Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Documents / DocumentPage.cs / 1305600 / DocumentPage.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DocumentPage.cs // // Description: Base for pages returned from the Paginator.GetPage. // // History: // 05/02/2003 : [....] - created. // 09/28/2004 : [....] - updated pagination model. // 08/29/2005 : [....] - updated pagination model. // //--------------------------------------------------------------------------- using System.Windows.Media; // Visual namespace System.Windows.Documents { ////// Base for pages returned from the Paginator.GetPage. /// public class DocumentPage : IDisposable { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Public constructor. /// /// The visual representation of this page. public DocumentPage(Visual visual) { _visual = visual; _pageSize = Size.Empty; _bleedBox = Rect.Empty; _contentBox = Rect.Empty; } ////// Public constructor. /// /// The visual representation of this page. /// The size of the page, including margins. /// The bounds the ink drawn by the page. /// The bounds of the content within the page. public DocumentPage(Visual visual, Size pageSize, Rect bleedBox, Rect contentBox) { _visual = visual; _pageSize = pageSize; _bleedBox = bleedBox; _contentBox = contentBox; } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Dispose the page. /// public virtual void Dispose() { _visual = null; _pageSize = Size.Empty; _bleedBox = Rect.Empty; _contentBox = Rect.Empty; OnPageDestroyed(EventArgs.Empty); } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ////// The visual representation of this page. /// public virtual Visual Visual { get { return _visual; } } ////// The size of the page, including margins. /// public virtual Size Size { get { if (_pageSize == Size.Empty && _visual != null) { return VisualTreeHelper.GetContentBounds(_visual).Size; } return _pageSize; } } ////// The bounds the ink drawn by the page, relative to the page size. /// public virtual Rect BleedBox { get { if (_bleedBox == Rect.Empty) { return new Rect(this.Size); } return _bleedBox; } } ////// The bounds of the content within the page, relative to the page size. /// public virtual Rect ContentBox { get { if (_contentBox == Rect.Empty) { return new Rect(this.Size); } return _contentBox; } } ////// Static representation of a non-existent page. /// public static readonly DocumentPage Missing = new MissingDocumentPage(); #endregion Public Properties //------------------------------------------------------------------- // // Public Events // //-------------------------------------------------------------------- #region Public Events ////// Called when the Visual for this page has been destroyed and /// can no longer be used for display. /// public event EventHandler PageDestroyed; #endregion Public Events //------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------- #region Protected Methods ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the PageDestroyed event. protected void OnPageDestroyed(EventArgs e) { if (this.PageDestroyed != null) { this.PageDestroyed(this, e); } } ////// Sets the visual representation of this page. /// /// The visual representation of this page. protected void SetVisual(Visual visual) { _visual = visual; } ////// Sets the size of the page. /// /// The size of the page, including margins. protected void SetSize(Size size) { _pageSize = size; } ////// Sets the bounds the ink drawn by the page. /// /// The bounds the ink drawn by the page, relative to the page size. protected void SetBleedBox(Rect bleedBox) { _bleedBox = bleedBox; } ////// Sets the bounds of the content within the page. /// /// The bounds of the content within the page, relative