Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Configuration / System / Configuration / TypeUtil.cs / 1305376 / TypeUtil.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Configuration.Internal; using System.Reflection; using System.Security; using System.Security.Permissions; namespace System.Configuration { internal static class TypeUtil { // // Since the config APIs were originally implemented in System.dll, // references to types without assembly names could be resolved to // System.dll in Everett. Emulate that behavior by trying to get the // type from System.dll // static private Type GetLegacyType(string typeString) { Type type = null; // // Ignore all exceptions, otherwise callers will get unexpected // exceptions not related to the original failure to load the // desired type. // try { Assembly systemAssembly = typeof(ConfigurationException).Assembly; type = systemAssembly.GetType(typeString, false); } catch { } return type; } // // Get the type specified by typeString. If it fails, try to retrieve it // as a type from System.dll. If that fails, return null or throw the original // exception as indicated by throwOnError. // static private Type GetTypeImpl(string typeString, bool throwOnError) { Type type = null; Exception originalException = null; try { type = Type.GetType(typeString, throwOnError); } catch (Exception e) { originalException = e; } if (type == null) { type = GetLegacyType(typeString); if (type == null && originalException != null) { throw originalException; } } return type; } // // Ask the host to get the type specified by typeString. If it fails, try to retrieve it // as a type from System.dll. If that fails, return null or throw the original // exception as indicated by throwOnError. // [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags)] static internal Type GetTypeWithReflectionPermission(IInternalConfigHost host, string typeString, bool throwOnError) { Type type = null; Exception originalException = null; try { type = host.GetConfigType(typeString, throwOnError); } catch (Exception e) { originalException = e; } if (type == null) { type = GetLegacyType(typeString); if (type == null && originalException != null) { throw originalException; } } return type; } [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags)] static internal Type GetTypeWithReflectionPermission(string typeString, bool throwOnError) { return GetTypeImpl(typeString, throwOnError); } [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags | ReflectionPermissionFlag.MemberAccess)] static internal object CreateInstanceWithReflectionPermission(string typeString) { Type type = GetTypeImpl(typeString, true); // catch the errors and report them object result = Activator.CreateInstance(type, true); // create non-public types return result; } [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags | ReflectionPermissionFlag.MemberAccess)] static internal object CreateInstanceWithReflectionPermission(Type type) { object result = Activator.CreateInstance(type, true); // create non-public types return result; } [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags)] static internal ConstructorInfo GetConstructorWithReflectionPermission(Type type, Type baseType, bool throwOnError) { type = VerifyAssignableType(baseType, type, throwOnError); if (type == null) { return null; } BindingFlags bindingFlags = BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic; ConstructorInfo ctor = type.GetConstructor(bindingFlags, null, CallingConventions.HasThis, Type.EmptyTypes, null); if (ctor == null && throwOnError) { throw new TypeLoadException(SR.GetString(SR.TypeNotPublic, type.AssemblyQualifiedName)); } return ctor; } [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags | ReflectionPermissionFlag.MemberAccess)] static internal object InvokeCtorWithReflectionPermission(ConstructorInfo ctor) { return ctor.Invoke(null); } static internal bool IsTypeFromTrustedAssemblyWithoutAptca(Type type) { Assembly assembly = type.Assembly; return assembly.GlobalAssemblyCache && !HasAptcaBit(assembly); } static internal Type VerifyAssignableType(Type baseType, Type type, bool throwOnError) { if (baseType.IsAssignableFrom(type)) { return type; } if (throwOnError) { throw new TypeLoadException( SR.GetString(SR.Config_type_doesnt_inherit_from_type, type.FullName, baseType.FullName)); } return null; } [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags | ReflectionPermissionFlag.MemberAccess)] private static bool HasAptcaBit(Assembly assembly) { Object[] attrs = assembly.GetCustomAttributes( typeof(System.Security.AllowPartiallyTrustedCallersAttribute), /*inherit*/ false); return (attrs != null && attrs.Length > 