Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridTableCollection.cs / 1 / DataGridTableCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Collections; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; ////// /// [ListBindable(false)] public class GridTableStylesCollection : BaseCollection ,IList { CollectionChangeEventHandler onCollectionChanged; ArrayList items = new ArrayList(); DataGrid owner = null; ///Represents a collection of ///objects in the /// control. /// int IList.Add(object value) { return this.Add((DataGridTableStyle) value); } /// /// void IList.Clear() { this.Clear(); } /// /// bool IList.Contains(object value) { return items.Contains(value); } /// /// int IList.IndexOf(object value) { return items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { throw new NotSupportedException(); } /// /// void IList.Remove(object value) { this.Remove((DataGridTableStyle)value); } /// /// void IList.RemoveAt(int index) { this.RemoveAt(index); } /// /// bool IList.IsFixedSize { get {return false;} } /// /// bool IList.IsReadOnly { get {return false;} } /// /// object IList.this[int index] { get { return items[index]; } set { throw new NotSupportedException(); } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get {return this.items.Count;} } /// /// bool ICollection.IsSynchronized { get {return false;} } /// /// object ICollection.SyncRoot { get {return this;} } /// /// IEnumerator IEnumerable.GetEnumerator() { return items.GetEnumerator(); } internal GridTableStylesCollection(DataGrid grid) { owner = grid; } /// /// /// protected override ArrayList List { get { return items; } } /* implemented in BaseCollection ///[To be supplied.] ////// Retrieves the number of GridTables in the collection. /// ////// The number of GridTables in the collection. /// public override int Count { get { return items.Count; } } */ ////// /// Retrieves the DataGridTable with the specified index. /// public DataGridTableStyle this[int index] { get { return (DataGridTableStyle)items[index]; } } ////// /// Retrieves the DataGridTable with the name provided. /// public DataGridTableStyle this[string tableName] { get { if (tableName == null) throw new ArgumentNullException("tableName"); int itemCount = items.Count; for (int i = 0; i < itemCount; ++i) { DataGridTableStyle table = (DataGridTableStyle)items[i]; // NOTE: case-insensitive if (String.Equals(table.MappingName, tableName, StringComparison.OrdinalIgnoreCase)) return table; } return null; } } internal void CheckForMappingNameDuplicates(DataGridTableStyle table) { if (String.IsNullOrEmpty(table.MappingName)) return; for (int i = 0; i < items.Count; i++) if ( ((DataGridTableStyle)items[i]).MappingName.Equals(table.MappingName) && table != items[i]) throw new ArgumentException(SR.GetString(SR.DataGridTableStyleDuplicateMappingName), "table"); } ////// /// public virtual int Add(DataGridTableStyle table) { // set the rowHeaderWidth on the newly added table to at least the minimum value // on its owner if (this.owner != null && this.owner.MinimumRowHeaderWidth() > table.RowHeaderWidth) table.RowHeaderWidth = this.owner.MinimumRowHeaderWidth(); if (table.DataGrid != owner && table.DataGrid != null) throw new ArgumentException(SR.GetString(SR.DataGridTableStyleCollectionAddedParentedTableStyle), "table"); table.DataGrid = owner; CheckForMappingNameDuplicates(table); table.MappingNameChanged += new EventHandler(TableStyleMappingNameChanged); int index = items.Add(table); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, table)); return index; } private void TableStyleMappingNameChanged(object sender, EventArgs pcea) { OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///Adds a ///to this collection. /// /// public virtual void AddRange(DataGridTableStyle[] tables) { if (tables == null) { throw new ArgumentNullException("tables"); } foreach(DataGridTableStyle table in tables) { table.DataGrid = owner; table.MappingNameChanged += new EventHandler(TableStyleMappingNameChanged); items.Add(table); } OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///[To be supplied.] ////// /// public event CollectionChangeEventHandler CollectionChanged { add { onCollectionChanged += value; } remove { onCollectionChanged -= value; } } ///[To be supplied.] ////// /// public void Clear() { for (int i = 0; i < items.Count; i++) { DataGridTableStyle element = (DataGridTableStyle)items[i]; element.MappingNameChanged -= new EventHandler(TableStyleMappingNameChanged); } items.Clear(); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///[To be supplied.] ////// /// Checks to see if a DataGridTableStyle is contained in this collection. /// public bool Contains(DataGridTableStyle table) { int index = items.IndexOf(table); return index != -1; } ////// /// public bool Contains(string name) { int itemCount = items.Count; for (int i = 0; i < itemCount; ++i) { DataGridTableStyle table = (DataGridTableStyle)items[i]; // NOTE: case-insensitive if (String.Compare(table.MappingName, name, true, CultureInfo.InvariantCulture) == 0) return true; } return false; } /* public override IEnumerator GetEnumerator() { return items.GetEnumerator(); } public override IEnumerator GetEnumerator(bool allowRemove) { if (!allowRemove) return GetEnumerator(); else throw new NotSupportedException(SR.GetString(SR.DataGridTableCollectionGetEnumerator)); } */ ///Checks to see if a ///with the given name /// is contained in this collection. /// /// protected void OnCollectionChanged(CollectionChangeEventArgs e) { if (onCollectionChanged != null) onCollectionChanged(this, e); DataGrid grid = owner; if (grid != null) { /* FOR DEMO: [....]: TableStylesCollection::OnCollectionChanged: set the datagridtble DataView dataView = ((DataView) grid.DataSource); if (dataView != null) { DataTable dataTable = dataView.Table; if (dataTable != null) { if (Contains(dataTable)) { grid.SetDataGridTable(this[dataTable]); } } } */ grid.checkHierarchy = true; } } ///[To be supplied.] ////// /// public void Remove(DataGridTableStyle table) { int tableIndex = -1; int itemsCount = items.Count; for (int i = 0; i < itemsCount; ++i) if (items[i] == table) { tableIndex = i; break; } if (tableIndex == -1) throw new ArgumentException(SR.GetString(SR.DataGridTableCollectionMissingTable), "table"); else RemoveAt(tableIndex); } ///[To be supplied.] ////// /// public void RemoveAt(int index) { DataGridTableStyle element = (DataGridTableStyle)items[index]; element.MappingNameChanged -= new EventHandler(TableStyleMappingNameChanged); items.RemoveAt(index); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Remove, element)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Collections; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; ////// /// [ListBindable(false)] public class GridTableStylesCollection : BaseCollection ,IList { CollectionChangeEventHandler onCollectionChanged; ArrayList items = new ArrayList(); DataGrid owner = null; ///Represents a collection of ///objects in the /// control. /// int IList.Add(object value) { return this.Add((DataGridTableStyle) value); } /// /// void IList.Clear() { this.Clear(); } /// /// bool IList.Contains(object value) { return items.Contains(value); } /// /// int IList.IndexOf(object value) { return items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { throw new NotSupportedException(); } /// /// void IList.Remove(object value) { this.Remove((DataGridTableStyle)value); } /// /// void IList.RemoveAt(int index) { this.RemoveAt(index); } /// /// bool IList.IsFixedSize { get {return false;} } /// /// bool IList.IsReadOnly { get {return false;} } /// /// object IList.this[int index] { get { return items[index]; } set { throw new NotSupportedException(); } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get {return this.items.Count;} } /// /// bool ICollection.IsSynchronized { get {return false;} } /// /// object ICollection.SyncRoot { get {return this;} } /// /// IEnumerator IEnumerable.GetEnumerator() { return items.GetEnumerator(); } internal GridTableStylesCollection(DataGrid grid) { owner = grid; } /// /// /// protected override ArrayList List { get { return items; } } /* implemented in BaseCollection ///[To be supplied.] ////// Retrieves the number of GridTables in the collection. /// ////// The number of GridTables in the collection. /// public override int Count { get { return items.Count; } } */ ////// /// Retrieves the DataGridTable with the specified index. /// public DataGridTableStyle this[int index] { get { return (DataGridTableStyle)items[index]; } } ////// /// Retrieves the DataGridTable with the name provided. /// public DataGridTableStyle this[string tableName] { get { if (tableName == null) throw new ArgumentNullException("tableName"); int itemCount = items.Count; for (int i = 0; i < itemCount; ++i) { DataGridTableStyle table = (DataGridTableStyle)items[i]; // NOTE: case-insensitive if (String.Equals(table.MappingName, tableName, StringComparison.OrdinalIgnoreCase)) return table; } return null; } } internal void CheckForMappingNameDuplicates(DataGridTableStyle table) { if (String.IsNullOrEmpty(table.MappingName)) return; for (int i = 0; i < items.Count; i++) if ( ((DataGridTableStyle)items[i]).MappingName.Equals(table.MappingName) && table != items[i]) throw new ArgumentException(SR.GetString(SR.DataGridTableStyleDuplicateMappingName), "table"); } ////// /// public virtual int Add(DataGridTableStyle table) { // set the rowHeaderWidth on the newly added table to at least the minimum value // on its owner if (this.owner != null && this.owner.MinimumRowHeaderWidth() > table.RowHeaderWidth) table.RowHeaderWidth = this.owner.MinimumRowHeaderWidth(); if (table.DataGrid != owner && table.DataGrid != null) throw new ArgumentException(SR.GetString(SR.DataGridTableStyleCollectionAddedParentedTableStyle), "table"); table.DataGrid = owner; CheckForMappingNameDuplicates(table); table.MappingNameChanged += new EventHandler(TableStyleMappingNameChanged); int index = items.Add(table); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, table)); return index; } private void TableStyleMappingNameChanged(object sender, EventArgs pcea) { OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///Adds a ///to this collection. /// /// public virtual void AddRange(DataGridTableStyle[] tables) { if (tables == null) { throw new ArgumentNullException("tables"); } foreach(DataGridTableStyle table in tables) { table.DataGrid = owner; table.MappingNameChanged += new EventHandler(TableStyleMappingNameChanged); items.Add(table); } OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///[To be supplied.] ////// /// public event CollectionChangeEventHandler CollectionChanged { add { onCollectionChanged += value; } remove { onCollectionChanged -= value; } } ///[To be supplied.] ////// /// public void Clear() { for (int i = 0; i < items.Count; i++) { DataGridTableStyle element = (DataGridTableStyle)items[i]; element.MappingNameChanged -= new EventHandler(TableStyleMappingNameChanged); } items.Clear(); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///[To be supplied.] ////// /// Checks to see if a DataGridTableStyle is contained in this collection. /// public bool Contains(DataGridTableStyle table) { int index = items.IndexOf(table); return index != -1; } ////// /// public bool Contains(string name) { int itemCount = items.Count; for (int i = 0; i < itemCount; ++i) { DataGridTableStyle table = (DataGridTableStyle)items[i]; // NOTE: case-insensitive if (String.Compare(table.MappingName, name, true, CultureInfo.InvariantCulture) == 0) return true; } return false; } /* public override IEnumerator GetEnumerator() { return items.GetEnumerator(); } public override IEnumerator GetEnumerator(bool allowRemove) { if (!allowRemove) return