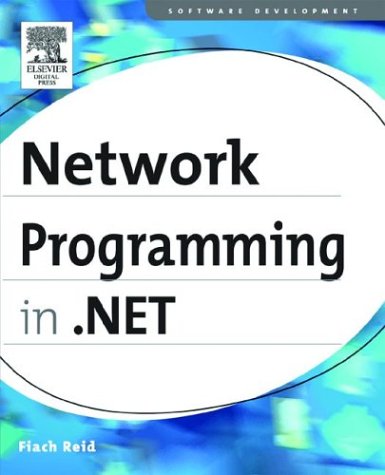
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BindingExpressionBase.cs
- Int32RectValueSerializer.cs
- MD5CryptoServiceProvider.cs
- Pen.cs
- InternalConfigEventArgs.cs
- MultiPropertyDescriptorGridEntry.cs
- XamlRtfConverter.cs
- TextBoxBase.cs
- ConnectionStringSettings.cs
- CopyCodeAction.cs
- Stream.cs
- TraceSource.cs
- Assert.cs
- EtwTrace.cs
- LineGeometry.cs
- Row.cs
- AttributeSetAction.cs
- Queue.cs
- CapabilitiesPattern.cs
- KeyedHashAlgorithm.cs
- DataBindingExpressionBuilder.cs
- Quad.cs
- webeventbuffer.cs
- CodeAccessSecurityEngine.cs
- GestureRecognizer.cs
- BaseCAMarshaler.cs
- SqlNotificationRequest.cs
- EntityContainerEntitySet.cs
- XPathCompileException.cs
- DataServiceContext.cs
- RadialGradientBrush.cs
- XmlConvert.cs
- CodeTypeReferenceExpression.cs
- QilTypeChecker.cs
- RouteValueDictionary.cs
- GeneralTransform2DTo3D.cs
- TimeSpanValidator.cs
- ServiceInfoCollection.cs
- XmlResolver.cs
- SerializationAttributes.cs
- GroupJoinQueryOperator.cs
- cookiecollection.cs
- SqlCommand.cs
- Control.cs
- ConfigurationStrings.cs
- DrawingBrush.cs
- AdornedElementPlaceholder.cs
- CompressedStack.cs
- InstalledFontCollection.cs
- _ListenerAsyncResult.cs
- Visual3DCollection.cs
- CodeTypeReferenceExpression.cs
- SingleResultAttribute.cs
- MultiByteCodec.cs
- XmlEnumAttribute.cs
- COAUTHINFO.cs
- BatchServiceHost.cs
- DataServiceOperationContext.cs
- ProcessModelSection.cs
- AppModelKnownContentFactory.cs
- BinaryUtilClasses.cs
- CTreeGenerator.cs
- FrameworkContentElement.cs
- VisualStyleTypesAndProperties.cs
- Trace.cs
- webeventbuffer.cs
- XhtmlConformanceSection.cs
- List.cs
- MaterialCollection.cs
- AssemblySettingAttributes.cs
- XPathArrayIterator.cs
- LinkClickEvent.cs
- UInt16Storage.cs
- WindowsListView.cs
- handlecollector.cs
- DynamicObjectAccessor.cs
- UmAlQuraCalendar.cs
- TextBox.cs
- CodeObjectCreateExpression.cs
- LinkButton.cs
- OdbcConnectionPoolProviderInfo.cs
- AutomationPatternInfo.cs
- WebPageTraceListener.cs
- TextParagraphView.cs
- TableLayout.cs
- ISO2022Encoding.cs
- ZipIOLocalFileDataDescriptor.cs
- RbTree.cs
- ExclusiveCanonicalizationTransform.cs
- TreeNodeBinding.cs
- WaitHandle.cs
- GridViewItemAutomationPeer.cs
- TemplatedAdorner.cs
- EventRouteFactory.cs
- ColumnResult.cs
- ListViewItem.cs
- FontConverter.cs
- XmlQueryCardinality.cs
- ZipIOLocalFileDataDescriptor.cs
- StrokeCollectionDefaultValueFactory.cs