Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Utility / SponsorHelper.cs / 1305600 / SponsorHelper.cs
using System; using System.Diagnostics; using System.Runtime.Remoting; using System.Runtime.Remoting.Lifetime; using System.Security; using System.Security.Permissions; namespace MS.Internal.Utility { #region SponsorHelper Class ////// We either mark the Sponsor as MarshalByRef or make it serializable. /// If we make it MarshalByRef, then this sponsor which is used to control /// the lifetime of a MBR object in turn needs to have another sponsor OR /// the sponsor can mark itself to remain alive for the life of the AppDomain /// by overriding InitializeLifetimeService and returning null OR the object /// can be marked as Serializeable instead of MBR in which case it is marshaled /// by value to the client appdomain and will not have the state of the host /// appdomain to make renewal decisions. In our case we don't have any state so /// its easier and better perf-wise to mark it Serializable. /// [Serializable] internal class SponsorHelper : ISponsor { #region Private Data private ILease _lease; private TimeSpan _timespan; #endregion Private Data #region Constructor internal SponsorHelper(ILease lease, TimeSpan timespan) { Debug.Assert(lease != null && timespan != null, "Lease and TimeSpan arguments cannot be null"); _lease = lease; _timespan = timespan; } #endregion Constructor #region ISponsor Interface TimeSpan ISponsor.Renewal(ILease lease) { if (lease == null) { throw new ArgumentNullException("lease"); } return _timespan; } #endregion ISponsor Interface #region Internal Methods ////// Critical - asserts permission for RemotingConfiguration /// TreatAsSafe - The constructor for this object is internal and this function does not take /// random parameters and hence can’t be used to keep random objects alive or access any other object /// in the application. /// [SecurityCritical, SecurityTreatAsSafe] [SecurityPermissionAttribute(SecurityAction.Assert, RemotingConfiguration = true)] internal void Register() { _lease.Register((ISponsor)this); } ////// Critical - asserts permission for RemotingConfiguration /// TreatAsSafe - The constructor for this object is internal and this function does not take /// random parameters and hence can’t be used to keep random objects alive or access any other object /// in the application. /// [SecurityCritical, SecurityTreatAsSafe] [SecurityPermissionAttribute(SecurityAction.Assert, RemotingConfiguration = true)] internal void Unregister() { _lease.Unregister((ISponsor)this); } #endregion Internal Methods } #endregion SponsorHelper Class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Diagnostics; using System.Runtime.Remoting; using System.Runtime.Remoting.Lifetime; using System.Security; using System.Security.Permissions; namespace MS.Internal.Utility { #region SponsorHelper Class ////// We either mark the Sponsor as MarshalByRef or make it serializable. /// If we make it MarshalByRef, then this sponsor which is used to control /// the lifetime of a MBR object in turn needs to have another sponsor OR /// the sponsor can mark itself to remain alive for the life of the AppDomain /// by overriding InitializeLifetimeService and returning null OR the object /// can be marked as Serializeable instead of MBR in which case it is marshaled /// by value to the client appdomain and will not have the state of the host /// appdomain to make renewal decisions. In our case we don't have any state so /// its easier and better perf-wise to mark it Serializable. /// [Serializable] internal class SponsorHelper : ISponsor { #region Private Data private ILease _lease; private TimeSpan _timespan; #endregion Private Data #region Constructor internal SponsorHelper(ILease lease, TimeSpan timespan) { Debug.Assert(lease != null && timespan != null, "Lease