Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / documents / ColumnResult.cs / 1 / ColumnResult.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: The ColumnResult class provides access to layout-calculated // information for a column. // // History: // 05/20/2003 : grzegorz - Moving from Avalon branch. // 06/25/2004 : grzegorz - Performance work. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Documents; using MS.Internal.PtsHost; using MS.Internal.PtsHost.UnsafeNativeMethods; using MS.Internal.Text; namespace MS.Internal.Documents { ////// The ColumnResult class provides access to layout-calculated /// information for a column. /// internal sealed class ColumnResult { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Constructor. /// /// Document page that owns the column. /// PTS track description. /// Content's offset. ////// Critical - as this can set the Critical variable _columnHandle to an arbitrary value. /// [SecurityCritical] internal ColumnResult(FlowDocumentPage page, ref PTS.FSTRACKDESCRIPTION trackDesc, Vector contentOffset) { _page = page; _columnHandle = trackDesc.pfstrack; _layoutBox = new Rect( TextDpi.FromTextDpi(trackDesc.fsrc.u), TextDpi.FromTextDpi(trackDesc.fsrc.v), TextDpi.FromTextDpi(trackDesc.fsrc.du), TextDpi.FromTextDpi(trackDesc.fsrc.dv)); _layoutBox.X += contentOffset.X; _layoutBox.Y += contentOffset.Y; _columnOffset = new Vector(TextDpi.FromTextDpi(trackDesc.fsrc.u), TextDpi.FromTextDpi(trackDesc.fsrc.v)); _hasTextContent = false; } ////// Constructor. /// /// Subpage that owns the column. /// PTS track description. /// Content's offset. ////// Critical - as this can set the Critical variable _columnHandle to an arbitrary value. /// [SecurityCritical] internal ColumnResult(BaseParaClient subpage, ref PTS.FSTRACKDESCRIPTION trackDesc, Vector contentOffset) { // Subpage must be figure, floater or subpage paraclient Invariant.Assert(subpage is SubpageParaClient || subpage is FigureParaClient || subpage is FloaterParaClient); _subpage = subpage; _columnHandle = trackDesc.pfstrack; _layoutBox = new Rect( TextDpi.FromTextDpi(trackDesc.fsrc.u), TextDpi.FromTextDpi(trackDesc.fsrc.v), TextDpi.FromTextDpi(trackDesc.fsrc.du), TextDpi.FromTextDpi(trackDesc.fsrc.dv)); _layoutBox.X += contentOffset.X; _layoutBox.Y += contentOffset.Y; _columnOffset = new Vector(TextDpi.FromTextDpi(trackDesc.fsrc.u), TextDpi.FromTextDpi(trackDesc.fsrc.v)); } #endregion Constructors //-------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods ////// Returns whether the position is contained in this column. /// /// A position to test. /// Apply strict validation rules. ////// True if column contains specified text position. /// Otherwise returns false. /// internal bool Contains(ITextPointer position, bool strict) { EnsureTextContentRange(); return _contentRange.Contains(position, strict); } #endregion Internal Methods //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- #region Internal Properties ////// Represents the beginning of the column’s contents. /// internal ITextPointer StartPosition { get { EnsureTextContentRange(); return _contentRange.StartPosition; } } ////// Represents the end of the column’s contents. /// internal ITextPointer EndPosition { get { EnsureTextContentRange(); return _contentRange.EndPosition; } } ////// The bounding rectangle of the column; this is relative to the /// parent bounding box. /// internal Rect LayoutBox { get { return _layoutBox; } } ////// Collection of ParagraphResults for the column’s contents. /// ////// Critical - as this calls Critical function GetParagraphResultsFromColumn. /// Safe - as the IntPtr _columnHandle