Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / NaturalLanguageHyphenator.cs / 1 / NaturalLanguageHyphenator.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: // Implementation of TextLexicalService abstract class used by TextFormatter for // document layout. This implementation is based on the hyphenation service in // NaturalLanguage6.dll - the component owned by the Natural Language Team. // // History: // 06/28/2005 : Worachai Chaoweeraprasit (Wchao) - created // //--------------------------------------------------------------------------- using System.Security; using System.Collections; using System.Globalization; using System.IO; using System.Runtime.InteropServices; using System.Windows.Media.TextFormatting; using MS.Win32; using MS.Internal; namespace System.Windows.Documents { ////// The NLG hyphenation-based implementation of TextLexicalService used by TextFormatter /// for line-breaking purpose. /// internal class NaturalLanguageHyphenator : TextLexicalService, IDisposable { ////// Critical: Holds a COM component instance that has unmanged code elevations /// [SecurityCritical] private IntPtr _hyphenatorResource; private bool _disposed; ////// Construct an NLG-based hyphenator /// ////// Critical: This code calls into NlCreateHyphenator, which elevates unmanaged code permission. /// TreatAsSafe: This function call takes no input parameters /// [SecurityCritical, SecurityTreatAsSafe] internal NaturalLanguageHyphenator() { try { _hyphenatorResource = UnsafeNativeMethods.NlCreateHyphenator(); } catch (DllNotFoundException) { } catch (EntryPointNotFoundException) { } } ////// Finalize hyphenator's unmanaged resource /// ~NaturalLanguageHyphenator() { CleanupInternal(true); } ////// Dispose hyphenator's unmanaged resource /// void IDisposable.Dispose() { GC.SuppressFinalize(this); CleanupInternal(false); } ////// Internal clean-up routine /// ////// Critical: This code calls into NlDestroyHyphenator, which elevates unmanaged code permission. /// TreatAsSafe: This function call takes no input memory block /// [SecurityCritical, SecurityTreatAsSafe] private void CleanupInternal(bool finalizing) { if (!_disposed && _hyphenatorResource != IntPtr.Zero) { UnsafeNativeMethods.NlDestroyHyphenator(ref _hyphenatorResource); _disposed = true; } } ////// TextFormatter to query whether the lexical services component could provides /// analysis for the specified culture. /// /// Culture whose text is to be analyzed ///Boolean value indicates whether the specified culture is supported public override bool IsCultureSupported(CultureInfo culture) { // Accept all cultures for the time being. Ideally NL6 should provide a way for the client // to test supported culture. return true; } ////// TextFormatter to get the lexical breaks of the specified raw text /// /// character array /// number of character in the character array to analyze /// culture of the specified character source ///lexical breaks of the text ////// Critical: This code calls NlHyphenate which is critical. /// TreatAsSafe: This code accepts a buffer that is length checked and returns /// data that is ok to return. /// [SecurityCritical, SecurityTreatAsSafe] public override TextLexicalBreaks AnalyzeText( char[] characterSource, int length, CultureInfo textCulture ) { Invariant.Assert( characterSource != null && characterSource.Length > 0 && length > 0 && length <= characterSource.Length ); if (_hyphenatorResource == IntPtr.Zero) { // No hyphenator available, no service delivered return null; } if (_disposed) { throw new ObjectDisposedException(SR.Get(SRID.HyphenatorDisposed)); } byte[] isHyphenPositions = new byte[(length + 7) / 8]; UnsafeNativeMethods.NlHyphenate( _hyphenatorResource, characterSource, length, ((textCulture != null && textCulture != CultureInfo.InvariantCulture) ? textCulture.LCID : 0), isHyphenPositions, isHyphenPositions.Length ); return new HyphenBreaks(isHyphenPositions, length); } ////// Private implementation of TextLexicalBreaks that encapsulates hyphen opportunities within /// a character string. /// private class HyphenBreaks : TextLexicalBreaks { private byte[] _isHyphenPositions; private int _numPositions; internal HyphenBreaks(byte[] isHyphenPositions, int numPositions) { _isHyphenPositions = isHyphenPositions; _numPositions = numPositions; } ////// Indexer for the value at the nth break index (bit nth of the logical bit array) /// private bool this[int index] { get { return (_isHyphenPositions[index / 8] & (1 << index % 8)) != 0; } } public override int Length { get { return _numPositions; } } public override int GetNextBreak(int currentIndex) { if (_isHyphenPositions != null && currentIndex >= 0) { int ich = currentIndex + 1; while (ich < _numPositions && !this[ich]) ich++; if (ich < _numPositions) return