Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / DbExpressionVisitor_TResultType.cs / 1305376 / DbExpressionVisitor_TResultType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Metadata.Edm; namespace System.Data.Common.CommandTrees { ////// The expression visitor pattern abstract base class that should be implemented by visitors that return a result value of a specific type. /// ///The type of the result value produced by the visitor. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbExpressionVisitor{ /// /// Called when an expression of an otherwise unrecognized type is encountered. /// /// The expression. public abstract TResultType Visit(DbExpression expression); ////// Typed visitor pattern method for DbAndExpression. /// /// The DbAndExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbAndExpression expression); ////// Typed visitor pattern method for DbApplyExpression. /// /// The DbApplyExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbApplyExpression expression); ////// Typed visitor pattern method for DbArithmeticExpression. /// /// The DbArithmeticExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbArithmeticExpression expression); ////// Typed visitor pattern method for DbCaseExpression. /// /// The DbCaseExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbCaseExpression expression); ////// Typed visitor pattern method for DbCastExpression. /// /// The DbCastExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbCastExpression expression); ////// Typed visitor pattern method for DbComparisonExpression. /// /// The DbComparisonExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbComparisonExpression expression); ////// Typed visitor pattern method for DbConstantExpression. /// /// The DbConstantExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbConstantExpression expression); ////// Typed visitor pattern method for DbCrossJoinExpression. /// /// The DbCrossJoinExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbCrossJoinExpression expression); ////// Visitor pattern method for DbDerefExpression. /// /// The DbDerefExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbDerefExpression expression); ////// Typed visitor pattern method for DbDistinctExpression. /// /// The DbDistinctExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbDistinctExpression expression); ////// Typed visitor pattern method for DbElementExpression. /// /// The DbElementExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbElementExpression expression); ////// Typed visitor pattern method for DbExceptExpression. /// /// The DbExceptExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbExceptExpression expression); ////// Typed visitor pattern method for DbFilterExpression. /// /// The DbFilterExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbFilterExpression expression); ////// Visitor pattern method for DbFunctionExpression /// /// The DbFunctionExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbFunctionExpression expression); ////// Visitor pattern method for DbEntityRefExpression. /// /// The DbEntityRefExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbEntityRefExpression expression); ////// Visitor pattern method for DbRefKeyExpression. /// /// The DbRefKeyExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbRefKeyExpression expression); ////// Typed visitor pattern method for DbGroupByExpression. /// /// The DbGroupByExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbGroupByExpression expression); ////// Typed visitor pattern method for DbIntersectExpression. /// /// The DbIntersectExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbIntersectExpression expression); ////// Typed visitor pattern method for DbIsEmptyExpression. /// /// The DbIsEmptyExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbIsEmptyExpression expression); ////// Typed visitor pattern method for DbIsNullExpression. /// /// The DbIsNullExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbIsNullExpression expression); ////// Typed visitor pattern method for DbIsOfExpression. /// /// The DbIsOfExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbIsOfExpression expression); ////// Typed visitor pattern method for DbJoinExpression. /// /// The DbJoinExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbJoinExpression expression); ////// Visitor pattern method for DbLambdaExpression. /// /// The DbLambdaExpression that is being visited. ///An instance of TResultType. public virtual TResultType Visit(DbLambdaExpression expression) { throw EntityUtil.NotSupported(); } ////// Visitor pattern method for DbLikeExpression. /// /// The DbLikeExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbLikeExpression expression); ////// Visitor pattern method for DbLimitExpression. /// /// The DbLimitExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbLimitExpression expression); #if METHOD_EXPRESSION ////// Typed visitor pattern method for MethodExpression. /// /// The Expression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(MethodExpression expression); #endif ////// Typed visitor pattern method for DbNewInstanceExpression. /// /// The DbNewInstanceExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbNewInstanceExpression expression); ////// Typed visitor pattern method for DbNotExpression. /// /// The DbNotExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbNotExpression expression); ////// Typed visitor pattern method for DbNullExpression. /// /// The DbNullExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbNullExpression expression); ////// Typed visitor pattern method for DbOfTypeExpression. /// /// The DbOfTypeExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbOfTypeExpression expression); ////// Typed visitor pattern method for DbOrExpression. /// /// The DbOrExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbOrExpression expression); ////// Typed visitor pattern method for DbParameterReferenceExpression. /// /// The DbParameterReferenceExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbParameterReferenceExpression expression); ////// Typed