is Critical for setting the value. /// internal ReadOnlyCollectionParagraphs { [SecurityCritical, SecurityTreatAsSafe] get { if (_paragraphs == null) { // Set _hasTextContent to true when getting paragraph collections if any paragraph has text content. _hasTextContent = false; if (_page != null) { _paragraphs = _page.GetParagraphResultsFromColumn(_columnHandle, _columnOffset, out _hasTextContent); } else { if (_subpage is FigureParaClient) { _paragraphs = ((FigureParaClient)_subpage).GetParagraphResultsFromColumn(_columnHandle, _columnOffset, out _hasTextContent); } else if (_subpage is FloaterParaClient) { _paragraphs = ((FloaterParaClient)_subpage).GetParagraphResultsFromColumn(_columnHandle, _columnOffset, out _hasTextContent); } else if (_subpage is SubpageParaClient) { _paragraphs = ((SubpageParaClient)_subpage).GetParagraphResultsFromColumn(_columnHandle, _columnOffset, out _hasTextContent); } else { Invariant.Assert(false, "Expecting Subpage, Figure or Floater ParaClient"); } } Debug.Assert(_paragraphs != null && _paragraphs.Count > 0); } return _paragraphs; } } /// /// Returns true if the column has any text content. This is determined by checking if any paragraph in the paragraphs collection /// has text content. A paragraph has text content if it includes some text characters besides figures and floaters. An EOP character is /// considered text content if it is the only character in the paragraph, but a paragraph that has only /// figures, floaters, EOP and no text has no text content. /// internal bool HasTextContent { get { if (_paragraphs == null) { // Creating paragraph results will query the page/subpage about text content in the paragrph collection and // set _hasTextContent appropriately ReadOnlyCollectionparagraphs = Paragraphs; } return _hasTextContent; } } /// /// Represents the column’s contents. /// internal TextContentRange TextContentRange { get { EnsureTextContentRange(); return _contentRange; } } #endregion Internal Properties //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods ////// Retrive TextContentRange if necessary. /// ////// Critical - as this calls Critical function GetTextContentRangeFromColumn. /// Safe - as the IntPtr _columnHandle is Critical for setting the value. /// [SecurityCritical, SecurityTreatAsSafe] private void EnsureTextContentRange() { if (_contentRange == null) { if (_page != null) { _contentRange = _page.GetTextContentRangeFromColumn(_columnHandle); } else { if (_subpage is FigureParaClient) { _contentRange = ((FigureParaClient)_subpage).GetTextContentRangeFromColumn(_columnHandle); } else if (_subpage is FloaterParaClient) { _contentRange = ((FloaterParaClient)_subpage).GetTextContentRangeFromColumn(_columnHandle); } else if (_subpage is SubpageParaClient) { _contentRange = ((SubpageParaClient)_subpage).GetTextContentRangeFromColumn(_columnHandle); } else { Invariant.Assert(false, "Expecting Subpage, Figure or Floater ParaClient"); } } Invariant.Assert(_contentRange != null); } } #endregion Private Methods //------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------- #region Private Fields ////// Document page that owns the column. /// private readonly FlowDocumentPage _page; ////// Subpage that owns the column. /// private readonly BaseParaClient _subpage; ////// Column handle (PTS track handle). /// ////// This member should be Critical for set as this gets passed on to unmanaged /// PTS functions via Paragraphs property. Since this is readonly, just marking /// the constructor Critical is good enough. This is marked as SecurityCritical/ /// SecurityTreatAsSafe. If ever we remove the readonly tag, we should