Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / MS / Internal / Shaping / ShapeTypeface.cs / 1 / ShapeTypeface.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2002 // // File: ShapeTypeface.cs // // Contents: GlyphTypeface with shaping capability // // Created: 10-13-2003 [....] ([....]) // //----------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Collections; using System.Collections.Generic; using System.Globalization; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Utility; using MS.Internal; using MS.Internal.FontCache; using MS.Internal.FontFace; using MS.Internal.TextFormatting; using FontFace = MS.Internal.FontFace; namespace MS.Internal.Shaping { ////// Typeface that is capable of shaping character string. Shaping is done /// thru shaping engines. /// internal class ShapeTypeface { private GlyphTypeface _glyphTypeface; private IDeviceFont _deviceFont; private ShapeState[] _shapeStates; private ShapingEngineManager _shapeManager; internal ShapeTypeface( ShapingEngineManager shapeManager, GlyphTypeface glyphTypeface, IDeviceFont deviceFont ) { Invariant.Assert(shapeManager != null && glyphTypeface != null); _shapeManager = shapeManager; _glyphTypeface = glyphTypeface; _deviceFont = deviceFont; } public override int GetHashCode() { return HashFn.HashMultiply(_glyphTypeface.GetHashCode()) + (_deviceFont == null ? 0 : _deviceFont.Name.GetHashCode()); } public override bool Equals(object o) { ShapeTypeface t = o as ShapeTypeface; if(t == null) return false; if (_deviceFont == null) { if (t._deviceFont != null) return false; } else { if (t._deviceFont == null || t._deviceFont.Name != _deviceFont.Name) return false; } return _glyphTypeface.Equals(t._glyphTypeface); } internal IDeviceFont DeviceFont { get { return _deviceFont; } } ////// Shape specified character string /// internal ushort[] GetGlyphs( CheckedCharPointer unicodeString, int stringLength, CultureInfo culture, Item item, ShapingOptions flags, FeatureSet featureSet, CheckedCharacterShapingPropertiesPointer characterProperties, CheckedUShortPointer clusterMap, out GlyphShapingProperties[] glyphProperties, out int glyphCount ) { ShapeState shapeState = GetShapeState(item.Script); return shapeState.GetGlyphs( unicodeString, stringLength, culture, item, _glyphTypeface, flags, featureSet, characterProperties, clusterMap, out glyphProperties, out glyphCount ); } ////// Get placement of the specified glyphs /// internal void GetGlyphPlacements( CharacterBufferRange characterBufferRange, CheckedUShortPointer glyphIndices, CheckedGlyphShapingPropertiesPointer glyphProperties, int glyphCount, Item item, CultureInfo culture, CheckedUShortPointer clusterMap, CheckedCharacterShapingPropertiesPointer characterProperties, int charLength, ShapingOptions flags, FeatureSet featureSet, double emSize, CheckedIntPointer glyphAdvances, CheckedGlyphOffsetPointer glyphOffsets ) { ShapeState shapeState = GetShapeState(item.Script); shapeState.GetGlyphPlacements( glyphIndices, glyphProperties, glyphCount, item, culture, clusterMap, characterProperties, charLength, flags, featureSet, _glyphTypeface, emSize / _glyphTypeface.FontFaceLayoutInfo.DesignEmHeight, glyphAdvances, glyphOffsets ); } ////// Get physical font face /// internal GlyphTypeface