0); } static private PermissionSet s_fullTrustPermissionSet; // Check if the caller is fully trusted static internal bool IsCallerFullTrust { get { bool isFullTrust = false; try { if (s_fullTrustPermissionSet == null) { s_fullTrustPermissionSet = new PermissionSet(PermissionState.Unrestricted); } s_fullTrustPermissionSet.Demand(); isFullTrust = true; } catch { } return isFullTrust; } } // Check if the type is allowed to be used in config by checking the APTCA bit internal static bool IsTypeAllowedInConfig(Type t) { // Note: // This code is copied from HttpRuntime.IsTypeAllowedInConfig, but modified in // how it checks for fulltrust this can be called from non-ASP.NET apps. // Allow everything in full trust if (IsCallerFullTrust) { return true; } // The APTCA bit is only relevant for assemblies living in the GAC, since the rest runs // under partial trust (VSWhidbey 422183) Assembly assembly = t.Assembly; if (!assembly.GlobalAssemblyCache) return true; // If it has the APTCA bit, allow it if (HasAptcaBit(assembly)) return true; // It's a GAC type without APTCA in partial trust scenario: block it return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Configuration.Internal; using System.Reflection; using System.Security; using System.Security.Permissions; namespace System.Configuration { internal static class TypeUtil { // // Since the config APIs were originally implemented in System.dll, // references to types without assembly names could be resolved to // System.dll in Everett. Emulate that behavior by trying to get the // type from System.dll // static private Type GetLegacyType(string typeString) { Type type = null; // // Ignore all exceptions, otherwise callers will get unexpected // exceptions not related to the original failure to load the // desired type. // try { Assembly systemAssembly = typeof(ConfigurationException).Assembly; type = systemAssembly.GetType(typeString, false); } catch { } return type; } // // Get the type specified by typeString. If it fails, try to retrieve it // as a type from System.dll. If that fails, return null or throw the original // exception as indicated by throwOnError. // static private Type GetTypeImpl(string typeString, bool throwOnError) { Type type = null; Exception originalException = null; try { type = Type.GetType(typeString, throwOnError); } catch (Exception e) { originalException = e; } if (type == null) { type = GetLegacyType(typeString); if (type == null && originalException != null) { throw originalException; } } return type; } // // Ask the host to get the type specified by typeString. If it fails, try to retrieve it // as a type from System.dll. If that fails, return null or throw the original // exception as indicated by throwOnError. // [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags)] static internal Type GetTypeWithReflectionPermission(IInternalConfigHost host, string typeString, bool throwOnError) { Type type = null; Exception originalException = null; try { type = host.GetConfigType(typeString, throwOnError); } catch (Exception e) { originalException = e; } if (type == null) { type = GetLegacyType(typeString); if (type == null && originalException != null) { throw originalException; } } return type; } [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags)] static internal Type GetTypeWithReflectionPermission(string typeString, bool throwOnError) { return GetTypeImpl(typeString, throwOnError); } [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags | ReflectionPermissionFlag.MemberAccess)] static internal object CreateInstanceWithReflectionPermission(string typeString) { Type type = GetTypeImpl(typeString, true); // catch the errors and report them object result = Activator.CreateInstance(type, true); // create non-public types return result; } [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags | ReflectionPermissionFlag.MemberAccess)] static internal object CreateInstanceWithReflectionPermission(Type type) { object result = Activator.CreateInstance(type, true); // create non-public types return result; } [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags)] static internal ConstructorInfo GetConstructorWithReflectionPermission(Type type, Type baseType, bool throwOnError) { type = VerifyAssignableType(baseType, type, throwOnError); if (type == null) { return null; } BindingFlags bindingFlags = BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic; ConstructorInfo ctor = type.GetConstructor(bindingFlags, null, CallingConventions.HasThis, Type.EmptyTypes, null); if (ctor == null && throwOnError) { throw new TypeLoadException(SR.GetString(SR.TypeNotPublic, type.AssemblyQualifiedName)); } return