Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / KeyEventArgs.cs / 1305600 / KeyEventArgs.cs
using System; using System.Security; using MS.Internal; using MS.Internal.PresentationCore; // SecurityHelper namespace System.Windows.Input { ////// The KeyEventArgs class contains information about key states. /// ///public class KeyEventArgs : KeyboardEventArgs { /// /// Constructs an instance of the KeyEventArgs class. /// /// /// The logical keyboard device associated with this event. /// /// /// The input source that provided this input. /// /// /// The time when the input occured. /// /// /// The key referenced by the event. /// ////// Critical - accepts PresentationSource. /// [SecurityCritical] public KeyEventArgs(KeyboardDevice keyboard, PresentationSource inputSource, int timestamp, Key key) : base(keyboard, timestamp) { if (inputSource == null) throw new ArgumentNullException("inputSource"); if (!Keyboard.IsValidKey(key)) throw new System.ComponentModel.InvalidEnumArgumentException("key", (int)key, typeof(Key)); _inputSource = inputSource; _realKey = key; _isRepeat = false; // Start out assuming that this is just a normal key. MarkNormal(); } ////// The input source that provided this input. /// ////// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - hands out _inputSource via UnsafeInputSource call. /// PublicOK - there is a demand. /// public PresentationSource InputSource { [SecurityCritical ] get { SecurityHelper.DemandUnrestrictedUIPermission(); return UnsafeInputSource; } } ////// The Key referenced by the event, if the key is not being /// handled specially. /// public Key Key { get {return _key;} } ////// The original key, as opposed to ///, which might /// have been changed (e.g. by MarkTextInput). /// /// Note: This should remain internal. When a processor obfuscates the key, /// such as changing characters to Key.TextInput, we're deliberately trying to /// hide it and make it hard to find. But internally we'd like an easy way to find /// it. So we have this internal, but it must remain internal. /// internal Key RealKey { get { return _realKey; } } ////// The Key referenced by the event, if the key is going to be /// processed by an IME. /// public Key ImeProcessedKey { get { return (_key == Key.ImeProcessed) ? _realKey : Key.None;} } ////// The Key referenced by the event, if the key is going to be /// processed by an system. /// public Key SystemKey { get { return (_key == Key.System) ? _realKey : Key.None;} } ////// The Key referenced by the event, if the the key is going to /// be processed by Win32 Dead Char System. /// public Key DeadCharProcessedKey { get { return (_key == Key.DeadCharProcessed) ? _realKey : Key.None; } } ////// The state of the key referenced by the event. /// public KeyStates KeyStates { get {return this.KeyboardDevice.GetKeyStates(_realKey);} } ////// Whether the key pressed is a repeated key or not. /// public bool IsRepeat { get {return _isRepeat;} } ////// Whether or not the key referenced by the event is down. /// ///public bool IsDown { get {return this.KeyboardDevice.IsKeyDown(_realKey);} } /// /// Whether or not the key referenced by the event is up. /// ///public bool IsUp { get {return this.KeyboardDevice.IsKeyUp(_realKey);} } /// /// Whether or not the key referenced by the event is toggled. /// ///public bool IsToggled { get {return this.KeyboardDevice.IsKeyToggled(_realKey);} } /// /// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// ///protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { