Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / XamlRtfConverter.cs / 1 / XamlRtfConverter.cs
//---------------------------------------------------------------------------- // // File: XamlRtfConverter.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Xaml-Rtf Converter. // //--------------------------------------------------------------------------- using System.IO; using System.Text; namespace System.Windows.Documents { ////// XamlRtfConverter is a static class that convert from/to rtf content to/from xaml content. /// internal class XamlRtfConverter { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// create new instance of XamlRtfConverter that convert the content between xaml and rtf. /// internal XamlRtfConverter() { } #endregion Constructors // ---------------------------------------------------------------------- // // Internal Methods // // --------------------------------------------------------------------- #region Internal Methods ////// Converts an xaml content to rtf content. /// /// /// The source xaml text content to be converted into Rtf content. /// ////// Well-formed representing rtf equivalent string for the source xaml content. /// internal string ConvertXamlToRtf(string xamlContent) { // Check the parameter validation if (xamlContent == null) { throw new ArgumentNullException("xamlContent"); } string rtfContent = string.Empty; if (xamlContent != string.Empty) { // Creating the converter that process the content data from Xaml to Rtf XamlToRtfWriter xamlToRtfWriter = new XamlToRtfWriter(xamlContent); // Set WpfPayload package that contained the image for the specified Xaml if (WpfPayload != null) { xamlToRtfWriter.WpfPayload = WpfPayload; } // Process the converting from xaml to rtf xamlToRtfWriter.Process(); // Set rtf content that representing resulting from Xaml to Rtf converting. rtfContent = xamlToRtfWriter.Output; } return rtfContent; } ////// Converts an rtf content to xaml content. /// /// /// The source rtf content that to be converted into xaml content. /// ////// Well-formed xml representing XAML equivalent content for the input rtf content string. /// internal string ConvertRtfToXaml(string rtfContent) { // Check the parameter validation if (rtfContent == null) { throw new ArgumentNullException("rtfContent"); } // xaml content to be converted from rtf string xamlContent = string.Empty; if (rtfContent != string.Empty) { // Create RtfToXamlReader instance for converting the content // from rtf to xaml and set ForceParagraph RtfToXamlReader rtfToXamlReader = new RtfToXamlReader(rtfContent); rtfToXamlReader.ForceParagraph = ForceParagraph; // Set WpfPayload package that contained the image for the specified Xaml if (WpfPayload != null) { rtfToXamlReader.WpfPayload = WpfPayload; } //Process the converting from rtf to xaml rtfToXamlReader.Process(); // Set Xaml content string that representing resulting Rtf-Xaml converting xamlContent = rtfToXamlReader.Output; } return xamlContent; } #endregion Internal Methods // ---------------------------------------------------------------------- // // Internal Properties // // ---------------------------------------------------------------------- #region Internal Properties // ForceParagraph property indicates whether ForcePagraph for RtfToXamlReader. internal bool ForceParagraph { get { return _forceParagraph; } set { _forceParagraph = value; } } // WpfPayload package property for getting or placing image data for Xaml content internal WpfPayload WpfPayload { get { return _wpfPayload; } set { _wpfPayload = value; } } #endregion Internal Properties // --------------------------------------------------------------------- // // Internal Fields // // ---------------------------------------------------------------------- #region Internal Fields // Rtf encoding codepage that is 1252 ANSI internal const int RtfCodePage = 1252; #endregion Internal Fields // --------------------------------------------------------------------- // // Private Fields // // --------------------------------------------------------------------- #region Private Fields // Flag that indicate the forcing paragragh for RtfToXamlReader private bool _forceParagraph; // The output WpfPayload package for placing image data into it private WpfPayload _wpfPayload; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: XamlRtfConverter.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Xaml-Rtf Converter. // //--------------------------------------------------------------------------- using System.IO; using System.Text; namespace System.Windows.Documents { ////// XamlRtfConverter is a static class that convert from/to rtf content to/from xaml content. /// internal class XamlRtfConverter { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// create new instance of XamlRtfConverter that convert the content between xaml and rtf. /// internal XamlRtfConverter() { } #endregion Constructors // ---------------------------------------------------------------------- // // Internal Methods // // --------------------------------------------------------------------- #region Internal Methods ////// Converts an xaml content to rtf content. /// /// /// The source xaml text content to be converted into Rtf content. /// ////// Well-formed representing rtf equivalent string for the source xaml content. /// internal string ConvertXamlToRtf(string xamlContent) { // Check the parameter validation if (xamlContent == null) { throw new ArgumentNullException("xamlContent"); } string rtfContent = string.Empty; if (xamlContent != string.Empty) { // Creating the converter that process the content data from Xaml to Rtf XamlToRtfWriter xamlToRtfWriter = new XamlToRtfWriter(xamlContent); // Set WpfPayload package that contained the image for the specified Xaml if (WpfPayload != null) { xamlToRtfWriter.WpfPayload = WpfPayload; } // Process the converting from xaml to rtf xamlToRtfWriter.Process(); // Set rtf content that representing resulting from Xaml to Rtf converting. rtfContent = xamlToRtfWriter.Output; } return rtfContent; } ////// Converts an rtf content to xaml content. /// /// /// The source rtf content that to be converted into xaml content. /// ////// Well-formed xml representing XAML equivalent content for the input rtf content string. /// internal string ConvertRtfToXaml(string rtfContent) { // Check the parameter validation if (rtfContent == null) { throw new ArgumentNullException("rtfContent"); } // xaml content to be converted from rtf string xamlContent = string.Empty; if (rtfContent != string.Empty) { // Create RtfToXamlReader instance for converting the content // from rtf to xaml and set ForceParagraph RtfToXamlReader rtfToXamlReader = new RtfToXamlReader(rtfContent); rtfToXamlReader.ForceParagraph = ForceParagraph; // Set WpfPayload package that contained the image for the specified Xaml if (WpfPayload != null) { rtfToXamlReader.WpfPayload = WpfPayload; } //Process the converting from rtf to xaml rtfToXamlReader.Process(); // Set Xaml content string that representing resulting Rtf-Xaml converting xamlContent = rtfToXamlReader.Output; } return xamlContent; } #endregion Internal Methods // ---------------------------------------------------------------------- // // Internal Properties // // ---------------------------------------------------------------------- #region Internal Properties // ForceParagraph property indicates whether ForcePagraph for RtfToXamlReader. internal bool ForceParagraph { get { return _forceParagraph; } set { _forceParagraph = value; } } // WpfPayload package property for getting or placing image data for Xaml content internal WpfPayload WpfPayload { get { return _wpfPayload; } set { _wpfPayload = value; } } #endregion Internal Properties // --------------------------------------------------------------------- // // Internal Fields // // ---------------------------------------------------------------------- #region Internal Fields // Rtf encoding codepage that is 1252 ANSI internal const int RtfCodePage = 1252; #endregion Internal Fields // --------------------------------------------------------------------- // // Private Fields // // --------------------------------------------------------------------- #region Private Fields // Flag that indicate the forcing paragragh for RtfToXamlReader private bool _forceParagraph; // The output WpfPayload package for placing image data into it private WpfPayload _wpfPayload; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
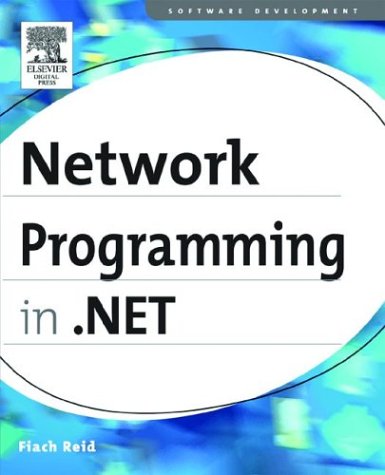
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataTablePropertyDescriptor.cs
- MatrixAnimationBase.cs
- ByteStorage.cs
- CheckPair.cs
- StylusCaptureWithinProperty.cs
- Color.cs
- ScriptHandlerFactory.cs
- RSAOAEPKeyExchangeFormatter.cs
- TypedServiceOperationListItem.cs
- BindingExpression.cs
- EntityDataSourceContextDisposingEventArgs.cs
- SystemException.cs
- Condition.cs
- ConfigurationPropertyCollection.cs
- ToolboxBitmapAttribute.cs
- XmlReturnWriter.cs
- CompositeFontParser.cs
- WebAdminConfigurationHelper.cs
- ObjectTypeMapping.cs
- AcceptorSessionSymmetricMessageSecurityProtocol.cs
- XPathNodePointer.cs
- WindowsListBox.cs
- _LazyAsyncResult.cs
- BinaryCommonClasses.cs
- BitmapEffect.cs
- SqlDataSourceEnumerator.cs
- PageThemeBuildProvider.cs
- SvcFileManager.cs
- EventProviderBase.cs
- DataAdapter.cs
- VectorAnimation.cs
- FuncCompletionCallbackWrapper.cs
- ImageIndexEditor.cs
- ComponentEditorForm.cs
- SqlUnionizer.cs
- DataKeyArray.cs
- PropertyMappingExceptionEventArgs.cs
- DataGridViewRowConverter.cs
- configsystem.cs
- FileUpload.cs
- DeclarativeCatalogPartDesigner.cs
- DependencyPropertyConverter.cs
- SoapExtensionReflector.cs
- XmlElement.cs
- RoutedPropertyChangedEventArgs.cs
- ClientWindowsAuthenticationMembershipProvider.cs
- PaperSource.cs
- SecureUICommand.cs
- DesignTimeDataBinding.cs
- BuildProviderUtils.cs
- MouseOverProperty.cs
- RadioButton.cs
- TypeBinaryExpression.cs
- TextAction.cs
- Condition.cs
- ReliabilityContractAttribute.cs
- WizardDesigner.cs
- GraphicsContext.cs
- IncrementalHitTester.cs
- RecommendedAsConfigurableAttribute.cs
- ConfigurationSection.cs
- ObjectNotFoundException.cs
- SharedPersonalizationStateInfo.cs
- DSACryptoServiceProvider.cs
- SqlProcedureAttribute.cs
- KnownTypes.cs
- RoleServiceManager.cs
- DispatcherFrame.cs
- PEFileEvidenceFactory.cs
- HttpResponse.cs
- ToolStripPanelCell.cs
- FormattedTextSymbols.cs
- JsonWriter.cs
- OrderedEnumerableRowCollection.cs
- ComEventsInfo.cs
- OdbcDataAdapter.cs
- SQLDecimalStorage.cs
- DecimalFormatter.cs
- TextOptions.cs
- Thread.cs
- ProfileEventArgs.cs
- CheckBox.cs
- EntitySqlException.cs
- Odbc32.cs
- DecimalStorage.cs
- FileSecurity.cs
- ListDictionary.cs
- OperandQuery.cs
- BitmapImage.cs
- BitmapData.cs
- BoundColumn.cs
- TextContainerChangeEventArgs.cs
- StylusPointPropertyInfo.cs
- ResourceKey.cs
- DataControlFieldTypeEditor.cs
- ReflectionTypeLoadException.cs
- DesignerAttributeInfo.cs
- LineGeometry.cs
- RegistrationServices.cs
- CodeBlockBuilder.cs