and TimeSpan arguments cannot be null"); _lease = lease; _timespan = timespan; } #endregion Constructor #region ISponsor Interface TimeSpan ISponsor.Renewal(ILease lease) { if (lease == null) { throw new ArgumentNullException("lease"); } return _timespan; } #endregion ISponsor Interface #region Internal Methods ////// Critical - asserts permission for RemotingConfiguration /// TreatAsSafe - The constructor for this object is internal and this function does not take /// random parameters and hence can’t be used to keep random objects alive or access any other object /// in the application. /// [SecurityCritical, SecurityTreatAsSafe] [SecurityPermissionAttribute(SecurityAction.Assert, RemotingConfiguration = true)] internal void Register() { _lease.Register((ISponsor)this); } ////// Critical - asserts permission for RemotingConfiguration /// TreatAsSafe - The constructor for this object is internal and this function does not take /// random parameters and hence can’t be used to keep random objects alive or access any other object /// in the application. /// [SecurityCritical, SecurityTreatAsSafe] [SecurityPermissionAttribute(SecurityAction.Assert, RemotingConfiguration = true)] internal void Unregister() { _lease.Unregister((ISponsor)this); } #endregion Internal Methods } #endregion SponsorHelper Class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
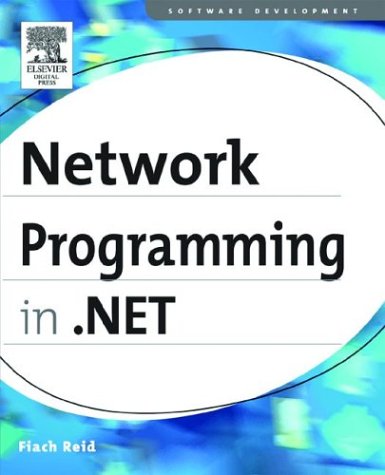
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RijndaelManagedTransform.cs
- DbConnectionStringBuilder.cs
- Underline.cs
- ZipArchive.cs
- XsdBuildProvider.cs
- MonikerSyntaxException.cs
- XamlReaderHelper.cs
- AsymmetricSignatureFormatter.cs
- BufferBuilder.cs
- assemblycache.cs
- CacheMemory.cs
- DataTemplate.cs
- ParseNumbers.cs
- iisPickupDirectory.cs
- XmlDocumentType.cs
- ComponentRenameEvent.cs
- IndicShape.cs
- ObjectQuery.cs
- XmlNode.cs
- OdbcCommandBuilder.cs
- EndpointAddress.cs
- HtmlToClrEventProxy.cs
- documentation.cs
- WebUtil.cs
- AppSettingsExpressionBuilder.cs
- initElementDictionary.cs
- PeekCompletedEventArgs.cs
- ToolStripProgressBar.cs
- DbSetClause.cs
- XamlLoadErrorInfo.cs
- AnnotationMap.cs
- Tokenizer.cs
- PassportAuthentication.cs
- SelectionEditor.cs
- FrameSecurityDescriptor.cs
- XamlVector3DCollectionSerializer.cs
- PageBuildProvider.cs
- ConstructorBuilder.cs
- DrawingBrush.cs
- Dynamic.cs
- ImageAttributes.cs
- InspectionWorker.cs
- NameSpaceEvent.cs
- WindowsEditBoxRange.cs
- PrivateFontCollection.cs
- CharacterMetrics.cs
- DelegateTypeInfo.cs
- sqlser.cs
- ObjectManager.cs
- OptimalBreakSession.cs
- Attributes.cs
- SafeNativeMethods.cs
- Converter.cs
- DataGridViewMethods.cs
- GridViewColumnCollectionChangedEventArgs.cs
- SynchronizedInputAdaptor.cs
- DataError.cs
- CodeObject.cs
- RetriableClipboard.cs
- ControllableStoryboardAction.cs
- ClientSettingsSection.cs
- DesignerVerbCollection.cs
- ListViewDataItem.cs
- FileLevelControlBuilderAttribute.cs
- TextFormatterImp.cs
- ConfigurationSectionGroup.cs
- DataPagerFieldItem.cs
- HttpModuleAction.cs
- AutoResizedEvent.cs
- PictureBox.cs
- XMLSchema.cs
- ColorAnimation.cs
- TextBox.cs
- Int16Animation.cs
- DataSpaceManager.cs
- OledbConnectionStringbuilder.cs
- SnapshotChangeTrackingStrategy.cs
- StateMachineHelpers.cs
- UnitySerializationHolder.cs
- CharEntityEncoderFallback.cs
- CopyOfAction.cs
- WorkflowApplicationUnloadedException.cs
- DataServiceHost.cs
- SqlCachedBuffer.cs
- BitmapCodecInfo.cs
- UncommonField.cs
- SystemIPInterfaceStatistics.cs
- MethodBuilderInstantiation.cs
- DeclarativeCatalogPart.cs
- TypefaceCollection.cs
- TableMethodGenerator.cs
- DateTimeParse.cs
- StrokeCollection2.cs
- DesignSurfaceServiceContainer.cs
- XmlSchemaAnnotation.cs
- BamlTreeUpdater.cs
- ValidationErrorEventArgs.cs
- DataObjectFieldAttribute.cs
- MailHeaderInfo.cs
- HideDisabledControlAdapter.cs