Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Data / System / Data / Common / System.Data_BID.cs / 6 / System.Data_BID.cs
//------------------------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------------------------- using System; using System.Text; using System.Security; using System.Reflection; using System.Security.Permissions; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; [module: BidIdentity("System.Data.1")] [module: BidMetaText(":FormatControl: InstanceID='' ")] [module: BidMetaText("Trace=1200; Scope=250;")] [module: BidMetaText(" ds = System.Data;" + "comm = System.Data.Common;" + "odbc = System.Data.Odbc;" + "oledb= System.Data.OleDb;" + "prov = System.Data.ProviderBase;" + "sc = System.Data.Sql;" + "sql = System.Data.SqlClient;" + "cqt = System.Data.Common.CommandTrees;" + "cqti = System.Data.Common.CommandTrees.Internal;" + "esql = System.Data.Common.EntitySql;" + "ec = System.Data.EntityClient;" + "dobj = System.Data.Objects;" + "md = System.Data.Metadata;" + "ra = System.Data.Query.ResultAssembly;" + "pc = System.Data.Query.PlanCompiler;" + "iqt = System.Data.Query.InternalTrees;" + "mp = System.Data.Mapping;" + "upd = System.Data.Mapping.Update;" + "vgen = System.Data.Mapping.ViewGeneration;" )] // // DbConnectionPool.cs: const Bid.ApiGroup PoolerTracePoints // [module: BidMetaText(" 0x00001000: Connection Pooling")] // // SqlDependency.cs: const Bid.ApiGroup NotificationsTracePoints // [module: BidMetaText(" 0x00002000: SqlDependency Notifications")] // // System\Data\Query\Bridge\IteratorSource.cs: internal const Bid.ApiGroup ResultAssemblyTracePoints // [module: BidMetaText(" 0x00004000: Result Assembly")] // // System\Data\Query\PlanCompiler\PlanCompiler.cs: internal const Bid.ApiGroup PlanCompilerTracePoints // [module: BidMetaText(" 0x00008000: Plan Compilation")] internal static partial class Bid { #if WINFSFunctionality private const string dllName = "DWPriv.dll"; #else private const string dllName = "System.Data.dll"; #endif // // Manually added wrappers // [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2); } internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3); } internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4); } internal static void PoolerScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, string fmtPrintfW2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, fmtPrintfW2); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, string fmtPrintfW2, string fmtPrintfW3) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, fmtPrintfW2, fmtPrintfW3); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, string fmtPrintfW2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, fmtPrintfW2); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, a2); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, string fmtPrintfW2, string fmtPrintfW3, string fmtPrintfW4) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, fmtPrintfW2, fmtPrintfW3, fmtPrintfW4); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, string fmtPrintfW2, string fmtPrintfW3) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, fmtPrintfW2, fmtPrintfW3); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, string fmtPrintfW2, System.Int32 a2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, fmtPrintfW2, a2); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, string fmtPrintfW2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, a2, fmtPrintfW2); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, string fmtPrintfW2, string fmtPrintfW3, System.Int32 a4) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, fmtPrintfW2, fmtPrintfW3, a4); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, string fmtPrintfW2, string fmtPrintfW3, string fmtPrintfW4, System.Int32 a5) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, fmtPrintfW2, fmtPrintfW3, fmtPrintfW4, a5); } else { hScp = NoData; } } internal static void NotificationsTrace(string fmtPrintfW) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW); } internal static void NotificationsTrace(string fmtPrintfW, string fmtPrintfW2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, fmtPrintfW2); } internal static void NotificationsTrace(string fmtPrintfW, System.Int32 a1) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } internal static void NotificationsTrace(string fmtPrintfW, System.Boolean a1) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } internal static void NotificationsTrace(string fmtPrintfW, string fmtPrintfW2, System.Int32 a1) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, fmtPrintfW2, a1); } internal static void NotificationsTrace(string fmtPrintfW, System.Int32 a1, string fmtPrintfW2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, fmtPrintfW2); } internal static void NotificationsTrace(string fmtPrintfW, System.Boolean a1, string fmtPrintfW2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, fmtPrintfW2); } internal static void NotificationsTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } internal static void NotificationsTrace(string fmtPrintfW, System.Boolean a1, System.Int32 a2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } internal static void NotificationsTrace(string fmtPrintfW, System.Int32 