Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Data / PropertyGroupDescription.cs / 1305600 / PropertyGroupDescription.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Description of grouping based on a property value. // // See spec at http://avalon/connecteddata/Specs/Grouping.mht // //--------------------------------------------------------------------------- using System; // StringComparison using System.ComponentModel; // [DefaultValue] using System.Globalization; // CultureInfo using System.Reflection; // PropertyInfo using System.Windows; // SR using System.Xml; // XmlNode using MS.Internal; // XmlHelper namespace System.Windows.Data { ////// Description of grouping based on a property value. /// public class PropertyGroupDescription : GroupDescription { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Initializes a new instance of PropertyGroupDescription. /// public PropertyGroupDescription() { } ////// Initializes a new instance of PropertyGroupDescription. /// /// /// The name of the property whose value is used to determine which group(s) /// an item belongs to. /// If PropertyName is null, the item itself is used. /// public PropertyGroupDescription(string propertyName) { UpdatePropertyName(propertyName); } ////// Initializes a new instance of PropertyGroupDescription. /// /// /// The name of the property whose value is used to determine which group(s) /// an item belongs to. /// If PropertyName is null, the item itself is used. /// /// /// This converter is applied to the property value (or the item) to /// produce the final value used to determine which group(s) an item /// belongs to. /// If the delegate returns an ICollection, the item is added to /// multiple groups - one for each member of the collection. /// public PropertyGroupDescription(string propertyName, IValueConverter converter) { UpdatePropertyName(propertyName); _converter = converter; } ////// Initializes a new instance of PropertyGroupDescription. /// /// /// The name of the property whose value is used to determine which group(s) /// an item belongs to. /// If PropertyName is null, the item itself is used. /// /// /// This converter is applied to the property value (or the item) to /// produce the final value used to determine which group(s) an item /// belongs to. /// If the delegate returns an ICollection, the item is added to /// multiple groups - one for each member of the collection. /// /// /// This governs the comparison between an item's value (as determined /// by PropertyName and Converter) and a group's name. /// It is ignored unless both comparands are strings. /// The default value is StringComparison.Ordinal. /// public PropertyGroupDescription(string propertyName, IValueConverter converter, StringComparison stringComparison) { UpdatePropertyName(propertyName); _converter = converter; _stringComparison = stringComparison; } #endregion Constructors #region Public Properties //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// The name of the property whose value is used to determine which group(s) /// an item belongs to. /// If PropertyName is null, the item itself is used. /// [DefaultValue(null)] public string PropertyName { get { return _propertyName; } set { UpdatePropertyName(value); OnPropertyChanged("PropertyName"); } } ////// This converter is applied to the property value (or the item) to /// produce the final value used to determine which group(s) an item /// belongs to. /// If the delegate returns an ICollection, the item is added to /// multiple groups - one for each member of the collection. /// [DefaultValue(null)] public IValueConverter Converter { get { return _converter; } set { _converter = value; OnPropertyChanged("Converter"); } } ////// This governs the comparison between an item's value (as determined /// by PropertyName and Converter) and a group's name. /// It is ignored unless both comparands are strings. /// The default value is StringComparison.Ordinal. /// [DefaultValue(StringComparison.Ordinal)] public StringComparison StringComparison { get { return _stringComparison; } set { _stringComparison = value; OnPropertyChanged("StringComparison"); } } #endregion Public Properties #region