Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / InlineCollection.cs / 1305600 / InlineCollection.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Collection of Inline elements // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; // Invariant using System.Windows.Markup; // ContentWrapper using System.Windows.Controls; // TextBlock using System.Collections; ////// Collection of Inline elements - elements allowed as children /// of Paragraph, Span and TextBlock elements. /// [ContentWrapper(typeof(Run))] [ContentWrapper(typeof(InlineUIContainer))] [WhitespaceSignificantCollection] public class InlineCollection : TextElementCollection, IList { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors // Constructor is internal. We allow InlineCollection creation only from inside owning elements such as TextBlock or TextElement. // Note that when a SiblingInlines collection is created for an Inline, the owner of collection is that member Inline object. // Flag isOwnerParent indicates whether owner is a parent or a member of the collection. internal InlineCollection(DependencyObject owner, bool isOwnerParent) : base(owner, isOwnerParent) { } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods /// /// Implementation of Add method from IList /// internal override int OnAdd(object value) { int index; string text = value as string; if (text != null) { index = AddText(text, true /* returnIndex */); } else { this.TextContainer.BeginChange(); try { UIElement uiElement = value as UIElement; if (uiElement != null) { index = AddUIElement(uiElement, true /* returnIndex */); } else { index = base.OnAdd(value); } } finally { this.TextContainer.EndChange(); } } return index; } ////// Adds an implicit Run element with a given text /// /// /// Text set as a Text property for implicit Run. /// public void Add(string text) { AddText(text, false /* returnIndex */); } ////// Adds an implicit InlineUIContainer with a given UIElement in it. /// /// /// UIElement set as a Child property for the implicit InlineUIContainer. /// public void Add(UIElement uiElement) { AddUIElement(uiElement, false /* returnIndex */); } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ////// Returns a first Inline element of this collection /// public Inline FirstInline { get { return this.FirstChild; } } ////// Returns a last Inline element of this collection /// public Inline LastInline { get { return this.LastChild; } } #endregion Public Properties //------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods ////// This method performs schema validation for inline collections. /// (1) We want to disallow nested Hyperlink elements. /// (2) Also, a Hyperlink element allows only these child types: Run, InlineUIContainer and Span elements other than Hyperlink. /// internal override void ValidateChild(Inline child) { base.ValidateChild(child); if (this.Parent is TextElement) { TextSchema.ValidateChild((TextElement)this.Parent, child, true /* throwIfIllegalChild */, true /* throwIfIllegalHyperlinkDescendent */); } else { if (!TextSchema.IsValidChildOfContainer(this.Parent.GetType(), child.GetType())) { throw new InvalidOperationException(SR.Get(SRID.TextSchema_ChildTypeIsInvalid, this.Parent.GetType().Name, child.GetType().Name)); } } } #endregion Internal Methods //------------------------------------------------------------------- // // Private Methods // //------------------------------------------------------------------- #region Private Methods // Worker for OnAdd and Add(string). // If returnIndex == true, uses the more costly IList.Add // to calculate and return the index of the newly inserted // Run, otherwise returns -1. private int AddText(string text, bool returnIndex) { if (text == null) { throw new ArgumentNullException("text"); } // Special case for TextBlock - to keep its simple content in simple state if (this.Parent is TextBlock) { TextBlock textBlock = (TextBlock)this.Parent; if (!textBlock.HasComplexContent) { textBlock.Text = textBlock.Text + text; return 0; // There's always one implicit Run with simple content, at index 0. } } this.TextContainer.BeginChange(); try { Run implicitRun = Run.CreateImplicitRun(this.Parent); int index; if (returnIndex) { index = base.OnAdd(implicitRun); } else { this.Add(implicitRun); index = -1; } // Set the Text property after inserting the Run to avoid allocating // a temporary TextContainer. implicitRun.Text = text; return index; } finally { this.TextContainer.EndChange(); } } // Worker for OnAdd and Add(UIElement). // If returnIndex == true, uses the more costly IList.Add // to calculate and return the index of the newly inserted // Run, otherwise returns -1. private int AddUIElement(UIElement uiElement, bool returnIndex) { if (uiElement == null) { throw new ArgumentNullException("uiElement"); } InlineUIContainer implicitInlineUIContainer = Run.CreateImplicitInlineUIContainer(this.Parent); int index; if (returnIndex) { index = base.OnAdd(implicitInlineUIContainer); } else { this.Add(implicitInlineUIContainer); index = -1; } implicitInlineUIContainer.Child = uiElement; return index; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Collection of Inline elements // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; // Invariant using System.Windows.Markup; // ContentWrapper using System.Windows.Controls; // TextBlock using System.Collections; ////// Collection of Inline elements - elements allowed as children /// of Paragraph, Span and TextBlock elements. /// [ContentWrapper(typeof(Run))] [ContentWrapper(typeof(InlineUIContainer))] [WhitespaceSignificantCollection] public class InlineCollection : TextElementCollection, IList { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors // Constructor is internal. We allow InlineCollection creation only from inside owning elements such as TextBlock or TextElement. // Note that when a SiblingInlines collection is created for an Inline, the owner of collection is that member Inline object. // Flag isOwnerParent indicates whether owner is a parent or a member of the collection. internal InlineCollection(DependencyObject owner, bool isOwnerParent) : base(owner, isOwnerParent) { } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods /// /// Implementation of Add method from IList /// internal override int OnAdd(object value) { int index; string text = value as string; if (text != null) { index = AddText(text, true /* returnIndex */); } else { this.TextContainer.BeginChange(); try { UIElement uiElement = value as UIElement; if (uiElement != null) { index = AddUIElement(uiElement, true /* returnIndex */); } else { index = base.OnAdd(value); } } finally { this.TextContainer.EndChange(); } } return index; } ////// Adds an implicit Run element with a given text /// /// /// Text set as a Text property for implicit Run. /// public void Add(string text) { AddText(text, false /* returnIndex */); } ////// Adds an implicit InlineUIContainer with a given UIElement in it. /// /// /// UIElement set as a Child property for the implicit InlineUIContainer. /// public void Add(UIElement uiElement) { AddUIElement(uiElement, false /* returnIndex */); } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ////// Returns a first Inline element of this collection /// public Inline FirstInline { get { return this.FirstChild; } } ////// Returns a last Inline element of this collection /// public Inline LastInline { get { return this.LastChild; } } #endregion Public Properties //------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods ////// This method performs schema validation for inline collections. /// (1) We want to disallow nested Hyperlink elements. /// (2) Also, a Hyperlink element allows only these child types: Run, InlineUIContainer and Span elements other than Hyperlink. /// internal override void ValidateChild(Inline child) { base.ValidateChild(child); if (this.Parent is TextElement) { TextSchema.ValidateChild((TextElement)this.Parent, child, true /* throwIfIllegalChild */, true /* throwIfIllegalHyperlinkDescendent */); } else { if (!TextSchema.IsValidChildOfContainer(this.Parent.GetType(), child.GetType())) { throw new InvalidOperationException(SR.Get(SRID.TextSchema_ChildTypeIsInvalid, this.Parent.GetType().Name, child.GetType().Name)); } } } #endregion Internal Methods //------------------------------------------------------------------- // // Private Methods // //------------------------------------------------------------------- #region