Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Configuration / behaviorssection.cs / 1 / behaviorssection.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Configuration { using System.Configuration; using System.Globalization; using System.ServiceModel; using System.Security; public partial class BehaviorsSection : ConfigurationSection { [ConfigurationProperty(ConfigurationStrings.EndpointBehaviors, Options = ConfigurationPropertyOptions.None)] public EndpointBehaviorElementCollection EndpointBehaviors { get { return (EndpointBehaviorElementCollection)base[ConfigurationStrings.EndpointBehaviors]; } } [ConfigurationProperty(ConfigurationStrings.ServiceBehaviors, Options = ConfigurationPropertyOptions.None)] public ServiceBehaviorElementCollection ServiceBehaviors { get { return (ServiceBehaviorElementCollection)base[ConfigurationStrings.ServiceBehaviors]; } } internal static BehaviorsSection GetSection() { return (BehaviorsSection)ConfigurationHelpers.GetSection(ConfigurationStrings.BehaviorsSectionPath); } ////// Critical - calls Critical method UnsafeGetSection which elevates in order to fetch config /// caller must guard access to resultant config section /// [SecurityCritical] internal static BehaviorsSection UnsafeGetSection() { return (BehaviorsSection)ConfigurationHelpers.UnsafeGetSection(ConfigurationStrings.BehaviorsSectionPath); } ////// Critical - calls Critical method UnsafeGetAssociatedSection which elevates in order to fetch config /// caller must guard access to resultant config section /// [SecurityCritical] internal static BehaviorsSection UnsafeGetAssociatedSection(ContextInformation evalContext) { return (BehaviorsSection)ConfigurationHelpers.UnsafeGetAssociatedSection(evalContext, ConfigurationStrings.BehaviorsSectionPath); } ////// Critical - calls UnsafeGetAssociatedSection which elevates /// Safe - doesn't leak resultant config /// [SecurityCritical, SecurityTreatAsSafe] internal static void ValidateEndpointBehaviorReference(string behaviorConfiguration, ContextInformation evaluationContext, ConfigurationElement configurationElement) { // ValidateBehaviorReference built on assumption that evaluationContext is valid. // This should be protected at the callers site. If assumption is invalid, then // configuration system is in an indeterminate state. Need to stop in a manner that // user code can not capture. if (null == evaluationContext) { DiagnosticUtility.DebugAssert("ValidateBehaviorReference() should only called with valid ContextInformation"); DiagnosticUtility.FailFast("ValidateBehaviorReference() should only called with valid ContextInformation"); } if (!String.IsNullOrEmpty(behaviorConfiguration)) { BehaviorsSection behaviors = (BehaviorsSection)ConfigurationHelpers.UnsafeGetAssociatedSection(evaluationContext, ConfigurationStrings.BehaviorsSectionPath); if (!behaviors.EndpointBehaviors.ContainsKey(behaviorConfiguration)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ConfigurationErrorsException(SR.GetString(SR.ConfigInvalidEndpointBehavior, behaviorConfiguration), configurationElement.ElementInformation.Source, configurationElement.ElementInformation.LineNumber)); } } } ////// Critical - calls UnsafeGetAssociatedSection which elevates /// Safe - doesn't leak resultant config /// [SecurityCritical, SecurityTreatAsSafe] internal static void ValidateServiceBehaviorReference(string behaviorConfiguration, ContextInformation evaluationContext, ConfigurationElement configurationElement) { // ValidateBehaviorReference