Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Configuration / SiteMapSection.cs / 2 / SiteMapSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class SiteMapSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propDefaultProvider = new ConfigurationProperty("defaultProvider", typeof(string), "AspNetXmlSiteMapProvider", null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnabled = new ConfigurationProperty("enabled", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProviders = new ConfigurationProperty("providers", typeof(ProviderSettingsCollection), null, ConfigurationPropertyOptions.None); private SiteMapProviderCollection _siteMapProviders; static SiteMapSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propDefaultProvider); _properties.Add(_propEnabled); _properties.Add(_propProviders); } public SiteMapSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("defaultProvider", DefaultValue = "AspNetXmlSiteMapProvider")] [StringValidator(MinLength = 1)] public string DefaultProvider { get { return (string)base[_propDefaultProvider]; } set { base[_propDefaultProvider] = value; } } [ConfigurationProperty("enabled", DefaultValue = true)] public bool Enabled { get { return (bool)base[_propEnabled]; } set { base[_propEnabled] = value; } } [ConfigurationProperty("providers")] public ProviderSettingsCollection Providers { get { return (ProviderSettingsCollection)base[_propProviders]; } } internal SiteMapProviderCollection ProvidersInternal { get { if (_siteMapProviders == null) { lock (this) { if (_siteMapProviders == null) { SiteMapProviderCollection siteMapProviders = new SiteMapProviderCollection(); ProvidersHelper.InstantiateProviders(Providers, siteMapProviders, typeof(SiteMapProvider)); _siteMapProviders = siteMapProviders; } } } return _siteMapProviders; } } internal void ValidateDefaultProvider() { if (!String.IsNullOrEmpty(DefaultProvider)) // make sure the specified provider has a provider entry in the collection { if (Providers[DefaultProvider] == null) { throw new ConfigurationErrorsException( SR.GetString(SR.Config_provider_must_exist, DefaultProvider), ElementInformation.Properties[_propDefaultProvider.Name].Source, ElementInformation.Properties[_propDefaultProvider.Name].LineNumber); } } } } // class SiteMapSection }
Link Menu
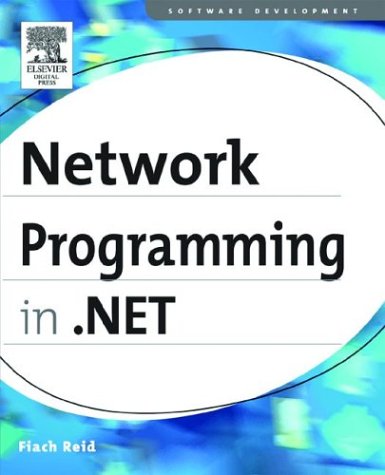
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SecurityChannelListener.cs
- Literal.cs
- TextEndOfSegment.cs
- DataGridItemEventArgs.cs
- DesignerSerializationOptionsAttribute.cs
- RegexFCD.cs
- HTMLTextWriter.cs
- ExpressionBuilderContext.cs
- AddingNewEventArgs.cs
- WsdlServiceChannelBuilder.cs
- SoapInteropTypes.cs
- TypeContext.cs
- EnvelopedPkcs7.cs
- QualifiedCellIdBoolean.cs
- XmlSchemaComplexType.cs
- Popup.cs
- SequenceDesignerAccessibleObject.cs
- BevelBitmapEffect.cs
- SafeNativeMethods.cs
- RowVisual.cs
- IdleTimeoutMonitor.cs
- SafeMarshalContext.cs
- TouchDevice.cs
- URI.cs
- SoapObjectWriter.cs
- ChangesetResponse.cs
- Pen.cs
- TaskFormBase.cs
- AutomationFocusChangedEventArgs.cs
- WindowsFormsSectionHandler.cs
- XmlSchemaAttributeGroupRef.cs
- ConstraintStruct.cs
- unsafeIndexingFilterStream.cs
- ActivityBuilderHelper.cs
- IntegerCollectionEditor.cs
- DbProviderServices.cs
- BooleanStorage.cs
- SafeRightsManagementQueryHandle.cs
- ContentValidator.cs
- ClientScriptItem.cs
- XPathBinder.cs
- SspiSafeHandles.cs
- UnwrappedTypesXmlSerializerManager.cs
- LinqDataSourceContextData.cs
- PassportAuthenticationEventArgs.cs
- TdsParserSafeHandles.cs
- HttpResponse.cs
- DescendentsWalker.cs
- BrowserDefinition.cs
- CombinedGeometry.cs
- BoolExpr.cs
- StatusStrip.cs
- SelectedDatesCollection.cs
- TargetInvocationException.cs
- ArgIterator.cs
- MaxSessionCountExceededException.cs
- SQLDateTime.cs
- ButtonFieldBase.cs
- OpacityConverter.cs
- TypeDescriptionProviderAttribute.cs
- dataprotectionpermissionattribute.cs
- XmlSchemaImport.cs
- PublisherMembershipCondition.cs
- DataTableMappingCollection.cs
- MaskedTextProvider.cs
- ChildTable.cs
- DataServiceClientException.cs
- Panel.cs
- WaitForChangedResult.cs
- EntityDataSourceWrapperCollection.cs
- MonitoringDescriptionAttribute.cs
- AuthenticationModuleElement.cs
- RepeaterItem.cs
- XmlSignificantWhitespace.cs
- PackageRelationshipSelector.cs
- ClientTargetCollection.cs
- XmlParserContext.cs
- AdapterUtil.cs
- PenContext.cs
- MediaContext.cs
- StickyNoteHelper.cs
- IntPtr.cs
- MouseWheelEventArgs.cs
- StringExpressionSet.cs
- DelegateSerializationHolder.cs
- CoreSwitches.cs
- ProxyHelper.cs
- ELinqQueryState.cs
- IMembershipProvider.cs
- UpdateCommandGenerator.cs
- XmlCodeExporter.cs
- MemberHolder.cs
- RadialGradientBrush.cs
- PackWebRequestFactory.cs
- TextRangeProviderWrapper.cs
- MailSettingsSection.cs
- XmlILConstructAnalyzer.cs
- PrePrepareMethodAttribute.cs
- Hash.cs
- SqlDataSourceView.cs