Private Methods // Worker for OnAdd and Add(string). // If returnIndex == true, uses the more costly IList.Add // to calculate and return the index of the newly inserted // Run, otherwise returns -1. private int AddText(string text, bool returnIndex) { if (text == null) { throw new ArgumentNullException("text"); } // Special case for TextBlock - to keep its simple content in simple state if (this.Parent is TextBlock) { TextBlock textBlock = (TextBlock)this.Parent; if (!textBlock.HasComplexContent) { textBlock.Text = textBlock.Text + text; return 0; // There's always one implicit Run with simple content, at index 0. } } this.TextContainer.BeginChange(); try { Run implicitRun = Run.CreateImplicitRun(this.Parent); int index; if (returnIndex) { index = base.OnAdd(implicitRun); } else { this.Add(implicitRun); index = -1; } // Set the Text property after inserting the Run to avoid allocating // a temporary TextContainer. implicitRun.Text = text; return index; } finally { this.TextContainer.EndChange(); } } // Worker for OnAdd and Add(UIElement). // If returnIndex == true, uses the more costly IList.Add // to calculate and return the index of the newly inserted // Run, otherwise returns -1. private int AddUIElement(UIElement uiElement, bool returnIndex) { if (uiElement == null) { throw new ArgumentNullException("uiElement"); } InlineUIContainer implicitInlineUIContainer = Run.CreateImplicitInlineUIContainer(this.Parent); int index; if (returnIndex) { index = base.OnAdd(implicitInlineUIContainer); } else { this.Add(implicitInlineUIContainer); index = -1; } implicitInlineUIContainer.Child = uiElement; return index; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
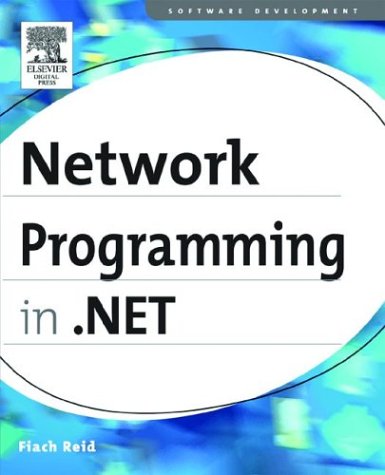
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeTimerHandle.cs
- ObjectViewListener.cs
- FragmentQueryKB.cs
- WebPermission.cs
- PreviewKeyDownEventArgs.cs
- TextBox.cs
- ValueOfAction.cs
- WebPart.cs
- ClientBuildManagerCallback.cs
- BitmapSource.cs
- NgenServicingAttributes.cs
- SafeNativeMethods.cs
- DynamicValidatorEventArgs.cs
- DispatcherHookEventArgs.cs
- AdapterSwitches.cs
- OAVariantLib.cs
- BridgeDataReader.cs
- SimpleApplicationHost.cs
- Options.cs
- CounterCreationDataConverter.cs
- StreamUpdate.cs
- UInt64Converter.cs
- ValueUnavailableException.cs
- SimpleTextLine.cs
- ScaleTransform3D.cs
- ScriptingJsonSerializationSection.cs
- FormatVersion.cs
- AppSettingsExpressionBuilder.cs
- MsmqIntegrationAppDomainProtocolHandler.cs
- __Filters.cs
- BaseDataBoundControl.cs
- SystemIPInterfaceProperties.cs
- SmiMetaDataProperty.cs
- PKCS1MaskGenerationMethod.cs
- ISAPIWorkerRequest.cs
- DataSourceControl.cs
- DetailsViewRow.cs
- DetailsViewInsertEventArgs.cs
- GeometryModel3D.cs
- SoapCommonClasses.cs
- X509RecipientCertificateServiceElement.cs
- DebuggerAttributes.cs
- ComponentEvent.cs
- DelegatingConfigHost.cs
- VirtualDirectoryMappingCollection.cs
- TreeNodeBindingDepthConverter.cs
- ClientFormsAuthenticationMembershipProvider.cs
- InvalidPrinterException.cs
- GroupDescription.cs
- EncoderNLS.cs
- SubMenuStyleCollection.cs
- ProtocolException.cs
- ResourcePool.cs
- ResourceDictionary.cs
- DataGridViewCellConverter.cs
- DataSourceSelectArguments.cs
- RelationshipNavigation.cs
- SqlConnectionManager.cs
- ArgumentException.cs
- IdnElement.cs
- DesignBindingEditor.cs
- UrlPath.cs
- SQLDecimal.cs
- BamlRecordHelper.cs
- FusionWrap.cs
- objectresult_tresulttype.cs
- PowerStatus.cs
- _DigestClient.cs
- RSAPKCS1SignatureFormatter.cs
- ConfigurationStrings.cs
- CellNormalizer.cs
- ContainsSearchOperator.cs
- AbsoluteQuery.cs
- StylusLogic.cs
- SQLDateTimeStorage.cs
- VirtualPathProvider.cs
- Tile.cs
- X509ChainElement.cs
- ContainerSelectorGlyph.cs
- RecognizerInfo.cs
- TreeBuilder.cs
- CustomTypeDescriptor.cs
- PanelStyle.cs
- FlowLayoutSettings.cs
- ToolStripRenderer.cs
- RelatedCurrencyManager.cs
- DataTablePropertyDescriptor.cs
- SymmetricKeyWrap.cs
- StringSource.cs
- FontFaceLayoutInfo.cs
- NotifyIcon.cs
- ComponentSerializationService.cs
- CollectionBuilder.cs
- DefaultMemberAttribute.cs
- CompositeControlDesigner.cs
- ClientUriBehavior.cs
- LazyInitializer.cs
- CompressStream.cs
- DbModificationCommandTree.cs
- SqlFormatter.cs