Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / Configuration / TraceSection.cs / 1 / TraceSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TraceSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propEnabled = new ConfigurationProperty("enabled", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propLocalOnly = new ConfigurationProperty("localOnly", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMostRecent = new ConfigurationProperty("mostRecent", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propPageOutput = new ConfigurationProperty("pageOutput", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequestLimit = new ConfigurationProperty("requestLimit", typeof(int), 10, null, StdValidatorsAndConverters.PositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMode = new ConfigurationProperty("traceMode", typeof(TraceDisplayMode), TraceDisplayMode.SortByTime, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _writeToDiagnosticTrace = new ConfigurationProperty("writeToDiagnosticsTrace", typeof(bool), false, ConfigurationPropertyOptions.None); static TraceSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propEnabled); _properties.Add(_propLocalOnly); _properties.Add(_propMostRecent); _properties.Add(_propPageOutput); _properties.Add(_propRequestLimit); _properties.Add(_propMode); _properties.Add(_writeToDiagnosticTrace); } public TraceSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("enabled", DefaultValue = false)] public bool Enabled { get { return (bool)base[_propEnabled]; } set { base[_propEnabled] = value; } } [ConfigurationProperty("mostRecent", DefaultValue = false)] public bool MostRecent { get { return (bool)base[_propMostRecent]; } set { base[_propMostRecent] = value; } } [ConfigurationProperty("localOnly", DefaultValue = true)] public bool LocalOnly { get { return (bool)base[_propLocalOnly]; } set { base[_propLocalOnly] = value; } } [ConfigurationProperty("pageOutput", DefaultValue = false)] public bool PageOutput { get { return (bool)base[_propPageOutput]; } set { base[_propPageOutput] = value; } } [ConfigurationProperty("requestLimit", DefaultValue = 10)] [IntegerValidator(MinValue = 0)] public int RequestLimit { get { return (int)base[_propRequestLimit]; } set { base[_propRequestLimit] = value; } } [ConfigurationProperty("traceMode", DefaultValue = TraceDisplayMode.SortByTime)] public TraceDisplayMode TraceMode { get { return (TraceDisplayMode)base[_propMode]; } set { base[_propMode] = value; } } [ConfigurationProperty("writeToDiagnosticsTrace", DefaultValue = false)] public bool WriteToDiagnosticsTrace { get { return (bool)base[_writeToDiagnosticTrace]; } set { base[_writeToDiagnosticTrace] = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TraceSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propEnabled = new ConfigurationProperty("enabled", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propLocalOnly = new ConfigurationProperty("localOnly", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMostRecent = new ConfigurationProperty("mostRecent", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propPageOutput = new ConfigurationProperty("pageOutput", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequestLimit = new ConfigurationProperty("requestLimit", typeof(int), 10, null, StdValidatorsAndConverters.PositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMode = new ConfigurationProperty("traceMode", typeof(TraceDisplayMode), TraceDisplayMode.SortByTime, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _writeToDiagnosticTrace = new ConfigurationProperty("writeToDiagnosticsTrace", typeof(bool), false, ConfigurationPropertyOptions.None); static TraceSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propEnabled); _properties.Add(_propLocalOnly); _properties.Add(_propMostRecent); _properties.Add(_propPageOutput); _properties.Add(_propRequestLimit); _properties.Add(_propMode); _properties.Add(_writeToDiagnosticTrace); } public TraceSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("enabled", DefaultValue = false)] public bool Enabled { get { return (bool)base[_propEnabled]; } set { base[_propEnabled] = value; } } [ConfigurationProperty("mostRecent", DefaultValue = false)] public bool MostRecent { get { return (bool)base[_propMostRecent]; } set { base[_propMostRecent] = value; } } [ConfigurationProperty("localOnly", DefaultValue = true)] public bool LocalOnly { get { return (bool)base[_propLocalOnly]; } set { base[_propLocalOnly] = value; } } [ConfigurationProperty("pageOutput", DefaultValue = false)] public bool PageOutput { get { return (bool)base[_propPageOutput]; } set { base[_propPageOutput] = value; } } [ConfigurationProperty("requestLimit", DefaultValue = 10)] [IntegerValidator(MinValue = 0)] public int RequestLimit { get { return (int)base[_propRequestLimit]; } set { base[_propRequestLimit] = value; } } [ConfigurationProperty("traceMode", DefaultValue = TraceDisplayMode.SortByTime)] public TraceDisplayMode TraceMode { get { return (TraceDisplayMode)base[_propMode]; } set { base[_propMode] = value; } } [ConfigurationProperty("writeToDiagnosticsTrace", DefaultValue = false)] public bool WriteToDiagnosticsTrace { get { return (bool)base[_writeToDiagnosticTrace]; } set { base[_writeToDiagnosticTrace] = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
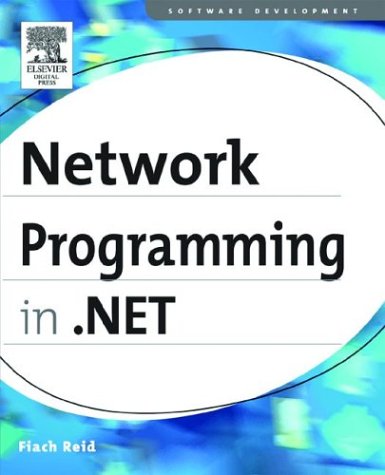
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntitySetDataBindingList.cs
- Simplifier.cs
- SQLResource.cs
- RemoteWebConfigurationHostStream.cs
- DataSourceCacheDurationConverter.cs
- Win32MouseDevice.cs
- HttpStreamMessageEncoderFactory.cs
- StrongTypingException.cs
- SessionEndingCancelEventArgs.cs
- HttpListenerTimeoutManager.cs
- DeviceSpecificDialogCachedState.cs
- WmfPlaceableFileHeader.cs
- VirtualDirectoryMapping.cs
- ColorContext.cs
- PeerNameRecordCollection.cs
- ScriptBehaviorDescriptor.cs
- SiteMapSection.cs
- WebPartConnectionsEventArgs.cs
- WebRequestModuleElement.cs
- DelegatedStream.cs
- Type.cs
- PathSegmentCollection.cs
- AxHostDesigner.cs
- CqlParser.cs
- BitStack.cs
- TextCompositionManager.cs
- XPathException.cs
- WorkingDirectoryEditor.cs
- PrivilegeNotHeldException.cs
- DataListItemEventArgs.cs
- XmlSchemaInfo.cs
- BamlRecords.cs
- MethodImplAttribute.cs
- ToolStripMenuItem.cs
- ObjectParameter.cs
- GrammarBuilderDictation.cs
- SqlNodeAnnotations.cs
- infer.cs
- JsonClassDataContract.cs
- HttpCapabilitiesSectionHandler.cs
- OdbcException.cs
- StaticTextPointer.cs
- COM2IProvidePropertyBuilderHandler.cs
- VarRemapper.cs
- ExpressionBindings.cs
- QuaternionIndependentAnimationStorage.cs
- ContextMenuStrip.cs
- DoubleConverter.cs
- WarningException.cs
- CompositeDuplexElement.cs
- StateManagedCollection.cs
- EnlistmentState.cs
- DBDataPermission.cs
- SafeLocalMemHandle.cs
- wgx_sdk_version.cs
- Variable.cs
- DuplicateWaitObjectException.cs
- WindowClosedEventArgs.cs
- PermissionSetTriple.cs
- WorkflowApplicationTerminatedException.cs
- XmlAutoDetectWriter.cs
- NativeMethods.cs
- IntSecurity.cs
- KnownTypesHelper.cs
- XmlSchemaAttributeGroup.cs
- HttpCacheParams.cs
- MetabaseServerConfig.cs
- MetaModel.cs
- EmissiveMaterial.cs
- Figure.cs
- QueryOptionExpression.cs
- SingletonConnectionReader.cs
- DBSchemaRow.cs
- Compilation.cs
- ZeroOpNode.cs
- TransactedBatchingElement.cs
- MatrixStack.cs
- FileInfo.cs
- MediaContextNotificationWindow.cs
- ToolStripHighContrastRenderer.cs
- isolationinterop.cs
- ISAPIApplicationHost.cs
- DataRowChangeEvent.cs
- StoryFragments.cs
- ColumnResult.cs
- IisTraceListener.cs
- SqlEnums.cs
- HTTPNotFoundHandler.cs
- MenuBase.cs
- ColorMatrix.cs
- mediaeventshelper.cs
- LinqDataSourceView.cs
- Floater.cs
- PeerUnsafeNativeMethods.cs
- ToolStripItem.cs
- ListBoxItem.cs
- WizardPanel.cs
- MultiTrigger.cs
- Semaphore.cs
- XmlLanguageConverter.cs