Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / PropertyMapper.cs / 2 / PropertyMapper.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * PropertyMapper.cs * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.UI { using System; using System.Collections; using System.Reflection; using System.Web.Util; using System.ComponentModel; internal sealed class PropertyMapper { private const char PERSIST_CHAR = '-'; private const char OM_CHAR = '.'; private const string STR_OM_CHAR = "."; /* * Maps persisted attribute names to the object model equivalents. * This class should not be instantiated by itself. */ private PropertyMapper() { } /* * Returns the PropertyInfo or FieldInfo corresponding to the * specified property name. */ internal static MemberInfo GetMemberInfo(Type ctrlType, string name, out string nameForCodeGen) { Type currentType = ctrlType; PropertyInfo propInfo = null; FieldInfo fieldInfo = null; string mappedName = MapNameToPropertyName(name); nameForCodeGen = null; int startIndex = 0; while (startIndex < mappedName.Length) { // parse thru dots of object model to locate PropertyInfo string propName; int index = mappedName.IndexOf(OM_CHAR, startIndex); if (index < 0) { propName = mappedName.Substring(startIndex); startIndex = mappedName.Length; } else { propName = mappedName.Substring(startIndex, index - startIndex); startIndex = index + 1; } BindingFlags flags = BindingFlags.Public | BindingFlags.Instance | BindingFlags.Static | BindingFlags.IgnoreCase; // try { propInfo = currentType.GetProperty(propName, flags); } catch (AmbiguousMatchException) { flags |= BindingFlags.DeclaredOnly; propInfo = currentType.GetProperty(propName, flags); } if (propInfo == null) { // could not find a public property, look for a public field fieldInfo = currentType.GetField(propName, flags); if (fieldInfo == null) { nameForCodeGen = null; break; } } propName = null; if (propInfo != null) { // found a public property currentType = propInfo.PropertyType; propName = propInfo.Name; } else { // found a public field currentType = fieldInfo.FieldType; propName = fieldInfo.Name; } // Throw if the type of not CLS-compliant (ASURT 83438) if (!IsTypeCLSCompliant(currentType)) { throw new HttpException(SR.GetString( SR.Property_Not_ClsCompliant, name, ctrlType.FullName, currentType.FullName)); } if (propName != null) { if (nameForCodeGen == null) nameForCodeGen = propName; else nameForCodeGen += STR_OM_CHAR + propName; } } if (propInfo != null) return propInfo; else return fieldInfo; } private static bool IsTypeCLSCompliant(Type type) { // We used to check this by looking up the CLSCompliantAttribute, but that was // way too inefficent. So instead, we just juck for a few specific types if ((type == typeof(SByte)) || (type == typeof(TypedReference)) || (type == typeof(UInt16)) || (type == typeof(UInt32)) || (type == typeof(UInt64)) || (type == typeof(UIntPtr))) { return false; } return true; } /* * Maps the specified persisted name to its object model equivalent. * The convention is to map all dashes to dots. * For example : Font-Size maps to Font.Size * HeaderStyle-Font-Name maps to HeaderStyle.Font.Name */ internal static string MapNameToPropertyName(string attrName) { return attrName.Replace(PERSIST_CHAR,OM_CHAR); } internal static object LocatePropertyObject(object obj, string mappedName, out string propertyName, bool inDesigner) { object currentObject = obj; Type currentType = obj.GetType(); propertyName = null; int index; int startIndex = 0; // step through the dots of the object model to extract the PropertyInfo // and object on which the property will be set while (startIndex < mappedName.Length) { index = mappedName.IndexOf(OM_CHAR, startIndex); // If we didn't find a separator, we're on the last piece if (index < 0) break; // There is a sub property, so get its info and iterate propertyName = mappedName.Substring(startIndex, index - startIndex); startIndex = index + 1; currentObject = FastPropertyAccessor.GetProperty(currentObject, propertyName, inDesigner); if (currentObject == null) return null; } // Avoid a useless call to Substring if possible if (startIndex > 0) propertyName = mappedName.Substring(startIndex); else propertyName = mappedName; return currentObject; } /* * Walks the object model using the mapped property name