GetEnumerator(); else throw new NotSupportedException(SR.GetString(SR.DataGridTableCollectionGetEnumerator)); } */ ///Checks to see if a ///with the given name /// is contained in this collection. /// /// protected void OnCollectionChanged(CollectionChangeEventArgs e) { if (onCollectionChanged != null) onCollectionChanged(this, e); DataGrid grid = owner; if (grid != null) { /* FOR DEMO: [....]: TableStylesCollection::OnCollectionChanged: set the datagridtble DataView dataView = ((DataView) grid.DataSource); if (dataView != null) { DataTable dataTable = dataView.Table; if (dataTable != null) { if (Contains(dataTable)) { grid.SetDataGridTable(this[dataTable]); } } } */ grid.checkHierarchy = true; } } ///[To be supplied.] ////// /// public void Remove(DataGridTableStyle table) { int tableIndex = -1; int itemsCount = items.Count; for (int i = 0; i < itemsCount; ++i) if (items[i] == table) { tableIndex = i; break; } if (tableIndex == -1) throw new ArgumentException(SR.GetString(SR.DataGridTableCollectionMissingTable), "table"); else RemoveAt(tableIndex); } ///[To be supplied.] ////// /// public void RemoveAt(int index) { DataGridTableStyle element = (DataGridTableStyle)items[index]; element.MappingNameChanged -= new EventHandler(TableStyleMappingNameChanged); items.RemoveAt(index); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Remove, element)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
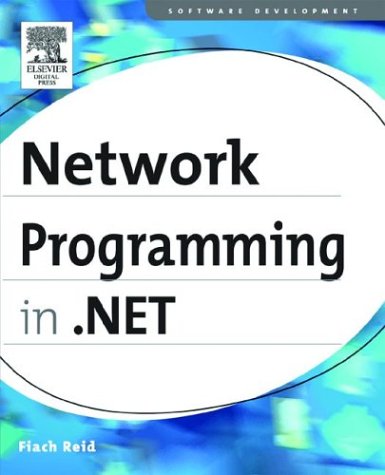
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridTextBox.cs
- SelectionRangeConverter.cs
- CriticalHandle.cs
- SQLMoney.cs
- KeyGestureValueSerializer.cs
- _SSPIWrapper.cs
- TypeConverterAttribute.cs
- QueryInterceptorAttribute.cs
- ListItemParagraph.cs
- DataSourceNameHandler.cs
- HandleInitializationContext.cs
- DependentList.cs
- XmlSchemaSimpleType.cs
- IISUnsafeMethods.cs
- AssemblyNameProxy.cs
- DataGridViewCellFormattingEventArgs.cs
- FunctionCommandText.cs
- Metafile.cs
- CalendarDayButton.cs
- VariableAction.cs
- WebSysDefaultValueAttribute.cs
- ChildTable.cs
- smtpconnection.cs
- CharAnimationUsingKeyFrames.cs
- SQLInt16Storage.cs
- ReferentialConstraintRoleElement.cs
- SqlDataAdapter.cs
- SelectedCellsChangedEventArgs.cs
- DefaultHttpHandler.cs
- WebPartDisplayModeCancelEventArgs.cs
- PasswordPropertyTextAttribute.cs
- StyleXamlParser.cs
- DataList.cs
- OracleParameterCollection.cs
- SingleQueryOperator.cs
- RoleService.cs
- IChannel.cs
- SEHException.cs
- PhysicalOps.cs
- XmlnsPrefixAttribute.cs
- FieldDescriptor.cs
- DataGridViewAdvancedBorderStyle.cs
- StringStorage.cs
- Int32AnimationBase.cs
- CallbackValidatorAttribute.cs
- FunctionParameter.cs
- JournalEntryStack.cs
- XsdBuilder.cs
- HostProtectionException.cs
- KeyConstraint.cs
- ParameterReplacerVisitor.cs
- DetailsViewModeEventArgs.cs
- StatusCommandUI.cs
- ElementUtil.cs
- OleCmdHelper.cs
- RefType.cs
- SourceElementsCollection.cs
- OdbcConnectionFactory.cs
- XmlDataSourceNodeDescriptor.cs
- XmlTypeMapping.cs
- ExtendedProperty.cs
- elementinformation.cs
- ValueUtilsSmi.cs
- XPathScanner.cs
- HttpAsyncResult.cs
- EntityChangedParams.cs
- ContextMarshalException.cs
- ToolStripStatusLabel.cs
- SpecialFolderEnumConverter.cs
- SrgsElementList.cs
- NameValuePair.cs
- DataBoundControlAdapter.cs
- InvalidFilterCriteriaException.cs
- GcHandle.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- Int16KeyFrameCollection.cs
- Point.cs
- CodeEntryPointMethod.cs
- EntityObject.cs
- webeventbuffer.cs
- EndPoint.cs
- XPathSingletonIterator.cs
- ExitEventArgs.cs
- FontSourceCollection.cs
- CustomAttributeFormatException.cs
- HttpCookie.cs
- TreeIterators.cs
- XmlBinaryReader.cs
- SingleAnimationBase.cs
- EventPrivateKey.cs
- RowVisual.cs
- PreservationFileReader.cs
- AsnEncodedData.cs
- StandardToolWindows.cs
- WebPartCancelEventArgs.cs
- Graphics.cs
- BamlLocalizer.cs
- ByteStreamGeometryContext.cs
- Int32RectConverter.cs
- ToolStripSettings.cs