to the page size. protected void SetContentBox(Rect contentBox) { _contentBox = contentBox; } #endregion Protected Methods //------------------------------------------------------------------- // // Protected Fields // //-------------------------------------------------------------------- #region Protected Fields ////// The visual representation of this page. /// private Visual _visual; ////// The size of the page, including margins. /// private Size _pageSize; ////// The bounds the ink drawn by the page, relative to the page size. /// private Rect _bleedBox; ////// The bounds of the content within the page, relative to the page size. /// private Rect _contentBox; #endregion Protected Fields //------------------------------------------------------------------- // // Missing // //-------------------------------------------------------------------- #region Missing private sealed class MissingDocumentPage : DocumentPage { public MissingDocumentPage() : base(null, Size.Empty, Rect.Empty, Rect.Empty) { } public override void Dispose() {} } #endregion Missing } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
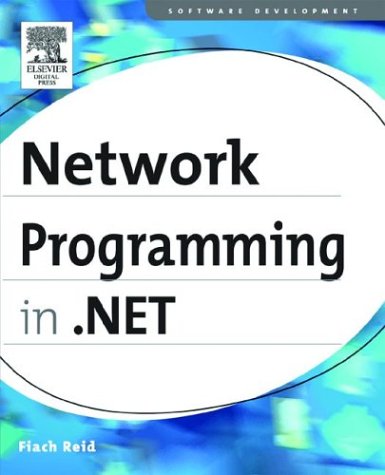
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlWriter.cs
- _ContextAwareResult.cs
- BulletedListEventArgs.cs
- ReadOnlyNameValueCollection.cs
- DoubleConverter.cs
- WindowProviderWrapper.cs
- TextBoxAutoCompleteSourceConverter.cs
- SoapCodeExporter.cs
- DesignerActionList.cs
- PropertyEmitterBase.cs
- DataSet.cs
- BamlStream.cs
- InternalBufferManager.cs
- XamlParser.cs
- CodeSubDirectoriesCollection.cs
- MarginsConverter.cs
- RegexTree.cs
- SecurityProtocolFactory.cs
- AmbientEnvironment.cs
- PermissionListSet.cs
- TypeUtil.cs
- SelectionListComponentEditor.cs
- ButtonBase.cs
- UserControlCodeDomTreeGenerator.cs
- WindowVisualStateTracker.cs
- TextBox.cs
- FusionWrap.cs
- ThrowHelper.cs
- Sql8ConformanceChecker.cs
- NamedElement.cs
- MenuItemCollection.cs
- XmlMembersMapping.cs
- RectIndependentAnimationStorage.cs
- SqlComparer.cs
- ToolBarOverflowPanel.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- ReliabilityContractAttribute.cs
- AccessControlList.cs
- RoutingBehavior.cs
- DataBindEngine.cs
- TextViewDesigner.cs
- ObjectViewQueryResultData.cs
- ZeroOpNode.cs
- StorageMappingItemLoader.cs
- KeyboardNavigation.cs
- XmlSchemaGroupRef.cs
- Comparer.cs
- cookiecollection.cs
- CodeFieldReferenceExpression.cs
- TypeSystemHelpers.cs
- DataContractJsonSerializerOperationFormatter.cs
- DocumentSchemaValidator.cs
- CatalogZoneBase.cs
- ContextMenuStripActionList.cs
- DnsEndPoint.cs
- InternalControlCollection.cs
- ServerValidateEventArgs.cs
- _ProxyChain.cs
- SqlBulkCopyColumnMapping.cs
- PermissionSetEnumerator.cs
- LoadItemsEventArgs.cs
- AutomationEvent.cs
- oledbmetadatacolumnnames.cs
- MobileControlPersister.cs
- XPathItem.cs
- CollectionBuilder.cs
- UIElementCollection.cs
- XmlWriterTraceListener.cs
- ValidationError.cs
- InvalidComObjectException.cs
- SEHException.cs
- ObjectPersistData.cs
- OutputCacheProfileCollection.cs
- FormViewCommandEventArgs.cs
- SqlRecordBuffer.cs
- ReadOnlyHierarchicalDataSourceView.cs
- HtmlTableRow.cs
- SelectionListComponentEditor.cs
- TextLineResult.cs
- MetricEntry.cs
- OuterGlowBitmapEffect.cs
- SharedStatics.cs
- PhonemeConverter.cs
- WebServiceResponseDesigner.cs
- VirtualizingPanel.cs
- TreeNodeMouseHoverEvent.cs
- NullableFloatSumAggregationOperator.cs
- AudioDeviceOut.cs
- BitFlagsGenerator.cs
- LocatorGroup.cs
- XmlSchemaValidator.cs
- InputScopeNameConverter.cs
- SqlErrorCollection.cs
- DisplayMemberTemplateSelector.cs
- PropertyChangeTracker.cs
- XmlMemberMapping.cs
- ObjectAnimationBase.cs
- AsyncDataRequest.cs
- TreeView.cs
- TraceXPathNavigator.cs