Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / System / Windows / Interop / MSG.cs / 1 / MSG.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Diagnostics.CodeAnalysis; using System.Security; using MS.Internal.WindowsBase; namespace System.Windows.Interop { ////// This class is the managed version of the Win32 MSG datatype. /// ////// For Avalon/WinForms interop to work, WinForms needs to be able to modify MSG structs as they are /// processed, so they are passed by ref (it's also a perf gain) /// /// - but in the Partial Trust scenario, this would be a security vulnerability; allowing partially trusted code /// to intercept and arbitrarily change MSG contents could potentially create a spoofing opportunity. /// /// - so rather than try to secure all posible current and future extensibility points against untrusted code /// getting write access to a MSG struct during message processing, we decided the simpler, more performant, and /// more secure, both now and going forward, solution was to secure write access to the MSG struct directly /// at the source. /// /// - get access is unrestricted and should in-line nicely for zero perf cost /// /// - set access is restricted via a call to SecurityHelper.DemandUnrestrictedUIPermission, which is optimized /// to a no-op in the Full Trust scenario, and will throw a security exception in the Partial Trust scenario /// /// - NOTE: This breaks Avalon/WinForms interop in the Partial Trust scenario, but that's not a supported /// scenario anyway. /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] [SuppressMessage("Microsoft.Naming", "CA1705:LongAcronymsShouldBePascalCased")] [SuppressMessage("Microsoft.Performance", "CA1815:OverrideEqualsAndOperatorEqualsOnValueTypes")] [StructLayout(LayoutKind.Sequential)] [Serializable] public struct MSG { ////// Critical: Setting critical data /// [SecurityCritical] [FriendAccessAllowed] // Built into Base, used by Core or Framework. internal MSG(IntPtr hwnd, int message, IntPtr wParam, IntPtr lParam, int time, int pt_x, int pt_y) { _hwnd = hwnd; _message = message; _wParam = wParam; _lParam = lParam; _time = time; _pt_x = pt_x; _pt_y = pt_y; } // // Public Properties: // ////// The handle of the window to which the message was sent. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Design", "CA1051:DoNotDeclareVisibleInstanceFields")] [SuppressMessage("Microsoft.Security", "CA2111:PointersShouldNotBeVisible")] [SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] public IntPtr hwnd { [SecurityCritical] get { return _hwnd; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _hwnd = value; } } ////// The Value of the window message. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// public int message { [SecurityCritical] get { return _message; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _message = value; } } ////// The wParam of the window message. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] [SuppressMessage("Microsoft.Security", "CA2111:PointersShouldNotBeVisible")] [SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] public IntPtr wParam { [SecurityCritical] get { return _wParam; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _wParam = value; } } ////// The lParam of the window message. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] [SuppressMessage("Microsoft.Security", "CA2111:PointersShouldNotBeVisible")] [SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] public IntPtr lParam { [SecurityCritical] get { return _lParam; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _lParam = value; } } ////// The time the window message was sent. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// public int time { [SecurityCritical] get { return _time; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _time = value; } } // In the original Win32, pt was a by-Value POINT structure ////// The X coordinate of the message POINT struct. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Naming", "CA1706:ShortAcronymsShouldBeUpperCased")] [SuppressMessage("Microsoft.Naming", "CA1707:IdentifiersShouldNotContainUnderscores")] public int pt_x { [SecurityCritical] get { return _pt_x; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _pt_x = value; } } ////// The Y coordinate of the message POINT struct. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Naming", "CA1706:ShortAcronymsShouldBeUpperCased")] [SuppressMessage("Microsoft.Naming", "CA1707:IdentifiersShouldNotContainUnderscores")] public int pt_y { [SecurityCritical] get { return _pt_y; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _pt_y = value; } } // // Internal data: // - do not alter the number, order or size of ANY of this members! // - they must agree EXACTLY with the native Win32 MSG structure ////// The handle of the window to which the message was sent. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private IntPtr _hwnd; ////// The Value of the window message. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private int _message; ////// The wParam of the window message. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private IntPtr _wParam; ////// The lParam of the window message. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private IntPtr _lParam; ////// The time the window message was sent. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private int _time; ////// The X coordinate of the message POINT struct. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private int _pt_x; ////// The Y coordinate of the message POINT struct. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private int _pt_y; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Diagnostics.CodeAnalysis; using System.Security; using MS.Internal.WindowsBase; namespace System.Windows.Interop { ////// This class is the managed version of the Win32 MSG datatype. /// ////// For Avalon/WinForms interop to work, WinForms needs to be able to modify MSG structs as they are /// processed, so they are passed by ref (it's also a perf gain) /// /// - but in the Partial Trust scenario, this would be a security vulnerability; allowing partially trusted code /// to intercept and arbitrarily change MSG contents could potentially create a spoofing opportunity. /// /// - so rather than try to secure all posible current and future extensibility points against untrusted code /// getting write access to a MSG struct during message processing, we decided the simpler, more performant, and /// more secure, both now and going forward, solution was to secure write access to the MSG struct directly /// at the source. /// /// - get access is unrestricted and should in-line nicely for zero perf cost /// /// - set access is restricted via a call to SecurityHelper.DemandUnrestrictedUIPermission, which is optimized /// to a no-op in the Full Trust scenario, and will throw a security exception in the Partial Trust scenario /// /// - NOTE: This breaks Avalon/WinForms interop in the Partial Trust scenario, but that's not a supported /// scenario anyway. /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] [SuppressMessage("Microsoft.Naming", "CA1705:LongAcronymsShouldBePascalCased")] [SuppressMessage("Microsoft.Performance", "CA1815:OverrideEqualsAndOperatorEqualsOnValueTypes")] [StructLayout(LayoutKind.Sequential)] [Serializable] public struct MSG { ////// Critical: Setting critical data /// [SecurityCritical] [FriendAccessAllowed] // Built into Base, used by Core or Framework. internal MSG(IntPtr hwnd, int message, IntPtr wParam, IntPtr lParam, int time, int pt_x, int pt_y) { _hwnd = hwnd; _message = message; _wParam = wParam; _lParam = lParam; _time = time; _pt_x = pt_x; _pt_y = pt_y; } // // Public Properties: // ////// The handle of the window to which the message was sent. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Design", "CA1051:DoNotDeclareVisibleInstanceFields")] [SuppressMessage("Microsoft.Security", "CA2111:PointersShouldNotBeVisible")] [SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] public IntPtr hwnd { [SecurityCritical] get { return _hwnd; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _hwnd = value; } } ////// The Value of the window message. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// public int message { [SecurityCritical] get { return _message; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _message = value; } } ////// The wParam of the window message. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] [SuppressMessage("Microsoft.Security", "CA2111:PointersShouldNotBeVisible")] [SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] public IntPtr wParam { [SecurityCritical] get { return _wParam; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _wParam = value; } } ////// The lParam of the window message. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] [SuppressMessage("Microsoft.Security", "CA2111:PointersShouldNotBeVisible")] [SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] public IntPtr lParam { [SecurityCritical] get { return _lParam; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _lParam = value; } } ////// The time the window message was sent. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// public int time { [SecurityCritical] get { return _time; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _time = value; } } // In the original Win32, pt was a by-Value POINT structure ////// The X coordinate of the message POINT struct. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Naming", "CA1706:ShortAcronymsShouldBeUpperCased")] [SuppressMessage("Microsoft.Naming", "CA1707:IdentifiersShouldNotContainUnderscores")] public int pt_x { [SecurityCritical] get { return _pt_x; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _pt_x = value; } } ////// The Y coordinate of the message POINT struct. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Naming", "CA1706:ShortAcronymsShouldBeUpperCased")] [SuppressMessage("Microsoft.Naming", "CA1707:IdentifiersShouldNotContainUnderscores")] public int pt_y { [SecurityCritical] get { return _pt_y; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _pt_y = value; } } // // Internal data: // - do not alter the number, order or size of ANY of this members! // - they must agree EXACTLY with the native Win32 MSG structure ////// The handle of the window to which the message was sent. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private IntPtr _hwnd; ////// The Value of the window message. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private int _message; ////// The wParam of the window message. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private IntPtr _wParam; ////// The lParam of the window message. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private IntPtr _lParam; ////// The time the window message was sent. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private int _time; ////// The X coordinate of the message POINT struct. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private int _pt_x; ////// The Y coordinate of the message POINT struct. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private int _pt_y; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
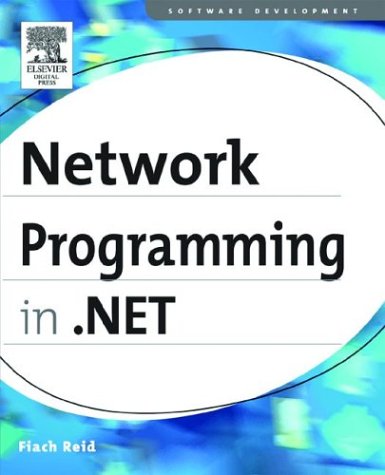
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StreamGeometry.cs
- SoapEnumAttribute.cs
- XomlCompilerResults.cs
- JavaScriptObjectDeserializer.cs
- DPAPIProtectedConfigurationProvider.cs
- AdRotator.cs
- SqlVersion.cs
- ValidationErrorEventArgs.cs
- PathGradientBrush.cs
- PrintDialogException.cs
- RefreshEventArgs.cs
- SynchronizationValidator.cs
- ApplicationContext.cs
- OrderByBuilder.cs
- DataGridViewCellParsingEventArgs.cs
- LoadItemsEventArgs.cs
- ResourceBinder.cs
- EventDescriptor.cs
- PresentationAppDomainManager.cs
- AnimationClock.cs
- XmlSchemaInclude.cs
- hresults.cs
- UniformGrid.cs
- SqlEnums.cs
- NetNamedPipeBindingElement.cs
- IdentityHolder.cs
- PrintDialog.cs
- WebPartTransformer.cs
- BaseValidator.cs
- LocalServiceSecuritySettingsElement.cs
- LogSwitch.cs
- MDIControlStrip.cs
- PtsHost.cs
- OpenTypeCommon.cs
- DocumentPageView.cs
- ListControlConvertEventArgs.cs
- SiteMapNodeItemEventArgs.cs
- DataGridViewElement.cs
- ItemCollection.cs
- SystemSounds.cs
- SafeNativeMethodsOther.cs
- RemotingConfigParser.cs
- SchemaNames.cs
- PocoPropertyAccessorStrategy.cs
- FixedSOMPageConstructor.cs
- Util.cs
- PowerStatus.cs
- LambdaCompiler.Generated.cs
- XmlSerializableWriter.cs
- QueryOperationResponseOfT.cs
- MsmqIntegrationAppDomainProtocolHandler.cs
- Stylesheet.cs
- DbConnectionPool.cs
- SrgsDocument.cs
- BaseDataListPage.cs
- ImageSourceValueSerializer.cs
- SqlResolver.cs
- KeyToListMap.cs
- WebPartEditorCancelVerb.cs
- SqlClientWrapperSmiStream.cs
- httpstaticobjectscollection.cs
- ConsoleKeyInfo.cs
- DataPagerCommandEventArgs.cs
- MessageBox.cs
- TraceSection.cs
- ImportContext.cs
- CookieProtection.cs
- XMLUtil.cs
- InnerItemCollectionView.cs
- ConnectionInterfaceCollection.cs
- AliasedExpr.cs
- CoTaskMemHandle.cs
- WebServiceParameterData.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- TextSpanModifier.cs
- DetailsViewDeleteEventArgs.cs
- ServiceDescription.cs
- ResolveNameEventArgs.cs
- SqlBulkCopyColumnMapping.cs
- BooleanProjectedSlot.cs
- RegexGroupCollection.cs
- TableAdapterManagerHelper.cs
- UITypeEditors.cs
- InvalidStoreProtectionKeyException.cs
- MdiWindowListItemConverter.cs
- LinqDataSourceSelectEventArgs.cs
- Selector.cs
- ControlCachePolicy.cs
- Currency.cs
- XamlToRtfWriter.cs
- ModulesEntry.cs
- Image.cs
- ItemChangedEventArgs.cs
- PixelShader.cs
- MeasurementDCInfo.cs
- SrgsItemList.cs
- TypeExtensionConverter.cs
- PhysicalAddress.cs
- InheritedPropertyChangedEventArgs.cs
- RSAOAEPKeyExchangeFormatter.cs