GlyphTypeface { get { return _glyphTypeface; } } ////// Get shaping state of the specified script /// private ShapeState GetShapeState(ScriptID script) { if( _shapeStates != null && _shapeStates[(int)script] != null) { return _shapeStates[(int)script]; } return CacheShapeState(script); } ////// Cache the new shaping state of the font face /// private ShapeState CacheShapeState(ScriptID script) { Debug.Assert(_shapeStates == null || _shapeStates[(int)script] == null); ShapeState shapeState; if(_shapeStates == null) { // first shaping state to create for this font face _shapeStates = new ShapeState[(int)ScriptID.Max]; } object engineState; shapeState = new ShapeState( _shapeManager.OnLoadFont( script, _glyphTypeface, out engineState ), engineState ); _shapeStates[(int)script] = shapeState; return shapeState; } ////// State of shaping engine in a font /// private class ShapeState { private IShapingEngine _shape; private object _engineState; private const int MaxStackGlyphs = 192; internal ShapeState( IShapingEngine shape, object engineState ) { _shape = shape; _engineState = engineState; } ////// Shape specified character string thru correspondent shaping engine /// ////// Critical - this method calls into shaping engine. /// Safe - this method returns glyph indices and shaping properties which are safe. /// [SecurityCritical, SecurityTreatAsSafe] internal ushort[] GetGlyphs( CheckedCharPointer unicodeString, int stringLength, CultureInfo culture, Item item, GlyphTypeface glyphTypeface, ShapingOptions flags, FeatureSet featureSet, CheckedCharacterShapingPropertiesPointer characterProperties, CheckedUShortPointer clusterMap, out GlyphShapingProperties[] glyphProperties, out int glyphCount ) { ushort[] glyphs; _shape[item.Flags].GetGlyphs( item, culture, glyphTypeface, featureSet, flags, _engineState, unicodeString, stringLength, characterProperties, clusterMap, out glyphs, out glyphProperties, out glyphCount ); return glyphs; } ////// Get glyph placement of the specified glyphs thru correspondent shaping engine /// ////// Critical - this method calls into shaping engine /// Safe - this method returns glyph placements which are safe. /// [SecurityCritical, SecurityTreatAsSafe] internal void GetGlyphPlacements( CheckedUShortPointer glyphIndices, CheckedGlyphShapingPropertiesPointer glyphProperties, int glyphCount, Item item, CultureInfo culture, CheckedUShortPointer clusterMap, CheckedCharacterShapingPropertiesPointer characterProperties, int charLength, ShapingOptions flags, FeatureSet featureSet, GlyphTypeface glyphTypeface, double designToReal, CheckedIntPointer glyphAdvances, CheckedGlyphOffsetPointer glyphOffsets ) { _shape[item.Flags].GetGlyphPlacements( item, culture, glyphTypeface, featureSet, flags, _engineState, characterProperties, clusterMap, charLength, glyphIndices, glyphProperties, glyphCount, designToReal, glyphAdvances, glyphOffsets ); } } } ////// Scaled shape typeface /// internal class ScaledShapeTypeface { private ShapeTypeface _shapeTypeface; private double _scaleInEm; private bool _nullShape; internal ScaledShapeTypeface( ShapingEngineManager shapeManager, GlyphTypeface glyphTypeface, IDeviceFont deviceFont, double scaleInEm, bool nullShape ) { _shapeTypeface = new ShapeTypeface(shapeManager, glyphTypeface, deviceFont); _scaleInEm = scaleInEm; _nullShape = nullShape; } internal ShapeTypeface ShapeTypeface { get { return _shapeTypeface; } } internal double ScaleInEm { get { return _scaleInEm; } } internal bool NullShape { get { return _nullShape; } } public override int GetHashCode() { int hash = _shapeTypeface.GetHashCode(); unsafe { hash = HashFn.HashMultiply(hash) + (int)(_nullShape ? 