Public Methods //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ ////// Return the group name(s) for the given item /// public override object GroupNameFromItem(object item, int level, CultureInfo culture) { object value; object xmlValue; // get the property value if (String.IsNullOrEmpty(PropertyName)) { value = item; } else if (AssemblyHelper.TryGetValueFromXmlNode(item, PropertyName, out xmlValue)) { value = xmlValue; } else if (item != null) { using (_propertyPath.SetContext(item)) { value = _propertyPath.GetValue(); } } else { value = null; } // apply the converter to the value if (Converter != null) { value = Converter.Convert(value, typeof(object), level, culture); } return value; } ////// Return true if the names match (i.e the item should belong to the group). /// public override bool NamesMatch(object groupName, object itemName) { string s1 = groupName as string; string s2 = itemName as string; if (s1 != null && s2 != null) { return String.Equals(s1, s2, StringComparison); } else { return Object.Equals(groupName, itemName); } } #endregion Public Methods #region Private Methods private void UpdatePropertyName(string propertyName) { _propertyName = propertyName; _propertyPath = !String.IsNullOrEmpty(propertyName) ? new PropertyPath(propertyName) : null; } private void OnPropertyChanged(string propertyName) { OnPropertyChanged(new PropertyChangedEventArgs(propertyName)); } #endregion Private Methods #region Private Fields //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ string _propertyName; PropertyPath _propertyPath; IValueConverter _converter; StringComparison _stringComparison = StringComparison.Ordinal; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
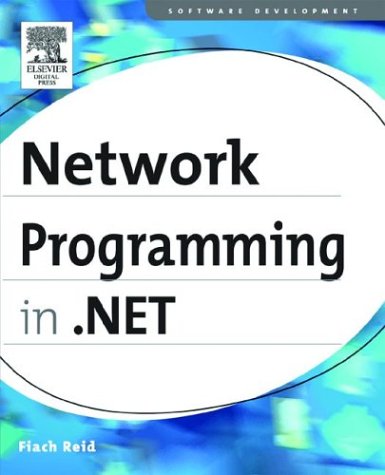
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyBuilder.cs
- NoneExcludedImageIndexConverter.cs
- ListViewItem.cs
- BoundConstants.cs
- ObjectNotFoundException.cs
- SchemaType.cs
- ToolStripGrip.cs
- XmlConvert.cs
- Bitmap.cs
- TrackingProfile.cs
- SqlCacheDependencySection.cs
- DummyDataSource.cs
- DecoderBestFitFallback.cs
- SoapFormatExtensions.cs
- RelationshipManager.cs
- TypeLibConverter.cs
- DateTimeConstantAttribute.cs
- DesignerVerbToolStripMenuItem.cs
- CalendarDataBindingHandler.cs
- FontUnit.cs
- DefaultValueConverter.cs
- AnchoredBlock.cs
- ModelService.cs
- RefreshResponseInfo.cs
- SystemException.cs
- LinqExpressionNormalizer.cs
- BackEase.cs
- TextChange.cs
- MethodRental.cs
- SoapFormatterSinks.cs
- TableChangeProcessor.cs
- DataGridViewColumnDividerDoubleClickEventArgs.cs
- HtmlElementCollection.cs
- SystemInformation.cs
- ContractTypeNameCollection.cs
- TemplateField.cs
- MemberDescriptor.cs
- Effect.cs
- IQueryable.cs
- Aggregates.cs
- SponsorHelper.cs
- NumericExpr.cs
- NativeMethods.cs
- ForwardPositionQuery.cs
- EntityException.cs
- RepeatInfo.cs
- CodeNamespaceCollection.cs
- HtmlEmptyTagControlBuilder.cs
- COM2TypeInfoProcessor.cs
- EntityDataSourceValidationException.cs
- ShapeTypeface.cs
- PermissionListSet.cs
- PasswordRecoveryAutoFormat.cs
- CreateUserWizardAutoFormat.cs
- ListViewContainer.cs
- ModelPerspective.cs
- DbReferenceCollection.cs
- FilterException.cs
- KnownTypes.cs
- ObjectSet.cs
- ProviderIncompatibleException.cs
- ScalarOps.cs
- PropertyMapper.cs
- CodeSnippetTypeMember.cs
- SiteMapProvider.cs
- QueryPrefixOp.cs
- RegistrySecurity.cs
- DataGridViewCellLinkedList.cs
- OdbcReferenceCollection.cs
- FlowDocumentReaderAutomationPeer.cs
- CrossSiteScriptingValidation.cs
- DrawingImage.cs
- tibetanshape.cs
- DataServiceExpressionVisitor.cs
- OdbcEnvironment.cs
- DiagnosticTrace.cs
- EpmCustomContentSerializer.cs
- StreamAsIStream.cs
- PipelineModuleStepContainer.cs
- GeometryGroup.cs
- BooleanFunctions.cs
- TabItemWrapperAutomationPeer.cs
- ExpressionQuoter.cs
- NumericUpDownAccelerationCollection.cs
- ContentPosition.cs
- CompiledRegexRunner.cs
- BaseCollection.cs
- SecurityHelper.cs
- DataException.cs
- FilterElement.cs
- ComponentChangedEvent.cs
- NativeMethods.cs
- SafeHandles.cs
- CountAggregationOperator.cs
- HybridObjectCache.cs
- XmlSchemaObjectTable.cs
- ValidatorCompatibilityHelper.cs
- DetailsViewUpdatedEventArgs.cs
- GlyphInfoList.cs
- WaitHandle.cs