Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Win32 / UxThemeWrapper.cs / 1 / UxThemeWrapper.cs
using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Runtime.InteropServices; using System.Security; using System.Windows; using System.Windows.Media; using System.Text; using MS.Win32; using MS.Internal; namespace MS.Win32 { ////// Wrapper class for loading UxTheme system theme data /// internal static class UxThemeWrapper { static UxThemeWrapper() { _isActive = SafeNativeMethods.IsUxThemeActive(); } internal static bool IsActive { get { return _isActive; } } internal static string ThemeName { get { if (IsActive) { if (_themeName == null) { EnsureThemeName(); } return _themeName; } else { return "classic"; } } } internal static string ThemeColor { get { Debug.Assert(IsActive, "Queried ThemeColor while UxTheme is not active."); if (_themeColor == null) { EnsureThemeName(); } return _themeColor; } } ////// Critical - as this code performs an elevation to get current theme name /// TreatAsSafe - the "critical data" is transformed into "safe data" /// all the info stored is the currrent theme name and current color - e.g. "Luna", "NormalColor" /// Does not contain a path - considered safe. /// [SecurityCritical, SecurityTreatAsSafe] private static void EnsureThemeName() { StringBuilder themeName = new StringBuilder(Win32.NativeMethods.MAX_PATH); StringBuilder themeColor = new StringBuilder(Win32.NativeMethods.MAX_PATH); if (UnsafeNativeMethods.GetCurrentThemeName(themeName, themeName.Capacity, themeColor, themeColor.Capacity, null, 0) == 0) { // Success _themeName = themeName.ToString(); _themeName = Path.GetFileNameWithoutExtension(_themeName); _themeColor = themeColor.ToString(); } else { // Failed to retrieve the name _themeName = _themeColor = String.Empty; } } internal static void OnThemeChanged() { _isActive = SafeNativeMethods.IsUxThemeActive(); _themeName = null; _themeColor = null; } private static bool _isActive; private static string _themeName; private static string _themeColor; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Runtime.InteropServices; using System.Security; using System.Windows; using System.Windows.Media; using System.Text; using MS.Win32; using MS.Internal; namespace MS.Win32 { ////// Wrapper class for loading UxTheme system theme data /// internal static class UxThemeWrapper { static UxThemeWrapper() { _isActive = SafeNativeMethods.IsUxThemeActive(); } internal static bool IsActive { get { return _isActive; } } internal static string ThemeName { get { if (IsActive) { if (_themeName == null) { EnsureThemeName(); } return _themeName; } else { return "classic"; } } } internal static string ThemeColor { get { Debug.Assert(IsActive, "Queried ThemeColor while UxTheme is not active."); if (_themeColor == null) { EnsureThemeName(); } return _themeColor; } } ////// Critical - as this code performs an elevation to get current theme name /// TreatAsSafe - the "critical data" is transformed into "safe data" /// all the info stored is the currrent theme name and current color - e.g. "Luna", "NormalColor" /// Does not contain a path - considered safe. /// [SecurityCritical, SecurityTreatAsSafe] private static void EnsureThemeName() { StringBuilder themeName = new StringBuilder(Win32.NativeMethods.MAX_PATH); StringBuilder