Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / Ink / EditBehavior.cs / 1 / EditBehavior.cs
//---------------------------------------------------------------------------- // // File: EditingBehavior.cs // // Description: // Base EditingBehavior for InkCanvas // Features: // // History: // 4/29/2003 waynezen: Rewrote for mid-stroke support // 3/15/2003 samgeo: Created // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows.Input; using System.Windows; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Interop; using System.Windows.Navigation; using System.Windows.Media; namespace MS.Internal.Ink { ////// Base class for all EditingBehaviors in the InkCanvas /// Please see the design detain at http://tabletpc/longhorn/Specs/Mid-Stroke%20and%20Pen%20Cursor%20Dev%20Design.mht /// internal abstract class EditingBehavior { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors ////// Constructor /// /// EditngCoordinator instance /// InkCanvas instance internal EditingBehavior(EditingCoordinator editingCoordinator, InkCanvas inkCanvas) { if (inkCanvas == null) { throw new ArgumentNullException("inkCanvas"); } if (editingCoordinator == null) { throw new ArgumentNullException("editingCoordinator"); } _inkCanvas = inkCanvas; _editingCoordinator = editingCoordinator; } #endregion Constructors //-------------------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------------------- #region Public Methods ////// An internal method which should be called from: /// EditingCoordinator.PushEditingBehavior /// EditingCoordinator.PopEditingBehavior /// The mehod's called when the behavior switching occurs. /// public void Activate() { // Debug verification which will never be compiled into release bits. EditingCoordinator.DebugCheckActiveBehavior(this); // Invoke the virtual OnActivate method. OnActivate(); } ////// An internal method which should be called from: /// EditingCoordinator.PushEditingBehavior /// EditingCoordinator.PopEditingBehavior /// The mehod's called when the behavior switching occurs. /// public void Deactivate() { // Debug verification which will never be compiled into release bits. EditingCoordinator.DebugCheckActiveBehavior(this); // Invoke the virtual OnDeactivate method. OnDeactivate(); } ////// An internal method which should be called from: /// EditingCoordinator.OnInkCanvasStylusUp /// EditingCoordinator.OnInkCanvasLostStylusCapture /// EditingCoordinator.UpdateEditingState /// The mehod's called when the current editing state is committed or discarded. /// /// A flag which indicates either editing is committed or discarded public void Commit(bool commit) { // Debug verification which will never be compiled into release bits. EditingCoordinator.DebugCheckActiveBehavior(this); // Invoke the virtual OnCommit method. OnCommit(commit); } ////// UpdateTransform /// Called by: EditingCoordinator.InvalidateBehaviorCursor /// public void UpdateTransform() { if ( !EditingCoordinator.IsTransformValid(this) ) { OnTransformChanged(); } } #endregion Public Methods //-------------------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------------------- #region Public Properties ////// Retrieve the cursor which is associated to this behavior. /// The method which should be called from: /// EditingCoordinator.InvalidateCursor /// EditingCoordinator.PushEditingBehavior /// EditingCoordinator.PopEditingBehavior /// ///The current cursor public Cursor Cursor { get { // If the cursor instance hasn't been created or is dirty, we will create a new instance. if ( _cachedCursor == null || !EditingCoordinator.IsCursorValid(this) ) { // Invoke the virtual method to get cursor. Then cache it. _cachedCursor = GetCurrentCursor(); } return _cachedCursor; } } #endregion Public Properties //------------------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------------------- #region Protected Methods ////// Called when the EditingBehavior is activated. EditingBehaviors /// should register for event handlers in this call /// Called from: /// EditingBehavior.Activate /// protected abstract void OnActivate(); ////// Called when the EditingBehavior is deactivated. EditingBehaviors /// should unregister from event handlers in this call /// Called from: /// EditingBehavior.Deactivate /// protected abstract void OnDeactivate(); ////// Called when the user editing is committed or discarded. /// Called from: /// EditingBehavior.Commit /// /// A flag which indicates either editing is committed or discarded protected abstract void OnCommit(bool commit); ////// Called when the new cursor instance is required. /// Called from: /// EditingBehavior.GetCursor /// ///protected abstract Cursor GetCurrentCursor(); /// /// Unload the dynamic behavior. The method should be only called from: /// SelectionEditingBehavior /// LassoSelectionBehavior /// protected void SelfDeactivate() { EditingCoordinator.DeactivateDynamicBehavior(); } ////// Calculate the transform which is accumalated with the InkCanvas' XXXTransform properties. /// ///protected Matrix GetElementTransformMatrix() { Transform layoutTransform = this.InkCanvas.LayoutTransform; Transform renderTransform = this.InkCanvas.RenderTransform; Matrix xf = layoutTransform.Value; xf *= renderTransform.Value; return xf; } /// /// OnTransformChanged /// protected virtual void OnTransformChanged() { // Defer to derived. } #endregion Protected Methods //------------------------------------------------------------------------------- // // Protected Properties // //------------------------------------------------------------------------------- #region Protected Properties ////// Provides access to the InkCanvas this EditingBehavior is attached to /// ///protected InkCanvas InkCanvas { get { return _inkCanvas; } } /// /// Provides access to the EditingStack this EditingBehavior is on /// ///protected EditingCoordinator EditingCoordinator { get { return _editingCoordinator; } } #endregion Protected Properties //------------------------------------------------------------------------------- // // Protected Fields // //-------------------------------------------------------------------------------- #region Private Fields /// /// The InkCanvas this EditingBehavior is operating on /// private InkCanvas _inkCanvas; ////// The EditingStack this EditingBehavior is on /// private EditingCoordinator _editingCoordinator; ////// Fields related to the cursor /// private Cursor _cachedCursor; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: EditingBehavior.cs // // Description: // Base EditingBehavior for InkCanvas // Features: // // History: // 4/29/2003 waynezen: Rewrote for mid-stroke support // 3/15/2003 samgeo: Created // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows.Input; using System.Windows; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Interop; using System.Windows.Navigation; using System.Windows.Media; namespace MS.Internal.Ink { ////// Base class for all EditingBehaviors in the InkCanvas /// Please see the design detain at http://tabletpc/longhorn/Specs/Mid-Stroke%20and%20Pen%20Cursor%20Dev%20Design.mht /// internal abstract class EditingBehavior { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors ////// Constructor /// /// EditngCoordinator instance /// InkCanvas instance internal EditingBehavior(EditingCoordinator editingCoordinator, InkCanvas inkCanvas) { if (inkCanvas == null) { throw new ArgumentNullException("inkCanvas"); } if (editingCoordinator == null) { throw new ArgumentNullException("editingCoordinator"); } _inkCanvas = inkCanvas; _editingCoordinator = editingCoordinator; } #endregion Constructors //-------------------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------------------- #region Public Methods ////// An internal method which should be called from: /// EditingCoordinator.PushEditingBehavior /// EditingCoordinator.PopEditingBehavior /// The mehod's called when the behavior switching occurs. /// public void Activate() { // Debug verification which will never be compiled into release bits. EditingCoordinator.DebugCheckActiveBehavior(this); // Invoke the virtual OnActivate method. OnActivate(); } ////// An internal method which should be called from: /// EditingCoordinator.PushEditingBehavior /// EditingCoordinator.PopEditingBehavior /// The mehod's called when the behavior switching occurs. /// public void Deactivate() { // Debug verification which will never be compiled into release bits. EditingCoordinator.DebugCheckActiveBehavior(this); // Invoke the virtual OnDeactivate method. OnDeactivate(); } ////// An internal method which should be called from: /// EditingCoordinator.OnInkCanvasStylusUp /// EditingCoordinator.OnInkCanvasLostStylusCapture /// EditingCoordinator.UpdateEditingState /// The mehod's called when the current editing state is committed or discarded. /// /// A flag which indicates either editing is committed or discarded public void Commit(bool commit) { // Debug verification which will never be compiled into release bits. EditingCoordinator.DebugCheckActiveBehavior(this); // Invoke the virtual OnCommit method. OnCommit(commit); } ////// UpdateTransform /// Called by: EditingCoordinator.InvalidateBehaviorCursor /// public void UpdateTransform() { if ( !EditingCoordinator.IsTransformValid(this) ) { OnTransformChanged(); } } #endregion Public Methods //-------------------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------------------- #region Public Properties ////// Retrieve the cursor which is associated to this behavior. /// The method which should be called from: /// EditingCoordinator.InvalidateCursor /// EditingCoordinator.PushEditingBehavior /// EditingCoordinator.PopEditingBehavior /// ///The current cursor public Cursor Cursor { get { // If the cursor instance hasn't been created or is dirty, we will create a new instance. if ( _cachedCursor == null || !EditingCoordinator.IsCursorValid(this) ) { // Invoke the virtual method to get cursor. Then cache it. _cachedCursor = GetCurrentCursor(); } return _cachedCursor; } } #endregion Public Properties //------------------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------------------- #region Protected Methods ////// Called when the