a1, System.Boolean a2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } internal static void NotificationsTrace(string fmtPrintfW, string fmtPrintfW2, string fmtPrintfW3, System.Int32 a1) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, fmtPrintfW2, fmtPrintfW3, a1); } internal static void NotificationsTrace(string fmtPrintfW, System.Boolean a1, string fmtPrintfW2, string fmtPrintfW3, string fmtPrintfW4) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, fmtPrintfW2, fmtPrintfW3, fmtPrintfW4); } internal static void NotificationsTrace(string fmtPrintfW, System.Int32 a1, string fmtPrintfW2, string fmtPrintfW3, string fmtPrintfW4) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, fmtPrintfW2, fmtPrintfW3, fmtPrintfW4); } /* internal static void NotificationsTrace(string fmtPrintfW, System.Guid a2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a2); } */ // // Manually edited wrappers // [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void TraceSqlReturn(string fmtPrintfW, System.Data.Odbc.ODBC32.RetCode a1) { if (((System.Data.Odbc.ODBC32.RetCode.SUCCESS != a1) || (modFlags & ApiGroup.StatusOk) != 0) && (modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)(short)a1); } internal static void TraceSqlReturn(string fmtPrintfW, System.Data.Odbc.ODBC32.RetCode a1, string a2) { if (((System.Data.Odbc.ODBC32.RetCode.SUCCESS != a1) || (modFlags & ApiGroup.StatusOk) != 0) && (modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)(short)a1, a2); } internal static void TraceSqlReturn(string fmtPrintfW, System.Data.Odbc.ODBC32.RetCode a1, string a2, string a3) { if (((System.Data.Odbc.ODBC32.RetCode.SUCCESS != a1) || (modFlags & ApiGroup.StatusOk) != 0) && (modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)(short)a1, a2, a3); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.Data.OleDb.OleDbHResult a1) { // if (((System.Data.OleDb.OleDbHResult.S_OK != a1) || (modFlags & ApiGroup.StatusOk) != 0) && (modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)a1); } internal static void Trace(string fmtPrintfW, System.Data.OleDb.OleDbHResult a1, System.String a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)a1,a2); } internal static void Trace(string fmtPrintfW, System.Data.OleDb.OleDbHResult a1, System.IntPtr a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)a1,a2); } internal static void Trace(string fmtPrintfW, System.Data.OleDb.OleDbHResult a1, System.Int32 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)a1,a2); } internal static void Trace(string fmtPrintfW, System.String a1, System.String a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.Boolean a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3, System.String a4, System.Int32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int64 a3, System.UInt32 a4, System.Int32 a5, System.UInt32 a6, System.UInt32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7); } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Guid a2) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1, a2); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Boolean a2, System.Int32 a3) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3); } else { hScp = NoData; } } // // Trace overloads // [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.IntPtr a1) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1); } internal static void Trace(string fmtPrintfW, System.Int32 a1) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.IntPtr a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.IntPtr a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.Int32 a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.String a4, System.String a5, System.Int32 a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4,a5,a6); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Boolean a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Boolean a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4, System.Int32 a5, System.Int32 a6, System.Int32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4,a5,a6,a7); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3, System.Int32 a4, System.Boolean a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4,a5); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int64 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int64 a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3 ); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.String a4, System.Int32 a5, System.Int64 a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4,a5,a6); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int64 a2, System.Int32 a3, System.Int32 a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int64 a3, System.Int32 a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4, System.String a5, System.String a6, System.String a7, System.Int32 a8) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4,a5,a6,a7,a8); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3, System.String a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4); } // // ScopeEnter overloads // internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Boolean a2) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.Boolean a3) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Boolean