visitor pattern method for DbProjectExpression. /// /// The DbProjectExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbProjectExpression expression); ////// Typed visitor pattern method for DbPropertyExpression. /// /// The DbPropertyExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbPropertyExpression expression); ////// Typed visitor pattern method for DbQuantifierExpression. /// /// The DbQuantifierExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbQuantifierExpression expression); ////// Typed visitor pattern method for DbRefExpression. /// /// The DbRefExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbRefExpression expression); ////// Typed visitor pattern method for DbRelationshipNavigationExpression. /// /// The DbRelationshipNavigationExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbRelationshipNavigationExpression expression); ////// Typed visitor pattern method for DbScanExpression. /// /// The DbScanExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbScanExpression expression); ////// Typed visitor pattern method for DbSortExpression. /// /// The DbSortExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbSortExpression expression); ////// Typed visitor pattern method for DbSkipExpression. /// /// The DbSkipExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbSkipExpression expression); ////// Typed visitor pattern method for DbTreatExpression. /// /// The DbTreatExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbTreatExpression expression); ////// Typed visitor pattern method for DbUnionAllExpression. /// /// The DbUnionAllExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbUnionAllExpression expression); ////// Typed visitor pattern method for DbVariableReferenceExpression. /// /// The DbVariableReferenceExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbVariableReferenceExpression expression); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Metadata.Edm; namespace System.Data.Common.CommandTrees { ////// The expression visitor pattern abstract base class that should be implemented by visitors that return a result value of a specific type. /// ///The type of the result value produced by the visitor. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbExpressionVisitor{ /// /// Called when an expression of an otherwise unrecognized type is encountered. /// /// The expression. public abstract TResultType Visit(DbExpression expression); ////// Typed visitor pattern method for DbAndExpression. /// /// The DbAndExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbAndExpression expression); ////// Typed visitor pattern method for DbApplyExpression. /// /// The DbApplyExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbApplyExpression expression); ////// Typed visitor pattern method for DbArithmeticExpression. /// /// The DbArithmeticExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbArithmeticExpression expression); ////// Typed visitor pattern method for DbCaseExpression. /// /// The DbCaseExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbCaseExpression expression); ////// Typed visitor pattern method for DbCastExpression. /// /// The DbCastExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbCastExpression expression); ////// Typed visitor pattern method for DbComparisonExpression. /// /// The DbComparisonExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbComparisonExpression expression); ////// Typed visitor pattern method for DbConstantExpression. /// /// The DbConstantExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbConstantExpression expression); ////// Typed visitor pattern method for DbCrossJoinExpression. /// /// The DbCrossJoinExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbCrossJoinExpression expression); ////// Visitor pattern method for DbDerefExpression. /// /// The DbDerefExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbDerefExpression expression); ////// Typed visitor pattern method for DbDistinctExpression. /// /// The DbDistinctExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbDistinctExpression expression); ////// Typed visitor pattern method for DbElementExpression. /// /// The DbElementExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbElementExpression expression); ////// Typed visitor pattern method for DbExceptExpression. /// /// The DbExceptExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbExceptExpression expression); ////// Typed visitor pattern method for DbFilterExpression. /// /// The DbFilterExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbFilterExpression expression); ////// Visitor pattern method for DbFunctionExpression /// /// The DbFunctionExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbFunctionExpression expression); ////// Visitor pattern method for DbEntityRefExpression. /// /// The DbEntityRefExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbEntityRefExpression expression); ////// Visitor pattern method for DbRefKeyExpression. /// /// The DbRefKeyExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbRefKeyExpression expression); ////// Typed visitor pattern method for DbGroupByExpression. /// /// The DbGroupByExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbGroupByExpression expression); ////// Typed visitor pattern method for DbIntersectExpression. /// /// The DbIntersectExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbIntersectExpression expression); ////// Typed visitor pattern method for DbIsEmptyExpression. /// /// The DbIsEmptyExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbIsEmptyExpression expression); ////// Typed visitor pattern method for DbIsNullExpression. /// /// The DbIsNullExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbIsNullExpression expression); ////// Typed visitor pattern method for DbIsOfExpression. /// /// The DbIsOfExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbIsOfExpression expression); ////// Typed visitor pattern method for DbJoinExpression. /// /// The DbJoinExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbJoinExpression expression); ////// Visitor pattern