EditingBehavior is activated. EditingBehaviors /// should register for event handlers in this call /// Called from: /// EditingBehavior.Activate /// protected abstract void OnActivate(); ////// Called when the EditingBehavior is deactivated. EditingBehaviors /// should unregister from event handlers in this call /// Called from: /// EditingBehavior.Deactivate /// protected abstract void OnDeactivate(); ////// Called when the user editing is committed or discarded. /// Called from: /// EditingBehavior.Commit /// /// A flag which indicates either editing is committed or discarded protected abstract void OnCommit(bool commit); ////// Called when the new cursor instance is required. /// Called from: /// EditingBehavior.GetCursor /// ///protected abstract Cursor GetCurrentCursor(); /// /// Unload the dynamic behavior. The method should be only called from: /// SelectionEditingBehavior /// LassoSelectionBehavior /// protected void SelfDeactivate() { EditingCoordinator.DeactivateDynamicBehavior(); } ////// Calculate the transform which is accumalated with the InkCanvas' XXXTransform properties. /// ///protected Matrix GetElementTransformMatrix() { Transform layoutTransform = this.InkCanvas.LayoutTransform; Transform renderTransform = this.InkCanvas.RenderTransform; Matrix xf = layoutTransform.Value; xf *= renderTransform.Value; return xf; } /// /// OnTransformChanged /// protected virtual void OnTransformChanged() { // Defer to derived. } #endregion Protected Methods //------------------------------------------------------------------------------- // // Protected Properties // //------------------------------------------------------------------------------- #region Protected Properties ////// Provides access to the InkCanvas this EditingBehavior is attached to /// ///protected InkCanvas InkCanvas { get { return _inkCanvas; } } /// /// Provides access to the EditingStack this EditingBehavior is on /// ///protected EditingCoordinator EditingCoordinator { get { return _editingCoordinator; } } #endregion Protected Properties //------------------------------------------------------------------------------- // // Protected Fields // //-------------------------------------------------------------------------------- #region Private Fields /// /// The InkCanvas this EditingBehavior is operating on /// private InkCanvas _inkCanvas; ////// The EditingStack this EditingBehavior is on /// private EditingCoordinator _editingCoordinator; ////// Fields related to the cursor /// private Cursor _cachedCursor; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
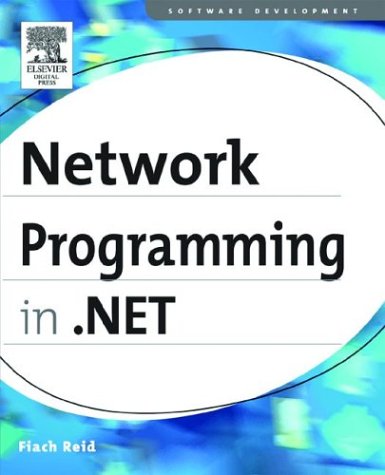
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FunctionDetailsReader.cs
- SmiEventStream.cs
- GroupBoxDesigner.cs
- SingleStorage.cs
- StaticFileHandler.cs
- ButtonBaseAutomationPeer.cs
- TypeElement.cs
- ScriptServiceAttribute.cs
- TextProviderWrapper.cs
- ValueSerializerAttribute.cs
- SafeProcessHandle.cs
- TraceContext.cs
- XmlTextAttribute.cs
- CodeTypeParameter.cs
- DirectoryInfo.cs
- ContextDataSourceView.cs
- MailDefinition.cs
- ToolStripItemClickedEventArgs.cs
- NetworkInformationPermission.cs
- SystemResourceKey.cs
- Font.cs
- ItemList.cs
- GridViewColumnHeader.cs
- AddInActivator.cs
- SocketPermission.cs
- BindingList.cs
- CodeTypeParameter.cs
- WSFederationHttpSecurityMode.cs
- HttpContext.cs
- FixedSOMTableRow.cs
- sqlpipe.cs
- LiteralTextContainerControlBuilder.cs
- Comparer.cs
- Normalization.cs
- PositiveTimeSpanValidator.cs
- NetworkAddressChange.cs
- localization.cs
- PropVariant.cs
- CatalogPart.cs
- SchemaImporter.cs
- ColumnHeaderCollectionEditor.cs
- DllNotFoundException.cs
- securestring.cs
- SplashScreenNativeMethods.cs
- StringBuilder.cs
- KeyedHashAlgorithm.cs
- RowCache.cs
- RevocationPoint.cs
- DataGridState.cs
- TdsParameterSetter.cs
- OpenCollectionAsyncResult.cs
- UnlockInstanceCommand.cs
- BindingRestrictions.cs
- AsyncPostBackTrigger.cs
- NullRuntimeConfig.cs
- LinkUtilities.cs
- ModifierKeysConverter.cs
- ExponentialEase.cs
- COM2PictureConverter.cs
- MessageDecoder.cs
- PeerApplication.cs
- CompilerGeneratedAttribute.cs
- RawUIStateInputReport.cs
- ClientScriptManager.cs
- XmlSerializerSection.cs
- FilteredSchemaElementLookUpTable.cs
- SequenceDesigner.xaml.cs
- DateTimeConstantAttribute.cs
- ErrorStyle.cs
- SiteMapSection.cs
- ToolStripDropTargetManager.cs
- XmlWrappingReader.cs
- EntityDataSourceChangedEventArgs.cs
- TypedDataSetSchemaImporterExtension.cs
- MetadataSource.cs
- StrongNameIdentityPermission.cs
- StrongNameUtility.cs
- NetNamedPipeSecurityMode.cs
- StringExpressionSet.cs
- SizeKeyFrameCollection.cs
- ResourceAssociationSetEnd.cs
- EdmProperty.cs
- QueryContinueDragEvent.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- TextEndOfParagraph.cs
- InkPresenter.cs
- SvcFileManager.cs
- ReadOnlyPropertyMetadata.cs
- HttpRawResponse.cs
- NavigateUrlConverter.cs
- ExpressionCopier.cs
- QuerySetOp.cs
- ObjectSpanRewriter.cs
- TextMarkerSource.cs
- connectionpool.cs
- ICspAsymmetricAlgorithm.cs
- PreProcessor.cs
- RootAction.cs
- ConfigXmlCDataSection.cs
- DataGridViewTextBoxCell.cs