to get the * value of an instance's property. */ internal static PropertyDescriptor GetMappedPropertyDescriptor(object obj, string mappedName, out object childObject, out string propertyName, bool inDesigner) { childObject = LocatePropertyObject(obj, mappedName, out propertyName, inDesigner); if (childObject == null) return null; PropertyDescriptorCollection properties = TypeDescriptor.GetProperties(childObject, inDesigner); return properties[propertyName]; } /* * Walks the object model using the mapped property name to set the * value of an instance's property. */ internal static void SetMappedPropertyValue(object obj, string mappedName, object value, bool inDesigner) { string propertyName; object childObj = LocatePropertyObject(obj, mappedName, out propertyName, inDesigner); if (childObj == null) return; FastPropertyAccessor.SetProperty(childObj, propertyName, value, inDesigner); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * PropertyMapper.cs * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.UI { using System; using System.Collections; using System.Reflection; using System.Web.Util; using System.ComponentModel; internal sealed class PropertyMapper { private const char PERSIST_CHAR = '-'; private const char OM_CHAR = '.'; private const string STR_OM_CHAR = "."; /* * Maps persisted attribute names to the object model equivalents. * This class should not be instantiated by itself. */ private PropertyMapper() { } /* * Returns the PropertyInfo or FieldInfo corresponding to the * specified property name. */ internal static MemberInfo GetMemberInfo(Type ctrlType, string name, out string nameForCodeGen) { Type currentType = ctrlType; PropertyInfo propInfo = null; FieldInfo fieldInfo = null; string mappedName = MapNameToPropertyName(name); nameForCodeGen = null; int startIndex = 0; while (startIndex < mappedName.Length) { // parse thru dots of object model to locate PropertyInfo string propName; int index = mappedName.IndexOf(OM_CHAR, startIndex); if (index < 0) { propName = mappedName.Substring(startIndex); startIndex = mappedName.Length; } else { propName = mappedName.Substring(startIndex, index - startIndex); startIndex = index + 1; } BindingFlags flags = BindingFlags.Public | BindingFlags.Instance | BindingFlags.Static | BindingFlags.IgnoreCase; // try { propInfo = currentType.GetProperty(propName, flags); } catch (AmbiguousMatchException) { flags |= BindingFlags.DeclaredOnly; propInfo = currentType.GetProperty(propName, flags); } if (propInfo == null) { // could not find a public property, look for a public field fieldInfo = currentType.GetField(propName, flags); if (fieldInfo == null) { nameForCodeGen = null; break; } } propName = null; if (propInfo != null) { // found a public property currentType = propInfo.PropertyType; propName = propInfo.Name; } else { // found a public field currentType = fieldInfo.FieldType; propName = fieldInfo.Name; } // Throw if the type of not CLS-compliant (ASURT 83438) if (!IsTypeCLSCompliant(currentType)) { throw new HttpException(SR.GetString( SR.Property_Not_ClsCompliant, name, ctrlType.FullName, currentType.FullName)); } if (propName != null) { if (nameForCodeGen == null) nameForCodeGen = propName; else nameForCodeGen += STR_OM_CHAR + propName; } } if (propInfo != null) return propInfo; else return fieldInfo; } private static bool IsTypeCLSCompliant(Type type) { // We used to check this by looking up the CLSCompliantAttribute, but that was // way too inefficent. So instead, we just juck for a few specific types if ((type == typeof(SByte)) || (type == typeof(TypedReference)) || (type == typeof(UInt16)) || (type == typeof(UInt32)) || (type == typeof(UInt64)) || (type == typeof(UIntPtr))) { return false; } return true; } /* * Maps the specified persisted name to its object model equivalent. * The convention is to map all dashes to dots. * For example : Font-Size maps to Font.Size * HeaderStyle-Font-Name maps to HeaderStyle.Font.Name */ internal static string MapNameToPropertyName(string attrName) { return attrName.Replace(PERSIST_CHAR,OM_CHAR); } internal static object LocatePropertyObject(object obj, string mappedName, out string propertyName, bool inDesigner) { object currentObject = obj; Type currentType = obj.GetType(); propertyName = null; int index; int startIndex = 