Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Recognition / RecognizerInfo.cs / 1 / RecognizerInfo.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Globalization; using System.Speech.AudioFormat; using System.Speech.Internal; using System.Speech.Internal.SapiInterop; using System.Speech.Internal.ObjectTokens; using RegistryEntry = System.Collections.Generic.KeyValuePair; namespace System.Speech.Recognition { /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo"]/*' /> // This represents the attributes various speech recognizers may, or may not support. public class RecognizerInfo { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors private RecognizerInfo (ObjectToken token, CultureInfo culture) { // Retrieve the token name _id = token.Name; // Retrieve default display name _description = token.Description; // Store full object token id for internal use. _sapiObjectTokenId = token.Id; _name = token.TokenName (); _culture = culture; // Enum all values and add to custom table foreach (string keyName in token.Attributes.GetValueNames ()) { string attributeValue; if (token.Attributes.TryGetString (keyName, out attributeValue)) { _attributes.InternalDictionary [keyName] = attributeValue; } } #if !SPEECHSERVER string audioFormats; if (token.Attributes.TryGetString ("AudioFormats", out audioFormats)) { _supportedAudioFormats = new ReadOnlyCollection (SapiAttributeParser.GetAudioFormatsFromString (audioFormats)); } else { _supportedAudioFormats = new ReadOnlyCollection (new List ()); } #endif } static internal RecognizerInfo Create (ObjectToken token) { // Token for recognizer should have Attributes. if (token.Attributes == null) { return null; } // Get other attributes string langId; // must have a language id if (!token.Attributes.TryGetString ("Language", out langId)) { return null; } CultureInfo cultureInfo = SapiAttributeParser.GetCultureInfoFromLanguageString (langId); if (cultureInfo != null) { return new RecognizerInfo(token, cultureInfo); } else { return null; } } #endregion //******************************************************************** // // Public Properties // //******************************************************************* #region public Properties /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo.Name"]/*' /> public string Id { get { return _id; } } /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo.Name"]/*' /> public string Name { get { return _name; } } /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo.DisplayName"]/*' /> public string Description { get { return _description; } } /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo.CultureInfo"]/*' /> public CultureInfo Culture { get { return _culture; } } #if !SPEECHSERVER /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo.SupportedAudioFormats"]/*' /> public ReadOnlyCollection SupportedAudioFormats { get { return _supportedAudioFormats; } } #endif /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo.AdditionalInfo"]/*' /> public IDictionary AdditionalInfo { get { return _attributes; } } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties internal string SapiObjectTokenId { get { return _sapiObjectTokenId; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields // This table stores each attribute private ReadOnlyDictionary _attributes = new ReadOnlyDictionary (); // Named attributes - these get initialized in constructor private string _id; private string _name; private string _description; private string _sapiObjectTokenId; private CultureInfo _culture; #if !SPEECHSERVER private ReadOnlyCollection _supportedAudioFormats; #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Globalization; using System.Speech.AudioFormat; using System.Speech.Internal; using System.Speech.Internal.SapiInterop; using System.Speech.Internal.ObjectTokens; using RegistryEntry = System.Collections.Generic.KeyValuePair; namespace System.Speech.Recognition { /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo"]/*' /> // This represents the attributes various speech recognizers may, or may not support. public class RecognizerInfo { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors private RecognizerInfo (ObjectToken token, CultureInfo culture) { // Retrieve the token name _id = token.Name; // Retrieve default display name _description = token.Description; // Store full object token id for internal use. _sapiObjectTokenId = token.Id; _name = token.TokenName (); _culture = culture; // Enum all values and add to custom table foreach (string keyName in token.Attributes.GetValueNames ()) { string attributeValue; if (token.Attributes.TryGetString (keyName, out attributeValue)) { _attributes.InternalDictionary [keyName] = attributeValue; } } #if !SPEECHSERVER string audioFormats; if (token.Attributes.TryGetString ("AudioFormats", out