ich; } // return negative value when break is not found. return -1; } public override int GetPreviousBreak(int currentIndex) { if (_isHyphenPositions != null && currentIndex < _numPositions) { int ich = currentIndex; while (ich > 0 && !this[ich]) ich--; if (ich > 0) return ich; } // return negative value when break is not found. return -1; } } private static class UnsafeNativeMethods { ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(Microsoft.Internal.DllImport.PresentationNative, PreserveSig = false)] internal static extern IntPtr NlCreateHyphenator(); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(Microsoft.Internal.DllImport.PresentationNative, PreserveSig = false)] internal static extern void NlDestroyHyphenator(ref IntPtr hyphenator); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(Microsoft.Internal.DllImport.PresentationNative, PreserveSig = false)] internal static extern void NlHyphenate( IntPtr hyphenator, [In, MarshalAs(UnmanagedType.LPArray, ArraySubType = UnmanagedType.U2, SizeParamIndex = 2)] char[] inputText, int textLength, int localeID, [In, MarshalAs(UnmanagedType.LPArray, SizeParamIndex = 5)] byte[] hyphenBreaks, int numPositions ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: // Implementation of TextLexicalService abstract class used by TextFormatter for // document layout. This implementation is based on the hyphenation service in // NaturalLanguage6.dll - the component owned by the Natural Language Team. // // History: // 06/28/2005 : Worachai Chaoweeraprasit (Wchao) - created // //--------------------------------------------------------------------------- using System.Security; using System.Collections; using System.Globalization; using System.IO; using System.Runtime.InteropServices; using System.Windows.Media.TextFormatting; using MS.Win32; using MS.Internal; namespace System.Windows.Documents { ////// The NLG hyphenation-based implementation of TextLexicalService used by TextFormatter /// for line-breaking purpose. /// internal class NaturalLanguageHyphenator : TextLexicalService, IDisposable { ////// Critical: Holds a COM component instance that has unmanged code elevations /// [SecurityCritical] private IntPtr _hyphenatorResource; private bool _disposed; ////// Construct an NLG-based hyphenator /// ////// Critical: This code calls into NlCreateHyphenator, which elevates unmanaged code permission. /// TreatAsSafe: This function call takes no input parameters /// [SecurityCritical, SecurityTreatAsSafe] internal NaturalLanguageHyphenator() { try { _hyphenatorResource = UnsafeNativeMethods.NlCreateHyphenator(); } catch (DllNotFoundException) { } catch (EntryPointNotFoundException) { } } ////// Finalize hyphenator's unmanaged resource /// ~NaturalLanguageHyphenator() { CleanupInternal(true); } ////// Dispose hyphenator's unmanaged resource /// void IDisposable.Dispose() { GC.SuppressFinalize(this); CleanupInternal(false); } ////// Internal clean-up routine /// ////// Critical: This code calls into NlDestroyHyphenator, which elevates unmanaged code permission. /// TreatAsSafe: This function call takes no input memory block /// [SecurityCritical, SecurityTreatAsSafe] private void CleanupInternal(bool finalizing) { if (!_disposed && _hyphenatorResource != IntPtr.Zero) { UnsafeNativeMethods.NlDestroyHyphenator(ref _hyphenatorResource); _disposed = true; } } ////// TextFormatter to query whether the lexical services component could provides /// analysis for the specified culture. /// /// Culture whose text is to be analyzed ///Boolean value indicates whether the specified culture is supported public override bool IsCultureSupported(CultureInfo culture) { // Accept all cultures for the time being. Ideally NL6 should provide a way for the client // to test supported culture. return true; } ////// TextFormatter to get the lexical breaks of the specified raw text /// /// character array /// number of character in the character array to analyze /// culture of the specified character source ///lexical breaks of the text ////// Critical: This code calls NlHyphenate which is critical. /// TreatAsSafe: This code accepts a buffer that is length checked and returns /// data that is ok to return. /// [SecurityCritical, SecurityTreatAsSafe] public override TextLexicalBreaks AnalyzeText( char[] characterSource, int length, CultureInfo textCulture ) { Invariant.Assert( characterSource != null && characterSource.Length > 0 && length > 0 && length <= characterSource.Length ); if (_hyphenatorResource == IntPtr.Zero) { // No hyphenator available, no service delivered return null; } if (_disposed) { throw new ObjectDisposedException(SR.Get(SRID.HyphenatorDisposed)); } byte[] isHyphenPositions = new byte[(length + 7) / 8]; UnsafeNativeMethods.NlHyphenate( _hyphenatorResource, characterSource, length, ((textCulture != null && textCulture != CultureInfo.InvariantCulture) ? textCulture.LCID : 0), isHyphenPositions, isHyphenPositions.Length ); return new HyphenBreaks(isHyphenPositions, length); } ////// Private implementation of TextLexicalBreaks that encapsulates hyphen opportunities within /// a character string. /// private class HyphenBreaks : TextLexicalBreaks { private byte[] _isHyphenPositions; private int _numPositions; internal HyphenBreaks(byte[] isHyphenPositions, int numPositions) { _isHyphenPositions = isHyphenPositions; _numPositions = numPositions; } ////// Indexer for the value at the nth break index (bit nth of the logical bit array) /// private bool this[int index] { get { return (_isHyphenPositions[index / 8] & (1 << index % 8)) != 0; } } public override int Length { get { return _numPositions; } } public override int GetNextBreak(int currentIndex) { if (_isHyphenPositions != null && currentIndex >= 0) { int ich = currentIndex + 1; while (ich < _numPositions && !this[ich]) ich++; if (ich < _numPositions) return ich; } // return negative value when break is not found. return -1; } public override int GetPreviousBreak(int currentIndex) { if (_isHyphenPositions != null && currentIndex < _numPositions) { int ich = currentIndex; while (ich > 0 && !this[ich]) ich--; if (ich > 0) return ich; } // return negative value when break is not found. return -1; } } private static class UnsafeNativeMethods { ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(Microsoft.Internal.DllImport.PresentationNative, PreserveSig = false)] internal static extern IntPtr NlCreateHyphenator(); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(Microsoft.Internal.DllImport.PresentationNative, PreserveSig = false)] internal static extern void NlDestroyHyphenator(ref IntPtr hyphenator); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(Microsoft.Internal.DllImport.PresentationNative, PreserveSig = false)] internal static extern void NlHyphenate( IntPtr hyphenator, [In, MarshalAs(UnmanagedType.LPArray, ArraySubType = UnmanagedType.U2, SizeParamIndex = 2)] char[] inputText, int textLength, int localeID, [In, MarshalAs(UnmanagedType.LPArray, SizeParamIndex = 5)] byte[] hyphenBreaks, int numPositions ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
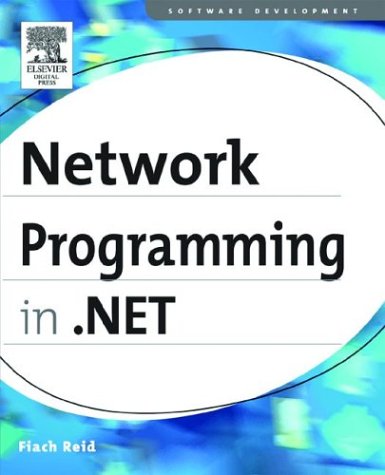
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ActionFrame.cs
- PropertyGridView.cs
- TextRangeEditTables.cs
- CodeMethodReturnStatement.cs
- EncodingTable.cs
- FontFamilyConverter.cs
- XPathEmptyIterator.cs
- DispatcherOperation.cs
- XMLUtil.cs
- SecurityPermission.cs
- URI.cs
- SystemIPInterfaceStatistics.cs
- SynchronizedDispatch.cs
- ContextMenu.cs
- StringComparer.cs
- WebConfigurationManager.cs
- GradientBrush.cs
- ByteStreamGeometryContext.cs
- AuthenticodeSignatureInformation.cs
- CodeDOMUtility.cs
- ActionFrame.cs
- FillErrorEventArgs.cs
- LayoutEditorPart.cs
- PropertyEmitterBase.cs
- OperandQuery.cs
- ResourceExpressionBuilder.cs
- MimeTypeMapper.cs
- WebPartHelpVerb.cs
- GestureRecognitionResult.cs
- XmlIlVisitor.cs
- BaseDataListActionList.cs
- SerTrace.cs
- relpropertyhelper.cs
- ResourceWriter.cs
- PersistChildrenAttribute.cs
- EncoderFallback.cs
- ConfigurationSectionHelper.cs
- DataGridViewImageColumn.cs
- PermissionSetEnumerator.cs
- DelegatedStream.cs
- __FastResourceComparer.cs
- MissingSatelliteAssemblyException.cs
- CodeMemberEvent.cs
- PolyBezierSegment.cs
- DetailsViewRow.cs
- DataRelation.cs
- UnsafeNativeMethods.cs
- SqlBulkCopyColumnMapping.cs
- PolicyManager.cs
- InstanceOwnerException.cs
- HtmlUtf8RawTextWriter.cs
- FontStretch.cs
- SchemaElementDecl.cs
- ClientConvert.cs
- NumberAction.cs
- XmlElementCollection.cs
- EllipticalNodeOperations.cs
- TableCell.cs
- EventTask.cs
- ProviderConnectionPointCollection.cs
- KeyEventArgs.cs
- ClientRuntimeConfig.cs
- AnnotationResourceCollection.cs
- DetailsViewInsertedEventArgs.cs
- MenuItemCollectionEditorDialog.cs
- TextAutomationPeer.cs
- ByteStack.cs
- TemplateControlCodeDomTreeGenerator.cs
- BamlMapTable.cs
- FlowNode.cs
- RedistVersionInfo.cs
- Normalization.cs
- ListBoxChrome.cs
- ChildDocumentBlock.cs
- QuaternionAnimation.cs
- Control.cs
- SweepDirectionValidation.cs
- BooleanFunctions.cs
- TabControl.cs
- BasePropertyDescriptor.cs
- FixedDocumentSequencePaginator.cs
- ChtmlLinkAdapter.cs
- ObjectIDGenerator.cs
- ArraySubsetEnumerator.cs
- ExportFileRequest.cs
- QualifierSet.cs
- MailSettingsSection.cs
- RowToFieldTransformer.cs
- Literal.cs
- coordinatorscratchpad.cs
- StsCommunicationException.cs
- InstanceData.cs
- CustomValidator.cs
- ListControlStringCollectionEditor.cs
- AssemblyName.cs
- wgx_sdk_version.cs
- WebDisplayNameAttribute.cs
- MouseActionConverter.cs
- RequiredAttributeAttribute.cs
- base64Transforms.cs