a3) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.String a4) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3,a4); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Boolean a3, System.Int32 a4) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3,a4); } else { hScp = NoData; } } // // Interop calls to pluggable hooks [SuppressUnmanagedCodeSecurity] applied // private static partial class NativeMethods { // // Manually edited wrappers // [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3, System.String a4, System.Int32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.Boolean a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int64 a3, System.UInt32 a4, System.Int32 a5, System.UInt32 a6, System.UInt32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.String a1, System.String a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, [In, MarshalAs(UnmanagedType.LPStruct)] Guid a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.String a1, System.String a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Boolean a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.String a1, System.String a2, System.String a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.Int32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.String a4, System.Int32 a5); // // Trace // [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Boolean a1); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, string fmtPrintfW2, System.Int32 a1); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Boolean a1, string fmtPrintfW2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Boolean a1, System.Int32 a2); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.IntPtr a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.IntPtr a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.Int32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.String a4, System.String a5, System.Int32 a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Boolean a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.String a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Boolean a1, System.String a2, System.String a3, System.String a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Boolean a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4, System.Int32 a5, System.Int32 a6, System.Int32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3, System.Int32 a4, System.Boolean a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int64 a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int64 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW1, string fmtPrintfW2, string fmtPrintfW3, System.Int64 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.String a4, System.Int32 a5, System.Int64 a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int64 a2, System.Int32 a3, System.Int32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int64 a3, System.Int32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4, System.String a5, System.String a6, System.String a7, System.Int32 a8); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3, System.String a4); // // ScopeEnter // [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Boolean a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.Boolean a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Boolean a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.String a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Boolean a3, System.Int32 a4); } // Native } // Bid // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------------------------- using System; using System.Text; using System.Security; using System.Reflection; using System.Security.Permissions; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; [module: BidIdentity("System.Data.1")] [module: BidMetaText(":FormatControl: InstanceID='' ")] [module: BidMetaText("Trace=1200; Scope=250;")] [module: BidMetaText(" ds = System.Data;" + "comm = System.Data.Common;" + "odbc = System.Data.Odbc;" + "oledb= System.Data.OleDb;" + "prov = System.Data.ProviderBase;" + "sc = System.Data.Sql;" + "sql = System.Data.SqlClient;" + "cqt = System.Data.Common.CommandTrees;" + "cqti = System.Data.Common.CommandTrees.Internal;" + "esql = System.Data.Common.EntitySql;" + "ec = System.Data.EntityClient;" + "dobj = System.Data.Objects;" + "md = System.Data.Metadata;" + "ra = System.Data.Query.ResultAssembly;" + "pc = System.Data.Query.PlanCompiler;" + "iqt = System.Data.Query.InternalTrees;" + "mp = System.Data.Mapping;" + "upd = System.Data.Mapping.Update;" + "vgen = System.Data.Mapping.ViewGeneration;" )] // // DbConnectionPool.cs: const Bid.ApiGroup PoolerTracePoints // [module: BidMetaText(" 0x00001000: Connection Pooling")] // // SqlDependency.cs: const Bid.ApiGroup NotificationsTracePoints // [module: BidMetaText(" 0x00002000: SqlDependency Notifications")] // // System\Data\Query\Bridge\IteratorSource.cs: internal const Bid.ApiGroup ResultAssemblyTracePoints // [module: BidMetaText(" 0x00004000: Result Assembly")] // // System\Data\Query\PlanCompiler\PlanCompiler.cs: internal const Bid.ApiGroup PlanCompilerTracePoints // [module: BidMetaText(" 0x00008000: Plan Compilation")] internal static partial class Bid { #if WINFSFunctionality private const string dllName = "DWPriv.dll"; #else private const string dllName = "System.Data.dll"; #endif // // Manually added wrappers // [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2); } internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3); } internal static void PoolerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4); } internal static void PoolerScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1) { if ((modFlags & System.Data.ProviderBase.DbConnectionPool.PoolerTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, string fmtPrintfW2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, fmtPrintfW2); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, string fmtPrintfW2, string fmtPrintfW3) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, fmtPrintfW2, fmtPrintfW3); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, string fmtPrintfW2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, fmtPrintfW2); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, a2); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, string fmtPrintfW2, string fmtPrintfW3, string fmtPrintfW4) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, fmtPrintfW2, fmtPrintfW3, fmtPrintfW4); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, string fmtPrintfW2, string fmtPrintfW3) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, fmtPrintfW2, fmtPrintfW3); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, string fmtPrintfW2, System.Int32 a2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, fmtPrintfW2, a2); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, string fmtPrintfW2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, a2, fmtPrintfW2); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, string fmtPrintfW2, string fmtPrintfW3, System.Int32 a4) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, fmtPrintfW2, fmtPrintfW3, a4); } else { hScp = NoData; } } internal static void NotificationsScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, string fmtPrintfW2, string fmtPrintfW3, string fmtPrintfW4, System.Int32 a5) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, fmtPrintfW2, fmtPrintfW3, fmtPrintfW4, a5); } else { hScp = NoData; } } internal static void NotificationsTrace(string fmtPrintfW) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW); } internal static void NotificationsTrace(string fmtPrintfW, string fmtPrintfW2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, fmtPrintfW2); } internal static void NotificationsTrace(string fmtPrintfW, System.Int32 a1) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } internal static void NotificationsTrace(string fmtPrintfW, System.Boolean a1) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } internal static void NotificationsTrace(string fmtPrintfW, string fmtPrintfW2, System.Int32 a1) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, fmtPrintfW2, a1); } internal static void NotificationsTrace(string fmtPrintfW, System.Int32 a1, string fmtPrintfW2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, fmtPrintfW2); } internal static void NotificationsTrace(string fmtPrintfW, System.Boolean a1, string fmtPrintfW2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, fmtPrintfW2); } internal static void NotificationsTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } internal static void NotificationsTrace(string fmtPrintfW, System.Boolean a1, System.Int32 a2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } internal static void NotificationsTrace(string fmtPrintfW, System.Int32 a1, System.Boolean a2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } internal static void NotificationsTrace(string fmtPrintfW, string fmtPrintfW2, string fmtPrintfW3, System.Int32 a1) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, fmtPrintfW2, fmtPrintfW3, a1); } internal static void NotificationsTrace(string fmtPrintfW, System.Boolean a1, string fmtPrintfW2, string fmtPrintfW3, string fmtPrintfW4) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, fmtPrintfW2, fmtPrintfW3, fmtPrintfW4); } internal static void NotificationsTrace(string fmtPrintfW, System.Int32 a1, string fmtPrintfW2, string fmtPrintfW3, string fmtPrintfW4) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, fmtPrintfW2, fmtPrintfW3, fmtPrintfW4); } /* internal static void NotificationsTrace(string fmtPrintfW, System.Guid a2) { if ((modFlags & System.Data.SqlClient.SqlDependency.NotificationsTracePoints) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a2); } */ // // Manually edited wrappers // [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void TraceSqlReturn(string fmtPrintfW, System.Data.Odbc.ODBC32.RetCode a1) { if (((System.Data.Odbc.ODBC32.RetCode.SUCCESS != a1) || (modFlags & ApiGroup.StatusOk) != 0) && (modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)(short)a1); } internal static void TraceSqlReturn(string fmtPrintfW, System.Data.Odbc.ODBC32.RetCode a1, string a2) { if (((System.Data.Odbc.ODBC32.RetCode.SUCCESS != a1) || (modFlags & ApiGroup.StatusOk) != 0) && (modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)(short)a1, a2); } internal static void TraceSqlReturn(string fmtPrintfW, System.Data.Odbc.ODBC32.RetCode a1, string a2, string a3) { if (((System.Data.Odbc.ODBC32.RetCode.SUCCESS != a1) || (modFlags & ApiGroup.StatusOk) != 0) && (modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)(short)a1, a2, a3); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.Data.OleDb.OleDbHResult a1) { // if (((System.Data.OleDb.OleDbHResult.S_OK != a1) || (modFlags & ApiGroup.StatusOk) != 0) && (modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)a1); } internal static void Trace(string fmtPrintfW, System.Data.OleDb.OleDbHResult a1, System.String a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)a1,a2); } internal static void Trace(string fmtPrintfW, System.Data.OleDb.OleDbHResult a1, System.IntPtr a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)a1,a2); } internal static void Trace(string fmtPrintfW, System.Data.OleDb.OleDbHResult a1, System.Int32 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, (int)a1,a2); } internal static void Trace(string fmtPrintfW, System.String a1, System.String a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.Boolean a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3, System.String a4, System.Int32 a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int64 a3, System.UInt32 a4, System.Int32 a5, System.UInt32 a6, System.UInt32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1,a2,a3,a4,a5,a6,a7); } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Guid a2) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1, a2); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Boolean a2, System.Int32 a3) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3); } else { hScp = NoData; } } // // Trace overloads // [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static void Trace(string fmtPrintfW, System.IntPtr a1) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1); } internal static void Trace(string fmtPrintfW, System.Int32 a1) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.IntPtr a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.IntPtr a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.Int32 a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.String a4, System.String a5, System.Int32 a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4,a5,a6); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Boolean a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Boolean a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4, System.Int32 a5, System.Int32 a6, System.Int32 a7) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4,a5,a6,a7); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3, System.Int32 a4, System.Boolean a5) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4,a5); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int64 a2) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int64 a3) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3 ); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.String a4, System.Int32 a5, System.Int64 a6) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4,a5,a6); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int64 a2, System.Int32 a3, System.Int32 a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int64 a3, System.Int32 a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4, System.String a5, System.String a6, System.String a7, System.Int32 a8) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4,a5,a6,a7,a8); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3, System.String a4) { if ((modFlags & ApiGroup.Trace) != 0 && modID != NoData) NativeMethods.Trace (modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW,a1,a2,a3,a4); } // // ScopeEnter overloads // internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Boolean a2) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.Boolean a3) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Boolean a3) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.String a4) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3,a4); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3); } else { hScp = NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Boolean a3, System.Int32 a4) { if ((modFlags & ApiGroup.Scope) != 0 && modID != NoData) { NativeMethods.ScopeEnter (modID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW,a1,a2,a3,a4); } else { hScp = NoData; } } // // Interop calls to pluggable hooks [SuppressUnmanagedCodeSecurity] applied // private static partial class NativeMethods { // // Manually edited wrappers // [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3, System.String a4, System.Int32 a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.Boolean a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int64 a3, System.UInt32 a4, System.Int32 a5, System.UInt32 a6, System.UInt32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.String a1, System.String a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, [In, MarshalAs(UnmanagedType.LPStruct)] Guid a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.String a1, System.String a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Boolean a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.String a1, System.String a2, System.String a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.Int32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.String a4, System.Int32 