make this /// a SecurityCriticalDataForSet object. /// [SecurityCritical, SecurityTreatAsSafe] private readonly IntPtr _columnHandle; ////// Layout rectangle of the column. /// private readonly Rect _layoutBox; ////// Offset of the column from the top of PTS page. /// private readonly Vector _columnOffset; ////// TextContentRanges representing the column's contents. /// private TextContentRange _contentRange; ////// The collection of ParagraphResults for the column's paragraphs. /// private ReadOnlyCollection_paragraphs; /// /// True if any of the column's paragraphs results has text content /// private bool _hasTextContent; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: The ColumnResult class provides access to layout-calculated // information for a column. // // History: // 05/20/2003 : grzegorz - Moving from Avalon branch. // 06/25/2004 : grzegorz - Performance work. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Documents; using MS.Internal.PtsHost; using MS.Internal.PtsHost.UnsafeNativeMethods; using MS.Internal.Text; namespace MS.Internal.Documents { ////// The ColumnResult class provides access to layout-calculated /// information for a column. /// internal sealed class ColumnResult { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Constructor. /// /// Document page that owns the column. /// PTS track description. /// Content's offset. ////// Critical - as this can set the Critical variable _columnHandle to an arbitrary value. /// [SecurityCritical] internal ColumnResult(FlowDocumentPage page, ref PTS.FSTRACKDESCRIPTION trackDesc, Vector contentOffset) { _page = page; _columnHandle = trackDesc.pfstrack; _layoutBox = new Rect( TextDpi.FromTextDpi(trackDesc.fsrc.u), TextDpi.FromTextDpi(trackDesc.fsrc.v), TextDpi.FromTextDpi(trackDesc.fsrc.du), TextDpi.FromTextDpi(trackDesc.fsrc.dv)); _layoutBox.X += contentOffset.X; _layoutBox.Y += contentOffset.Y; _columnOffset = new Vector(TextDpi.FromTextDpi(trackDesc.fsrc.u), TextDpi.FromTextDpi(trackDesc.fsrc.v)); _hasTextContent = false; } ////// Constructor. /// /// Subpage that owns the column. /// PTS track description. /// Content's offset. ////// Critical - as this can set the Critical variable _columnHandle to an arbitrary value. /// [SecurityCritical] internal ColumnResult(BaseParaClient subpage, ref PTS.FSTRACKDESCRIPTION trackDesc, Vector contentOffset) { // Subpage must be figure, floater or subpage paraclient Invariant.Assert(subpage is SubpageParaClient || subpage is FigureParaClient || subpage is FloaterParaClient); _subpage = subpage; _columnHandle = trackDesc.pfstrack; _layoutBox = new Rect( TextDpi.FromTextDpi(trackDesc.fsrc.u), TextDpi.FromTextDpi(trackDesc.fsrc.v), TextDpi.FromTextDpi(trackDesc.fsrc.du), TextDpi.FromTextDpi(trackDesc.fsrc.dv)); _layoutBox.X += contentOffset.X; _layoutBox.Y += contentOffset.Y; _columnOffset = new Vector(TextDpi.FromTextDpi(trackDesc.fsrc.u), TextDpi.FromTextDpi(trackDesc.fsrc.v)); } #endregion Constructors //-------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods ////// Returns whether the position is contained in this column. /// /// A position to test. /// Apply strict validation rules. ////// True if column contains specified text position. /// Otherwise returns false. /// internal bool Contains(ITextPointer position, bool strict) { EnsureTextContentRange(); return _contentRange.Contains(position, strict); } #endregion Internal Methods //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- #region Internal Properties ////// Represents the beginning of the column’s contents. /// internal ITextPointer StartPosition { get { EnsureTextContentRange(); return _contentRange.StartPosition; } } ////// Represents the end of the column’s contents. /// internal ITextPointer EndPosition { get { EnsureTextContentRange(); return _contentRange.EndPosition; } } ////// The bounding rectangle of the column; this is relative to the /// parent bounding box. /// internal Rect LayoutBox { get { return _layoutBox; } } ////// Collection of ParagraphResults for the column’s contents. /// ////// Critical - as this calls Critical function GetParagraphResultsFromColumn. /// Safe - as the IntPtr _columnHandle is Critical for setting the value. /// internal ReadOnlyCollectionParagraphs { [SecurityCritical, SecurityTreatAsSafe] get { if (_paragraphs == null) { // Set _hasTextContent to true when getting paragraph collections if any paragraph has text content. _hasTextContent = false; if (_page != null) { _paragraphs = _page.GetParagraphResultsFromColumn(_columnHandle, _columnOffset, out _hasTextContent); } else { if (_subpage is FigureParaClient) { _paragraphs = ((FigureParaClient)_subpage).GetParagraphResultsFromColumn(_columnHandle, _columnOffset, out _hasTextContent); } else if (_subpage is FloaterParaClient) { _paragraphs = ((FloaterParaClient)_subpage).GetParagraphResultsFromColumn(_columnHandle, _columnOffset, out _hasTextContent); } else if (_subpage is SubpageParaClient) { _paragraphs = ((SubpageParaClient)_subpage).GetParagraphResultsFromColumn(_columnHandle, _columnOffset, out _hasTextContent); } else { Invariant.Assert(false, "Expecting Subpage, Figure or Floater ParaClient"); } } Debug.Assert(_paragraphs != null && _paragraphs.Count > 0); } return _paragraphs; } } /// /// Returns true if the column has any text content. This is determined by checking if any paragraph in the paragraphs collection /// has text content. A paragraph has text content if it includes some text characters besides figures and floaters. An EOP character is /// considered text content if it is the only character in the paragraph, but a paragraph that has only /// figures, floaters, EOP and no text has no text content. /// internal bool HasTextContent { get { if (_paragraphs == null) { // Creating paragraph results will query the page/subpage about text content in the paragrph collection and // set _hasTextContent appropriately ReadOnlyCollectionparagraphs = Paragraphs; } return _hasTextContent; } } /// /// Represents the column’s contents. /// internal TextContentRange TextContentRange { get { EnsureTextContentRange(); return _contentRange; } } #endregion Internal Properties //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods ////// Retrive TextContentRange if necessary. /// ////// Critical - as this calls Critical function GetTextContentRangeFromColumn. /// Safe - as the IntPtr _columnHandle is Critical for setting the value. /// [SecurityCritical, SecurityTreatAsSafe] private void EnsureTextContentRange() { if (_contentRange == null) { if (_page != null) { _contentRange = _page.GetTextContentRangeFromColumn(_columnHandle); } else { if (_subpage is FigureParaClient) { _contentRange = ((FigureParaClient)_subpage).GetTextContentRangeFromColumn(_columnHandle); } else if (_subpage is FloaterParaClient) { _contentRange = ((FloaterParaClient)_subpage).GetTextContentRangeFromColumn(_columnHandle); } else if (_subpage is SubpageParaClient) { _contentRange = ((SubpageParaClient)_subpage).GetTextContentRangeFromColumn(_columnHandle); } else { Invariant.Assert(false, "Expecting Subpage, Figure or Floater ParaClient"); } } Invariant.Assert(_contentRange != null); } } #endregion Private Methods //------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------- #region Private Fields ////// Document page that owns the column. /// private readonly FlowDocumentPage _page; ////// Subpage that owns the column. /// private readonly BaseParaClient _subpage; ////// Column handle (PTS track handle). /// ////// This member should be Critical for set as this gets passed on to unmanaged /// PTS functions via Paragraphs property. Since this is readonly, just marking /// the constructor Critical is good enough. This is marked as SecurityCritical/ /// SecurityTreatAsSafe. If ever we remove the readonly tag, we should make this /// a SecurityCriticalDataForSet object. /// [SecurityCritical, SecurityTreatAsSafe] private readonly IntPtr _columnHandle; ////// Layout rectangle of the column. /// private readonly Rect _layoutBox; ////// Offset of the column from the top of PTS page. /// private readonly Vector _columnOffset; ////// TextContentRanges representing the column's contents. /// private TextContentRange _contentRange; ////// The collection of ParagraphResults for the column's paragraphs. /// private ReadOnlyCollection_paragraphs; /// /// True if any of the column's paragraphs results has text content /// private bool _hasTextContent; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
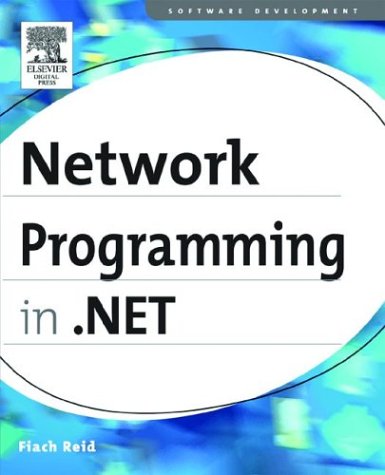
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Parser.cs
- SettingsAttributeDictionary.cs
- XomlCompilerParameters.cs
- SqlWorkflowPersistenceService.cs
- TabControlCancelEvent.cs
- TextRangeEditTables.cs
- ModelItemDictionary.cs
- XmlJsonReader.cs
- Tuple.cs
- ModifierKeysValueSerializer.cs
- BeginStoryboard.cs
- ChtmlTextBoxAdapter.cs
- CannotUnloadAppDomainException.cs
- RectValueSerializer.cs
- UiaCoreProviderApi.cs
- HttpListenerPrefixCollection.cs
- BaseProcessor.cs
- mediaeventargs.cs
- BooleanExpr.cs
- KeyBinding.cs
- ServicePointManagerElement.cs
- DataPagerFieldCollection.cs
- DataViewManagerListItemTypeDescriptor.cs
- ParseHttpDate.cs
- COM2PropertyPageUITypeConverter.cs
- LabelAutomationPeer.cs
- WebServiceErrorEvent.cs
- SafeEventLogWriteHandle.cs
- UnsignedPublishLicense.cs
- CopyNodeSetAction.cs
- ActivityStateQuery.cs
- xamlnodes.cs
- LazyTextWriterCreator.cs
- DataGridTable.cs
- LinearGradientBrush.cs
- OdbcHandle.cs
- CompilationPass2TaskInternal.cs
- ImageIndexConverter.cs
- Label.cs
- MouseBinding.cs
- AccessDataSourceView.cs
- XmlSecureResolver.cs
- FileDialogCustomPlacesCollection.cs
- XMLDiffLoader.cs
- XmlUrlResolver.cs
- DesignParameter.cs
- Clause.cs
- PriorityRange.cs
- ImageAnimator.cs
- WCFBuildProvider.cs
- CustomAttributeFormatException.cs
- httpserverutility.cs
- CacheRequest.cs
- InputLanguageManager.cs
- XmlComplianceUtil.cs
- BitmapEffect.cs
- WebPartChrome.cs
- NonPrimarySelectionGlyph.cs
- HttpListenerResponse.cs
- StrongNamePublicKeyBlob.cs
- DrawingState.cs
- AsmxEndpointPickerExtension.cs
- ContextStack.cs
- SchemaTypeEmitter.cs
- XmlSchemaSet.cs
- SchemaTypeEmitter.cs
- SemaphoreSecurity.cs
- ISessionStateStore.cs
- TableProviderWrapper.cs
- DetailsViewDeletedEventArgs.cs
- HexParser.cs
- HyperlinkAutomationPeer.cs
- HuffModule.cs
- FileUtil.cs
- OutputWindow.cs
- KeyGesture.cs
- SystemInformation.cs
- SqlDataSourceView.cs
- ServiceBehaviorElementCollection.cs
- RequestValidator.cs
- COAUTHIDENTITY.cs
- PersistencePipeline.cs
- TTSEvent.cs
- MappingItemCollection.cs
- Hash.cs
- dsa.cs
- ModelItemExtensions.cs
- ExpressionEditorAttribute.cs
- Attributes.cs
- SystemIPv6InterfaceProperties.cs
- NonSerializedAttribute.cs
- TrustLevelCollection.cs
- UserValidatedEventArgs.cs
- SudsWriter.cs
- XmlILModule.cs
- InputManager.cs
- ExpressionBuilder.cs
- DBSqlParser.cs
- CompoundFileStorageReference.cs
- Translator.cs