audioFormats)) { _supportedAudioFormats = new ReadOnlyCollection (SapiAttributeParser.GetAudioFormatsFromString (audioFormats)); } else { _supportedAudioFormats = new ReadOnlyCollection (new List ()); } #endif } static internal RecognizerInfo Create (ObjectToken token) { // Token for recognizer should have Attributes. if (token.Attributes == null) { return null; } // Get other attributes string langId; // must have a language id if (!token.Attributes.TryGetString ("Language", out langId)) { return null; } CultureInfo cultureInfo = SapiAttributeParser.GetCultureInfoFromLanguageString (langId); if (cultureInfo != null) { return new RecognizerInfo(token, cultureInfo); } else { return null; } } #endregion //******************************************************************** // // Public Properties // //******************************************************************* #region public Properties /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo.Name"]/*' /> public string Id { get { return _id; } } /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo.Name"]/*' /> public string Name { get { return _name; } } /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo.DisplayName"]/*' /> public string Description { get { return _description; } } /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo.CultureInfo"]/*' /> public CultureInfo Culture { get { return _culture; } } #if !SPEECHSERVER /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo.SupportedAudioFormats"]/*' /> public ReadOnlyCollection SupportedAudioFormats { get { return _supportedAudioFormats; } } #endif /// TODOC <_include file='doc\RecognizerInfo.uex' path='docs/doc[@for="RecognizerInfo.AdditionalInfo"]/*' /> public IDictionary AdditionalInfo { get { return _attributes; } } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties internal string SapiObjectTokenId { get { return _sapiObjectTokenId; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields // This table stores each attribute private ReadOnlyDictionary _attributes = new ReadOnlyDictionary (); // Named attributes - these get initialized in constructor private string _id; private string _name; private string _description; private string _sapiObjectTokenId; private CultureInfo _culture; #if !SPEECHSERVER private ReadOnlyCollection _supportedAudioFormats; #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
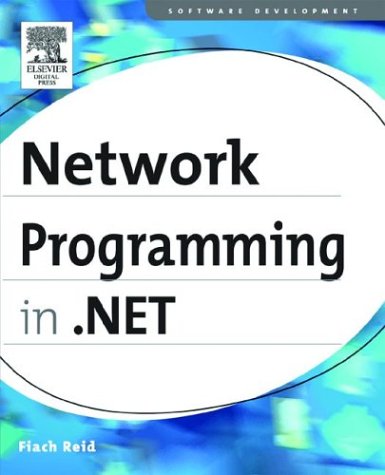
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PenLineCapValidation.cs
- DoubleAnimationUsingKeyFrames.cs
- RbTree.cs
- WebPartZoneDesigner.cs
- ApplicationBuildProvider.cs
- InternalPermissions.cs
- CacheModeValueSerializer.cs
- TypeForwardedToAttribute.cs
- BamlStream.cs
- SQLMoneyStorage.cs
- SByte.cs
- Window.cs
- Decorator.cs
- InvokeProviderWrapper.cs
- ValueTypeFixupInfo.cs
- HWStack.cs
- SliderAutomationPeer.cs
- NameScopePropertyAttribute.cs
- CodeCommentStatementCollection.cs
- UserPreferenceChangingEventArgs.cs
- HandlerFactoryWrapper.cs
- MarkupCompilePass2.cs
- SqlDataSourceSelectingEventArgs.cs
- XmlSchemaInfo.cs
- IsolatedStorageFile.cs
- CellCreator.cs
- OrderedDictionary.cs
- FactoryGenerator.cs
- PersistenceContextEnlistment.cs
- SerializerWriterEventHandlers.cs
- MatrixTransform.cs
- AuthenticatedStream.cs
- EmbossBitmapEffect.cs
- OletxEnlistment.cs
- FloatMinMaxAggregationOperator.cs
- NativeConfigurationLoader.cs
- uribuilder.cs
- DropSource.cs
- EmbeddedMailObject.cs
- TextClipboardData.cs
- DesignTimeType.cs
- TreeViewCancelEvent.cs
- SQLDecimalStorage.cs
- DataTableTypeConverter.cs
- ProxyAttribute.cs
- TransformerInfoCollection.cs
- DefaultParameterValueAttribute.cs
- FrugalMap.cs
- ExpressionReplacer.cs
- CodeTypeParameter.cs
- _SslSessionsCache.cs
- FrameworkTextComposition.cs
- Stream.cs
- TextDecorations.cs
- EnvironmentPermission.cs
- DefaultTraceListener.cs
- _LoggingObject.cs
- AmbientLight.cs
- PathGeometry.cs
- ClientType.cs
- ChildrenQuery.cs
- ControlIdConverter.cs
- QuaternionConverter.cs
- HttpAsyncResult.cs
- DataGridViewColumnDesigner.cs
- HandleExceptionArgs.cs
- XamlFrame.cs
- RowVisual.cs
- ByteAnimationUsingKeyFrames.cs
- MatrixTransform.cs
- Decoder.cs
- EdmPropertyAttribute.cs
- FocusManager.cs
- __Filters.cs
- Quad.cs
- OperationExecutionFault.cs
- RequestTimeoutManager.cs
- BoundColumn.cs
- UIPropertyMetadata.cs
- FillRuleValidation.cs
- ProjectionCamera.cs
- HttpStreamMessage.cs
- DataPointer.cs
- XPathAxisIterator.cs
- VisualStyleRenderer.cs
- HttpWrapper.cs
- SessionKeyExpiredException.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- ExpressionCopier.cs
- DescendantQuery.cs
- SoapIncludeAttribute.cs
- Inflater.cs
- CompoundFileStorageReference.cs
- ActivityPreviewDesigner.cs
- IntPtr.cs
- propertyentry.cs
- CheckedListBox.cs
- ConfigXmlDocument.cs
- StyleSheetRefUrlEditor.cs
- XmlLinkedNode.cs