1 : 0); hash = HashFn.HashMultiply(hash) + _scaleInEm.GetHashCode(); return HashFn.HashScramble(hash); } } public override bool Equals(object o) { ScaledShapeTypeface t = o as ScaledShapeTypeface; if (t == null) return false; return _shapeTypeface.Equals(t._shapeTypeface) && _scaleInEm == t._scaleInEm && _nullShape == t._nullShape; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
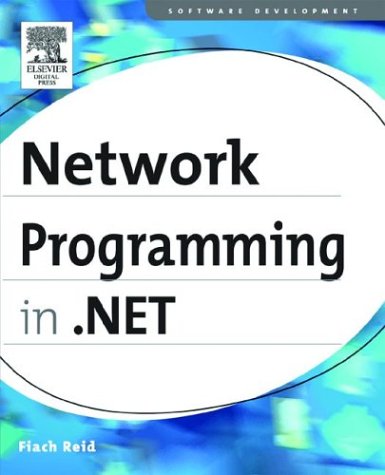
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _FtpDataStream.cs
- SafeNativeMethods.cs
- Message.cs
- SignatureResourcePool.cs
- SqlSelectStatement.cs
- FeatureSupport.cs
- HelpInfo.cs
- SmtpDigestAuthenticationModule.cs
- ListViewCommandEventArgs.cs
- XmlIgnoreAttribute.cs
- InternalTypeHelper.cs
- Avt.cs
- OdbcFactory.cs
- RecognizedPhrase.cs
- GorillaCodec.cs
- PropertyChangedEventManager.cs
- AutomationPropertyInfo.cs
- ColumnHeaderConverter.cs
- StringInfo.cs
- XmlEventCache.cs
- SecurityHelper.cs
- PropertySegmentSerializer.cs
- EventBuilder.cs
- ParameterToken.cs
- GraphicsContainer.cs
- SyndicationElementExtension.cs
- ExpressionBinding.cs
- JsonStringDataContract.cs
- TrustLevelCollection.cs
- HashAlgorithm.cs
- Subtree.cs
- PreservationFileReader.cs
- DataObjectAttribute.cs
- FormViewRow.cs
- CompilerWrapper.cs
- ConnectionManagementElementCollection.cs
- TabRenderer.cs
- PlanCompilerUtil.cs
- XhtmlTextWriter.cs
- ExpressionTextBox.xaml.cs
- PropertyGridCommands.cs
- PersonalizableTypeEntry.cs
- CodeExpressionStatement.cs
- MatrixAnimationUsingPath.cs
- WindowsTreeView.cs
- MainMenu.cs
- GlyphInfoList.cs
- HwndKeyboardInputProvider.cs
- ConversionContext.cs
- MatrixUtil.cs
- Operators.cs
- DbConnectionClosed.cs
- ArraySortHelper.cs
- FileLevelControlBuilderAttribute.cs
- XhtmlTextWriter.cs
- UIElement3D.cs
- DerivedKeyCachingSecurityTokenSerializer.cs
- SqlTypesSchemaImporter.cs
- ValidatedControlConverter.cs
- TypeSystem.cs
- sqlcontext.cs
- RoutedPropertyChangedEventArgs.cs
- ObservableDictionary.cs
- QueryExecutionOption.cs
- BCLDebug.cs
- SplitterEvent.cs
- ObjectDataProvider.cs
- DnsPermission.cs
- EffectiveValueEntry.cs
- RichListBox.cs
- MultiSelector.cs
- SignedPkcs7.cs
- MdImport.cs
- CompositeTypefaceMetrics.cs
- X509SecurityTokenAuthenticator.cs
- StreamReader.cs
- DataGridViewImageColumn.cs
- _LocalDataStoreMgr.cs
- NumberFormatter.cs
- AutomationElementIdentifiers.cs
- ComponentCommands.cs
- TextSegment.cs
- EventDescriptor.cs
- ThreadPool.cs
- SafeNativeMethods.cs
- MethodCallConverter.cs
- WorkflowMarkupSerializerMapping.cs
- ProtectedConfiguration.cs
- ResXDataNode.cs
- WebPartManagerInternals.cs
- NumberFormatInfo.cs
- BamlRecords.cs
- SvcMapFileSerializer.cs
- MenuItemCollection.cs
- RecommendedAsConfigurableAttribute.cs
- EdmType.cs
- LocalsItemDescription.cs
- ProcessModelInfo.cs
- EntityContainerAssociationSet.cs
- GeometryHitTestResult.cs