KeyEventHandler handler = (KeyEventHandler) genericHandler; handler(genericTarget, this); } internal void SetRepeat( bool newRepeatState ) { _isRepeat = newRepeatState; } internal void MarkNormal() { _key = _realKey; } internal void MarkSystem() { _key = Key.System; } internal void MarkImeProcessed() { _key = Key.ImeProcessed; } internal void MarkDeadCharProcessed() { _key = Key.DeadCharProcessed; } /// /// Critical - hands out _inputSource. /// internal PresentationSource UnsafeInputSource { [SecurityCritical] get { return _inputSource; } } internal int ScanCode { get {return _scanCode;} set {_scanCode = value;} } internal bool IsExtendedKey { get {return _isExtendedKey;} set {_isExtendedKey = value;} } private Key _realKey; private Key _key; ////// Critical - PresentationSource required elevations to create it. /// [SecurityCritical] private PresentationSource _inputSource; private bool _isRepeat; private int _scanCode; private bool _isExtendedKey; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
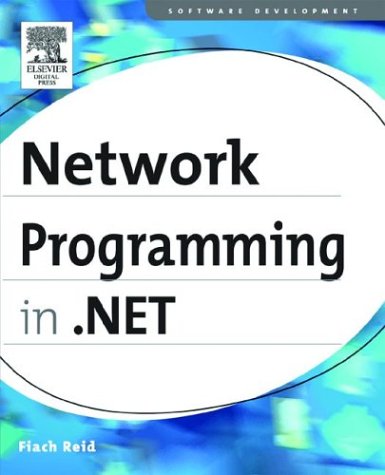
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XMLUtil.cs
- CommonDialog.cs
- SoapFault.cs
- TemplateAction.cs
- WebPartEditorApplyVerb.cs
- ComAdminInterfaces.cs
- DescendantQuery.cs
- LogicalMethodInfo.cs
- GPPOINT.cs
- XmlAttribute.cs
- CustomAttributeBuilder.cs
- Brush.cs
- BindingCollection.cs
- WebControlParameterProxy.cs
- ZipFileInfo.cs
- BuildResultCache.cs
- TreeViewImageIndexConverter.cs
- XhtmlBasicCalendarAdapter.cs
- control.ime.cs
- StoreAnnotationsMap.cs
- Win32.cs
- WebRequestModuleElementCollection.cs
- DataGridColumnHeaderItemAutomationPeer.cs
- ErrorHandler.cs
- CryptoApi.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- BaseCodePageEncoding.cs
- SerializationInfoEnumerator.cs
- SelectorAutomationPeer.cs
- ConnectionStringsSection.cs
- ToolStripProgressBar.cs
- RootProfilePropertySettingsCollection.cs
- XmlSchemaComplexContentRestriction.cs
- LockCookie.cs
- RunInstallerAttribute.cs
- ClientTargetSection.cs
- DefaultSection.cs
- ScrollChrome.cs
- DataServiceSaveChangesEventArgs.cs
- InputChannelAcceptor.cs
- TextTreeInsertElementUndoUnit.cs
- UInt64Storage.cs
- Drawing.cs
- IPeerNeighbor.cs
- FactoryMaker.cs
- EnumType.cs
- XmlMembersMapping.cs
- ListViewSortEventArgs.cs
- TemplateInstanceAttribute.cs
- ConfigDefinitionUpdates.cs
- TableStyle.cs
- CheckoutException.cs
- NullReferenceException.cs
- Maps.cs
- DataBinder.cs
- GrowingArray.cs
- FusionWrap.cs
- GenericPrincipal.cs
- SamlAdvice.cs
- DiscoveryRequestHandler.cs
- Odbc32.cs
- FixedTextPointer.cs
- OutputCacheSection.cs
- UxThemeWrapper.cs
- WebInvokeAttribute.cs
- Enum.cs
- Baml6Assembly.cs
- TypeToken.cs
- ClientType.cs
- TextEffectCollection.cs
- MappingModelBuildProvider.cs
- ScrollChrome.cs
- ComboBoxItem.cs
- DataColumnCollection.cs
- NativeMethods.cs
- WorkItem.cs
- ScrollPattern.cs
- SlipBehavior.cs
- AlternationConverter.cs
- ToolStripPanelCell.cs
- UserInitiatedNavigationPermission.cs
- DeflateInput.cs
- ExpressionPrinter.cs
- CancellationHandler.cs
- validation.cs
- MsmqPoisonMessageException.cs
- OutputScopeManager.cs
- HelpProvider.cs
- AdapterDictionary.cs
- TypeHelpers.cs
- SecurityUtils.cs
- TextBoxView.cs
- DoubleLinkList.cs
- LayoutEvent.cs
- DataControlCommands.cs
- SerializationEventsCache.cs
- NameValueSectionHandler.cs
- Model3DCollection.cs
- ServiceInstanceProvider.cs
- SessionStateSection.cs