Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / AutomationFocusChangedEventArgs.cs / 1 / AutomationFocusChangedEventArgs.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Focus event args class (Client Side) // // History: // 06/17/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Delegate to handle focus change events /// #if (INTERNAL_COMPILE) internal delegate void AutomationFocusChangedEventHandler( object sender, AutomationFocusChangedEventArgs e ); #else public delegate void AutomationFocusChangedEventHandler( object sender, AutomationFocusChangedEventArgs e ); #endif // AutomationFocusChangedEventArgs has two distinct uses: // - when used by provider code, it is basically a wrapper for the previous focus provider, // which gets passed through as a parameter to the UiaCore API. // - on the client side, it is used to deliver focus events to the client - these events // may originate within the client via focus winevents, or may be the result of notifications // from core. In both cases, the ClientApi code creates an instance of the args, with an // AutomationElement indicating the previous focus. ////// Focus event args class /// #if (INTERNAL_COMPILE) internal class AutomationFocusChangedEventArgs : AutomationEventArgs #else public class AutomationFocusChangedEventArgs : AutomationEventArgs #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Client-side constructor for focus event args. /// /// Indicates object id. /// Indicates id of the child. public AutomationFocusChangedEventArgs(int idObject, int idChild) : base(AutomationElement.AutomationFocusChangedEvent) { _idObject = idObject; _idChild = idChild; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Returns the object id /// public int ObjectId { get { return _idObject; } } ////// Returns the child id /// public int ChildId { get { return _idChild; } } #endregion Public Properties //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields private int _idObject; // private int _idChild; // #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Focus event args class (Client Side) // // History: // 06/17/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Delegate to handle focus change events /// #if (INTERNAL_COMPILE) internal delegate void AutomationFocusChangedEventHandler( object sender, AutomationFocusChangedEventArgs e ); #else public delegate void AutomationFocusChangedEventHandler( object sender, AutomationFocusChangedEventArgs e ); #endif // AutomationFocusChangedEventArgs has two distinct uses: // - when used by provider code, it is basically a wrapper for the previous focus provider, // which gets passed through as a parameter to the UiaCore API. // - on the client side, it is used to deliver focus events to the client - these events // may originate within the client via focus winevents, or may be the result of notifications // from core. In both cases, the ClientApi code creates an instance of the args, with an // AutomationElement indicating the previous focus. ////// Focus event args class /// #if (INTERNAL_COMPILE) internal class AutomationFocusChangedEventArgs : AutomationEventArgs #else public class AutomationFocusChangedEventArgs : AutomationEventArgs #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Client-side constructor for focus event args. /// /// Indicates object id. /// Indicates id of the child. public AutomationFocusChangedEventArgs(int idObject, int idChild) : base(AutomationElement.AutomationFocusChangedEvent) { _idObject = idObject; _idChild = idChild; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Returns the object id /// public int ObjectId { get { return _idObject; } } ////// Returns the child id /// public int ChildId { get { return _idChild; } } #endregion Public Properties //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields private int _idObject; // private int _idChild; // #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
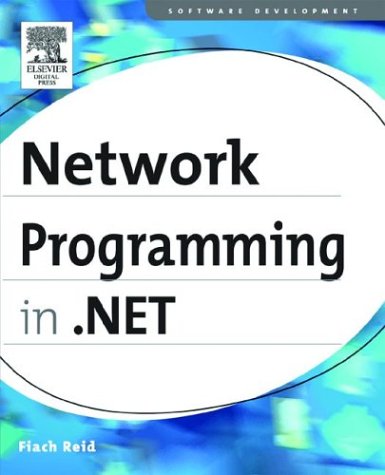
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TransformGroup.cs
- EntityStoreSchemaGenerator.cs
- MenuItem.cs
- BamlRecords.cs
- DataTableMapping.cs
- VSWCFServiceContractGenerator.cs
- ParserContext.cs
- XPathDocumentBuilder.cs
- TextPenaltyModule.cs
- MetadataUtilsSmi.cs
- SqlDataSourceQueryConverter.cs
- NullableIntAverageAggregationOperator.cs
- SecurityTokenTypes.cs
- RemoteHelper.cs
- CompiledQuery.cs
- XmlSchemaSimpleTypeUnion.cs
- DeferrableContent.cs
- SessionState.cs
- DataGridViewToolTip.cs
- BindingExpressionBase.cs
- CategoryEditor.cs
- Internal.cs
- LogRestartAreaEnumerator.cs
- webclient.cs
- DeploymentSectionCache.cs
- TypeUnloadedException.cs
- TranslateTransform.cs
- HtmlTableCellCollection.cs
- SuppressIldasmAttribute.cs
- SoapServerMessage.cs
- CodeAssignStatement.cs
- RIPEMD160Managed.cs
- HtmlAnchor.cs
- GenerateHelper.cs
- CharConverter.cs
- HTTPNotFoundHandler.cs
- Stack.cs
- Query.cs
- LayoutTableCell.cs
- ErrorRuntimeConfig.cs
- Operator.cs
- XamlPointCollectionSerializer.cs
- ProfileGroupSettingsCollection.cs
- Attributes.cs
- LazyLoadBehavior.cs
- SQLMembershipProvider.cs
- SQLMoneyStorage.cs
- DesignerView.cs
- TextTreeRootTextBlock.cs
- ListChangedEventArgs.cs
- ColumnMap.cs
- X509ChainElement.cs
- ReachDocumentReferenceSerializerAsync.cs
- BindingBase.cs
- SharedHttpsTransportManager.cs
- MetadataItemEmitter.cs
- TextWriter.cs
- ResourceDescriptionAttribute.cs
- Dictionary.cs
- Base64Decoder.cs
- StylusPointPropertyId.cs
- ServiceHttpHandlerFactory.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- ContentPosition.cs
- WindowsScrollBar.cs
- SignatureHelper.cs
- BCryptNative.cs
- DateTime.cs
- SmtpFailedRecipientsException.cs
- CompileLiteralTextParser.cs
- SingleBodyParameterMessageFormatter.cs
- UnlockInstanceAsyncResult.cs
- GridViewUpdateEventArgs.cs
- XhtmlConformanceSection.cs
- PrimaryKeyTypeConverter.cs
- TextViewDesigner.cs
- CapabilitiesSection.cs
- OdbcInfoMessageEvent.cs
- ProfileGroupSettingsCollection.cs
- FileDataSourceCache.cs
- AliasedExpr.cs
- ToolStripTextBox.cs
- OutOfProcStateClientManager.cs
- MapPathBasedVirtualPathProvider.cs
- WebDisplayNameAttribute.cs
- DesignerValidatorAdapter.cs
- ConstrainedDataObject.cs
- CompilerState.cs
- DtcInterfaces.cs
- FlowDocumentScrollViewer.cs
- UnsafeNativeMethods.cs
- CompilerScope.cs
- NavigationEventArgs.cs
- AsyncPostBackTrigger.cs
- RequestQueue.cs
- HebrewNumber.cs
- Vector.cs
- ConvertEvent.cs
- DecoderReplacementFallback.cs
- PropertySourceInfo.cs