0; // step through the dots of the object model to extract the PropertyInfo // and object on which the property will be set while (startIndex < mappedName.Length) { index = mappedName.IndexOf(OM_CHAR, startIndex); // If we didn't find a separator, we're on the last piece if (index < 0) break; // There is a sub property, so get its info and iterate propertyName = mappedName.Substring(startIndex, index - startIndex); startIndex = index + 1; currentObject = FastPropertyAccessor.GetProperty(currentObject, propertyName, inDesigner); if (currentObject == null) return null; } // Avoid a useless call to Substring if possible if (startIndex > 0) propertyName = mappedName.Substring(startIndex); else propertyName = mappedName; return currentObject; } /* * Walks the object model using the mapped property name to get the * value of an instance's property. */ internal static PropertyDescriptor GetMappedPropertyDescriptor(object obj, string mappedName, out object childObject, out string propertyName, bool inDesigner) { childObject = LocatePropertyObject(obj, mappedName, out propertyName, inDesigner); if (childObject == null) return null; PropertyDescriptorCollection properties = TypeDescriptor.GetProperties(childObject, inDesigner); return properties[propertyName]; } /* * Walks the object model using the mapped property name to set the * value of an instance's property. */ internal static void SetMappedPropertyValue(object obj, string mappedName, object value, bool inDesigner) { string propertyName; object childObj = LocatePropertyObject(obj, mappedName, out propertyName, inDesigner); if (childObj == null) return; FastPropertyAccessor.SetProperty(childObj, propertyName, value, inDesigner); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
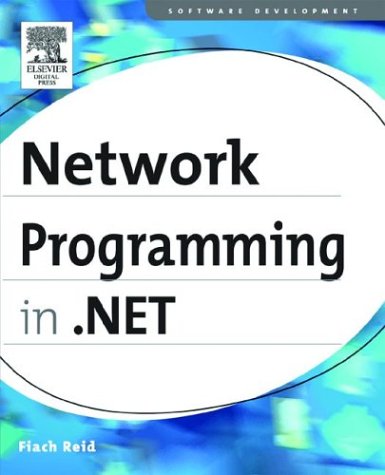
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompositeActivityTypeDescriptorProvider.cs
- ItemCheckEvent.cs
- X509Utils.cs
- CombinedGeometry.cs
- InfocardInteractiveChannelInitializer.cs
- InputElement.cs
- WebPartPersonalization.cs
- XmlSerializerVersionAttribute.cs
- UrlAuthorizationModule.cs
- DataControlField.cs
- LocalServiceSecuritySettingsElement.cs
- TextContainer.cs
- BrowserCapabilitiesFactoryBase.cs
- LocalizedNameDescriptionPair.cs
- CalendarDataBindingHandler.cs
- ToolBar.cs
- DataTableNewRowEvent.cs
- CqlParserHelpers.cs
- CodeCommentStatementCollection.cs
- EditBehavior.cs
- XmlObjectSerializerWriteContextComplex.cs
- Formatter.cs
- PeerNearMe.cs
- TextRenderer.cs
- SettingsPropertyValueCollection.cs
- BeginStoryboard.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- SqlTypesSchemaImporter.cs
- WebPartEventArgs.cs
- FactoryId.cs
- UnsafeNativeMethodsPenimc.cs
- XmlChildEnumerator.cs
- MarkerProperties.cs
- UnmanagedHandle.cs
- ImageKeyConverter.cs
- CodeAssignStatement.cs
- httpstaticobjectscollection.cs
- WebPartChrome.cs
- Quad.cs
- XmlILModule.cs
- ThemeInfoAttribute.cs
- DateTimeParse.cs
- CardSpaceShim.cs
- DiscoveryMessageSequence11.cs
- StringComparer.cs
- SystemIcmpV4Statistics.cs
- HttpChannelFactory.cs
- CompositeControlDesigner.cs
- JoinTreeNode.cs
- CollectionChangeEventArgs.cs
- SqlMethods.cs
- PeerTransportListenAddressValidator.cs
- Point.cs
- UserInitiatedNavigationPermission.cs
- ObjectManager.cs
- TreeNodeSelectionProcessor.cs
- SponsorHelper.cs
- PropertyDescriptorGridEntry.cs
- LineSegment.cs
- CapacityStreamGeometryContext.cs
- HttpListenerPrefixCollection.cs
- ClosureBinding.cs
- ConnectivityStatus.cs
- MatrixStack.cs
- ReadWriteObjectLock.cs
- DeviceSpecificDesigner.cs
- DispatcherEventArgs.cs
- RenderDataDrawingContext.cs
- TextElementCollection.cs
- InternalTypeHelper.cs
- HtmlContainerControl.cs
- Crypto.cs
- XmlSchemaAny.cs
- JournalNavigationScope.cs
- _HTTPDateParse.cs
- MouseDevice.cs
- HuffCodec.cs
- SqlInternalConnectionTds.cs
- DataGrid.cs
- ObjectViewFactory.cs
- EnumUnknown.cs
- ScriptingAuthenticationServiceSection.cs
- LongMinMaxAggregationOperator.cs
- Tokenizer.cs
- PageAdapter.cs
- CollectionType.cs
- Int32Collection.cs
- Soap.cs
- StructuralType.cs
- ISFClipboardData.cs
- DBDataPermission.cs
- CultureSpecificStringDictionary.cs
- HttpApplication.cs
- BaseValidator.cs
- DecoderReplacementFallback.cs
- UnionExpr.cs
- ProjectionCamera.cs
- ScrollChrome.cs
- WebPartActionVerb.cs
- FixUpCollection.cs