a5); // // Trace // [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.IntPtr a1); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Boolean a1); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, string fmtPrintfW2, System.Int32 a1); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Boolean a1, string fmtPrintfW2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Boolean a1, System.Int32 a2); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.IntPtr a2, System.IntPtr a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.IntPtr a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.Int32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.String a4, System.String a5, System.Int32 a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Boolean a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.String a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Boolean a1, System.String a2, System.String a3, System.String a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Boolean a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4, System.Int32 a5, System.Int32 a6, System.Int32 a7); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.Int32 a3, System.Int32 a4, System.Boolean a5); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int64 a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int64 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW1, string fmtPrintfW2, string fmtPrintfW3, System.Int64 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3, System.String a4, System.Int32 a5, System.Int64 a6); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int64 a2, System.Int32 a3, System.Int32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int64 a3, System.Int32 a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4, System.String a5, System.String a6, System.String a7, System.Int32 a8); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidTraceCW")] extern internal static void Trace (IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3, System.String a4); // // ScopeEnter // [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Boolean a2); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.String a2, System.Boolean a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Boolean a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.String a4); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3); [DllImport(dllName, CharSet=CharSet.Unicode, CallingConvention=CallingConvention.Cdecl, EntryPoint="DllBidScopeEnterCW")] extern internal static void ScopeEnter (IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Boolean a3, System.Int32 a4); } // Native } // Bid // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
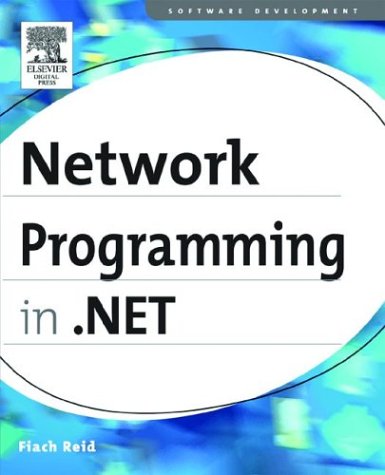
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolboxItemSnapLineBehavior.cs
- TreeWalker.cs
- HttpProfileGroupBase.cs
- GPRECT.cs
- RsaSecurityToken.cs
- UniqueConstraint.cs
- TextParagraphProperties.cs
- HwndMouseInputProvider.cs
- SourceFileBuildProvider.cs
- SharedPerformanceCounter.cs
- KeyNotFoundException.cs
- EventDescriptor.cs
- X509KeyIdentifierClauseType.cs
- ExecutionContext.cs
- Line.cs
- TemplatedMailWebEventProvider.cs
- CqlParserHelpers.cs
- Compilation.cs
- ClientCultureInfo.cs
- SoapSchemaMember.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- MatrixTransform.cs
- ListBoxItem.cs
- IResourceProvider.cs
- UriTemplateTableMatchCandidate.cs
- SerializationFieldInfo.cs
- RegularExpressionValidator.cs
- TypeUtil.cs
- OutputScopeManager.cs
- XmlElementAttribute.cs
- ReadOnlyCollection.cs
- MultiplexingFormatMapping.cs
- DesignerTextViewAdapter.cs
- SqlStream.cs
- WindowsAuthenticationModule.cs
- WinCategoryAttribute.cs
- ConfigurationManagerHelper.cs
- PointLightBase.cs
- SqlDataSourceQueryEditor.cs
- AuthenticateEventArgs.cs
- ArrayConverter.cs
- MessageQueueTransaction.cs
- DispatchWrapper.cs
- ArgumentException.cs
- ObjectConverter.cs
- IconHelper.cs
- CoreSwitches.cs
- WorkflowViewStateService.cs
- SafeFileMappingHandle.cs
- SmiSettersStream.cs
- NamedPermissionSet.cs
- XmlUnspecifiedAttribute.cs
- DataViewManagerListItemTypeDescriptor.cs
- updatecommandorderer.cs
- Task.cs
- XPathDocumentIterator.cs
- RoutedCommand.cs
- LabelDesigner.cs
- Win32.cs
- DetailsViewModeEventArgs.cs
- SystemUnicastIPAddressInformation.cs
- Utils.cs
- EventLogger.cs
- RegexParser.cs
- MetadataException.cs
- NullExtension.cs
- InputLanguageSource.cs
- SafeThreadHandle.cs
- DecimalConverter.cs
- NativeMethods.cs
- FileInfo.cs
- _OverlappedAsyncResult.cs
- ManifestResourceInfo.cs
- Brushes.cs
- EntitySqlQueryBuilder.cs
- CustomErrorCollection.cs
- TextTrailingWordEllipsis.cs
- OutputCacheProfileCollection.cs
- ToolStripRenderer.cs
- XmlSignatureManifest.cs
- SqlRewriteScalarSubqueries.cs
- TdsRecordBufferSetter.cs
- ServicesUtilities.cs
- HealthMonitoringSectionHelper.cs
- Track.cs
- FlowPosition.cs
- DetailsViewInsertedEventArgs.cs
- HtmlTextArea.cs
- EmissiveMaterial.cs
- ModifiableIteratorCollection.cs
- TrackBar.cs
- mediaeventshelper.cs
- ProtocolsConfiguration.cs
- NullableLongMinMaxAggregationOperator.cs
- RuleAction.cs
- TabControl.cs
- DateTimeStorage.cs
- SqlInfoMessageEvent.cs
- WebBrowserDocumentCompletedEventHandler.cs
- ObjectDataSourceStatusEventArgs.cs