Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Configuration / OutputCacheSection.cs / 3 / OutputCacheSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class OutputCacheSection : ConfigurationSection { internal const bool DefaultOmitVaryStar = false; private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propEnableOutputCache = new ConfigurationProperty("enableOutputCache", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableFragmentCache = new ConfigurationProperty("enableFragmentCache", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propSendCacheControlHeader = new ConfigurationProperty("sendCacheControlHeader", typeof(bool), HttpRuntimeSection.DefaultSendCacheControlHeader, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propOmitVaryStar = new ConfigurationProperty("omitVaryStar", typeof(bool), DefaultOmitVaryStar, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableKernelCacheForVaryByStar = new ConfigurationProperty("enableKernelCacheForVaryByStar", typeof(bool), false, ConfigurationPropertyOptions.None); private bool sendCacheControlHeaderCached = false; private bool sendCacheControlHeaderCache; private bool omitVaryStarCached = false; private bool omitVaryStar; private bool enableKernelCacheForVaryByStarCached = false; private bool enableKernelCacheForVaryByStar; private bool enableOutputCacheCached = false; private bool enableOutputCache; static OutputCacheSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propEnableOutputCache); _properties.Add(_propEnableFragmentCache); _properties.Add(_propSendCacheControlHeader); _properties.Add(_propOmitVaryStar); _properties.Add(_propEnableKernelCacheForVaryByStar); } public OutputCacheSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("enableOutputCache", DefaultValue = true)] public bool EnableOutputCache { get { if (enableOutputCacheCached == false) { enableOutputCache = (bool)base[_propEnableOutputCache]; enableOutputCacheCached = true; } return enableOutputCache; } set { base[_propEnableOutputCache] = value; enableOutputCache = value; } } [ConfigurationProperty("enableFragmentCache", DefaultValue = true)] public bool EnableFragmentCache { get { return (bool)base[_propEnableFragmentCache]; } set { base[_propEnableFragmentCache] = value; } } [ConfigurationProperty("sendCacheControlHeader", DefaultValue = HttpRuntimeSection.DefaultSendCacheControlHeader)] public bool SendCacheControlHeader { get { if (sendCacheControlHeaderCached == false) { sendCacheControlHeaderCache = (bool)base[_propSendCacheControlHeader]; sendCacheControlHeaderCached = true; } return sendCacheControlHeaderCache; } set { base[_propSendCacheControlHeader] = value; sendCacheControlHeaderCache = value; } } [ConfigurationProperty("omitVaryStar", DefaultValue = DefaultOmitVaryStar)] public bool OmitVaryStar { get { if (omitVaryStarCached == false) { omitVaryStar = (bool)base[_propOmitVaryStar]; omitVaryStarCached = true; } return omitVaryStar; } set { base[_propOmitVaryStar] = value; omitVaryStar = value; } } [ConfigurationProperty("enableKernelCacheForVaryByStar", DefaultValue = false)] public bool EnableKernelCacheForVaryByStar { get { if (enableKernelCacheForVaryByStarCached == false) { enableKernelCacheForVaryByStar = (bool)base[_propEnableKernelCacheForVaryByStar]; enableKernelCacheForVaryByStarCached = true; } return enableKernelCacheForVaryByStar; } set { base[_propEnableKernelCacheForVaryByStar] = value; enableKernelCacheForVaryByStar = value; } } } }
Link Menu
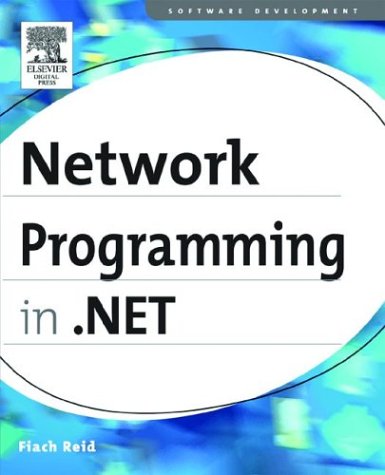
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LinearGradientBrush.cs
- ArgumentNullException.cs
- TextBox.cs
- DelegatingTypeDescriptionProvider.cs
- TextAnchor.cs
- precedingsibling.cs
- ValueType.cs
- EmptyReadOnlyDictionaryInternal.cs
- HttpProcessUtility.cs
- ValueChangedEventManager.cs
- StatusBar.cs
- TempFiles.cs
- SortFieldComparer.cs
- PeerToPeerException.cs
- WebPartVerbsEventArgs.cs
- PersonalizationProviderHelper.cs
- COAUTHINFO.cs
- IndexedGlyphRun.cs
- StorageAssociationSetMapping.cs
- ConfigXmlElement.cs
- EventRoute.cs
- ClonableStack.cs
- XmlSchemaGroupRef.cs
- ObjectSecurity.cs
- DecimalConstantAttribute.cs
- SubMenuStyle.cs
- OracleBinary.cs
- StrongName.cs
- DocumentDesigner.cs
- PropertyTab.cs
- HtmlInputFile.cs
- MessageContractExporter.cs
- ResourceCategoryAttribute.cs
- Stackframe.cs
- xml.cs
- BitVec.cs
- QueryPrefixOp.cs
- Region.cs
- PriorityBindingExpression.cs
- DataGridLengthConverter.cs
- tooltip.cs
- VectorKeyFrameCollection.cs
- SafeProcessHandle.cs
- ButtonChrome.cs
- ContextMenuAutomationPeer.cs
- Message.cs
- DataSourceCollectionBase.cs
- ConfigurationSectionGroupCollection.cs
- WebPartConnectionsDisconnectVerb.cs
- Literal.cs
- UTF7Encoding.cs
- FieldDescriptor.cs
- PrincipalPermission.cs
- CompositeScriptReference.cs
- XmlUtil.cs
- SignedPkcs7.cs
- PrimaryKeyTypeConverter.cs
- initElementDictionary.cs
- Emitter.cs
- TablePattern.cs
- ContractDescription.cs
- RelationshipType.cs
- UnaryNode.cs
- UIElementParagraph.cs
- PropertyGroupDescription.cs
- InertiaTranslationBehavior.cs
- ContextMenu.cs
- latinshape.cs
- PrivacyNoticeElement.cs
- DragCompletedEventArgs.cs
- XmlCharType.cs
- MulticastDelegate.cs
- ISAPIWorkerRequest.cs
- SequenceQuery.cs
- FormViewPageEventArgs.cs
- MenuItemStyleCollection.cs
- NamespaceCollection.cs
- Helper.cs
- DispatcherExceptionFilterEventArgs.cs
- DataShape.cs
- StyleSelector.cs
- LicenseContext.cs
- DrawItemEvent.cs
- HttpContext.cs
- ComponentCommands.cs
- Drawing.cs
- configsystem.cs
- BamlLocalizableResourceKey.cs
- AlgoModule.cs
- SharedConnectionWorkflowTransactionService.cs
- QilList.cs
- FileDialog.cs
- DataGridViewRowEventArgs.cs
- DynamicArgumentDialog.cs
- DataFormats.cs
- SamlAuthorityBinding.cs
- ManifestResourceInfo.cs
- unsafeIndexingFilterStream.cs
- FocusTracker.cs
- KeyedHashAlgorithm.cs