themeColor = new StringBuilder(Win32.NativeMethods.MAX_PATH); if (UnsafeNativeMethods.GetCurrentThemeName(themeName, themeName.Capacity, themeColor, themeColor.Capacity, null, 0) == 0) { // Success _themeName = themeName.ToString(); _themeName = Path.GetFileNameWithoutExtension(_themeName); _themeColor = themeColor.ToString(); } else { // Failed to retrieve the name _themeName = _themeColor = String.Empty; } } internal static void OnThemeChanged() { _isActive = SafeNativeMethods.IsUxThemeActive(); _themeName = null; _themeColor = null; } private static bool _isActive; private static string _themeName; private static string _themeColor; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
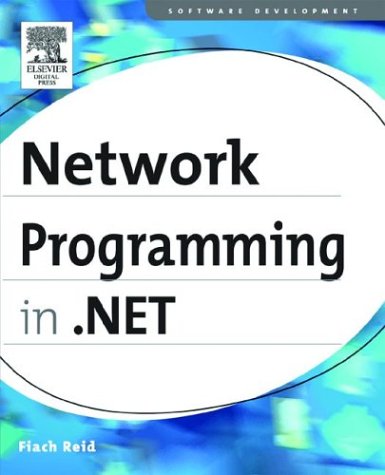
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeProcessHandle.cs
- TiffBitmapEncoder.cs
- WorkflowApplicationUnhandledExceptionEventArgs.cs
- GrowingArray.cs
- PrintingPermission.cs
- DependencyObjectProvider.cs
- EventPrivateKey.cs
- WebPartDisplayModeCollection.cs
- AppDomainResourcePerfCounters.cs
- MultipleCopiesCollection.cs
- XmlDomTextWriter.cs
- TcpProcessProtocolHandler.cs
- NavigationWindow.cs
- WebPartManager.cs
- OracleRowUpdatingEventArgs.cs
- MarkupCompiler.cs
- DbConnectionInternal.cs
- TdsParserSessionPool.cs
- NotSupportedException.cs
- SoapParser.cs
- DataObjectFieldAttribute.cs
- ClockGroup.cs
- EdmValidator.cs
- DataGridTextBoxColumn.cs
- Choices.cs
- TextMessageEncodingElement.cs
- SQLDoubleStorage.cs
- OdbcRowUpdatingEvent.cs
- TransformerInfo.cs
- SettingsPropertyValueCollection.cs
- TreeViewHitTestInfo.cs
- Serializer.cs
- EdmProviderManifest.cs
- XmlSchemaValidator.cs
- OleDbParameter.cs
- SharedPersonalizationStateInfo.cs
- SelectionWordBreaker.cs
- XamlSerializationHelper.cs
- DPTypeDescriptorContext.cs
- FixedTextBuilder.cs
- AffineTransform3D.cs
- BitConverter.cs
- regiisutil.cs
- WindowsToolbarItemAsMenuItem.cs
- WebPartManagerInternals.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- AutomationPropertyInfo.cs
- IntSecurity.cs
- UrlParameterWriter.cs
- AppLevelCompilationSectionCache.cs
- BindToObject.cs
- CounterSet.cs
- ComEventsSink.cs
- CompiledQuery.cs
- ListItemViewControl.cs
- OSFeature.cs
- LabelExpression.cs
- IISUnsafeMethods.cs
- PartialTrustVisibleAssemblyCollection.cs
- ThemeConfigurationDialog.cs
- DiscoveryClientDocuments.cs
- WeakReference.cs
- TableLayoutPanel.cs
- ColumnHeader.cs
- CodeSnippetExpression.cs
- PackageRelationshipSelector.cs
- ToolboxService.cs
- DrawingAttributeSerializer.cs
- Int32RectValueSerializer.cs
- GridViewUpdatedEventArgs.cs
- PackageProperties.cs
- PageContentAsyncResult.cs
- HandleValueEditor.cs
- PageClientProxyGenerator.cs
- ClientScriptManagerWrapper.cs
- Scheduling.cs
- StickyNoteContentControl.cs
- ZipIOLocalFileHeader.cs
- WebBrowserNavigatingEventHandler.cs
- AuthenticationServiceManager.cs
- ToolTipService.cs
- TabPanel.cs
- ObjectAnimationUsingKeyFrames.cs
- DrawingGroupDrawingContext.cs
- SqlDataSourceQueryConverter.cs
- WebPartEditVerb.cs
- UpdateCompiler.cs
- PointCollectionConverter.cs
- FullTextBreakpoint.cs
- IPHostEntry.cs
- nulltextcontainer.cs
- COMException.cs
- StateMachine.cs
- GrammarBuilderWildcard.cs
- SourceFilter.cs
- XmlQueryContext.cs
- odbcmetadatacolumnnames.cs
- Attribute.cs
- ApplicationManager.cs
- ThrowHelper.cs