Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / MS / Internal / TextFormatting / TextPenaltyModule.cs / 1 / TextPenaltyModule.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextPenaltyModule.cs // // Contents: Critical handle wrapping unmanaged text penalty module for // penalty calculation of optimal paragraph vis PTS direct access. // // Spec: [....]/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 4-4-2006 [....] ([....]) // //----------------------------------------------------------------------- using System; using System.Security; using System.Windows.Media; using System.Windows.Media.TextFormatting; using System.Runtime.InteropServices; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.TextFormatting { ////// Critical handle wrapper of unmanaged text penalty module. This class /// is used exclusively by Framework thru friend-access. It provides direct /// access to the underlying dangerous handle to the unmanaged resource whose /// lifetime is bound to the the underlying LS context. /// [FriendAccessAllowed] // used by Framework internal sealed class TextPenaltyModule : IDisposable { private SecurityCriticalDataForSet_ploPenaltyModule; // Pointer to LS penalty module private bool _isDisposed; /// /// This constructor is called by PInvoke when returning the critical handle /// ////// Critical - as this calls the setter of _ploPenaltyModule. /// Safe - as it does not set the value arbitrarily from the value it receives from caller. /// [SecurityCritical, SecurityTreatAsSafe] internal TextPenaltyModule(SecurityCriticalDataForSetploc) { IntPtr ploPenaltyModule; LsErr lserr = UnsafeNativeMethods.LoAcquirePenaltyModule(ploc.Value, out ploPenaltyModule); if (lserr != LsErr.None) { TextFormatterContext.ThrowExceptionFromLsError(SR.Get(SRID.AcquirePenaltyModuleFailure, lserr), lserr); } _ploPenaltyModule.Value = ploPenaltyModule; } /// /// Finalize penalty module /// ~TextPenaltyModule() { Dispose(false); } ////// Explicitly clean up penalty module /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Critical - as this calls method to dispose unmanaged penalty module. /// Safe - as it does not arbitrarily set critical data. /// [SecurityCritical, SecurityTreatAsSafe] private void Dispose(bool disposing) { if (_ploPenaltyModule.Value != IntPtr.Zero) { UnsafeNativeMethods.LoDisposePenaltyModule(_ploPenaltyModule.Value); _ploPenaltyModule.Value = IntPtr.Zero; _isDisposed = true; GC.KeepAlive(this); } } ////// This method should only be called by Framework to authorize direct access to /// unsafe LS penalty module for exclusive use of PTS during optimal paragraph /// penalty calculation. /// ////// Critical - as this returns pointer to unmanaged memory owned by LS. /// [SecurityCritical] internal IntPtr DangerousGetHandle() { if (_isDisposed) { throw new ObjectDisposedException(SR.Get(SRID.TextPenaltyModuleHasBeenDisposed)); } IntPtr penaltyModuleInternalHandle; LsErr lserr = UnsafeNativeMethods.LoGetPenaltyModuleInternalHandle(_ploPenaltyModule.Value, out penaltyModuleInternalHandle); if (lserr != LsErr.None) TextFormatterContext.ThrowExceptionFromLsError(SR.Get(SRID.GetPenaltyModuleHandleFailure, lserr), lserr); GC.KeepAlive(this); return penaltyModuleInternalHandle; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
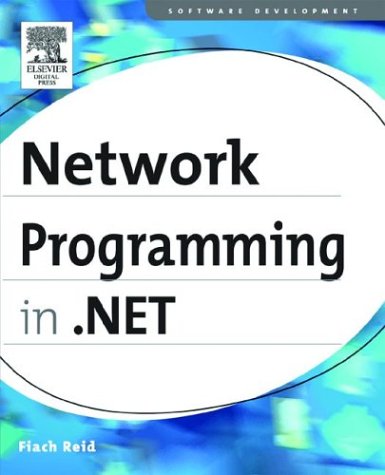
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DynamicValidatorEventArgs.cs
- XPathParser.cs
- IndexOutOfRangeException.cs
- validation.cs
- TextRenderer.cs
- DataGridViewCellCollection.cs
- WindowAutomationPeer.cs
- SqlGatherConsumedAliases.cs
- RenderCapability.cs
- HierarchicalDataBoundControl.cs
- HorizontalAlignConverter.cs
- DescendentsWalker.cs
- StdValidatorsAndConverters.cs
- RoutedCommand.cs
- LogEntryDeserializer.cs
- Exception.cs
- GridItemPattern.cs
- TypedDataSourceCodeGenerator.cs
- EventLevel.cs
- PeerNameRegistration.cs
- SqlXmlStorage.cs
- BookmarkUndoUnit.cs
- regiisutil.cs
- SafeCryptoKeyHandle.cs
- SharedStream.cs
- Underline.cs
- SyndicationLink.cs
- Timer.cs
- EntitySqlQueryState.cs
- Substitution.cs
- SynchronizedInputAdaptor.cs
- FontNameConverter.cs
- Form.cs
- _IPv4Address.cs
- LightweightCodeGenerator.cs
- WindowsGraphics2.cs
- ReadOnlyCollectionBase.cs
- WbemException.cs
- Semaphore.cs
- RefreshEventArgs.cs
- EtwTrackingParticipant.cs
- DataContractSerializerFaultFormatter.cs
- ExternalDataExchangeService.cs
- SelectedDatesCollection.cs
- WebAdminConfigurationHelper.cs
- PackageDigitalSignatureManager.cs
- ClientConfigurationSystem.cs
- SrgsElement.cs
- SetUserLanguageRequest.cs
- ZipIOFileItemStream.cs
- ImageSourceConverter.cs
- RuntimeIdentifierPropertyAttribute.cs
- DesignerEditorPartChrome.cs
- DeviceSpecificDesigner.cs
- FormatConvertedBitmap.cs
- PrivilegedConfigurationManager.cs
- NameObjectCollectionBase.cs
- AttributeInfo.cs
- EventLogEntryCollection.cs
- SafeEventLogReadHandle.cs
- ButtonPopupAdapter.cs
- CodeMemberEvent.cs
- IPHostEntry.cs
- Subtree.cs
- FixedTextContainer.cs
- SByte.cs
- RemotingConfigParser.cs
- ValueSerializerAttribute.cs
- SystemTcpConnection.cs
- InstanceDescriptor.cs
- ScalarConstant.cs
- DataGridColumnHeader.cs
- base64Transforms.cs
- Condition.cs
- Point3DCollection.cs
- ICspAsymmetricAlgorithm.cs
- TraceHandler.cs
- KeyFrames.cs
- ProcessHost.cs
- DisplayMemberTemplateSelector.cs
- BitmapSizeOptions.cs
- HttpSocketManager.cs
- MobileSysDescriptionAttribute.cs
- SpeechSeg.cs
- HeaderedItemsControl.cs
- ColumnMapTranslator.cs
- TypeDelegator.cs
- SingleBodyParameterMessageFormatter.cs
- ExtensionQuery.cs
- KeyGestureValueSerializer.cs
- ScriptRegistrationManager.cs
- GenerateDerivedKeyRequest.cs
- RequestCache.cs
- ProfileGroupSettings.cs
- NameValuePair.cs
- TemplateAction.cs
- AutoScrollExpandMessageFilter.cs
- CustomExpressionEventArgs.cs
- PreloadedPackages.cs
- ObjectParameter.cs