built on assumption that evaluationContext is valid. // This should be protected at the callers site. If assumption is invalid, then // configuration system is in an indeterminate state. Need to stop in a manner that // user code can not capture. if (null == evaluationContext) { DiagnosticUtility.DebugAssert("ValidateBehaviorReference() should only called with valid ContextInformation"); DiagnosticUtility.FailFast("ValidateBehaviorReference() should only called with valid ContextInformation"); } if (!String.IsNullOrEmpty(behaviorConfiguration)) { BehaviorsSection behaviors = (BehaviorsSection)ConfigurationHelpers.UnsafeGetAssociatedSection(evaluationContext, ConfigurationStrings.BehaviorsSectionPath); if (!behaviors.ServiceBehaviors.ContainsKey(behaviorConfiguration)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ConfigurationErrorsException(SR.GetString(SR.ConfigInvalidServiceBehavior, behaviorConfiguration), configurationElement.ElementInformation.Source, configurationElement.ElementInformation.LineNumber)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
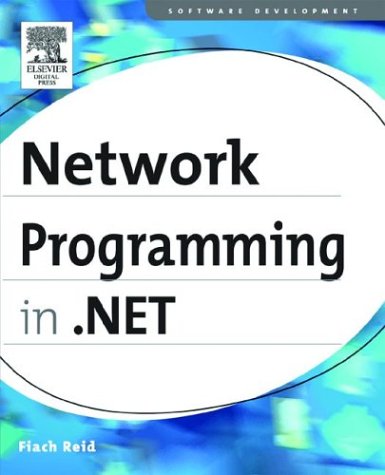
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ComponentCache.cs
- CompositeCollectionView.cs
- HtmlElementEventArgs.cs
- ProfilePropertySettingsCollection.cs
- ThemeableAttribute.cs
- BamlBinaryReader.cs
- HtmlInputImage.cs
- CodeDomDecompiler.cs
- BaseTemplateParser.cs
- LinqDataSourceInsertEventArgs.cs
- HtmlTableRow.cs
- TimeStampChecker.cs
- dsa.cs
- _HTTPDateParse.cs
- Control.cs
- XmlSerializationWriter.cs
- MethodBuilder.cs
- XmlNullResolver.cs
- InlinedAggregationOperatorEnumerator.cs
- MachineKeySection.cs
- EntityCommandCompilationException.cs
- BamlRecordReader.cs
- StrokeNode.cs
- DataGridViewSelectedColumnCollection.cs
- UnionExpr.cs
- HtmlContainerControl.cs
- EntityDataSourceWizardForm.cs
- _Win32.cs
- TextAutomationPeer.cs
- hebrewshape.cs
- HtmlPageAdapter.cs
- PeerValidationBehavior.cs
- AttributeCollection.cs
- WindowsFormsHost.cs
- OleDbRowUpdatingEvent.cs
- WithParamAction.cs
- TrackingProfileCache.cs
- XmlSchemaSequence.cs
- MsmqElementBase.cs
- SessionStateSection.cs
- SchemaImporterExtensionElement.cs
- DWriteFactory.cs
- ParserHooks.cs
- DataSourceCache.cs
- SqlRowUpdatedEvent.cs
- MDIControlStrip.cs
- HtmlFormWrapper.cs
- ThrowHelper.cs
- HtmlAnchor.cs
- validationstate.cs
- TextRangeEdit.cs
- Timer.cs
- EntityContainerAssociationSet.cs
- BinaryUtilClasses.cs
- CopyCodeAction.cs
- DataServiceProcessingPipeline.cs
- ExtenderControl.cs
- TextWriterEngine.cs
- StructuralObject.cs
- TextTreeNode.cs
- serverconfig.cs
- IdentityVerifier.cs
- VisemeEventArgs.cs
- FacetValueContainer.cs
- GlyphTypeface.cs
- ToolStripHighContrastRenderer.cs
- Delegate.cs
- JavascriptCallbackMessageInspector.cs
- DataObjectEventArgs.cs
- TypeConstant.cs
- TextRangeAdaptor.cs
- DataSourceHelper.cs
- DbgUtil.cs
- WebSysDescriptionAttribute.cs
- TreeViewImageIndexConverter.cs
- StyleXamlParser.cs
- FloaterParagraph.cs
- SecurityDescriptor.cs
- ListDictionary.cs
- PeoplePickerWrapper.cs
- MultiPropertyDescriptorGridEntry.cs
- Signature.cs
- FixUpCollection.cs
- GacUtil.cs
- JournalEntryStack.cs
- CancelEventArgs.cs
- ProfileGroupSettingsCollection.cs
- PageAdapter.cs
- WebBrowserDesigner.cs
- XmlSchemaObjectTable.cs
- COM2ColorConverter.cs
- precedingsibling.cs
- XamlTreeBuilder.cs
- ScriptingRoleServiceSection.cs
- CompositeFontFamily.cs
- XhtmlBasicImageAdapter.cs
- Rect3DValueSerializer.cs
- TypeNameHelper.cs
- ScaleTransform.cs
- SQLString.cs