Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / ValueOfAction.cs / 1 / ValueOfAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class ValueOfAction : CompiledAction { private const int ResultStored = 2; private int selectKey = Compiler.InvalidQueryKey; private bool disableOutputEscaping; private static Action s_BuiltInRule = new BuiltInRuleTextAction(); internal static Action BuiltInRule() { Debug.Assert(s_BuiltInRule != null); return s_BuiltInRule; } internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, selectKey != Compiler.InvalidQueryKey, Keywords.s_Select); CheckEmpty(compiler); } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Select)) { this.selectKey = compiler.AddQuery(value); } else if (Keywords.Equals(name, compiler.Atoms.DisableOutputEscaping)) { this.disableOutputEscaping = compiler.GetYesNo(value); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch (frame.State) { case Initialized: Debug.Assert(frame != null); Debug.Assert(frame.NodeSet != null); string value = processor.ValueOf(frame, this.selectKey); if (processor.TextEvent(value, disableOutputEscaping)) { frame.Finished(); } else { frame.StoredOutput = value; frame.State = ResultStored; } break; case ResultStored: Debug.Assert(frame.StoredOutput != null); processor.TextEvent(frame.StoredOutput); frame.Finished(); break; default: Debug.Fail("Invalid ValueOfAction execution state"); break; } } } internal class BuiltInRuleTextAction : Action { private const int ResultStored = 2; internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch (frame.State) { case Initialized: Debug.Assert(frame != null); Debug.Assert(frame.NodeSet != null); string value = processor.ValueOf(frame.NodeSet.Current); if (processor.TextEvent(value, /*disableOutputEscaping:*/false)) { frame.Finished(); } else { frame.StoredOutput = value; frame.State = ResultStored; } break; case ResultStored: Debug.Assert(frame.StoredOutput != null); processor.TextEvent(frame.StoredOutput); frame.Finished(); break; default: Debug.Fail("Invalid BuiltInRuleTextAction execution state"); break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class ValueOfAction : CompiledAction { private const int ResultStored = 2; private int selectKey = Compiler.InvalidQueryKey; private bool disableOutputEscaping; private static Action s_BuiltInRule = new BuiltInRuleTextAction(); internal static Action BuiltInRule() { Debug.Assert(s_BuiltInRule != null); return s_BuiltInRule; } internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, selectKey != Compiler.InvalidQueryKey, Keywords.s_Select); CheckEmpty(compiler); } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Select)) { this.selectKey = compiler.AddQuery(value); } else if (Keywords.Equals(name, compiler.Atoms.DisableOutputEscaping)) { this.disableOutputEscaping = compiler.GetYesNo(value); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch (frame.State) { case Initialized: Debug.Assert(frame != null); Debug.Assert(frame.NodeSet != null); string value = processor.ValueOf(frame, this.selectKey); if (processor.TextEvent(value, disableOutputEscaping)) { frame.Finished(); } else { frame.StoredOutput = value; frame.State = ResultStored; } break; case ResultStored: Debug.Assert(frame.StoredOutput != null); processor.TextEvent(frame.StoredOutput); frame.Finished(); break; default: Debug.Fail("Invalid ValueOfAction execution state"); break; } } } internal class BuiltInRuleTextAction : Action { private const int ResultStored = 2; internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch (frame.State) { case Initialized: Debug.Assert(frame != null); Debug.Assert(frame.NodeSet != null); string value = processor.ValueOf(frame.NodeSet.Current); if (processor.TextEvent(value, /*disableOutputEscaping:*/false)) { frame.Finished(); } else { frame.StoredOutput = value; frame.State = ResultStored; } break; case ResultStored: Debug.Assert(frame.StoredOutput != null); processor.TextEvent(frame.StoredOutput); frame.Finished(); break; default: Debug.Fail("Invalid BuiltInRuleTextAction execution state"); break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
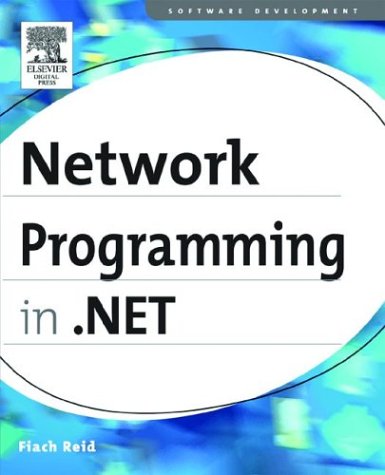
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HuffModule.cs
- XPathDocument.cs
- ContextMenuStrip.cs
- WindowsRegion.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- XmlDocumentFragment.cs
- WasEndpointConfigContainer.cs
- WebPartDisplayModeCancelEventArgs.cs
- TypeViewSchema.cs
- TrustExchangeException.cs
- RadioButtonFlatAdapter.cs
- SelectionChangedEventArgs.cs
- OutputCacheProviderCollection.cs
- DBConcurrencyException.cs
- GPPOINTF.cs
- PrimaryKeyTypeConverter.cs
- OlePropertyStructs.cs
- ScriptRef.cs
- CompositeScriptReferenceEventArgs.cs
- MediaContextNotificationWindow.cs
- TdsParserHelperClasses.cs
- EditorPart.cs
- DatePickerAutomationPeer.cs
- C14NUtil.cs
- GridViewRowEventArgs.cs
- __ComObject.cs
- Mappings.cs
- StatusCommandUI.cs
- Journal.cs
- Point3DValueSerializer.cs
- SqlDataSourceParameterParser.cs
- NativeMethodsCLR.cs
- StringFormat.cs
- UserNameSecurityToken.cs
- ImageMetadata.cs
- WebPartTransformerCollection.cs
- HttpResponse.cs
- DictionaryMarkupSerializer.cs
- HtmlCommandAdapter.cs
- DataListGeneralPage.cs
- SocketElement.cs
- ItemsControlAutomationPeer.cs
- ConfigXmlWhitespace.cs
- AuthenticatingEventArgs.cs
- ClickablePoint.cs
- DataReceivedEventArgs.cs
- Mappings.cs
- KeyBinding.cs
- DocumentStatusResources.cs
- ProxyWebPart.cs
- TableHeaderCell.cs
- ValidationHelper.cs
- DataGridViewEditingControlShowingEventArgs.cs
- SourceChangedEventArgs.cs
- EmptyEnumerable.cs
- CannotUnloadAppDomainException.cs
- TextBoxDesigner.cs
- StrokeFIndices.cs
- XmlDataSourceDesigner.cs
- DataGridViewTopRowAccessibleObject.cs
- BaseParser.cs
- NonBatchDirectoryCompiler.cs
- DependencyPropertyAttribute.cs
- XMLUtil.cs
- UnsafeNativeMethods.cs
- PersistencePipeline.cs
- GenericTextProperties.cs
- EntityDataSourceSelectedEventArgs.cs
- SystemIcmpV4Statistics.cs
- WindowInteropHelper.cs
- TrackingMemoryStream.cs
- TraceProvider.cs
- GroupDescription.cs
- FunctionDescription.cs
- WebControlAdapter.cs
- HttpVersion.cs
- ObjectReferenceStack.cs
- Section.cs
- HebrewNumber.cs
- SrgsDocumentParser.cs
- RecordManager.cs
- UriScheme.cs
- SeparatorAutomationPeer.cs
- WeakReferenceEnumerator.cs
- SelectionUIHandler.cs
- TransactedReceiveScope.cs
- SqlProviderManifest.cs
- GeneralTransform.cs
- ConfigPathUtility.cs
- ToolStripRenderer.cs
- FontInfo.cs
- SocketException.cs
- HttpCookie.cs
- WindowsListViewScroll.cs
- QueryAccessibilityHelpEvent.cs
- Size3DConverter.cs
- BuildDependencySet.cs
- TextEditorContextMenu.cs
- CreationContext.cs
- WeakReferenceKey.cs