ctor; } [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags | ReflectionPermissionFlag.MemberAccess)] static internal object InvokeCtorWithReflectionPermission(ConstructorInfo ctor) { return ctor.Invoke(null); } static internal bool IsTypeFromTrustedAssemblyWithoutAptca(Type type) { Assembly assembly = type.Assembly; return assembly.GlobalAssemblyCache && !HasAptcaBit(assembly); } static internal Type VerifyAssignableType(Type baseType, Type type, bool throwOnError) { if (baseType.IsAssignableFrom(type)) { return type; } if (throwOnError) { throw new TypeLoadException( SR.GetString(SR.Config_type_doesnt_inherit_from_type, type.FullName, baseType.FullName)); } return null; } [ReflectionPermission(SecurityAction.Assert, Flags=ReflectionPermissionFlag.NoFlags | ReflectionPermissionFlag.MemberAccess)] private static bool HasAptcaBit(Assembly assembly) { Object[] attrs = assembly.GetCustomAttributes( typeof(System.Security.AllowPartiallyTrustedCallersAttribute), /*inherit*/ false); return (attrs != null && attrs.Length > 0); } static private PermissionSet s_fullTrustPermissionSet; // Check if the caller is fully trusted static internal bool IsCallerFullTrust { get { bool isFullTrust = false; try { if (s_fullTrustPermissionSet == null) { s_fullTrustPermissionSet = new PermissionSet(PermissionState.Unrestricted); } s_fullTrustPermissionSet.Demand(); isFullTrust = true; } catch { } return isFullTrust; } } // Check if the type is allowed to be used in config by checking the APTCA bit internal static bool IsTypeAllowedInConfig(Type t) { // Note: // This code is copied from HttpRuntime.IsTypeAllowedInConfig, but modified in // how it checks for fulltrust this can be called from non-ASP.NET apps. // Allow everything in full trust if (IsCallerFullTrust) { return true; } // The APTCA bit is only relevant for assemblies living in the GAC, since the rest runs // under partial trust (VSWhidbey 422183) Assembly assembly = t.Assembly; if (!assembly.GlobalAssemblyCache) return true; // If it has the APTCA bit, allow it if (HasAptcaBit(assembly)) return true; // It's a GAC type without APTCA in partial trust scenario: block it return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
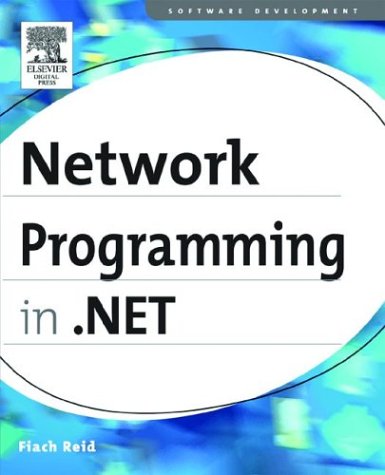
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NamespaceEmitter.cs
- WinEventWrap.cs
- ConfigUtil.cs
- MediaContextNotificationWindow.cs
- LocatorBase.cs
- HtmlContainerControl.cs
- ParentQuery.cs
- ConfigurationValidatorBase.cs
- DBCommandBuilder.cs
- BufferedGraphicsContext.cs
- AppDomainCompilerProxy.cs
- DbConnectionStringBuilder.cs
- CatalogZone.cs
- GenericUI.cs
- DynamicActivityXamlReader.cs
- ContainsRowNumberChecker.cs
- CodeActivityContext.cs
- HandleValueEditor.cs
- MouseEventArgs.cs
- SourceFileBuildProvider.cs
- SafeSystemMetrics.cs
- PanningMessageFilter.cs
- MediaElement.cs
- ImportedNamespaceContextItem.cs
- XmlDictionaryReaderQuotasElement.cs
- InfoCardCryptoHelper.cs
- MissingMethodException.cs
- HtmlHistory.cs
- GeometryDrawing.cs
- DataKeyPropertyAttribute.cs
- MetadataException.cs
- MethodMessage.cs
- DocumentOrderQuery.cs
- TileBrush.cs
- EntityRecordInfo.cs
- ExecutionContext.cs
- ProxyWebPart.cs
- EnvelopedPkcs7.cs
- PathNode.cs
- ButtonChrome.cs
- SpecialFolderEnumConverter.cs
- BypassElement.cs
- TextTreeObjectNode.cs
- RelationHandler.cs
- PreparingEnlistment.cs
- BufferModesCollection.cs
- SubclassTypeValidatorAttribute.cs
- XslUrlEditor.cs
- DiagnosticsConfiguration.cs
- NonParentingControl.cs
- SecurityException.cs
- MetaModel.cs
- EventLogger.cs
- ColumnReorderedEventArgs.cs
- SiteMapNodeItemEventArgs.cs
- CredentialCache.cs
- SpecularMaterial.cs
- AuthenticationService.cs
- SqlDataSource.cs
- ProfileInfo.cs
- Compilation.cs
- ListCollectionView.cs
- SamlAuthenticationStatement.cs
- ChildChangedEventArgs.cs
- MailMessage.cs
- EntitySqlQueryCacheEntry.cs
- Parsers.cs
- PointValueSerializer.cs
- Transform3DCollection.cs
- OdbcInfoMessageEvent.cs
- MSG.cs
- Range.cs
- ViewPort3D.cs
- listviewsubitemcollectioneditor.cs
- SafeLibraryHandle.cs
- DataViewSettingCollection.cs
- DependencyPropertyHelper.cs
- BoolExpression.cs
- ObjectStateManagerMetadata.cs
- SelectionHighlightInfo.cs
- _NativeSSPI.cs
- ProfileInfo.cs
- GridViewRowCollection.cs
- TextHidden.cs
- SQLDoubleStorage.cs
- MissingFieldException.cs
- EncryptedPackageFilter.cs
- XmlSchemas.cs
- PnrpPeerResolverElement.cs
- RsaSecurityKey.cs
- DelimitedListTraceListener.cs
- RemoteWebConfigurationHost.cs
- EntityContainerAssociationSetEnd.cs
- ElementsClipboardData.cs
- SystemColors.cs
- LocalBuilder.cs
- StrongNameMembershipCondition.cs
- UserControlCodeDomTreeGenerator.cs
- MenuItemCollection.cs
- AsymmetricSignatureDeformatter.cs