method for DbLambdaExpression. /// /// The DbLambdaExpression that is being visited. ///An instance of TResultType. public virtual TResultType Visit(DbLambdaExpression expression) { throw EntityUtil.NotSupported(); } ////// Visitor pattern method for DbLikeExpression. /// /// The DbLikeExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbLikeExpression expression); ////// Visitor pattern method for DbLimitExpression. /// /// The DbLimitExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbLimitExpression expression); #if METHOD_EXPRESSION ////// Typed visitor pattern method for MethodExpression. /// /// The Expression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(MethodExpression expression); #endif ////// Typed visitor pattern method for DbNewInstanceExpression. /// /// The DbNewInstanceExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbNewInstanceExpression expression); ////// Typed visitor pattern method for DbNotExpression. /// /// The DbNotExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbNotExpression expression); ////// Typed visitor pattern method for DbNullExpression. /// /// The DbNullExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbNullExpression expression); ////// Typed visitor pattern method for DbOfTypeExpression. /// /// The DbOfTypeExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbOfTypeExpression expression); ////// Typed visitor pattern method for DbOrExpression. /// /// The DbOrExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbOrExpression expression); ////// Typed visitor pattern method for DbParameterReferenceExpression. /// /// The DbParameterReferenceExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbParameterReferenceExpression expression); ////// Typed visitor pattern method for DbProjectExpression. /// /// The DbProjectExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbProjectExpression expression); ////// Typed visitor pattern method for DbPropertyExpression. /// /// The DbPropertyExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbPropertyExpression expression); ////// Typed visitor pattern method for DbQuantifierExpression. /// /// The DbQuantifierExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbQuantifierExpression expression); ////// Typed visitor pattern method for DbRefExpression. /// /// The DbRefExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbRefExpression expression); ////// Typed visitor pattern method for DbRelationshipNavigationExpression. /// /// The DbRelationshipNavigationExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbRelationshipNavigationExpression expression); ////// Typed visitor pattern method for DbScanExpression. /// /// The DbScanExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbScanExpression expression); ////// Typed visitor pattern method for DbSortExpression. /// /// The DbSortExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbSortExpression expression); ////// Typed visitor pattern method for DbSkipExpression. /// /// The DbSkipExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbSkipExpression expression); ////// Typed visitor pattern method for DbTreatExpression. /// /// The DbTreatExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbTreatExpression expression); ////// Typed visitor pattern method for DbUnionAllExpression. /// /// The DbUnionAllExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbUnionAllExpression expression); ////// Typed visitor pattern method for DbVariableReferenceExpression. /// /// The DbVariableReferenceExpression that is being visited. ///An instance of TResultType. public abstract TResultType Visit(DbVariableReferenceExpression expression); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
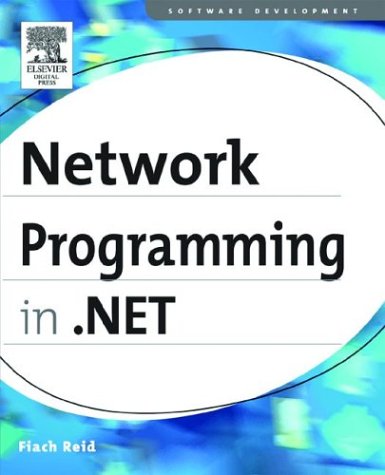
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeNodeCollection.cs
- CriticalExceptions.cs
- Container.cs
- MissingFieldException.cs
- TraceProvider.cs
- SkewTransform.cs
- CollectionViewProxy.cs
- UnsafeNativeMethods.cs
- GenericEnumerator.cs
- SecurityKeyType.cs
- ToolStripMenuItem.cs
- DragAssistanceManager.cs
- AppSettingsExpressionBuilder.cs
- TextTreeRootTextBlock.cs
- BufferedReadStream.cs
- CallbackHandler.cs
- LinearGradientBrush.cs
- ByteKeyFrameCollection.cs
- RepeaterCommandEventArgs.cs
- ClientRoleProvider.cs
- ConsumerConnectionPoint.cs
- EventEntry.cs
- CompatibleComparer.cs
- RegistrationServices.cs
- Point3D.cs
- Control.cs
- WebPartZone.cs
- TransactionFlowElement.cs
- RelativeSource.cs
- BulletedList.cs
- ConsumerConnectionPoint.cs
- FileUtil.cs
- Accessible.cs
- Base64Encoder.cs
- XmlDataLoader.cs
- ApplicationBuildProvider.cs
- Size3D.cs
- Registry.cs
- SerializationStore.cs
- DataGridAutoGeneratingColumnEventArgs.cs
- PixelFormatConverter.cs
- ArrayList.cs
- TemplateEditingFrame.cs
- GPRECT.cs
- SqlColumnizer.cs
- RegexNode.cs
- HttpModuleActionCollection.cs
- _SingleItemRequestCache.cs
- XmlArrayAttribute.cs
- FileSystemInfo.cs
- CurrencyManager.cs
- HtmlTableRow.cs
- Transform3DCollection.cs
- _DisconnectOverlappedAsyncResult.cs
- TabItemAutomationPeer.cs
- PagerSettings.cs
- Renderer.cs
- ReturnEventArgs.cs
- StrokeCollectionDefaultValueFactory.cs
- WebPartDescription.cs
- TextServicesDisplayAttribute.cs
- ReadOnlyDictionary.cs
- SqlStatistics.cs
- ComponentDispatcher.cs
- IssuerInformation.cs
- NameScopePropertyAttribute.cs
- CapabilitiesState.cs
- CngKey.cs
- WindowsFont.cs
- OutputWindow.cs
- KeyEvent.cs
- IPipelineRuntime.cs
- XmlSignificantWhitespace.cs
- MultipleViewProviderWrapper.cs
- XamlWrapperReaders.cs
- cookie.cs
- PostBackOptions.cs
- ValidationRuleCollection.cs
- WebPartDescription.cs
- Tokenizer.cs
- DataGridViewUtilities.cs
- CustomValidator.cs
- Receive.cs
- WorkflowDebuggerSteppingAttribute.cs
- BevelBitmapEffect.cs
- TextBox.cs
- TraceXPathNavigator.cs
- RegexWorker.cs
- SortFieldComparer.cs
- IsolatedStoragePermission.cs
- BuildManagerHost.cs
- XmlQueryTypeFactory.cs
- SslStreamSecurityUpgradeProvider.cs
- NameTable.cs
- HttpConfigurationContext.cs
- CharAnimationBase.cs
- WebPartVerbCollection.cs
- XmlLoader.cs
- CrossAppDomainChannel.cs
- CheckBoxList.cs